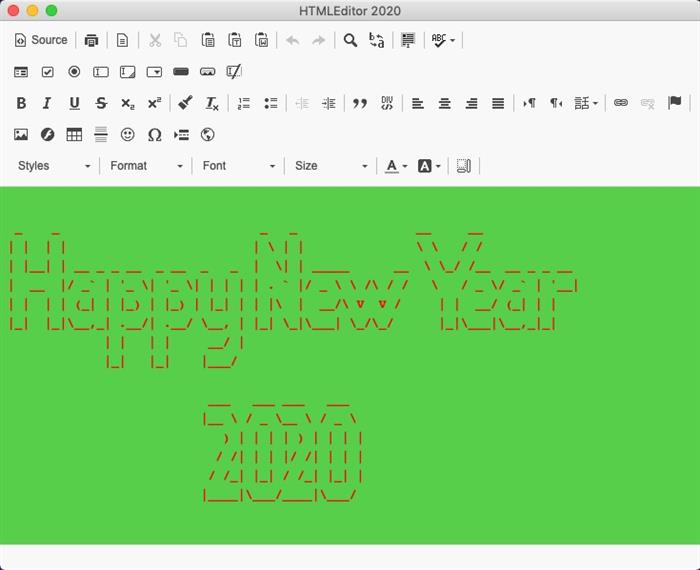
介绍 (Introduction)
With .NET Core 3, C# developers have now many possibilities to develop truly multiplatform GUI applications. You can find a study of these solutions in a recently released book:
借助.NET Core 3,C#开发人员现在可以开发出真正的多平台GUI应用程序。 您可以在最近发行的书中找到有关这些解决方案的研究:
Electron.NET is one of the solutions presented in this book, it provides all means to code a GUI application for Windows, Linux and Mac OS X based on the same .NET Core 3 project. Electron.NET is the .NET Core wrapper around the famous Electron framework (based on NodeJS).
Electron.NET是本书介绍的解决方案之一,它提供了所有基于同一.NET Core 3项目为Windows,Linux和Mac OS X编写GUI应用程序的方法。 Electron.NET是围绕着著名的Electron框架(基于NodeJS)的.NET Core包装器。
For illustrating the use of this tool, I will develop an HTML Editor application. This prototype program illustrates the Electron.NET’s capacity to use JavaScript component inside a cross platform desktop application. In our case, the CKeditor is used for the implementation of the editor itself.
为了说明该工具的用法,我将开发一个HTML编辑器应用程序。 该原型程序说明了Electron.NET在跨平台桌面应用程序中使用JavaScript组件的能力。 在我们的例子中, CKeditor用于实现编辑器本身。
An Electron.NET application is built from an ASP.NET MVC web application, and, therefore extended with the specifics features of a desktop application by using the Electron wrapper for .NET Core: Electron.NET.
Electron.NET应用程序是从ASP.NET MVC Web应用程序构建的,因此通过使用.NET Core的Electron包装器,扩展了桌面应用程序的特定功能:Electron.NET。
先决条件 (Prerequisites)
Before reviewing the development of the HTMLEditor
application with Electron.NET, you have to install the appropriate development environment.
在使用Electron.NET审查HTMLEditor
应用程序的开发之前,您必须安装适当的开发环境。
So you should have installed on your computer:
因此,您应该已经在计算机上安装了:
NodeJS
节点JS
NPM
NPM
Electron.NET global tool for dotnet CLI (see the GitHub website)
适用于dotnet CLI的Electron.NET全局工具(请参阅GitHub网站)
Check the GitHub web site of the project: https://github.com/ElectronNET/Electron.NET.
检查该项目的GitHub网站: https : //github.com/ElectronNET/Electron.NET 。
The HtmlEditor
application use the 5.30.1 version of Electron.NET.
HtmlEditor
应用程序使用5.30.1版本的Electron.NET。
Be careful, you have to double check that you are using the appropriate .NET Core version, the current version of Electron.NET is targeting the .NET Core 3.0 (if you use the 3.1 version of .NET Core, it will not work… not even install).
请注意,您必须仔细检查是否使用了适当的.NET Core版本,Electron.NET的当前版本是针对.NET Core 3.0的(如果使用3.1版的.NET Core,它将无法正常工作……甚至没有安装)。
应用程式编码 (Application Coding)
The development of the application can be described as a simple process.
应用程序的开发可以描述为一个简单的过程。
1.创建ASP.NET MVC应用程序 (1. Creation of the ASP.NET MVC Application)
First, you have to create a standard .NET Core 3 ASP.NET MVC application, with the following command for example, but you can use Visual Studio.
