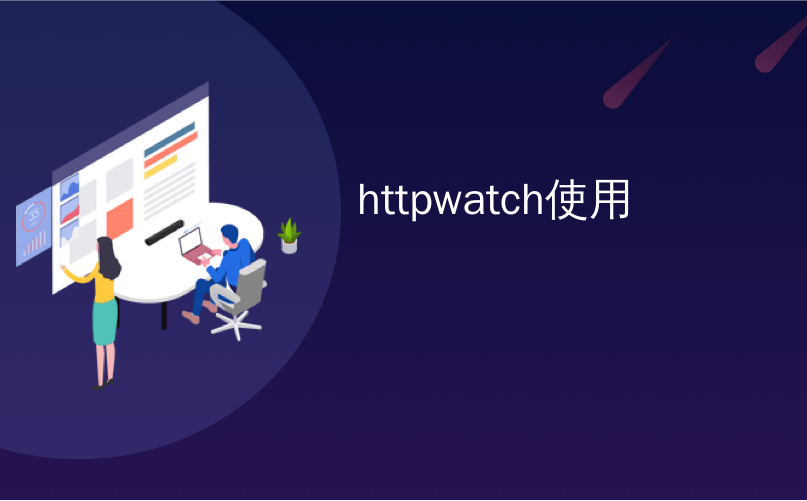
httpwatch使用
In part 1 I demonstrated how you can use PHP to script and automate HTTPWatch. And how you can get data back, either reading the API docs or using a quick HAR hack to get a lot of data in one go.
在第1部分中,我演示了如何使用PHP编写脚本并自动执行HTTPWatch。 以及如何获取数据,无论是阅读API文档还是使用快速的HAR hack一次获取大量数据。
Now I want to share a little class I wrote to make all that a little easier.
现在,我想分享我写的一堂小课,以使所有这一切变得容易些。
The code is here on GitHub.
代码在GitHub上。
基本用法 (Basic usage)
Open IE, navigate, close:
打开IE,浏览并关闭:
$http = new HTTPWatch();
$http->go('http://phpied.com/');
$http->done();
To do the same in Firefox, just pass "ff" to the constructor:
要在Firefox中执行相同的操作,只需将“ ff”传递给构造函数:
$http = new HTTPWatch('ff');
$http->go('http://phpied.com/');
$http->done();
The constructor accepts a second param with options, like empty cache, hide browser (ie only), etc, largely underused for the time being.
构造函数接受带有选项的第二个参数,例如空缓存,隐藏浏览器(即,仅)等,目前暂时未充分使用。
处理HTTPWatch插件 (Handle to the HTTPWatch plugin)
After $http = new HTTPWatch();
a watch
property will be added to $http
. This is the HTTPWatch instance which gives you access to all its APIs, so you can do e.g.
在$http = new HTTPWatch();
一个watch
属性将被添加到$http
。 这是HTTPWatch实例,可让您访问其所有API,因此您可以执行例如
$summary = $http->watch->Log->Entries->Summary;
数据输出 (Data out)
My main motivation behind this class, other than simpler api, has been to provide the ability to just dump all the data that HTTPWatch collects in a quick print_r()
. That has been a challenge with the COM PHP bridge, but I found a hack around it. In any event, most of the HTTPWatch API I've exported to a second PHP file - the HTTPWatchAPI.php script. (This is an auto-generated file, created by another script, but let's leave that out for now.)
除了更简单的api之外,我在该类背后的主要目的是提供能够将HTTPWatch收集的所有数据转储到快速print_r()
。 对于COM PHP桥,这一直是一个挑战,但是我发现它周围存在一个黑手。 无论如何,我已将大多数HTTPWatch API导出到第二个PHP文件-HTTPWatchAPI.php脚本。 (这是一个自动生成的文件,由另一个脚本创建,但现在暂时不进行介绍。)
So after you've navigated to a page you have two convenient methods to grab a bunch of data from HTTPWatch. The first is:
因此,导航到页面后,您可以通过两种便捷的方法从HTTPWatch捕获大量数据。 第一个是:
$http->getSummary();
This gives a summary stats for the http observation session. The second is
这提供了http观察会话的摘要统计信息。 第二个是
$http->getEntries();
It gives you details about every HTTPWatch log entry - be it cached or an actual HTTP request.
