This is an example where I wanted a person to check one or more values in a list item, using a lookup column. Then, each of the selected items they chose had a value. I needed to get the sum of those values, perhaps have some multipliers on some of them, and get a weight value. It was not happening for me in SharePoint Designer or using calculated columns, but this was something that was simple using JavaScript or C#. What was I to do… OK well here is what I did, and it worked out pretty well actually…
这是一个示例,我希望一个人使用查阅列来检查列表项中的一个或多个值。 然后,他们选择的每个选定项目都有一个值。 我需要获取这些值的总和,也许在其中一些乘数上得到权重值。 对于我来说,这不是在SharePoint Designer中或使用计算所得的列发生的,但是使用JavaScript或C#却很简单。 我该怎么做...好吧,这就是我所做的,实际上效果很好...
First, a custom list called “listings” was made.
首先,创建了一个名为“列表”的自定义列表。
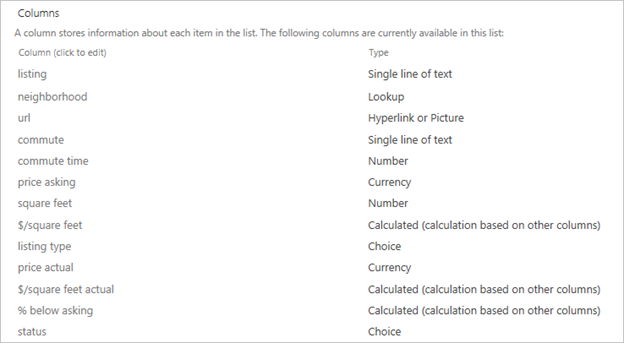
The purpose of the list was to keep track of real estate listings. We put certain criteria that were important here, such as details of the listing. Some things are one-to-one relationship, so those would be columns in this list such as the price, the square feet, etc. But…
该清单的目的是跟踪房地产清单。 我们在这里放置了一些重要的标准,例如清单的详细信息。 有些事情是一对一的关系,因此这些都是该列表中的列,例如价格,平方英尺等。但是……
Next, a separate custom list called “features” was made.
接下来,制作了一个单独的自定义列表,称为“功能”。
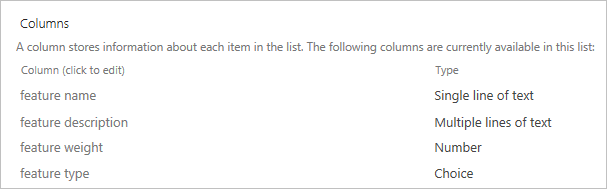
There are some criteria that there is a one-to-many relationship, where one listing might have several of the features we would want. This second list held a series of features, and each feature had a weight assigned which was a numerical value. The higher the weight, the more important it is to us.
有一些标准存在一对多关系,其中一个清单可能具有我们想要的几种功能。 第二个列表包含一系列功能,并且每个功能都有一个权重,该权重是一个数值。 重量越高,对我们来说就越重要。
Back to the “listings” custom list...
返回“列表”自定义列表...

I added a lookup column called “features” with “allow multiple values” enabled. Initially I had also checked the “add a column to show each of these additional fields” also enabled for the “feature weight” number value. The problem here was that…
我添加了一个名为“功能”的查询列,并启用了“允许多个值”。 最初,我还检查了“添加一列以显示这些附加字段中的每一个”,同时也为“特征权重”数字值启用了此功能。 这里的问题是……
1) this “features:feature weight” column would show up in the SharePoint list and views, but was not accessible for use in calculated columns.
1)此“功能:功能权重”列将显示在SharePoint列表和视图中,但不可在计算列中使用。
2) also, this same column was not accessible from within SharePoint Designer 2013 either.
2)此外,也无法从SharePoint Designer 2013中访问同一列。
The changes I made to begin addressing this was to just have the “features” column still there, without bothering to have the “feature weight” additional column. Then, a “Score” column with type set as “number” was added. This would be used later on…
为了解决这个问题,我所做的更改是仅保留“功能”列,而无需增加“功能权重”列。 然后,添加了一个“得分”列,其类型设置为“数字”。 稍后将使用...
The first part of the solution was to put some .js and .txt files into the Site Assets document library...
解决方案的第一部分是将一些.js和.txt文件放入站点资产文档库中...
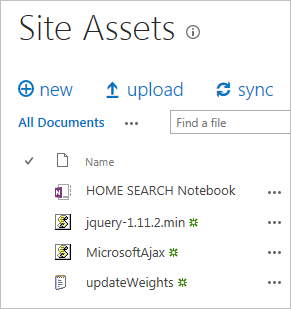
To be prepared, I put jQuery and MicrosoftAjax .js files, and a new empty updateWeights.txt file.