首先,您必须使用以下命令创建一个标准的.NET Core 3 ASP.NET MVC应用程序,但是您可以使用Visual Studio。
dotnet new mvc -o Multiplatform.HtmlEditor
2.将ElectronNET.API NuGet程序包添加到项目中 (2. Add ElectronNET.API NuGet Packages to the Project)
For adding the NuGet dependencies, you can use the familar "NuGet package Manager
" of Visual Studio:
要添加NuGet依赖项,可以使用Visual Studio的熟悉的“ NuGet package Manager
”:
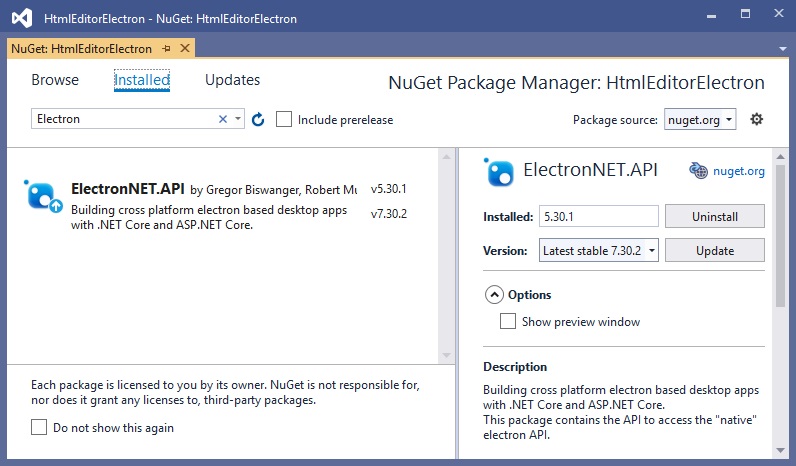
You have to install the CLI tool as well. For adding the required dotnet CLI Electron.NET global tool:
您还必须安装CLI工具。 要添加所需的dotnet CLI Electron.NET全局工具:
dotnet tool install ElectronNET.CLI -g --version 5.30.1
3.在应用程序中实例化ElectronNET.API (3. Instantiate ElectronNET.API in the Application)
This is done by adding an instance of the Electron wrapper in the MVC app. Actually, in the startup.cs file:
这是通过在MVC应用程序中添加Electron包装器的实例来完成的。 实际上,在startup.cs文件中:
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
if (env.IsDevelopment()) {
app.UseDeveloperExceptionPage();
} else {
app.UseExceptionHandler("/Home/Error");
}
app.UseStaticFiles();
app.UseRouting();
app.UseEndpoints(endpoints => {
endpoints.MapControllerRoute(
name: "default",
pattern: "{controller=Home}/{action=Index}/{id?}");
});
// Open the Electron-Window here
//Task.Run(async () => await Electron.WindowManager.CreateWindowAsync());
if (HybridSupport.IsElectronActive) {
ElectronBootstrap();
}
}
public async void ElectronBootstrap()
{
var browserWindow = await Electron.WindowManager.CreateWindowAsync
(new BrowserWindowOptions {
Width = 1000,
Height = 800,
Show = false,
});
browserWindow.OnReadyToShow += () => browserWindow.Show();
browserWindow.SetTitle("HTMLEditor 2020");
}
The application is running now in a desktop container, but does not integrate any features:
该应用程序现在在桌面容器中运行,但未集成任何功能:
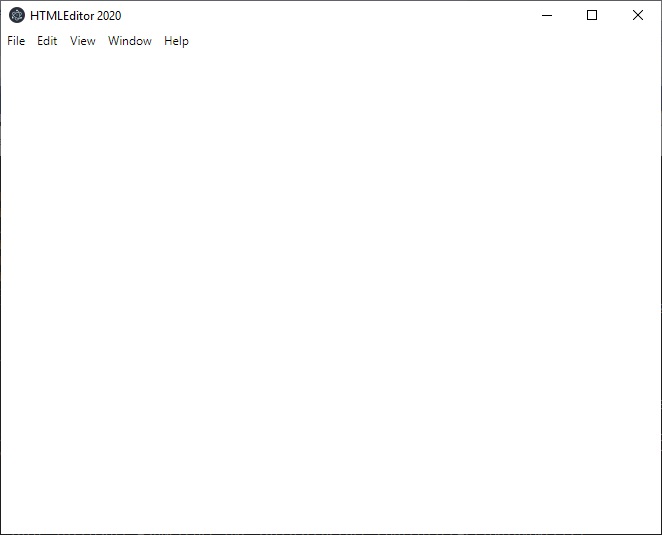
Besides adding Electron in the application, the default ASP.NET MVC application has been cleaned from the unnecessary code (pages, logging, config …).
