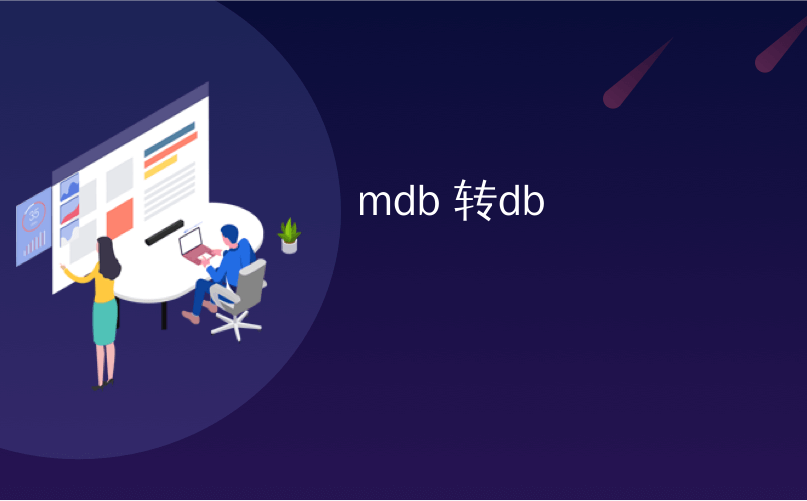
mdb 转db
介绍(Intro)
Recently I had to move an existing project from using PEAR::DB to PEAR::MDB2 - the new database abstraction layer. I took notes on the parts of the code I needed to change, I hope they can benefit someone who's doing the same. Many thanks go to Lukas Smith, the lead developer, he was always responding very fast to my reports and questions in the PEAR mailing list.
最近,我不得不将现有项目从使用PEAR :: DB迁移到PEAR :: MDB2-新的数据库抽象层。 我记下了需要更改的代码部分,希望它们可以使这样做的人受益。 非常感谢首席开发人员Lukas Smith ,他一直对PEAR邮件列表中的报告和问题做出非常快速的响应。
One thing to notice in MDB2 is that it tries not to do any unnecessary work and does many things only on demand. For example when you create an object, that doesn't mean that a connection is established. It is established only when you make the first real database access, a SELECT
for example.
在MDB2中要注意的一件事是,它试图不做任何不必要的工作,并且仅在需要时才做很多事情。 例如,当您创建对象时,这并不意味着已建立连接。 仅当您首次进行真正的数据库访问(例如SELECT
时,才会建立该数据库。
I assume you have an idea of PEAR::DB, since this posting illustrates a DB-to-MDB2 endeavour, but even if you don't, I hope the posting will still be useful as an intro to DB and MDB2.
我假设您有一个PEAR :: DB的想法,因为此发布说明了从DB到MDB2的工作,但是即使您没有,我希望该发布对DB和MDB2的介绍还是有用的。
包括库 (Including the libs)
So first off, including the libs (I assume you have PEAR on your machine).
所以首先,包括库(我假设您的计算机上装有PEAR)。
require_once 'DB.php';
require_once 'MDB2.php';
One thing to note here is that installing MDB2 doesn't install any of the database wrappers. So if you use MySQL for example, you'd need to install it separately: pear install MDB2_Driver_mysql-beta
in addition to pear install MDB2-beta
这里要注意的一件事是,安装MDB2不会安装任何数据库包装程序。 因此,例如,如果您使用MySQL,则需要单独安装它:除pear install MDB2-beta
外, pear install MDB2-beta
pear install MDB2_Driver_mysql-beta
pear install MDB2-beta
MDB2 is now a stable release! So you can now remove the "-beta
" monkier when installing the packages.
MDB2现在是一个稳定的发行版! 因此,您现在可以在安装软件包时删除“ -beta
” Monkier。
DSN (DSN)
Next - the DSN string. It's the same for MDB2 as for DB.
