python 逻辑运算符
Python logical operators allow us to perform logical AND, OR, and NOT operation between boolean values. In this article, we will look into the logical operator’s execution in more detail.
Python逻辑运算符使我们能够在布尔值之间执行逻辑AND,OR和NOT运算。 在本文中,我们将更详细地研究逻辑运算符的执行。
Python逻辑运算符 (Python Logical Operators)
There are three logical operators in Python.
Python中有三个逻辑运算符。
Logical Operator | Description | Simple Example |
---|---|---|
and | Logical AND Operator | flag = True and True = True |
or | Logical OR Operator | flag = False or True = True |
not | Logical NOT Operator | flag = not(False) = True |
逻辑运算符 | 描述 | 简单的例子 |
---|---|---|
和 | 逻辑与运算符 | 标志= True和True = True |
要么 | 逻辑或运算符 | 标志= False或True = True |
不 | 逻辑非运算符 | 标志=不(False)= True |
Python逻辑AND运算子 (Python Logical AND Operator)
Here is a simple example of a logical and operator.
这是一个逻辑和运算符的简单示例。
x = 10
y = 20
if x > 0 and y > 0:
print('Both x and y are positive numbers')
Output: Both x and y are positive numbers
输出 : Both x and y are positive numbers
Before the logical operation is performed, the expressions are evaluated. So the above if condition is similar to below:
在执行逻辑运算之前,先对表达式求值。 因此,上述if条件类似于以下内容:
if (x > 0) and (y > 0):
print('Both x and y are positive numbers')
In Python, every variable or object has a boolean value. We have explained this in more detail at Python bool() function.
在Python中,每个变量或对象都有一个布尔值。 我们已经在Python bool()函数中对此进行了更详细的解释。
if x and y:
print('Both x and y have boolean value as True')
Output: Both x and y have boolean value as True
because ‘x’ and ‘y’ len() function will return 2 i.e. > 0.
输出 : Both x and y have boolean value as True
因为'x'和' y'len()函数将返回2,即> 0。
False
, then the further expressions are not evaluated.
False
,则不对其他表达式进行计算。
Let’s confirm the above statement by defining a custom object. Python uses __bool__()
function to get the boolean value of an object.
让我们通过定义一个自定义对象来确认上述声明。 Python使用__bool__()
函数获取对象的布尔值。
class Data:
id = 0
def __init__(self, i):
self.id = i
def __bool__(self):
print('Data bool method called')
return True if self.id > 0 else False
d1 = Data(-10)
d2 = Data(10)
if d1 and d2:
print('Both d1 and d2 ids are positive')
else:
print('Both d1 and d2 ids are not positive')
Output:
输出:
Data bool method called
At least one of d1 and d2 ids is negative
Notice that __bool__() function is called only once, confirmed from the print statement output.
请注意,从print语句输出中确认,__bool __()函数仅被调用一次。
Below diagram depicts the flow chart of logical and operation.
下图描述了逻辑和操作流程图。
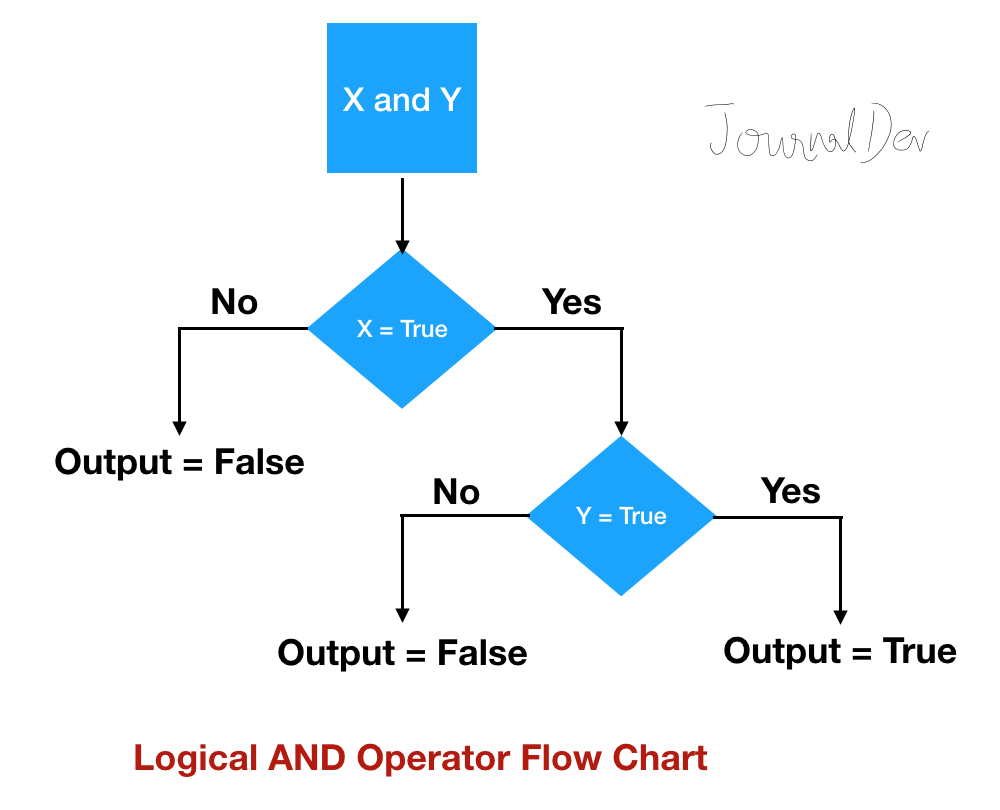
Python Logical and Operator Flow Chart
Python逻辑和运算符流程图
Python逻辑或运算符 (Python Logical OR Operator)
Let’s look at a simple example of logical OR operator.
