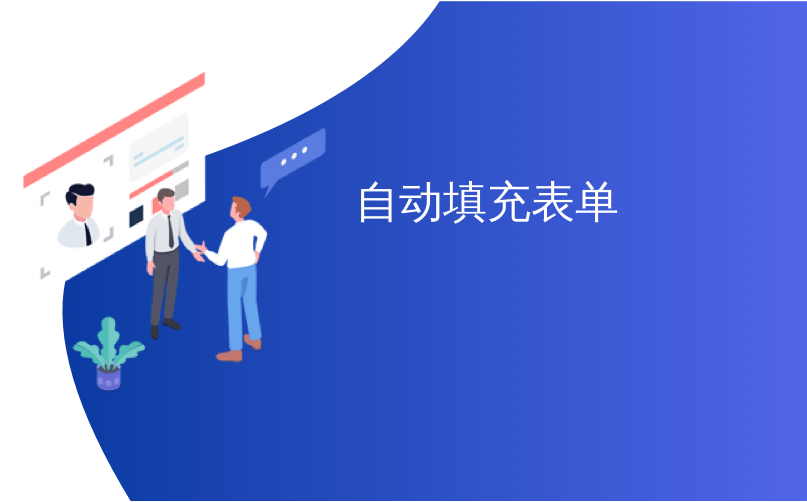
自动填充表单
介绍(Intro)
So here's the thing, we all know we hate forms, the only thing we hate more than forms themselves is actually filling out forms. But the forms are the interface to our web apps, so we cannot do without them. What we would love to do without, but can't, is skip the application testing part where you fill out forms like there's no tomorrow, just to make sure your app is rock solid.
因此,我们都知道我们讨厌表格,除表格本身之外,我们唯一讨厌的东西实际上是填写表格。 但是这些表单是我们Web应用程序的界面,因此我们离不开它们。 我们希望没有但不能做的是跳过应用程序测试部分,在该部分中填写表格,就像没有明天一样,只是确保您的应用程序坚如磐石。
And filling out forms is a pain. So for some time I wanted to get my hands on a little something that can fill out a form, any form, with a click of a button. JavaScript is ideal for such a task and the best sort of a "little something" is probably a bookmarklet. That is how this bookmark was born.
填写表格很痛苦。 因此,一段时间以来,我想动一点点东西,只需单击一下按钮就可以填写表格。 JavaScript非常适合这种任务,“小事”的最佳类别可能是小书签。 这就是书签的诞生方式。
这是什么,它做什么? (What is it, what it does?)
This is a bookmarklet. You go to page that has one or more forms and you click the bookmarklet. It completes the form for you with some random data. The whole thinking was to have a form ready to be submitted and generating as less validation errors as possible. Here are some details:
这是一个书签。 您转到具有一个或多个表单的页面,然后单击小书签。 它会为您提供一些随机数据来填写表格。 整个想法是准备好要提交的表单,并生成尽可能少的验证错误。 以下是一些详细信息:
- All defaults are kept as they are 所有默认设置均保持不变
- All passwords fields are completed with the same password, in case there is a password/password confirmation combo. The default password is "secret" 万一有密码/密码确认组合,则所有密码字段都用相同的密码填写。 默认密码是“秘密”
- If a text field has the string "email" in its name, the auto-generated value would be a random string @ example.org 如果文本字段的名称中包含字符串“ email”,则自动生成的值将是一个随机字符串@ example.org
- If a text field has the string "name" in its name, a name-looking value will be generated. 如果文本字段的名称中包含字符串“ name”,则将生成一个看起来像名字的值。
- All checkboxes will be checked (who knows which one of them might be "Accept terms" or anything else that is required) 所有复选框都将被选中(谁知道其中哪个复选框可能是“接受条款”或其他必填项)
- Multi-selects will have a random number of random options selected 多选选项将随机选择一个随机选项
安装(Install)
Right-click and bookmark or drag to your personal bookmarks toolbar.
右键单击并添加书签或拖动到个人书签工具栏。
演示版(Demo)
这是演示。
代码 (The code)
The demo and the code below are "normal" code, with proper indentation and all. The actual bookmark though has to be on one line and as small as possible, so it's pretty much unreadable. Ah, and while the demo will work in IE, the bookmarklet won't, because it's too big for IE. IE allows up to about 500 characters in the URL (or a bookmarklet) while mine is about 2000 "compressed" or 3000 cleaner. So unless I do something heroic in compressing the script, it won't work on IE. No biggie I'd say, since you'll be testing your application and most likely you use Firefox anyway.
演示和下面的代码是“常规”代码,带有适当的缩进和全部。 尽管实际的书签必须在一行中,并且必须尽可能地小,所以它几乎是不可读的。 嗯,虽然该演示可以在IE中运行,但书签无法使用,因为它对于IE来说太大了。 IE允许URL(或小书签)中最多包含500个字符,而我的则是2000个“压缩”或3000个更清洁的字符。 因此,除非我在压缩脚本方面做一些英勇的工作,否则它将无法在IE上运行。 没什么好说的,因为您将测试您的应用程序,并且很可能仍然使用Firefox。
大图 (The big picture)
Using JSON, the class/object is called auto
and it has the following interface:
使用JSON,该类/对象称为auto
,它具有以下接口:
var auto ={
// a list of names that will be used to generate
// normal looking names
names: "Steve Buscemi Catherine Keener ...",
// this is where all the random words will come from
blurb: "phpBB is a free...",
// default password to be used in all password fields
password: "secret",
// the main function that does all
fillerup: function() {},
// helper function, returns randomly selected words
// coming from this.blurb
getRandomString: function (how_many_words) {},
// helper function, returns randomly selected names
// coming from this.names
getRandomName: function () {},
// returns this.password
getPassword: function () {},
// returns a random int from 0 to count
getRand: function (count) {}
}
The actual form fill-out is initiated by calling auto.fillerup()
实际的表单填写是通过调用auto.fillerup()
启动的
As you can probably guess, the only interesting function is fillerup()
, so let me show you what it does.
