c语言 ++ --运算符
Operators are special type of functions, that takes one or more arguments and produces a new value. For example : addition (+), substraction (-), multiplication (*) etc, are all operators. Operators are used to perform various operations on variables and constants.
运算符是函数的一种特殊类型,它接受一个或多个参数并产生一个新值。 例如:加法(+),减法(-),乘法(*)等都是运算符。 运算符用于对变量和常量执行各种操作。
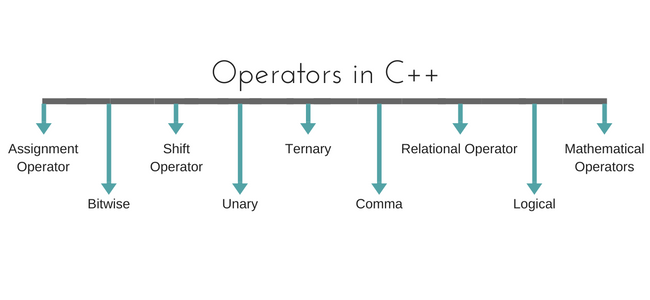
运营商类型 (Types of operators)
Assignment Operator
赋值运算符
Mathematical Operators
数学运算符
Relational Operators
关系运算符
Logical Operators
逻辑运算符
Bitwise Operators
按位运算符
Shift Operators
移位运算符
Unary Operators
一元运算符
Ternary Operator
三元运算符
Comma Operator
逗号运算符
赋值运算符( =
) (Assignment Operator (=
))
Operates '=' is used for assignment, it takes the right-hand side (called rvalue) and copy it into the left-hand side (called lvalue). Assignment operator is the only operator which can be overloaded but cannot be inherited.
操作符“ =”用于赋值,它采用右侧(称为rvalue)并将其复制到左侧(称为lvalue)。 赋值运算符是唯一可以重载但不能继承的运算符。
数学运算符 (Mathematical Operators)
There are operators used to perform basic mathematical operations. Addition (+) , subtraction (-) , diversion (/) multiplication (*) and modulus (%) are the basic mathematical operators. Modulus operator cannot be used with floating-point numbers.
有用于执行基本数学运算的运算符。 基本的数学运算符是加法(+),减法(-),转移(/)乘积(*)和模数(%)。 模运算符不能与浮点数一起使用。
C++ and C also use a shorthand notation to perform an operation and assignment at same type. Example,
C ++和C还使用简写形式来执行相同类型的操作和赋值。 例子
int x=10;
x += 4 // will add 4 to 10, and hence assign 14 to X.
x -= 5 // will subtract 5 from 10 and assign 5 to x.
关系运算符 (Relational Operators)
These operators establish a relationship between operands. The relational operators are : less than () , less than or equal to (<=), greater than equal to (>=), equivalent (==) and not equivalent (!=).
这些运算符在操作数之间建立关系。 关系运算符是:小于(),小于或等于(<=),大于等于(> =),等效(==)和不等效(!=)。
You must notice that assignment operator is (=) and there is a relational operator, for equivalent (==). These two are different from each other, the assignment operator assigns the value to any variable, whereas equivalent operator is used to compare values, like in if-else conditions, Example
您必须注意,赋值运算符为(=),并且有一个关系运算符,相当于(==)。 这两个彼此不同,赋值运算符将值分配给任何变量,而等效运算符用于比较值,例如在if-else条件下, 示例
int x = 10; //assignment operator
x=5; // again assignment operator
if(x == 5) // here we have used equivalent relational operator, for comparison
{
cout <
逻辑运算符 (Logical Operators)
The logical operators are AND (&&) and OR (||). They are used to combine two different expressions together.
逻辑运算符为AND(&&)和OR(||)。 它们用于将两个不同的表达式组合在一起。
If two statement are connected using AND operator, the validity of both statements will be considered, but if they are connected using OR operator, then either one of them must be valid. These operators are mostly used in loops (especially while
loop) and in Decision making.
如果使用AND运算符连接了两个语句,则将考虑两个语句的有效性,但是如果使用OR运算符连接两个语句,则其中之一必须有效。 这些运算符主要用于循环(尤其是while
循环)和决策制定中。
按位运算符 (Bitwise Operators)
There are used to change individual bits into a number. They work with only integral data types like char
, int
and long
and not with floating point values.
用于将单个位更改为数字。 它们仅适用于整型数据类型,例如char
, int
和long
,而不适用于浮点值。
Bitwise AND operators
&
按位与运算符
&
Bitwise OR operator
|
按位或运算符
|
And bitwise XOR operator
^
和按位XOR运算符
^
And, bitwise NOT operator
~
并且,按位NOT运算符
~
They can be used as shorthand notation too, & =
, |=
, ^=
, ~=
etc.
它们也可以用作速记符号, & =
, |=
, ^=
, ~=
等。
移位运算符 (Shift Operators)
Shift Operators are used to shift Bits of any variable. It is of three types,
移位运算符用于移位任何变量的位。 它有三种类型,
Left Shift Operator
<<
左移运算符
<<
Right Shift Operator
>>
右移运算符
>>
Unsigned Right Shift Operator
>>>
无符号右移运算符
>>>
一元运算符 (Unary Operators)
These are the operators which work on only one operand. There are many unary operators, but increment ++
and decrement --
operators are most used.
这些是仅对一个操作数起作用的运算符。 一元运算符有很多,但是递增++
和减量--
运算符最常用。
Other Unary Operators : address of &
, dereference *
, new and delete, bitwise not ~
, logical not !
, unary minus -
and unary plus +
.
其他一元运算符: &
地址,dereference *
, new和delete ,按位不~
,逻辑不!
,一元减-
和一元加+
。
三元运算符 (Ternary Operator)
The ternary if-else ? :
is an operator which has three operands.
三元if-else ? :
? :
是具有三个操作数的运算符。
int a = 10;
a > 5 ? cout << "true" : cout << "false"
逗号运算符 (Comma Operator)
This is used to separate variable names and to separate expressions. In case of expressions, the value of last expression is produced and used.
这用于分隔变量名和表达式。 对于表达式,将生成并使用最后一个表达式的值。
Example :
范例 :
int a,b,c; // variables declaration using comma operator
a=b++, c++; // a = c++ will be done.
翻译自: https://www.studytonight.com/cpp/operators-and-their-types.php
c语言 ++ --运算符