c+ 引用传递坑
介绍 (Introduction)
In this tutorial, we are going to discuss the concept of pass by reference in C++.
在本教程中,我们将讨论C ++中按引用传递的概念。
在C ++中将参数传递给函数 (Passing Arguments to a Function in C++)
We can pass objects or variables by any of the two methods, namely, pass by value and pass by reference. Both work on different mechanisms and have their own pros and cons.
我们可以通过两种方法中的任何一种来传递对象或变量,即按值 传递和按引用传递 。 两者都在不同的机制上工作,各有优缺点。
Let us have a deeper look at both of the above-mentioned techniques individually.
让我们分别更深入地研究上述两种技术。
在C ++中按值传递 (Pass by Value in C++)
In pass by value method, the called function creates a whole new set of variables and copies the values of the arguments. Hence, it does not have access to the original variables and can only work on the copies made earlier.
在按值传递方法中,被调用函数将创建一组全新的变量并复制参数值。 因此,它无权访问原始变量,并且只能处理较早创建的副本。
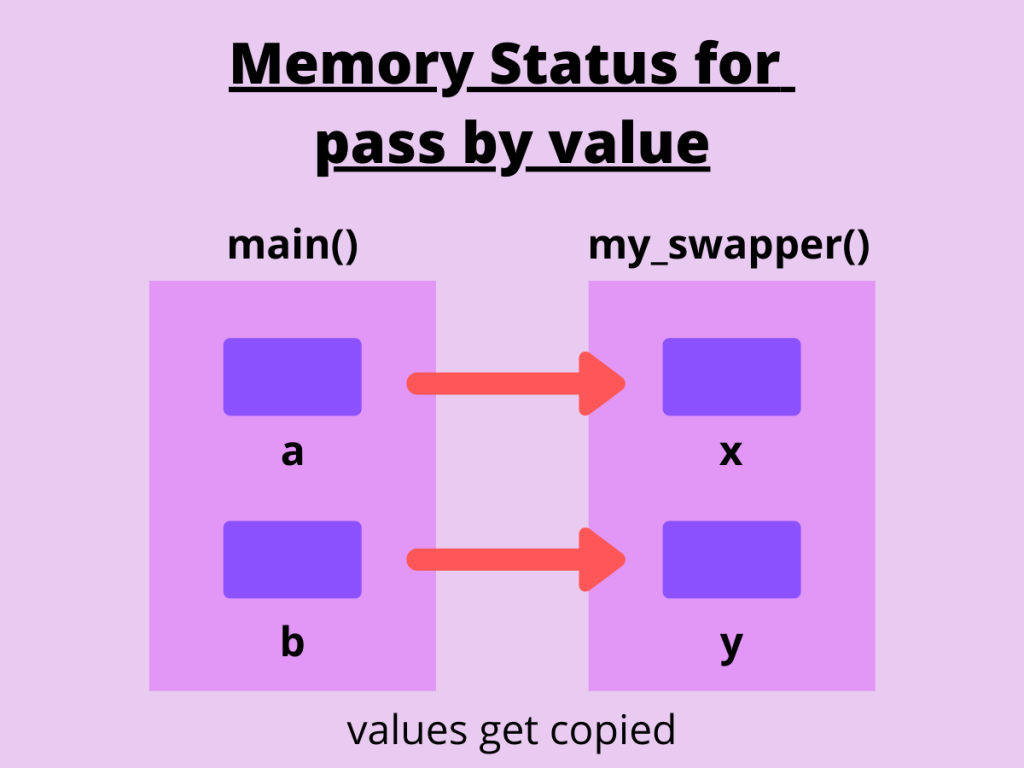
The above block diagram depicts the working of the method for the example below.
上面的框图描述了以下示例的方法的工作。
#include <iostream>
using namespace std;
void my_swapper(int x, int y)
{
int temp;
temp=x;
x=y;
y=temp;
//swapping takes place among x & y
//and not in a & b!
cout<<"Swapped values are: ";
cout<<"a="<<x<<" b="<<y<<endl;
}
int main()
{
int a= 10, b= 20;
cout<<"Original Values: ";
cout<<"a="<<a<<" b="<<b<<endl; //original values
my_swapper(a,b); //pass by value
cout<<"After swap: ";
cout<<"a="<<a<<" b="<<b<<endl;
//pass by value causes no change in original values
return 0;
}
Output:
输出 :
Original Values: a=10 b=20
Swapped values are: a=20 b=10
After swap: a=10 b=20
Here, x
and y
are the copies which store the values of the variables a
and b
respectively. As we can see the function my_swapper()
only swaps the values among the copies and cannot change the original variables.
