javascript编程题
In this article I'll describe cross-browser techniques for using the mouse wheel (or "scroll wheel") in your JavaScript programming for HTML pages. I'll show example Javascript for option selection and image zooming.
在本文中,我将介绍跨浏览器技术,这些技术在HTML页面JavaScript编程中使用鼠标滚轮(或“滚轮”)。 我将显示用于选择选项和图像缩放的示例Javascript。
The mouse wheel is generally used for scrolling, but it can also be convenient for other U/I functionality. Image zooming is a common use; for instance in Google Maps, spinning the mouse wheel zooms you in or out. I've seen the mouse wheel used to raise or lower the "camera viewpoint" in first-person 3D games. Another use I've seen is to cycle though a series of objects. In HalfLife, et al., you can choose a weapon with the wheel -- though it's a bit awkward (it's better to use the direct choice keys [1,2,3...] if you want to avoid becoming fragmeat by the time you find the weapon you want.)
鼠标滚轮通常用于滚动,但对于其他U / I功能也很方便。 图像缩放是常见的用法; 例如在Google Maps中,旋转鼠标滚轮可以放大或缩小。 我已经看到了用于提高或降低第一人称3D游戏中“摄像机视点”的鼠标滚轮。 我看到的另一个用途是循环一系列对象。 在HalfLife等人中,您可以选择带有方向盘的武器-尽管有点笨拙(如果要避免被车轮撞碎,最好使用直接选择键[1,2,3 ...]找到所需武器的时间。)
I was revising an old JavaScript program I'd written years ago (when mouse wheels were uncommon) and I realized that I could improve the UI by supporting mousewheel events.
我正在修改几年前(当鼠标滚轮不常见时)编写的旧JavaScript程序,我意识到可以通过支持鼠标滚轮事件来改善UI。
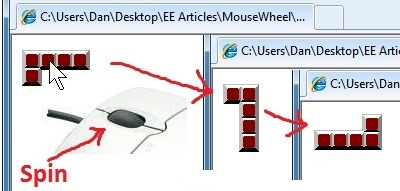
The goal of Pentominos is to place all 12 pieces into the puzzle board. But each piece can be rotated and/or flipped in various ways, so there are a total of 62 unique shapes (piece orientations). In the earlier version, each time you click on a piece in the lower frame, it would cycle from one orientation to the next. This version still handles that, but it also lets you use the mouse wheel to spin through the options.
Pentominos的目标是将所有12个碎片放入拼图板。 但是每个零件都可以通过各种方式旋转和/或翻转,因此共有62种独特的形状(零件方向)。 在较早的版本中,每次单击下部框架中的一块时,它将从一个方向循环到另一个方向。 这个版本仍然可以解决这个问题,但是它也允许您使用鼠标滚轮来旋转选项。
One challenge of supporting the mouse wheel is that there are differences in how each of the major webbrowsers implement the event handling. In the following code, I'll show the basic technique that works for (at least) IE, Firefox, and Chrome.
支持鼠标滚轮的一个挑战是,每个主要的网络浏览器在实现事件处理方面的方式都存在差异。 在以下代码中,我将展示适用于(至少)IE,Firefox和Chrome的基本技术。
What do you need to know about a mousewheel event?
关于鼠标滚轮事件,您需要了解什么?
The direction of the spin. We'll set up a 旋转的方向。 我们将 delta value of +1 to mean "forward/larger" and +1表示“向前/更大”,将 -1 to mean "backward/smaller." -1表示为“向后/较小”。
Mouse location. The X,Y coordinates in the event object are the same as for mouse clicks, and they can be used without any special handling. 鼠标位置。 事件对象中的X,Y坐标与单击鼠标时的坐标相同,无需任何特殊处理即可使用它们。
The on-screen object that is in play. This can be determined by the mouse location OR you can assign a separate event listener for each object that can be affected by wheel spins (that's the technique I'll show here). 屏幕上正在播放的对象。 这可以通过鼠标的位置来确定,也可以为每个可能受滚轮旋转影响的对象分配一个单独的事件侦听器(这就是我将在此处显示的技术)。
How much of a spin was given. The mouse wheel itself usually works in single-step increments. You roll the wheel one "notch" at a time, and each "wheel click" is an individual event. It is possible to keep track of these events and synthesize a "velocity" or "distance" even an "inertia" for use in your program, but we won't go into that here. 旋转了多少。 鼠标滚轮本身通常以单步增量工作。 您一次滚动滚轮一个“凹口”,每次“滚轮咔嗒”都是一个单独的事件。 可以跟踪这些事件并合成“速度”或“距离”甚至是“惯性”以供您在程序中使用,但是我们在这里不做介绍。
In the following code snippets, the HTML page is assumed to include...