您可能会猜到,唯一有趣的函数是fillerup()
,所以让我向您展示它的作用。
fillerup() (fillerup())
In case you're wondering, the name of the function comes from a Sting song: Fill'er up, son, unleaded. I need a full tank of gas where I'm headed ...
如果您想知道,该函数的名称来自一首Sting歌曲:儿子,加油,无铅。 我要去的地方满满的汽油...
The function starts by identifying all the elements candidate to be completed:
该功能首先确定要完成的所有候选元素:
var all_inputs = document.getElementsByTagName('input');
var all_selects = document.getElementsByTagName('select');
var all_textareas = document.getElementsByTagName('textarea');
OK, we have our work cut out for us, let's start by looping through the select
s:
好的,我们的工作已经完成,让我们开始遍历select
s:
// selects
for (var i = 0, max = all_selects.length; i < max; i++) {
var sel = all_selects[i]; // current element
if (sel.selectedIndex != -1
&& sel.options[sel.selectedIndex].value) {
continue; // has a default value, skip it
}
var howmany = 1; // how many options we'll select
if (sel.type == 'select-multiple') { // multiple selects
// random number of options will be selected
var howmany = 1 + this.getRand(sel.options.length - 1);
}
for (var j = 0; j < howmany; j++) {
var index = this.getRand(sel.options.length - 1);
sel.options[index].selected = 'selected';
// @todo - Check if the selected index actually
// has a value otherwise try again
}
}
Then - textarea
s, they cannot be simpler. We only check if there isn't already a value and if there's none, we get two "paragraphs" of 10 words each.
然后textarea
,它们再简单不过了。 我们仅检查是否还没有值,如果没有,则得到两个“段落”,每个段落有10个字。
// textareas
for (var i = 0, max = all_textareas.length; i < max; i++) {
var ta = all_textareas[i];
if (!ta.value) {
ta.value = this.getRandomString(10)
+ '\\n\\n'
+ this.getRandomString(10);
}
}
Next (and last), come the input
s. They are a bit more complicated as there are too many of them. Here's the overall code with the skipped details for each input type.
接下来(也是最后一个), input
s。 因为它们太多了,所以它们有点复杂。 以下是带有每种输入类型的跳过详细信息的总体代码。
// inputs
for (var i = 0, max = all_inputs.length; i < max; i++) {
var inp = all_inputs[i];
var type = inp.getAttribute('type');
if (!type) {
type = 'text'; // default is 'text''
}
if (type == 'checkbox') {...}
if (type == 'radio') {...}
if (type == 'password') {...}
if (type == 'text') {...}
}
We're absolutely unforgiving when it comes to checkboxes - just check them all, no questions asked, take no prisoners.
对于复选框,我们绝对不会原谅-只需选中所有复选框,不问任何问题,就不用囚犯了。
if (type == 'checkbox') {
// check'em all
// who knows which ones are required
inp.setAttribute('checked', 'checked');
/* ... ooor random check-off
if (!inp.getAttribute('checked')) {
if (Math.round(Math.random())) { // 0 or 1
inp.setAttribute('checked', 'checked');
}
}
*/
}
Next, do the radio
s. They are a bit more complicated, because once we have an element, before checking it, we need to verify that there are no other radios with the same name (and in the same form) are already selected and checked.
接下来,做radio
。 它们有点复杂,因为一旦有了一个元素,在检查它之前,我们需要验证是否没有其他同名(形式相同)的无线电已经被选中和检查。
if (type == 'radio') {
var to_update = true;
// we assume this radio needs to be checked
// but in any event we'll check first
var name = inp.name;
var input_array = inp.form.elements[inp.name];
for (var j = 0; j < input_array.length; j++) {
if (input_array[j].checked) {
// match! already has a value
to_update = false;
continue;
}
}
if (to_update) {
// ok, ok, checking the radio
// only ... randomly
var index = this.getRand(input_array.length - 1);
input_array[index].setAttribute('checked', 'checked');
}
}
Passwords - trivial, just make sure you always set the same password.
密码-微不足道,只需确保始终设置相同的密码即可。
if (type == 'password') {
if (!inp.value) {
inp.value = this.getPassword();
}
}
And finally - the text inputs. We try to guess the nature of the text field by its name. Here there's plenty of room for improvement and more guesses.
最后-文本输入。 我们尝试通过其名称来猜测文本字段的性质。 这里有很大的改进空间和更多的猜测。
if (type == 'text') {
if (!inp.value) {
// try to be smart about some stuff
// like email and name
if (inp.name.indexOf('name') != -1) { // name
inp.value = this.getRandomName() + ' ' + this.getRandomName();
} else if (inp.name.indexOf('email') != -1) { // email address
inp.value = this.getRandomString(1) + '@example.org';
} else {
inp.value = this.getRandomString(1);
}
}
}
狂欢 (C'est tout)
That's it, hope you liked it and start using it 😉 Any comment or suggestions - let me know.
就是这样,希望您喜欢它并开始使用它😉任何意见或建议-让我知道。
Tell your friends about this post on Facebook and Twitter
在Facebook和Twitter上告诉您的朋友有关此帖子的信息
自动填充表单