在此, x
和y
是分别存储变量a
和b
的值的副本。 如我们所见,函数my_swapper()
仅在副本之间交换值,而不能更改原始变量。
Pass by value is useful when the original values are not to be modified and ensures that the function cannot harm the original value.
当不希望修改原始值时, 按值传递很有用,并确保函数不会损害原始值。
在C ++中通过引用传递 (Pass by Reference in C++)
The method to pass by reference in C++ uses a different mechanism. Instead of passing values to a function being called, a reference to the original variable is created or passed. A reference is an alias for a pre-defined variable. That is, the same variable can be accessed using any of the variable or reference name.
C ++中通过引用传递的方法使用不同的机制。 无需将值传递给调用的函数,而是创建或传递对原始变量的引用 。 引用是预定义变量的别名。 即,可以使用任何变量或引用名称来访问相同的变量。
Now let us look at an example to understand the same.
现在让我们看一个例子来理解它。
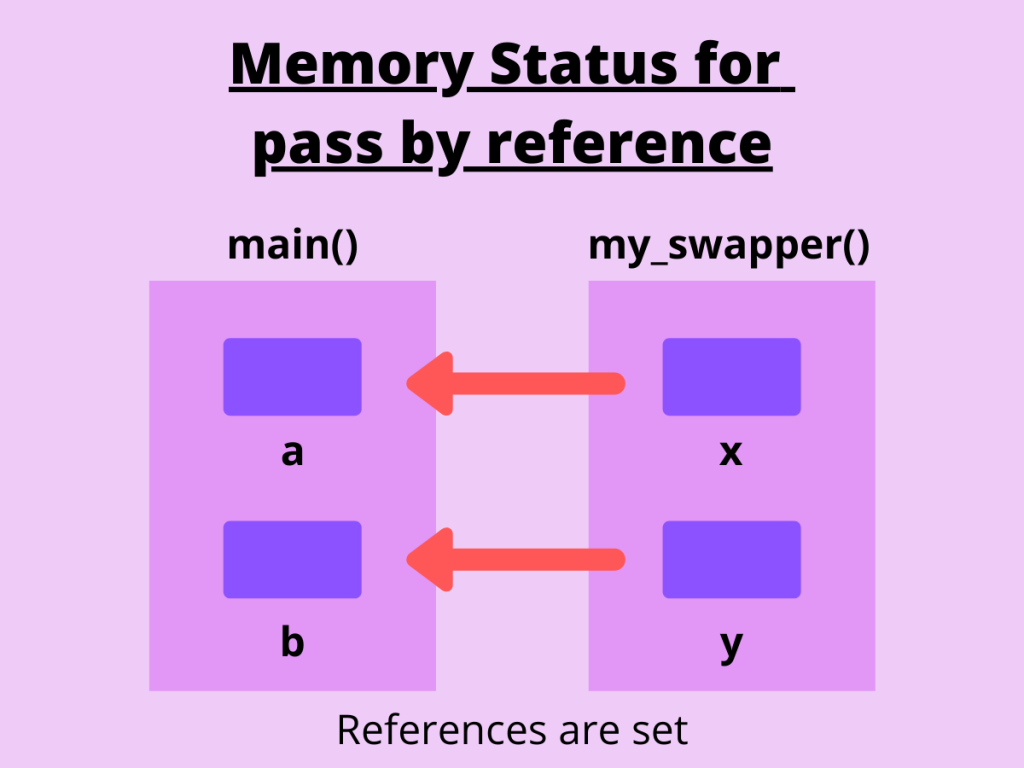
Similarly, the above block diagram depicts the working of the method for the example below.
