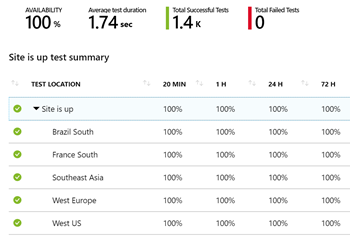
ASP.NET Core 2.2 is out and released and upgrading my podcast site was very easy. Once I had it updated I wanted to take advantage of some of the new features.
ASP.NET Core 2.2已发布并发布,升级我的播客站点非常容易。 更新后,我想利用一些新功能。
For example, I have used a number of "health check" services like elmah.io, pingdom.com, or Azure's Availability Tests. I have tests that ping my website from all over the world and alert me if the site is down or unavailable.
例如,我使用了许多“运行状况检查”服务,例如elmah.io , pingdom.com或Azure的可用性测试。 我进行了测试,可以对来自世界各地的网站执行ping操作,并在该网站关闭或不可用时提醒我。
I've wanted to make my Health Endpoint Monitoring more formal. You likely have a service that does an occasional GET request to a page and looks at the HTML, or maybe just looks for an HTTP 200 Response. For the longest time most site availability tests are just basic pings. Recently folks have been formalizing their health checks.
我想使我的“健康端点监视”更加正式。 您可能有一项服务,该服务偶尔会向页面执行GET请求并查看HTML,或者只是寻找HTTP 200响应。 在最长的时间内,大多数站点可用性测试只是基本的ping操作。 最近人们一直在对他们的健康检查进行正规化。
You can make these tests more robust by actually having the health check endpoint check deeper and then return something meaningful. That could be as simple as "Healthy" or "Unhealthy" or it could be a whole JSON payload that tells you what's working and what's not. It's up to you!
通过使运行状况检查终结点实际检查更深,然后返回有意义的内容,可以使这些测试更可靠。 这可能很简单,例如“健康”或“不健康”,也可能是一个完整的JSON有效负载,告诉您什么在起作用,什么在不起作用。 由你决定!
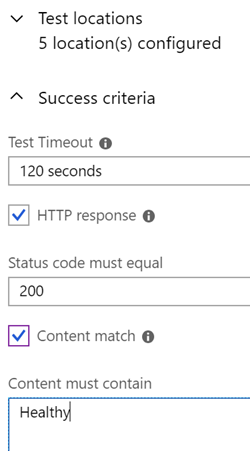
Is your database up? Maybe it's up but in read-only mode? Are your dependent services up? If one is down, can you recover? For example, I use some 3rd party back-end services that might be down. If one is down I could used cached data but my site is less than "Healthy," and I'd like to know. Is my disk full? Is my CPU hot? You get the idea.
您的数据库启动了吗? 也许启动了,但是处于只读模式? 您的依存服务是否正常? 如果发生故障,您可以恢复吗? 例如,我使用了一些可能关闭的第三方后端服务。 如果发生故障,我可以使用缓存的数据,但是我的站点小于“正常”,我想知道。 我的磁盘已满吗? 我的CPU很热吗? 你明白了。
You also need to distinguish between a "liveness" test and a "readiness" test. Liveness failures mean the site is down, dead, and needs fixing. Readiness tests mean it's there but perhaps isn't ready to serve traffic. Waking up, or busy, for example.
您还需要区分“活力”测试和“就绪”测试。 活动失败意味着该站点已关闭,死亡,需要修复。 准备测试意味着它已经存在,但可能还没有准备好为流量服务。 例如,醒来或忙碌。
If you just want your app to report it's liveness, just use the most basic ASP.NET Core 2.2 health check in your Startup.cs. It'll take you minutes to setup.
如果仅希望您的应用报告其活跃程度,则只需在Startup.cs中使用最基本的ASP.NET Core 2.2运行状况检查。 这将需要几分钟的时间进行设置。
// Startup.cs
public void ConfigureServices(IServiceCollection services)
{
services.AddHealthChecks(); // Registers health check services
}
public void Configure(IApplicationBuilder app)
{
app.UseHealthChecks("/healthcheck");
}
Now you can add a content check in your Azure or Pingdom, or tell Docker or Kubenetes if you're alive or not. Docker has a HEALTHCHECK directive for example:
现在,您可以在Azure或Pingdom中添加内容检查,或者告诉Docker或Kubenetes是否还活着。 Docker具有HEALTHCHECK指令,例如:
# Dockerfile
...
HEALTHCHECK CMD curl --fail http://localhost:5000/healthcheck || exit
If you're using Kubernetes you could hook up the Healthcheck to a K8s "readinessProbe" to help it make decisions about your app at scale.
如果您使用的是Kubernetes,则可以将Healthcheck连接到K8s的“ readinessProbe”,以帮助其对您的应用进行大规模决策。
Now, since determining "health" is up to you, you can go as deep as you'd like! The BeatPulse open source project has integrated with the ASP.NET Core Health Check API and set up a repository at https://github.com/Xabaril/AspNetCore.Diagnostics.HealthChecks that you should absolutely check out!
现在,由于确定“健康”取决于您,因此您可以根据自己的意愿进行深入研究! BeatPulse开源项目已经与ASP.NET Core Health Check API集成在一起,并在https://github.com/Xabaril/AspNetCore.Diagnostics.HealthChecks上建立了一个存储库,您应该绝对检查一下!