在以下代码段中,假定HTML页面包括...
<body onload="DoSetup();">
<div id="oInfo"></div>
<img id="oImage" src="PL0.jpg" alt="The 'L' Piece">
oImage that will be the target of mousewheel events and a DIV for debugging and displaying information.
oImage将成为鼠标滚轮事件的目标,以及用于调试和显示信息的DIV。
Here's the core of this article... the actual cross-browser event handler:
这是本文的核心...实际的跨浏览器事件处理程序:
[step="" title="Event Handler"]
[step =“” title =“事件处理程序”]
function onMouseWheelSpin(e) {
var nDelta = 0;
if (!e) { // For IE, access the global (window) event object
e = window.event;
}
// cross-bowser handling of eventdata to boil-down delta (+1 or -1)
if ( e.wheelDelta ) { // IE and Opera
nDelta= e.wheelDelta;
if ( window.opera ) { // Opera has the values reversed
nDelta= -nDelta;
}
}
else if (e.detail) { // Mozilla FireFox
nDelta= -e.detail;
}
if (nDelta > 0) {
HandleMouseSpin( 1, e.clientX, e.clientY );
}
if (nDelta < 0) {
HandleMouseSpin( -1, e.clientX, e.clientY );
}
if ( e.preventDefault ) { // Mozilla FireFox
e.preventDefault();
}
e.returnValue = false; // cancel default action
}
[step="" title="Event Handler Setup"]
[step =“” title =“事件处理程序设置”]
//-------- set up to handle wheel events on object: oImage
function DoSetup() {
if (oImage.addEventListener) {
oImage.addEventListener('DOMMouseScroll', onMouseWheelSpin, false);
oImage.addEventListener('mousewheel', onMouseWheelSpin, false); // Chrome
}
else {
oImage.onmousewheel= onMouseWheelSpin;
}
}
<img id="oImage" src="PL0.jpg" onmousewheel="SomeFn()">
<img id =“ oImage” src =“ PL0.jpg” onmousewheel =“
but using a DoSetup() function like this is the most flexible since it lets you use some cross-browser compatibility logic, as shown.
但是使用这样的DoSetup()函数是最灵活的,因为它使您可以使用一些跨浏览器的兼容性逻辑,如图所示。
[/step]
[/步]
We have an object (oImage) and a way to set up a wheel handler, so now we just need to provide some program code that will do something with or to that object when the mousewheel spins on it. Each "wheel click" will trigger the event which will call your custom function named HandleMouseSpin().
我们有一个对象( oImage )和一种设置滚轮处理程序的方法,因此现在我们只需要提供一些程序代码即可在鼠标滚轮旋转时对该对象进行处理。 每次“滚轮单击”都会触发该事件,该事件将调用名为HandleMouseSpin()的自定义函数。
Item/Option Selection
项目/选项选择
In this first example, we'll cycle through the eight possible orientations for a particular pentomino piece, as illustrated in fig1-1, above. I have eight JPG files named PL0.jpg, PL1.jpg,... PL7.jpg so all I need to do is is change the src attribute of that object to swap-in a different version of the puzzle piece. A "wheel forward" event (delta= +1) goes forward though the list and a "wheel backward" event (delta= -1) goes backward though the list. Upon reaching the end of the list, it wraps to the other end.
