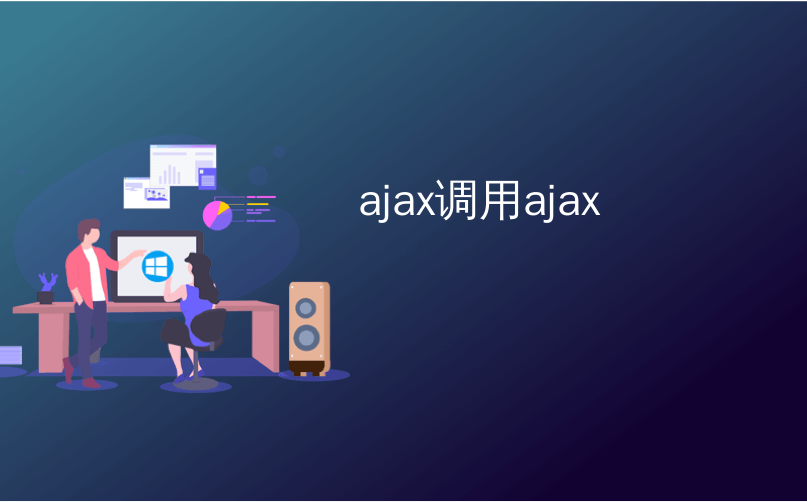
ajax调用ajax
So here's the case: rotate ads on a website, even when the page is not reloaded. If the chances are that the visitor will spend more time on a page, it makes sense to opt-in for displaying more than one ad at one page load. I've done this in the past using iframes
, but hey, it's 21st century (the last time I checked) and we have AJAX! 😉
因此,就是这种情况:即使没有重新加载页面,也可以在网站上轮播广告。 如果访问者有机会在页面上花费更多时间,则可以选择在一个页面加载时选择显示多个广告。 我过去使用iframes
完成了此操作,但是,嘿,这是21世纪(我上次检查的时间),我们拥有AJAX! 😉
I also wanted to have the flexibility to display any ad type, which means I should be able to rotate pieces of HTML, rather than just images. Using HTML chunks, the ad can be an image, or formatted text, or swf, etc.
我还希望能够灵活地显示任何广告类型,这意味着我应该能够旋转HTML片段,而不仅仅是图像。 使用HTML块,广告可以是图像,格式文本或swf等。
演示版 (Demo)
If you're curious to see the demo, go straight ahead (nothing impressive, I must admit, just rotating stuff 😉 ).
如果您想观看演示,请直接前进(我必须承认,只是旋转东西no令人印象深刻)。
忙起来 (Getting busy)
The "architecture" is fairly trivial - one HTML page (demo.html) and one JavaScript (ajax-banner.js) that makes XMLHTTP requests to a server-side script (ajax-banner.php). The server side (that has all the logic to figure out the next advertisement to show) returns XML response which is received by the caller JS and then displayed in a div
on the HTML page.
“架构”非常简单-一个HTML页面(demo.html)和一个JavaScript(ajax-banner.js),向服务器端脚本(ajax-banner.php)发出XMLHTTP请求。 服务器端(具有找出下一个要显示的广告的全部逻辑)返回XML响应,该响应由调用方JS接收,然后显示在HTML页面的div
。
<div id="ajax-banner"></div>
服务器端脚本 (The server-side script)
For the purposes of the example, the ad logic is simple - an array of HTML chunks, we pick a random one and return it as part of the XML response. In addition to the HTML chunk, as part of the XML response, the server-side script also produces an "instruction" as to how long should the ad be displayed.
就本示例而言,广告逻辑很简单-HTML块数组,我们随机选择一个,并将其作为XML响应的一部分返回。 除了HTML块之外,作为XML响应的一部分,服务器端脚本还会生成一条有关广告应显示多长时间的“指令”。
Here's how the script looks like:
脚本如下所示:
<?php
// an array of banners
$banners = array (
'<em>ban</em>ner 1',
'banner <strong>2.0.</strong>',
'<h1>big banner</h1>',
'Inline JS won\'t run :( <script>alert("boom!")</script>',
'External JS won\'t run :(( <script src="test.js"></script>',
'<img src="phpied.gif" />'
);
// pick a random one
$html = $banners[array_rand($banners)];
// send XML headers
header('Content-type: text/xml');
echo '<?xml version="1.0" ?>';
// print the XML response
?>
<banner>
<content><?php echo htmlentities($html); ?></content>
<reload>5000</reload>
</banner>
JavaScript(The javascript)
The javascript consists of three functions. The main "dispatcher" is nextAd()
which loads the next ad in the div
. nextAd()
calls the makeHttpRequest()
function to send a request to the server-side script and then passes the response to loadBanner()
which actually updates the banner div.
