javascript入门
JavaScript can be used in a browser to change parts of a webpage dynamically. It begins with the following pattern: If condition W is true, do thing X to target Y after event Z. Below are some tips and tricks to help you get started with JavaScript and form good habits that can make your life easier in the long run. (Note: examples in this article use jQuery for simplicity.)
可以在浏览器中使用JavaScript来动态更改网页的各个部分。 它从以下模式开始:如果条件W为true,则在事件Z之后对X进行操作以将Y定位为目标。 。 (注意:为简单起见,本文中的示例使用jQuery。)
从描述开始 (
Start with Descriptions)
Start by writing comments which describe in plain language what you’re trying to do. Outline where you will need variables, functions, and events. You can always add more complexity like loops and data later on. For example after document ready, whenever someone clicks on a specific button, increment a counter on the page.
首先写评论,用简单的语言描述您要做什么。 概述需要变量,函数和事件的位置。 以后您总是可以添加更多的复杂性,例如循环和数据。 例如,准备好文档后,只要有人单击特定按钮,就在页面上增加一个计数器。
学习除文档就绪以外的事件 (
Learn Events Other than Document Ready )
Half of JavaScript is knowing what you can do, while the other half is knowing when you can do it.
JavaScript的一半知道您可以做什么,而另一半则知道您什么时候可以做到。
Document ready is not the only trigger. It’s very common to re-run the same function on document.ready, ajaxComplete, and window.resize. Modern JavaScript frameworks may use something like componentReady instead of documentReady. You can also run functions on scroll, click, touch, change, etc.
准备好文档不是唯一的触发因素。 在document.ready,ajaxComplete和window.resize上重新运行相同的功能是很常见的。 现代JavaScript框架可能会使用诸如componentReady之类的东西,而不是documentReady。 您还可以在滚动,单击,触摸,更改等上运行功能。
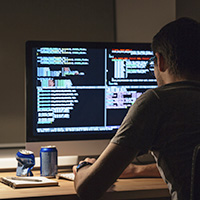
了解如何使用参数 (
Learn How to Use Parameters)
You will want a technique for running the same function with different options. Parameters facilitate reuse by allowing variables to be passed into a function.
您将需要一种使用不同选项运行相同功能的技术。 参数通过允许将变量传递到函数中来促进重用。
使所有功能可重用 (
Make All Functions Reuseable)
Don’t write events inside of functions. If the function is separate from the events, you can call that function any time. Instead of saying function X = make some button blue on click, you could say function X = make some button blue, function Y = on click run function X, and function Z = on scroll run function X.
不要在函数内部编写事件。 如果该函数与事件分开,则可以随时调用该函数。 您可以说函数X =使按钮变蓝,函数Y =单击运行函数X,单击功能Z =滚动运行函数X,而不是说功能X =使按钮变蓝色。
明确命名事物 (
Name Things Explicitly)
Keep it straightforward. When naming something, base it on the type of object. If the object is a variable then the name should start with a noun and be followed by an adjective (ex: buttonPrimary). If the object is a function then it should start with a verb, followed by a noun (ex: disableButton). If the object is a target then it should start with the word target (ex: targetButton).
保持简单明了。 命名时,请基于对象的类型。 如果对象是变量,则名称应以名词开头,后跟形容词(例如:buttonPrimary)。 如果对象是函数,则应以动词开头,后跟一个名词(例如:disableButton)。 如果对象是目标,则应以单词target(例如:targetButton)开头。
Functions with the purpose of running groups of functions should start with a word like “run”.
以运行功能组为目的的功能应以“ run”之类的词开头。
targetButton = $('#buttonPrimary');
targetBackgroundColor = '#000';
changeButtonBackgroundColor = function(targetButton, targetBackgroundColor) {};
animateButton = function(targetButton);
runButtonActions = function() {
changeButtonBackgroundColor();
animateButton();
}
$(window.document).ajaxComplete(function() {runButtonActions();}
$(window).resize(function() {runButtonActions();}
在操作之前检查是否存在 (
Check if Things Exist Before Manipulating Them)
A common JavaScript error is trying to do something to an element that doesn’t exist. This is easily preventable by always confirming that something exists before you try to modify it.
常见JavaScript错误是尝试对不存在的元素进行处理。 通过在尝试修改之前始终确认某些内容,可以很容易地避免这种情况。
()
if ($('#targetThing'')[0]){//do something because it exists};
在外面使用单引号 (
Use Single Quotes Outside)
JavaScript allows you to use single quotes and double quotes in the same statement. It will know what you mean as long as they are paired accordingly. That said, get into the habit of using single quotes as the outermost mark for anything which isn’t a string, HTML string, or JSON.
JavaScript允许您在同一条语句中使用单引号和双引号。 只要它们进行了配对,它就会知道您的意思。 也就是说,养成习惯使用单引号作为不是字符串,HTML字符串或JSON的任何内容的最外层标记。
()
alert('Say "Hello"');
alert("A string that's double quoted and contains a single quote");
alert('<div id="thing">');
var jsonObject = '{"foo":"bar"}';
控制台记录一切 (
Console Log Everything)
JavaScript troubleshooting is not straightforward. You should start by adding a console.log for all parts of a troublesome function. Logging makes it possible to differentiate between expected and observed behaviors. Maybe your setter isn’t working. Maybe your result is a string instead of a number. Maybe the element you’re targeting isn’t on the page. Without console logging it will be hard to track down what’s wrong.
JavaScript故障排除并不简单。 您应该首先为麻烦的功能的所有部分添加console.log。 日志记录可以区分预期行为和观察到的行为。 也许您的二传手没有工作。 也许您的结果是字符串而不是数字。 也许您定位的元素不在页面上。 如果没有控制台日志记录,将很难找到问题所在。
采用样式指南 (
Adopt a Styleguide)
Styleguides encourage good habits when writing code. Many large companies such as Google and Airbnb make their styleguides public online. It’s in your best interest to learn from their examples.
样式指南在编写代码时鼓励良好的习惯。 许多大型公司(例如Google和Airbnb)都会在网上公开其样式指南。 从他们的例子中学习是您的最大利益。
超越基础 (
Beyond the Basics)
Once you’ve mastered the basics of JavaScript without a framework like jQuery, you can move on to using server-side JavaScript via tools like Node.js, or database JavaScript via tools like MongoDB. These days there are even tools to build mobile, desktop, wearable, and IoT applications using JavaScript.
无需使用jQuery之类的框架即可掌握JavaScript的基础知识之后,就可以继续通过Node.js之类的工具来使用服务器端JavaScript,或者通过MongoDB之类的工具来使用数据库JavaScript。 如今,甚至有使用JavaScript构建移动,桌面,可穿戴和IoT应用程序的工具。
JavaScript is everywhere and it’s worth your time to learn how to use it. Luckily Live by Experts Exchange is here to connect you with talented JavaScript developers from around the world, instantly.
JavaScript无处不在,值得您花时间学习如何使用它。 通过Experts Exchange幸运地进行Live交流 ,可以立即与世界各地有才华JavaScript开发人员联系。
翻译自: https://www.experts-exchange.com/articles/28548/Get-Started-with-JavaScript.html
javascript入门