在第一个示例中,我们将针对一个特定的戊米诺骨肉片循环浏览八个可能的方向,如上面的图1-1所示。 我有八个JPG文件,分别名为PL 0 .jpg,PL 1 .jpg,... PL 7 .jpg,因此,我要做的就是更改该对象的src属性,以换入其他版本的拼图。 “ Wheel Forward”事件( delta = +1 )通过列表前进,而“ Wheel backing”事件( delta = -1 )则通过列表前进。 到达列表的末尾时,它会绕到另一端。
[step="" title="Example HandleMouseSpin function"]Item Selection
[step =“” title =“ HandleMouseSpin函数示例”] 项目选择
//----------- change the image from PL0.jpg to PL1, PL2,... PL7... PL0
var gnOrient = 0;
function HandleMouseSpin( nDelta, x,y ) {
gnOrient += nDelta;
if (gnOrient < 0) gnOrient = 7;
if (gnOrient > 7) gnOrient = 0;
oImage.src="PL"+gnOrient+".jpg";
oInfo.innerHTML = "Delta: " + nDelta + "<br>" + oImage.src;
}
Image Magnification
图像放大
As a second example, we'll use the same HTML, but change the handler so that mousewheel action enlarges or shrinks the image.
作为第二个示例,我们将使用相同HTML,但是更改处理程序,以使鼠标滚轮操作放大或缩小图像。
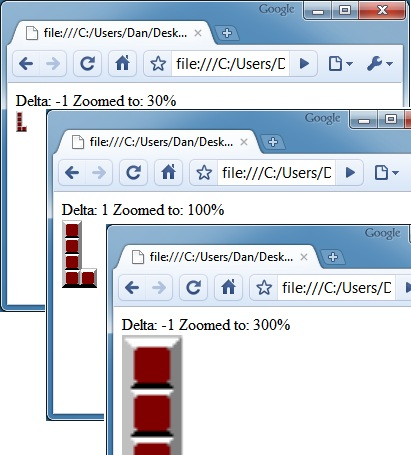
[step="" title="Example HandleMouseSpin function"]Image Zoom
[step =“” title =“ HandleMouseSpin函数示例”] 图片缩放
//----------- increase/decrease the size of the image
var gnOrigWide = -1;
var gnZoomLevel= 100;
function HandleMouseSpin(nDelta, x, y) {
if (gnOrigWide == -1) {
gnOrigWide = oImage.width;
}
gnZoomLevel += nDelta * 10;
if ( gnZoomLevel < 10) gnZoomLevel=10;
var nNewWide = (gnOrigWide * gnZoomLevel) / 100;
oImage.width = nNewWide;
oInfo.innerHTML = "Delta: " + nDelta + "<br>zoomed to: " + gnZoomLevel +"%";
}
Summary
摘要
This article shows how to add cross-browser mousewheel awareness to your HTML pages. Since virtually every mouse has a "scroll wheel" nowadays, it makes sense to use it to enrich the experience of your viewer.
本文介绍如何向HTML页面添加跨浏览器的鼠标滚轮感知。 由于如今几乎每只鼠标都有一个“滚轮”,因此使用它来丰富查看器的体验是很有意义的。
However, you should take care to not override the normal functioning of the mousewheel unless there is good reason...
但是,除非有充分的理由,否则应注意 ...
Users expect to be able to use the wheel for normal page (and textarea) scrolling. But when you have a non-scrolling page, or if you handle the event only on specific objects (not the document or the window as a whole) then it can be a useful shortcut for your users.
用户希望能够使用滚轮进行正常的页面(和文本区域)滚动。 但是,当您拥有非滚动页面时,或者如果仅在特定对象(而不是整个文档或窗口 )上处理事件,则对于用户而言,它可能是有用的快捷方式。
=-=-=-=-=-=-=-=-=-=-=-=-=-
=-=-=-=-=-=-=-=-=-=-=-=-=- =-=-=-=-=- =-=-=-=-=- =-=-=-=-=- =-=-=-=-=- =-=-=-=-=- =-=-=-=
If you liked this article and want to see more from this author, please click the Yes button near the:
如果您喜欢这篇文章,并希望从该作者那里获得更多信息,请单击旁边的是按钮:
Was this article helpful?
本文是否有帮助?
label that is just below and to the right of this text. Thanks!
此文字下方和右侧的标签。
=-=-=-=-=-=-=-=-=-=-=-=-=-
=-=-=-=-=-=-=-=-=-=-=-=-=- =-=-=-=-=- =-=-=-=-=- =-=-=-=-=- =-=-=-=-=- =-=-=-=-=- =-=-=-=
翻译自: https://www.experts-exchange.com/articles/2281/Mouse-Wheel-Programming-in-JavaScript.html
javascript编程题