下一个-DSN字符串。 MDB2与DB相同。
$dsn = 'mysql://root@localhost/db2mdb2';
BTW, MDB2 can also accept an array of all the connection details, as opposed to a DSN string. And so does DB (Thanks for the clarification, Justin!)
顺便说一句,与DSN字符串相反,MDB2也可以接受所有连接详细信息的数组。 DB也是如此(感谢您的澄清,Justin!)
创建实例 (Creating instances)
$db =& DB::connect($dsn);
$mdb2 =& MDB2::factory($dsn);
MDB2 provides a factory method to create an instance. At this time no database connection is yet established. MDB2 also provides a singleton()
method to create an instance.
MDB2提供了一种创建实例的工厂方法。 目前,尚未建立数据库连接。 MDB2还提供了singleton()
方法来创建实例。
提取模式 (Fetchmode)
It is the same in both DB and MDB2, just note the prefix of the constant.
在DB和MDB2中都是一样的,只需要注意常量的前缀即可。
// set fetchmode
$db->setFetchMode(DB_FETCHMODE_ASSOC);
$mdb2->setFetchMode(MDB2_FETCHMODE_ASSOC);
简单的选择(Simple SELECTs)
There are the methods to select one row, one column, one cell and a bunch of records. DB prefixes them with get
, while MDB2 uses query
.
有选择一行,一列,一个单元格和一堆记录的方法。 DB用get
前缀它们,而MDB2使用query
。
// select several records and shove them into an array
$all = $db->getAll('SELECT * FROM people');
$all = $mdb2->queryAll('SELECT * FROM people');
// select one cell
$one = $db->getOne('SELECT name FROM people WHERE id = 1');
$one = $mdb2->queryOne('SELECT name FROM people WHERE id = 1');
// one row
$row = $db->getRow('SELECT * FROM people WHERE id = 1');
$row = $mdb2->queryRow('SELECT * FROM people WHERE id = 1');
// a column
$col = $db->getCol('SELECT name FROM people');
$col = $mdb2->queryCol('SELECT name FROM people');
报价值(Quoting values)
In DB, the suggested method to quote is quoteSmart()
. In MDB2 it's quote()
and it accepts a second parameter, which tells the type of the value to be quoted. If the second parameter is omitted, MDB2 will try to guess the type.
在DB中,建议的引用方法是quoteSmart()
。 在MDB2中,它是quote()
并接受第二个参数,该参数告诉要引用的值的类型。 如果省略第二个参数,则MDB2将尝试猜测类型。
$one = $db->getOne(
'SELECT name FROM people WHERE id = '
. $db->quoteSmart(1)
);
$one = $mdb2->queryOne(
'SELECT name FROM people WHERE id = '
. $db->quote(1, 'integer')
);
序列表(Sequence tables)
If you use sequence tables, both libs will provide you with a nextId()
method:
如果使用序列表,则两个库都将为您提供nextId()
方法:
echo $db->nextId('people_db');
echo $mdb2->nextId('people_mdb2');
The only difference is that when DB creates a sequence table (with one field and one value), the name of the field is id
, where MDB2 will use sequence
. If you're translating an existing project to MDB2 like me, and the sequence tables are already created by DB, you have the option of renaming this field in the database for all sequence tables, or you can set an MDB2 option and you're good to go.