让我们看一个逻辑OR运算符的简单示例。
x = 10
y = 20
if x > 0 or y > 0:
print('At least one of x and y is positive number')
Output: At least one of x and y is positive number
输出: At least one of x and y is positive number
True
, then the further expressions are not evaluated.
True
,则不对其他表达式进行计算。
Let’s confirm this with a simple code snippet. We will reuse the Data class defined earlier.
让我们用一个简单的代码片段来确认这一点。 我们将重用之前定义的Data类。
d1 = Data(10)
d2 = Data(20)
# The expressions are evaluated until it's required
if d1 or d2:
print('At least one of d1 and d2 id is a positive number')
else:
print('Both d1 and d2 id are negative')
Output:
输出:
Data bool method called
At least one of d1 and d2 id is a positive number
Notice that the __bool__() method is called only once and since it’s evaluated to True
, further expressions are not evaluated.
请注意,__ bool __()方法仅被调用一次,并且由于它被评估为True
,因此不会评估其他表达式。
Let’s run another example where both Data object boolean value will return False
.
让我们运行另一个示例,其中两个Data对象的布尔值都将返回False
。
d1 = Data(-10)
d2 = Data(-20)
if d1 or d2:
print('At least one of d1 and d2 id is a positive number')
else:
print('Both d1 and d2 id are negative')
Output:
输出:
Data bool method called
Data bool method called
Both d1 and d2 id are negative
Finally, below image depicts the flow chart of logical or operator.
最后,下图描述了逻辑或运算符的流程图。
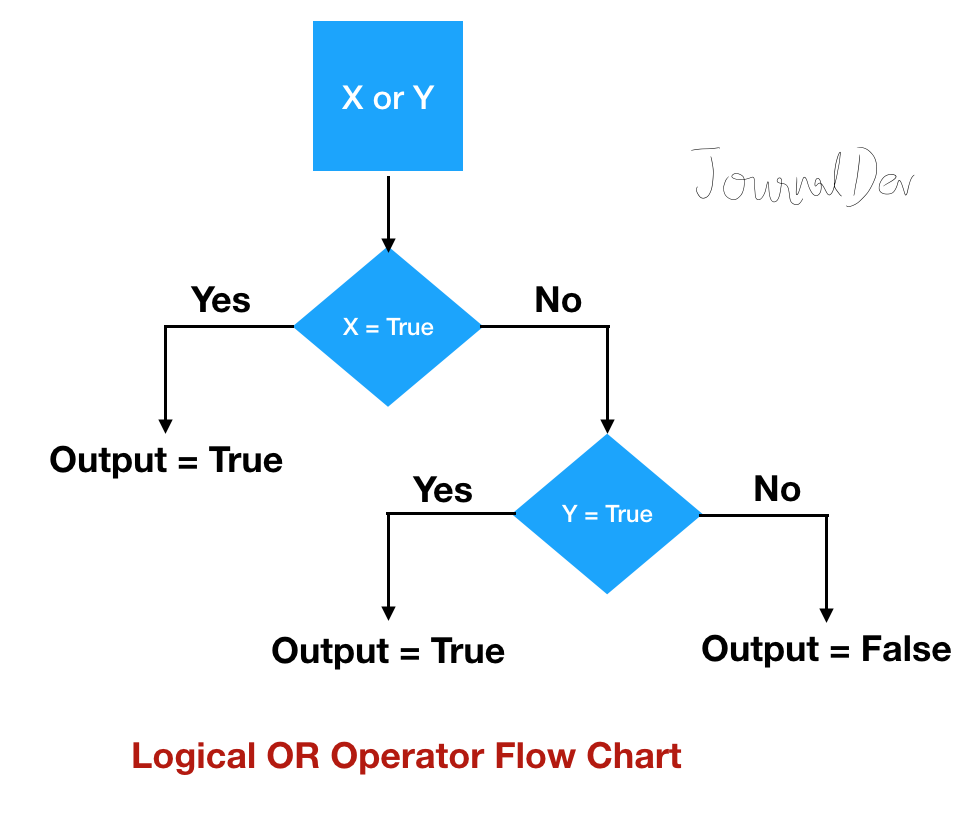
Python Logical or Operator
Python逻辑或运算符
Python逻辑非运算符 (Python Logical NOT Operator)
This is a very simple operator that works with a single boolean value. If the boolean value is True
then not operator will return False
and vice-versa.