Using these add on methods you can check the health of everything - SQL Server, PostgreSQL, Redis, ElasticSearch, any URI, and on and on. Just add the package you need and then add the extension you want.
使用这些添加方法,您可以检查所有内容的运行状况-SQL Server,PostgreSQL,Redis,ElasticSearch,任何URI,等等。 只需添加所需的软件包,然后添加所需的扩展名即可。
You don't usually want your health checks to be heavy but as I said, you could take the results of the "HealthReport" list and dump it out as JSON. If this is too much code going on (anonymous types, all on one line, etc) then just break it up. Hat tip to Dejan.
通常,您不希望您的健康检查繁重,但是正如我所说,您可以获取“ HealthReport”列表的结果并将其作为JSON转储。 如果执行的代码太多(匿名类型,全部一行,等等),则将其分解。 向Dejan致意。
app.UseHealthChecks("/hc",
new HealthCheckOptions {
ResponseWriter = async (context, report) =>
{
var result = JsonConvert.SerializeObject(
new {
status = report.Status.ToString(),
errors = report.Entries.Select(e => new { key = e.Key, value = Enum.GetName(typeof(HealthStatus), e.Value.Status) })
});
context.Response.ContentType = MediaTypeNames.Application.Json;
await context.Response.WriteAsync(result);
}
});
At this point my endpoint doesn't just say "Healthy," it looks like this nice JSON response.
在这一点上,我的端点不仅说“健康”,而且看起来像一个不错的JSON响应。
{
status: "Healthy",
errors: [ ]
}
I could add a Url check for my back end API. If it's down (or in this case, unauthorized) I'll get this a nice explanation. I can decide if this means my site is unhealthy or degraded. I'm also pushing the results into Application Insights which I can then query on and make charts against.
我可以为我的后端API添加一个网址检查。 如果出现故障(或在这种情况下为未经授权),我将为您提供一个很好的解释。 我可以决定这是否意味着我的网站不健康或降级。 我还将结果推送到Application Insights中,然后可以查询并针对这些图表进行绘制。
services.AddHealthChecks()
.AddApplicationInsightsPublisher()
.AddUrlGroup(new Uri("https://api.simplecast.com/v1/podcasts.json"),"Simplecast API",HealthStatus.Degraded)
.AddUrlGroup(new Uri("https://rss.simplecast.com/podcasts/4669/rss"), "Simplecast RSS", HealthStatus.Degraded);
Here is the response, cool, eh?
这是回应,很酷,是吗?
{
status: "Degraded",
errors: [
{
key: "Simplecast API",
value: "Degraded"
},
{
key: "Simplecast RSS",
value: "Healthy"
}
]
}
This JSON is custom, but perhaps I could use the a built in writer for a free reasonable default and then hook up a free default UI?
此JSON是自定义的,但也许我可以使用内置的writer作为免费的合理默认值,然后连接免费的默认UI?
app.UseHealthChecks("/hc", new HealthCheckOptions()
{
Predicate = _ => true,
ResponseWriter = UIResponseWriter.WriteHealthCheckUIResponse
});
app.UseHealthChecksUI(setup => { setup.ApiPath = "/hc"; setup.UiPath = "/healthcheckui";);
Then I can hit /healthcheckui and it'll call the API endpoint and I get a nice little bootstrappy client-side front end for my health check. A mini dashboard if you will. I'll be using Application Insights and the API endpoint but it's nice to know this is also an option!
然后,我可以点击/ healthcheckui,它将调用API端点,并且我得到了一个不错的引导程序客户端前端,用于进行健康检查。 如果愿意,可以使用迷你仪表板。 我将使用Application Insights和API端点,但是很高兴知道这也是一个选择!
If I had a database I could check one or more of those for health well. The possibilities are endless and up to you.
如果我有数据库,则可以很好地检查其中一个或多个健康状况。 无限的可能性由您决定。
public void ConfigureServices(IServiceCollection services)
{
services.AddHealthChecks()
.AddSqlServer(
connectionString: Configuration["Data:ConnectionStrings:Sql"],
healthQuery: "SELECT 1;",
name: "sql",
failureStatus: HealthStatus.Degraded,
tags: new string[] { "db", "sql", "sqlserver" });
}
It's super flexible. You can even set up ASP.NET Core Health Checks to have a webhook that sends a Slack or Teams message that lets the team know the health of the site.
超级灵活。 您甚至可以设置ASP.NET Core运行状况检查,使其具有一个Webhook,该Webhook发送Slack或Teams消息,使团队知道网站的运行状况。
Check it out. It'll take less than an hour or so to set up the basics of ASP.NET Core 2.2 Health Checks.
看看这个。 设置ASP.NET Core 2.2运行状况检查的基础将花费不到一个小时左右的时间。
Sponsor: Preview the latest JetBrains Rider with its Assembly Explorer, Git Submodules, SQL language injections, integrated performance profiler and more advanced Unity support.
赞助商:预览最新的JetBrains Rider,包括其Assembly Explorer,Git子模块,SQL语言注入,集成的性能分析器以及更高级的Unity支持。