唯一的区别是,当DB创建一个序列表(具有一个字段和一个值)时,该字段的名称为id
,其中MDB2将使用sequence
。 如果要像我一样将现有项目转换为MDB2,并且序列表已经由DB创建,则可以选择为所有序列表在数据库中重命名此字段,或者可以设置MDB2选项,然后好去。
$mdb2->setOption('seqcol_name','id');
自动执行(Auto execute)
Say you have the data:
假设您有数据:
$data = array('id' => 5, 'name' => 'Cameron');
To auto-insert it using DB, you'd do:
要使用DB自动插入,您可以执行以下操作:
$db->autoExecute('people', $data, DB_AUTOQUERY_INSERT);
For MDB2, the auto execution is probably not considered an often-used feature, so it's not in the base instance. You need to load an additional module to have access to it:
对于MDB2,自动执行可能不被视为常用功能,因此它不在基本实例中。 您需要加载其他模块才能访问它:
$mdb2->loadModule('Extended');
Now you can
现在你可以
$mdb2->autoExecute('people', $data, MDB2_AUTOQUERY_INSERT);
The above will work in PHP5 only. In PHP4, due to the limited support of object overloading (Thanks again to Lukas for clarifying this!), you'd need to do:
以上仅适用于PHP5。 在PHP4中,由于对象重载的支持有限(再次感谢Lukas阐明了这一点!),您需要执行以下操作:
$mdb2->extended->autoExecute('people', $data, MDB2_AUTOQUERY_INSERT);
Note that the second way will also work in PHP5.
请注意,第二种方法也可以在PHP5中使用。
准备好的陈述 (Prepared statements)
In DB:
在数据库中:
$statement = $db->prepare('INSERT INTO people VALUES (?, ?)');
$data = array(6, 'Chris');
$db->execute($statement, $data);
$db->freePrepared($statement);
In MDB it's almost the same, only that the statement becomes an object and you call its (as opposed to MDB2 main object's) methods to execute and to release memory:
在MDB中,几乎是一样的,只是该语句成为一个对象,然后调用它的方法(与MDB2主对象相对)来执行和释放内存:
$statement = $mdb2->prepare('INSERT INTO people VALUES (?, ?)');
$data = array(7, 'Dave');
$statement->execute($data);
$statement->free();
执行多个(Execute multiple)
The same applies to executing a statement with with multiple "rows" of data from an array. executeMultiple()
is in the Extended MDB2 module, so you need to load it:
这同样适用于执行带有数组中多个“行”数据的语句。 executeMultiple()
在扩展MDB2模块中,因此您需要加载它:
DB:
D B:
$statement = $db->prepare('INSERT INTO people VALUES (?, ?)');
$data = array(
array(8, 'James'),
array(9, 'Cliff')
);
$db->executeMultiple($statement, $data);
$db->freePrepared($statement);
MDB2:
MDB2:
$statement = $mdb2->prepare('INSERT INTO people VALUES (?, ?)');
$data = array(
array(10, 'Kirk'),
array(11, 'Lars')
);
$mdb2->loadModule('Extended');
$mdb2->extended->executeMultiple($statement, $data);
$statement->free();
交易次数(Transactions)
In DB:
在数据库中:
$db->autoCommit();
$result = $db->query('DELETE people'); // will cause an error
if (PEAR::isError($result)) {
$db->rollback(); //echo 'rollback';
} else {
$db->commit(); //echo 'commit';
}
In MDB2 you have to check if transactions are supported in your RDBMS. Then during the transaction, you can always check "Am I in transaction?"
在MDB2中,您必须检查RDBMS中是否支持事务。 然后,在交易过程中,您始终可以选中“我正在交易吗?”
if ($mdb2->supports('transactions')) {
$mdb2->beginTransaction();
}
$result = $mdb2->query('DELETE people');
if (PEAR::isError($result)) {
if ($mdb2->in_transaction) {
$mdb2->rollback(); // echo 'rollback';
}
} else {
if ($mdb2->in_transaction) {
$mdb2->commit(); // echo 'commit';
}
}
示例脚本(Example script)
You can download a script that has the examples above and play with it. Here's also the sql file to recreate the database:
您可以下载包含以上示例的脚本并进行操作。 这也是重新创建数据库的sql文件:
Any questions or comments are welcome 😉 Thanks for reading!
欢迎任何问题或评论😉感谢您的阅读!
Tell your friends about this post on Facebook and Twitter
在Facebook和Twitter上告诉您的朋友有关此帖子的信息
mdb 转db