这是一个非常简单的运算符,可用于单个布尔值。 如果布尔值是True
则not运算符将返回False
,反之亦然。
Let’s look at a simple example of “not” operator.
让我们看一个简单的“非”运算符示例。
a = 37
if not (a % 3 == 0 or a % 4 == 0):
print('37 is not divisible by either 3 or 4')
Output: 37 is not divisible by either 3 or 4
输出: 37 is not divisible by either 3 or 4
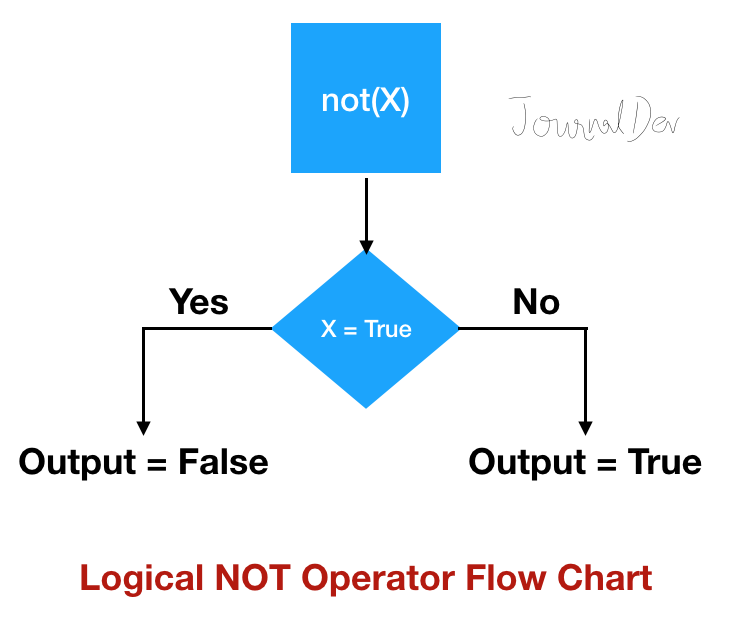
Python Logical Not Operator
Python逻辑非运算符
从左到右评估Python运算符 (Python Operators Are Evaluated From Left to Right)
Let’s look at an example where we have multiple logical operators. We are not mixing different types of Python operators, so that operator precedence won’t come in picture and it will be easier to explain the output.
让我们看一个示例,其中有多个逻辑运算符。 我们不会混合使用不同类型的Python运算符 ,因此不会显示运算符优先级,并且将更易于解释输出。
We will also override the __bool__() function to print “id” value.
我们还将覆盖__bool __()函数以显示“ id”值。
def my_bool(data):
print('Data bool method called, id =', data.id)
return True if data.id > 0 else False
Data.__bool__ = my_bool
d1 = Data(10)
d2 = Data(-20)
d3 = Data(20)
# evaluated from left to right, confirmed from the __bool__() print statement
if d1 and d2 or d3:
print('At least one of them have a positive id.')
Output:
输出:
Data bool method called, id = 10
Data bool method called, id = -20
Data bool method called, id = 20
At least one of them have a positive id.
Notice that the boolean value is calculated for d1 first, thus confirming that the execution is happening from left to right.
注意,首先为d1计算布尔值,从而确认执行是从左到右进行的。
逻辑运算符可用于任何自定义对象吗? (Logical operators work with any custom object?)
In Python, every object has a boolean value. So logical operators will work with any custom objects too. However, we can implement __bool__() function to define our custom rules for an object boolean value.
在Python中,每个对象都有一个布尔值。 因此,逻辑运算符也可以使用任何自定义对象。 但是,我们可以实现__bool __()函数来为对象布尔值定义自定义规则。
摘要 (Summary)
Python logical operators allow us to perform logical operations. We looked into logical operators in detail, how their execution is optimized to take the least amount of time. We also confirmed that the operator’s execution is performed from left to right side.
Python逻辑运算符使我们能够执行逻辑运算。 我们详细研究了逻辑运算符,如何优化它们的执行以花费最少的时间。 我们还确认操作员的执行是从左到右执行的。
翻译自: https://www.journaldev.com/26711/python-logical-operators
python 逻辑运算符