同样,以上框图描述了以下示例的方法的工作。
#include <iostream>
using namespace std;
void my_swapper(int &x, int &y) //references to variables created
{
int temp;
temp=x;
x=y;
y=temp;
//swapping takes place and is reflected in original values
cout<<"Swapped values are: ";
cout<<"a="<<x<<" b="<<y<<endl;
}
int main()
{
int a=10, b=20;
cout<<"Original Values: ";
cout<<"a="<<a<<" b="<<b<<endl; //original values
my_swapper(a,b); //pass by reference
cout<<"After swap: ";
cout<<"a="<<a<<" b="<<b<<endl;
//pass by reference causes change in original values
return 0;
}
Output:
输出 :
Original Values: a=10 b=20
Swapped values are: a=20 b=10
After swap: a=20 b=10
Here, a
and b
are passed to the my_swapper()
function. Hence, x
and y
become the reference variables which are alias to a and b respectively.
在此,将a
和b
传递给my_swapper()
函数。 因此, x
和y
成为a和b的别名的参考变量。
In this way swapping x and y practically affect the variables a and b. As we can see from the above output, this time a
and b
are actually swapped using their references. This also brings us to the concept of pointers in C++ which we covered earlier.
这样,交换x和y实际上会影响变量a 和b。 我们可以从上面的输出,这时候看到a
和b
实际上是使用它们的引用进行交换的。 这也使我们想到了前面介绍的C ++中的指针概念 。
示例–使用C ++中的“按引用传递”以英尺或英寸为单位转换距离 (Example – Converting distance in feet or inches using Pass by Reference in C++)
Further, let us look at an example to understand the use of the pass by reference method in C++.
此外,让我们看一个示例,以了解C ++中按引用传递方法的用法。
#include <iostream>
using namespace std;
void convert(float &, char &,char &);//function prototype
int main()
{
//variable initialisation
float distance;
char choice, type='F';
cout<<"Enter Distance in feet: ";
cin>>distance;
cout<<"\nYou want distance in feet or inches? (F/I): ";
cin>>choice;
switch(choice)
{
case 'F': convert(distance,type,choice);
break;
case 'I': convert(distance,type,choice);
break;
default: cout<<"\n\nError!!!"; //if wrong choice is entered
exit(0);
}
cout<<"\nDistance = "<<distance<<" "<<type;
return 0;
}
//pass by reference
void convert(float &d, char &t, char &ch)
{
switch(ch)
{
case 'F': if(t=='I')
{
d=d/12;
t='F';
}
break;
case 'I': if(t=='F')
{
d=d*12;
t='I';
}
break;
}
}
Output:
输出 :
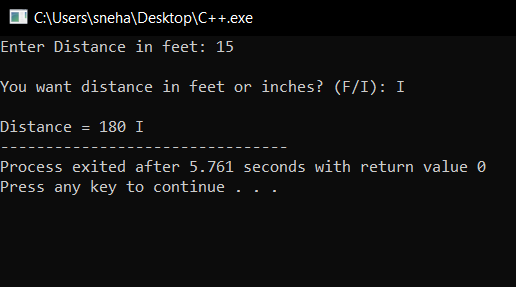
In the above code, we convert distance in feet to feet or inches. Similar to the previous example this time inside the function convert()
, d
, t
and ch
are references to the variables distance
, type
and choice
respectively. Hence, change in any of the references updates the original values too.
在上面的代码中,我们将以英尺为单位的距离转换为英尺或英寸。 与前面的示例类似,这次在函数convert()
, d
, t
和ch
分别引用了变量distance
, type
和choice
。 因此,任何参考中的更改也会更新原始值。
结论 (Conclusion)
So in this tutorial, we learned the concept of pass by reference in C++, its working mechenism and use.
因此,在本教程中,我们学习了C ++中的按引用传递的概念,其有效的机制和用法。
For any further questions, feel free to use the comments below.
如有其他疑问,请随时使用以下评论。
参考资料 (References)
- References – Documentation, 参考资料 –文档,
- Pass by Reference / Value in C++, Stackoverflow Question. 在C ++中按引用/值传递 ,Stackoverflow问题。
- passing object by reference in C++ – Stackoverflow Question. C ++中通过引用传递对象 – Stackoverflow问题。
翻译自: https://www.journaldev.com/38174/pass-by-reference-c-plus-plus
c+ 引用传递坑