python中的oop
As we have already discussed about the general concepts related to Class, Objects, Inheritance etc, using an example in the previous tutorial. In this tutorial, we will elaborate more on these topics.
正如我们已经使用上一教程中的示例讨论了与类,对象,继承等相关的一般概念一样。 在本教程中,我们将详细介绍这些主题。
对象 (Objects)
As already discussed in the previous tutorial, an object is a physical entity, whereas class is a logical defintion. An object can be anything like - a student while designing a school's record registry, or a pen in stationary's item management program, or a car in manufacture's car database program. There are three important characteristics by which it is identified, they are:
如上一教程中已讨论的,对象是物理实体,而类是逻辑定义。 对象可以是任何东西,例如,学生在设计学校的记录注册表时,或文具的物品管理程序中的笔,或制造商的汽车数据库程序中的汽车。 识别它的三个重要特征是:
Identity: Identity refers to some piece of information that can be used to identify the object of a class. It can be the name of the student, the company name of the car, etc.
身份 :身份是指可用于识别类对象的某些信息。 它可以是学生的姓名,汽车的公司名称等。
Properties: The attributes of that object are called properties. Like age, gender, DOB for a student; or type of engine, number of gears for a car.
属性 :该对象的属性称为属性。 像年龄,性别,学生DOB; 或发动机的类型,汽车的齿轮数。
Behaviour: Behaviour of any object is equivalent to the functions that it can perform. In OOP it is possible to assign some functions to objects of a class. Taking the example forward, like a student can read/write, the car can accelerate and so on.
行为 :任何对象的行为都等同于它可以执行的功能。 在OOP中,可以将某些功能分配给类的对象。 举例来说,就像学生可以读/写,汽车可以加速等等。
类 (Class)
A class is a blueprint where attributes and behaviour is defined. For example, if Mahatma Gandhi, Sachin Tendulkar, you and me are objects, then Human Being is a class. An object is the fundamental concept of OOP but classes provide an ability to define similar type of objects.
类是定义属性和行为的蓝图。 例如,如果圣雄甘地,萨钦·滕杜尔卡(Sachin Tendulkar),您和我是对象,那么人类就是一类。 对象是OOP的基本概念,但是类提供了定义相似类型的对象的能力。
In class, both data, and the functions that will be operating on that data are bundled as a unit.
在课堂上,数据和将对该数据进行操作的功能都作为一个单元捆绑在一起。
For example, let's say there is a class named Car
, which has some basic data like - name, model, brand, date of manufacture, engine etc, and some functions like turn the engine on, apply brakes, accelerate, change gear, blow horn etc. Now that all the basic features are defined in our class Car
, we can create its objects by setting values for properties name, model etc, and the object of the class Car
will be able to use the functions defined in it.
例如,假设有一个名为Car
的类,它具有一些基本数据,例如名称,型号,品牌,制造日期,发动机等,以及一些功能,如打开发动机 , 施加制动 , 加速 , 改变档位 , 打击喇叭等。现在,所有的基本功能都在我们的类中定义的Car
,我们可以通过对属性名称,型号等,该类的对象设定值来创建它的对象Car
将能够使用它定义的函数。
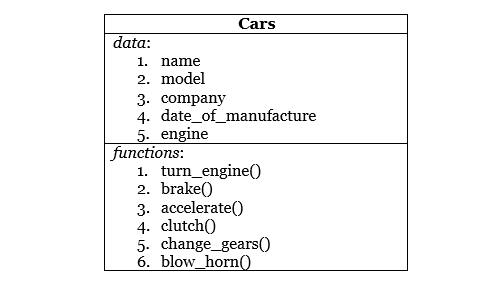
资料隐藏 (Data Hiding)
Data hiding helps us to define the privacy of data from the outside world or to be precise, from other classes. The reason behind doing this is to create several levels of accessing an object's data and prevent it from accidental modification. Also, hiding, or setting up privacy levels, can be done for functions as well.
数据隐藏可帮助我们定义来自外部世界的数据隐私,或者确切地说,来自其他类别的数据。 这样做的原因是要创建访问对象数据的多个级别,并防止意外修改。 同样,也可以针对功能进行隐藏或设置隐私级别。
In OOP, data inside the classes can be defined as public, private or protected. Private data or function are the ones that cannot be accessed or seen from outside the class whereas, public data or functions can be accessed from anywhere. Protected data or functions, more or less act like public but should not be accessed from outside.