为了准备,我放置了jQuery和MicrosoftAjax .js文件,以及一个新的空updateWeights.txt文件。
*One place you can find the MicrosoftAjax.js file is at: http://ajax.aspnetcdn.com/ajax/4.0/1/MicrosoftAjax.js
*You can get jQuery at: https://jquery.com/download/
*您可以在以下位置找到MicrosoftAjax.js文件的位置: http : //ajax.aspnetcdn.com/ajax/4.0/1/MicrosoftAjax.js
*您可以通过以下网址获取jQuery: https : //jquery.com/download/
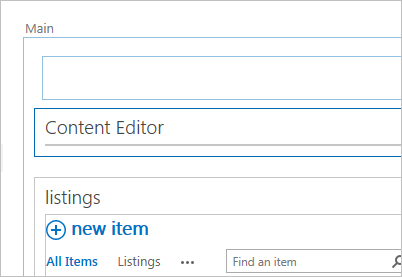
On the “listings” custom view page, I added a Content Editor web part to the page.
在“列表”自定义视图页面上,我在页面上添加了内容编辑器Web部件。
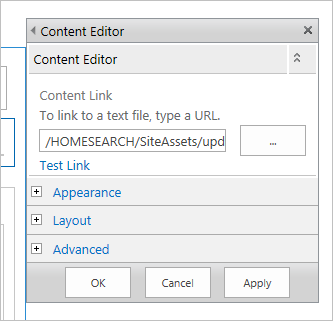
I set the Content Editor properties so that the “Content Link” option was set to point to the updateWeights.txt file.
我设置了内容编辑器属性,以便将“内容链接”选项设置为指向updateWeights.txt文件。

The updateWeights.txt file starts out with references fo the jQuery and MicrosoftAjax files.
updateWeights.txt文件以jQuery和MicrosoftAjax文件的引用开头。
Then another JavaScript section that has all of the other code...
然后是另一个JavaScript部分,其中包含所有其他代码...
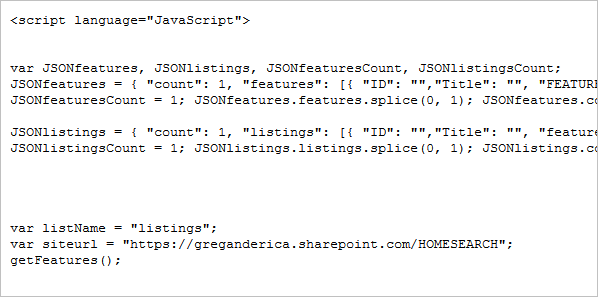
This may not be the best way to accomplish what is probably a simple task, but what I did was just create two JSON arrays to dump the data from “features” and “listings” into. Then let’s say one listing had four or five features tagged in that records. The weights for each of the tagged features would be added up, then that sum value would be sent back up to update the listing record…
这可能不是完成可能是一个简单任务的最佳方法,但是我所做的只是创建两个JSON数组以将数据从“功能”和“列表”转储到其中。 然后,假设一个清单在该记录中标记了四个或五个功能。 每个标记功能的权重将相加,然后将该总和发送回去以更新列表记录…

There is a function called GetItemTypeForListName, but I actually didn’t use this because it was giving errors later on when trying to use. For me, I just ended up finding and hard-coding the item type later on.
有一个名为GetItemTypeForListName的函数,但实际上我没有使用它,因为稍后在尝试使用时会出现错误。 对我来说,我最后才找到项目类型并对其进行硬编码。
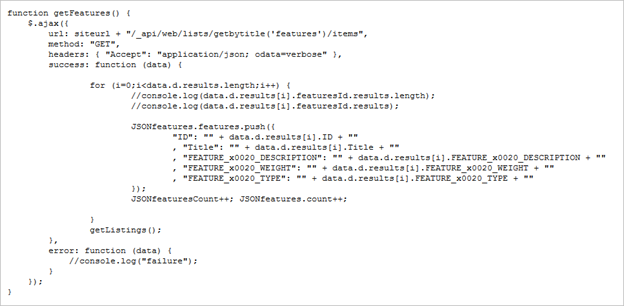
I made the first function called getFeatures(), which uses ajax to pull down the list of features into a JSONfeatures JSON array. Then once done, this calls the next function called getListings()…
我制作了第一个名为getFeatures()的函数,该函数使用ajax将功能列表下拉到JSONfeatures JSON数组中。 然后,一旦完成,它将调用下一个名为getListings()的函数。
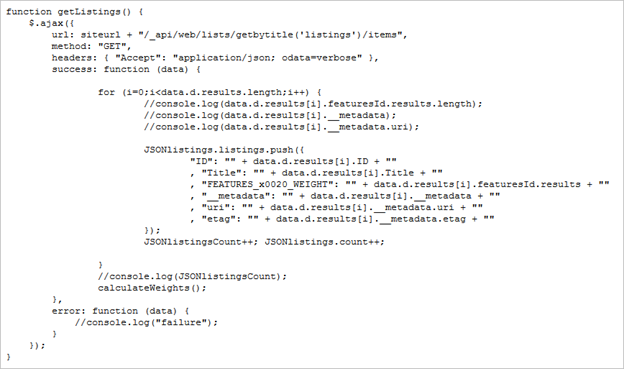
getListings() does the same thing as getFeatures(), but to the JSONlistings JSON array. Once done, this calls a calculateWeights() function…
getListings()与getFeatures()的作用相同,但是对JSONlistings JSON数组具有相同的作用。 完成后,这将调用calculateWeights()函数…
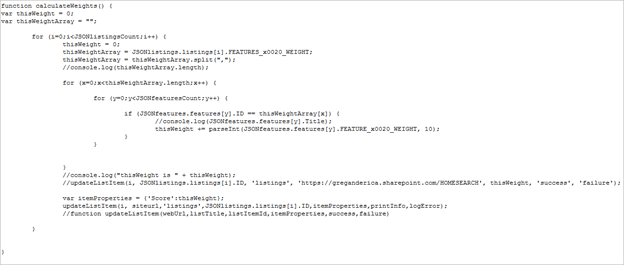
This function loops through each of the listings, and for each one, it loops through the list of tagged features. For each tagged feature, it loops through the list of features to find the weight for that specific feature. This is added to a temporary variable that sums up how much overall weight the listing has. Once the features are all looped through, the current listing item is updated by a call to a updateListItem() function. Then the process is repeated for the next listing.
