Stack as we know is a Last In First Out(LIFO) data structure. It has the following operations :
众所周知,堆栈是后进先出(LIFO)数据结构。 它具有以下操作:
push: push an element into the stack
推入:将元素推入堆栈
pop: remove the last element added
pop:删除最后添加的元素
top: returns the element at top of stack
top:返回堆栈顶部的元素
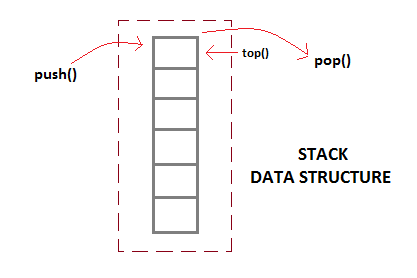
使用链表实现堆栈 (Implementation of Stack using Linked List)
Stacks can be easily implemented using a linked list. Stack is a data structure to which a data can be added using the push()
method and data can be removed from it using the pop()
method. With Linked list, the push operation can be replaced by the addAtFront()
method of linked list and pop operation can be replaced by a function which deletes the front node of the linked list.
使用链接列表可以轻松实现堆栈。 堆栈是一种数据结构,可以使用push()
方法向其中添加数据,并可以使用pop()
方法从中删除数据。 使用链接列表时,可以用链接列表的addAtFront()
方法代替推入操作,而可以用删除链接列表的前节点的函数来代替弹出操作。
In this way our Linked list will virtually become a Stack with push()
and pop()
methods.
这样,我们的链接列表实际上将通过push()
和pop()
方法变成一个堆栈。
First we create a class node. This is our Linked list node class which will have data in it and a node pointer to store the address of the next node element.
首先,我们创建一个类节点 。 这是我们的链接列表节点类,该类中将包含数据和一个节点指针以存储下一个节点元素的地址。
class node
{
int data;
node *next;
};
Then we define our stack class,
然后我们定义堆栈类,
class Stack
{
node *front; // points to the head of list
public:
Stack()
{
front = NULL;
}
// push method to add data element
void push(int);
// pop method to remove data element
void pop();
// top method to return top data element
int top();
};
将数据插入堆栈(链接列表) (Inserting Data in Stack (Linked List))
In order to insert an element into the stack, we will create a node and place it in front of the list.
为了将元素插入堆栈,我们将创建一个节点并将其放置在列表的前面。
void Stack :: push(int d)
{
// creating a new node
node *temp;
temp = new node();
// setting data to it
temp->data = d;
// add the node in front of list
if(front == NULL)
{
temp->next = NULL;
}
else
{
temp->next = front;
}
front = temp;
}
Now whenever we will call the push()
function a new node will get added to our list in the front, which is exactly how a stack behaves.
现在,每当我们调用push()
函数时,都会在前面的列表中添加一个新节点,这正是堆栈的行为方式。
从堆栈中删除元素(链接列表) (Removing Element from Stack (Linked List))
In order to do this, we will simply delete the first node, and make the second node, the head of the list.
为此,我们将简单地删除第一个节点,并使第二个节点成为列表的开头。
void Stack :: pop()
{
// if empty
if(front == NULL)
cout << "UNDERFLOW\n";
// delete the first element
else
{
node *temp = front;
front = front->next;
delete(temp);
}
}
返回栈顶(链接列表) (Return Top of Stack (Linked List))
In this, we simply return the data stored in the head of the list.
在此,我们仅返回存储在列表开头的数据。
int Stack :: top()
{
return front->data;
}
结论 (Conclusion)
When we say "implementing Stack using Linked List", we mean how we can make a Linked List behave like a Stack, after all they are all logical entities. So for any data structure to act as a Stack, it should have push()
method to add data on top and pop()
method to remove data from top. Which is exactly what we did and hence accomplished to make a Linked List behave as a Stack.
当我们说“使用链表实现栈”时,是指我们如何使链表的行为像栈一样,毕竟它们都是逻辑实体。 因此,对于要用作堆栈的任何数据结构,它都应具有push()
方法在顶部添加数据,而pop()
方法从顶部删除数据。 这正是我们所做的并因此完成的工作,以使链接列表的行为像堆栈一样。
翻译自: https://www.studytonight.com/data-structures/stack-using-linked-list