在OOP中,可以将类内的数据定义为public , private或protected 。 私有数据或功能是无法从课堂之外访问或看到的,而公共数据或功能则可以从任何地方访问。 受保护的数据或功能或多或少地像公共行为,但不应从外部访问。
数据抽象 (Abstraction of Data)
Classes use the concept of abstraction. A class encapsulates the relevant data and functions that operate on data by hiding the complex implementation details from the user. The user needs to focus on what a class does rather than how it does.
类使用抽象的概念。 类通过向用户隐藏复杂的实现细节来封装相关数据和对数据进行操作的功能。 用户需要关注类的工作方式,而不是工作方式。
封装形式 (Encapsulation)
Encapsulation, is one of the core reason for the existence of an object. Since the beginning we have been talking about objects, its data, functions, privacy etc., now it's time to know how is all this kept bounded. The answer is encapsulation. Much as it may sound like a capsule, it is pretty much the same. Here we try to encapsulate the data and functions together which belongs to the same class.
封装是对象存在的核心原因之一。 从一开始我们就一直在谈论对象,其数据,功能,隐私等,现在是时候知道所有这些如何保持界限了。 答案是封装。 尽管听起来像是胶囊,但几乎是一样的。 在这里,我们尝试将属于同一类的数据和函数封装在一起。
遗产 (Inheritance)
As explained in the previous tutorial, inheritance is about defining a set of core properties and functions in one place and then re-using them by inheriting the class in which they are defined.
如上一教程中所述,继承是在一个地方定义一组核心属性和函数,然后通过继承定义它们的类来重用它们。
Python supports Simple, Multiple and MultiLevel Inheritance. We will be covering these in details in inheritance tutorial.
Python支持Simple , Multiple和MultiLevel继承。 我们将在继承教程中详细介绍这些内容。
多态性 (Polymorphism)
Polymorphism, or Poly + Morph, means "many formsb. Precisely, Polymorphism is the property of any function or operator that can behave differently depending upon the input that they are fed with.
“多态”或“ Poly + Morph ”的意思是“许多形式 。准确地说,多态是任何函数或运算符的属性,根据输入的内容,它们的行为可能有所不同。
Polymorphism can be achieved in tow different forms, they are:
多态性可以以两种不同的形式实现,它们是:
功能重载 (Function Overloading)
In OOP, it is possible to make a function act differently using function overloading. All we have to do is, create different functions with same name having different parameters. For example, consider a function add()
, which adds all its parameters and returns the result. In python we will define it as,
在OOP中,可以使用函数重载使函数的行为有所不同。 我们要做的就是创建具有相同名称,具有不同参数的不同函数。 例如,考虑一个函数add()
,它添加所有参数并返回结果。 在python中,我们将其定义为
def add(a, b):
return a + b;
This is capable of executing function calls like:
这能够执行如下函数调用:
>>> add(4,5)
The above add
function will always take 2 numbers as input, but what if you want to add 3 numbers at once or maybe 4 numbers.
上面的add
功能将始终以2个数字作为输入,但是如果您想一次添加3个数字或4个数字,该怎么办。
Hence, in OOP you can simply define the function add
once again, this time with 3 parameters, and this mechanism is known as Function Overloading.
因此,在OOP中,您可以简单地再次定义函数add
,这次使用3个参数,这种机制称为Function Overloading 。
# to add 3 numbers
def add(a, b, c):
return a + b + c;
# to add 4 numbers
def add(a, b, c, d):
return a + b + c + d;
Now we can call add()
function with two, three or four parameters. As you can see here, function add()
now has multiple forms.
现在我们可以使用两个,三个或四个参数调用add()
函数。 如您所见,函数add()
现在具有多种形式。
运算符重载 (Operator Overloading )
You know what operators are: Addition, Division, Multiplication etc. Python is capable of reading operators differently depending upon the situation. For example,
您知道什么是运算符: 加法 , 除法 , 乘法等。Python能够根据情况以不同的方式读取运算符。 例如,
>>>print(2 + 5)
It will give you output 7
, but doing
它会给你输出7
,但是
>>>print( "hello" + "world")
Gives you output "helloworld"
. We can see here, how +
operator when used with numbers performs mathematical addition operation but when used with strings it performs concatenation.
给您输出"helloworld"
。 我们在这里可以看到, +
运算符与数字一起使用时如何执行数学加法运算,而与字符串一起使用时如何执行串联运算。
Similarly, the multiplication operator also acts differently based on the datatype of the variables with which it is used. For example,
类似地,乘法运算符还根据与其一起使用的变量的数据类型采取不同的行动。 例如,
>>>print( 3*7)
Gives, 21
, while on string,
在弦上给出21
>>> print("hello"*3)
it gives, "hellohellohello"
. This is yet another example of operator overloading.
它给出了"hellohellohello"
。 这是操作员重载的另一个例子。
python中的oop