此功能循环遍历每个清单,并且对于每个清单,遍历标记的功能列表。 对于每个标记的功能,它会循环浏览功能列表,以查找该特定功能的权重。 这被添加到一个临时变量中,该变量汇总了列表的总权重。 一旦所有功能都经过遍历,就可以通过调用updateListItem()函数来更新当前列表项。 然后为下一个清单重复该过程。
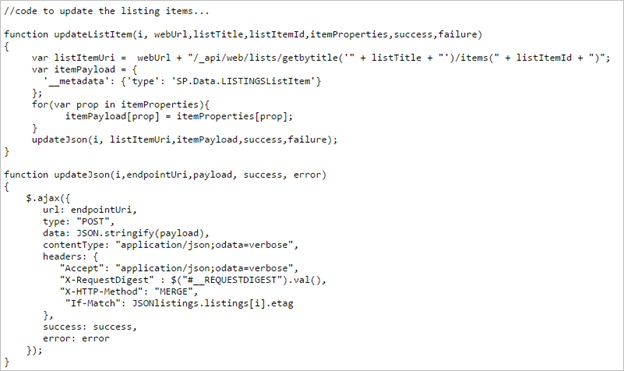
The updateListItem() function is sent the JSON item ID, the website URL, the title of the list, the list item ID to be updated, the “Score” value to merge, and the names of which functions to call upon success or failure. This function puts it all together, then sends it to another function called updateJson() which gets the JSON item ID, the endpoint URI which is basically the URL reformatted to be sent to the API, the payload which is basically the list of properties to be updated, and the success/failure functions again.
向updateListItem()函数发送JSON项ID,网站URL,列表标题,要更新的列表项ID,要合并的“ Score”值以及成功或失败时要调用的函数的名称。 此函数将所有内容放在一起,然后将其发送到另一个名为updateJson()的函数,该函数获取JSON项ID,端点URI(基本上是将其重新格式化为要发送给API的URL),有效负载(基本上是要获取的属性的列表)进行更新,然后成功/失败再次起作用。
The end result is that...
最终结果是...
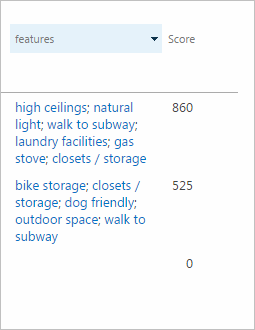
...the listings view gets the Score column updated with the sum of all features that are tagged from within that listing’s edit page. Phew!
...列表视图会更新“分数”列,其中会更新该列表的编辑页面中标记的所有功能的总和。 !
I put all my related files that I used in this example up on my newly setup GitHub repository, in case it might be useful for anyone else (and to make it easier for myself to use in the future). The URL for that, which is where you can get the full updateWeights.txt example file, is at:
https://github.com/gregbesso/SharePoint-JavaScriptAPI-Example
我将本示例中使用的所有相关文件放在新设置的GitHub存储库中,以防它对其他任何人有用(并使其将来更易于使用)。 可以在其中获得完整的updateWeights.txt示例文件的URL位于:
https://github.com/gregbesso/SharePoint-JavaScriptAPI-示例
If anyone finds this useful, let me know. Would like to see if other people run into similar situations like I did. Or if there was an easier way to accomplish this same thing let me know also 😛
如果有人觉得这有用,请告诉我。 想看看其他人是否也遇到过像我一样的情况。 或者如果有更简单的方法来完成相同的事情,请让我知道😛
Also, for the full MSDN reference of the JavaScript API for SharePoint 2013, check out this link:
https://msdn.microsoft.com/en-us/library/office/jj193034.aspx
另外,有关SharePoint 2013JavaScript API的完整MSDN参考,请查看以下链接:
https://msdn.microsoft.com/zh-CN/library/office/jj193034.aspx