JavaScript由三个功能组成。 主要的“调度程序”是nextAd()
,它会在div
加载下一个广告。 nextAd()
调用makeHttpRequest()
函数将请求发送到服务器端脚本,然后将响应传递给loadBanner()
,后者实际上会更新loadBanner()
div。
makeHttpRequest() (makeHttpRequest())
I've been using this simple function I came up with for a few projects (like theInvisibleAd.com) No AJAX libraries or toolkits are necessary as the task is pretty straightforward.
我一直在为几个项目(例如theInvisibleAd.com )使用这个简单的函数,因为任务非常简单,因此不需要AJAX库或工具包。
This function makes an HTTP request and passes the response to another function, specified on-the-fly. The function is the bare basics of the AJAX thing and if you're interested in the details you can check my article on SitePoint where I've used it to create a simple web console application.
此函数发出HTTP请求,并将响应传递给即时指定的另一个函数。 该功能只是AJAX的基础知识,如果您对详细信息感兴趣,可以在SitePoint上查看我的文章,我曾在该文章中使用它来创建简单的Web控制台应用程序。
nextAd() (nextAd())
This function makes the request and passes the response to loadBanner()
. The HTTP request uses the current time in milliseconds to try to prevent the client browser from using a cached copy from the previous request.
该函数发出请求并将响应传递给loadBanner()
。 HTTP请求使用当前时间(以毫秒为单位)来尝试阻止客户端浏览器使用前一个请求的缓存副本。
function nextAd()
{
var now = new Date();
var url = 'ajax-banner.php?ts=' + now.getTime();
makeHttpRequest(url, 'loadBanner', true);
}
loadBanner() (loadBanner())
loadBanner()
accepts the XML response generated by the server-side script. The XML has two tags - content
(the HTML to be displayed) and reload
(the allocated time for this ad to be displayed). The banner div is updated with the new HTML content using innerHTML
the simplest, yet questionable by some purists, including myself, way of updating an already loaded page with JS.
loadBanner()
接受服务器端脚本生成的XML响应。 XML具有两个标签- content
(要显示HTML)和reload
(为此广告显示的分配时间)。 标语div使用innerHTML
使用新HTML内容进行更新,这是最简单的方法,但对于某些纯粹主义者(包括我自己)来说,这也是用JS更新已经加载的页面的方式所质疑的。
Once the ad is updated, old timeout is cleared (if any) and a new timeout is set, the time in milliseconds after which we'll call nextAd()
again to make the next HTTP request.
广告更新后,将清除旧的超时(如果有的话)并设置新的超时,以毫秒为单位的时间,之后我们将再次调用nextAd()
发出下一个HTTP请求。
function loadBanner(xml)
{
var html_content = xml.getElementsByTagName('content').item(0).firstChild.nodeValue;
var reload_after = xml.getElementsByTagName('reload').item(0).firstChild.nodeValue;
document.getElementById('ajax-banner').innerHTML = html_content;
try {
clearTimeout(to);
} catch (e) {}
to = setTimeout("nextAd()", parseInt(reload_after));
}
结论(Conclusion)
There's one drawback to this method though - any javascripts (inline or external) in the ad's body won't get executed. I have a remedy for this - including JavaScripts on the fly, outlined in this posting, but in this case I need to know something about the HTML returned by the server-side script, which can be passed as another tag in the XML, saying something like <is-this-js> -> you bettcha
! In this case the solution will become less of a one-size-fits-all, but still a viable option.
不过,这种方法有一个缺点-广告体内的所有javascript(内联或外部javascript)都不会执行。 我有这样的补救措施-包括在运行JavaScript,中概述这次调职,但在这种情况下,我需要了解一下服务器端脚本返回HTML,它可以作为XML的另一个标签通过,说类似于<is-this-js> -> you bettcha
! 在这种情况下,解决方案将不再是千篇一律的,而是一种可行的选择。
Overall, if you don't plan to use JS in your ads, the solution outlined in this post is very simple and seems like ideal for replacing iframe-based banner rotations using sexier AJAX.
总体而言,如果您不打算在广告中使用JS,则本文中概述的解决方案非常简单,对于使用性感的AJAX替换基于iframe的横幅广告轮换而言,它似乎是理想的选择。
Tell your friends about this post on Facebook and Twitter
在Facebook和Twitter上告诉您的朋友有关此帖子的信息
ajax调用ajax