它为您提供了有关每个HTTPWatch日志条目的详细信息-无论是缓存还是实际的HTTP请求。
Here's an example of what getSummary() can give you. Here's how this file was generated:
这是getSummary()可以为您提供的示例。 此文件的生成方式如下:
$http = new HTTPWatch();
$http->go("http://google.com");
print_r($http->getSummary());
$http->done();
And here's some output print_r()-ed from getEntries(). Here's the code that produced it:
这是getEntries()的一些输出print_r()-ed 。 这是产生它的代码:
$http = new HTTPWatch();
$http->go("http://google.com");
print_r($http->getEntries());
$http->done();
If you look carefully at the dump, you may notice something like [Stream] => [BYTESTREAM]
. Most of the times you don't need the raw HTTP streams (gzipped, chunked, etc), but you can get them if you want by setting:
如果仔细查看转储,您可能会注意到[Stream] => [BYTESTREAM]
。 大多数时候,您不需要原始HTTP流(压缩,分块等),但是如果需要,可以通过设置以下内容来获取它们:
$http->skipStreams = false;
Here's the same google.com example, this time including the raw streams. And the code:
这是相同的google.com示例,这次包括原始流。 和代码:
$http = new HTTPWatch();
$http->go("http://google.com");
$http->skipStreams = false;
print_r($http->getEntries());
$http->done();
免费与付费 (Free vs. paid)
One pain with HTTPWatch is that the free version has restrictions. The summary for example doesn't include TimingSummaries
and WarningSummaries
properties. The entry log has almost nothing - no headers or content or streams. My class handles that by giving you as much as it can. If you're using the free version, it will return the limited data for the restricted URLs, but still the full data for those URLs that HTTPWatch's demo version allows - the top Alexa sites.
HTTPWatch的一个难题是免费版本有限制。 例如,摘要不包含TimingSummaries
和WarningSummaries
属性。 条目日志几乎没有内容-没有标题,内容或流。 我的课通过给你尽可能多的东西来解决这个问题。 如果您使用的是免费版本,它将返回受限URL的受限数据,但仍返回HTTPWatch演示版本允许的URL的完整数据-顶级Alexa网站。
So here's a dump of visiting http://givepngachance.com with my free HTTPWatch edition.
因此,这里有我免费的HTTPWatch版本访问http://givepngachance.com的转储。
The data has restricted information about givepngachance.com URL but full data related to the embeded youtube.com resource.
数据具有关于Givepngachance.com URL的受限信息,但是与嵌入的youtube.com资源相关的完整数据。
The code:
代码:
$http = new HTTPWatch();
$http->go("http://givepngachance.com");
print_r($http->getEntries());
$http->done();
再次与视频 (Again with the video)
If you've read part 1, you've probably seen the video, but here's the link again (try the HD version). This is a screencapture of loading FF and IE using my new class. The code that produced it is:
如果您已经阅读了第1部分,则可能已经看过视频了,但是这里又是链接(尝试HD版本)。 这是使用我的新类加载FF和IE的屏幕截图。 产生它的代码是:
$ie = new HTTPWatch();
$ie->go('http://google.com/');
$sum = $ie->getSummary();
$ff = new HTTPWatch('ff');
$ff->go('http://google.com/');
$sumff = $ff->getSummary();
echo "\nRun 1 ";
echo $ie->watch->Log->BrowserName, ' ';
echo $ie->watch->Log->BrowserVersion;
echo "\nSent: ", $sum['BytesSent'], "; Received: ", $sum['BytesReceived'];
echo "\nRun 2 ";
echo $ff->watch->Log->BrowserName, ' ';
echo $ff->watch->Log->BrowserVersion;
echo "\nSent: ", $sumff['BytesSent'], "; Received: ", $sumff['BytesReceived'];
$ie->done();
$ff->done();
As you can see I'm accessing the HTTPWatch plugin object ($http->watch
) directly to get the browser version and name. I didn't think this was worth wrapping in a more convenient API the way I did with getSummary()
and getEntries()
.
如您所见,我正在直接访问HTTPWatch插件对象( $http->watch
)以获取浏览器的版本和名称。 我认为这不值得像我对getSummary()
和getEntries()
所做的那样包装在更方便的API中。
The result of this is:
结果是:
$ php examples.php
Run 1 Internet Explorer 6.0.2900.5512
Sent: 7102; Received: 89188
Run 2 Firefox 3.5.6
Sent: 6388; Received: 166473
If you're wondering why FF gets twice the bytes, it's because google.com in IE6 is very basic - no search-as-you-type so much less JavaScript and one less sprite.
如果您想知道FF为什么会得到两倍的字节,那是因为IE6中的google.com非常基础-无需键入即可搜索,因此JavaScript更少,精灵也更少。
那是所有人 (That's all folks)
Enjoy, fork and keep an eye on what's up with the HTTP traffic. What goes through the tubes is too important not to be observed and monitored 🙂
尽情享受,分叉并密切注意HTTP流量的最新动态。 穿过管子的东西太重要了,以至于不能观察和监视🙂
Tell your friends about this post on Facebook and Twitter
在Facebook和Twitter上告诉您的朋友有关此帖子的信息
翻译自: https://www.phpied.com/automating-httpwatch-with-php-2/
httpwatch使用