除了在应用程序中添加Electron之外,还从不必要的代码(页面,日志记录,配置…)中清除了默认的ASP.NET MVC应用程序。
4.将自定义菜单添加到应用程序 (4. Add Custom Menu to the Application)
Once the application is running as desktop app, you have to modify the default native menu. For doing that, the “native” menu of the application is implemented with a dedicated MVC Controller: MenusController.cs.
当应用程序作为桌面应用程序运行后,您必须修改默认的本机菜单。 为此,应用程序的“本机”菜单是通过专用的MVC Controller: MenusController.cs实现的 。
>
public class MenusController : Controller
{
public IActionResult Index()
{
if (HybridSupport.IsElectronActive) {
var menu = new MenuItem[] {
new MenuItem {
Label = "File", Submenu = new MenuItem[] {
new MenuItem {
Label = "Open HTML",
Accelerator = "CmdOrCtrl+O",
Click = async () =>
{
// Open file HTML
var mainWindow = Electron.WindowManager.BrowserWindows.First();
var options = new OpenDialogOptions
{
Title = "Open HTML file",
Filters = new FileFilter[]
{
new FileFilter { Name = "HTML",
Extensions = new string[] {"html","htm" } }
}
};
var result = await Electron.Dialog.ShowOpenDialogAsync
(mainWindow, options);
if (result.First() != "")
{
string OpenfilePath = result.First();
string strContent = FileOperation.openRead(OpenfilePath);
//Call Render JS
var mainWindow1 =
Electron.WindowManager.BrowserWindows.First();
Electron.IpcMain.Send
(mainWindow1,"setContent",strContent);
mainWindow.SetTitle(OpenfilePath);
}
}
},
new MenuItem { Label = "Save HTML",
Accelerator = "CmdOrCtrl+S",
Click = async () =>
{
var mainWindow = Electron.WindowManager.BrowserWindows.First();
Electron.IpcMain.Send(mainWindow,"saveContent");
}
},
new MenuItem { Type = MenuType.separator },
new MenuItem {
Label = "Exit",
Accelerator = "CmdOrCtrl+X",
Click = () =>
{
// Exit app
Electron.App.Exit();
}
},
}
},
new MenuItem {
Label = "Help", Submenu = new MenuItem[] {
new MenuItem
{
Label = "About",
Accelerator = "CmdOrCtrl+R",
Click = async () =>
{
// open native message windows
var options = new MessageBoxOptions
("This is a demo application for Electron.NEt
and .NET CORE 3.");
options.Type = MessageBoxType.info;
options.Title = "About HTMLEditor";
await Electron.Dialog.ShowMessageBoxAsync(options);
}
}
}
}
};
Electron.Menu.SetApplicationMenu(menu);
}
return View();
}
}
Notice that the menu controller can be used for calling (sync or async) the several features of the application.
请注意,菜单控制器可用于调用(同步或异步)应用程序的多个功能。
5.在您的应用程序中添加JavaScript HTML编辑器 (5. Add the JavaScript HTML Editor in Your Application)
The HTML editor itself is a freely available JavaScript component: CKeditor4
. The web site allows you to personalize the menu of the editor by generating a config.js file. This file is directly downloadable from the ckeditor4 web site: https://github.com/ckeditor/ckeditor4.
HTML编辑器本身是一个免费JavaScript组件: CKeditor4
。 该网站允许您通过生成config.js文件来个性化编辑器的菜单。 该文件可直接从ckeditor4网站下载: https : //github.com/ckeditor/ckeditor4 。
A dedicated web site allows you to configure the editor with a dedicated graphical interface. You can find this app online at https://ckeditor.com/cke4/builder.
专用网站允许您使用专用图形界面配置编辑器。 您可以在https://ckeditor.com/cke4/builder上在线找到该应用程序。
Once you have created the appropriate configuration file, you have to add it to your MVC project (on the client side).
创建适当的配置文件后,必须将其添加到MVC项目中(在客户端)。
The required components (js, css, img) for the implementation of the editor have to be added to the project. Here is the files arborescence of the client dependencies for adding the ckeditor4
HTML editor to your project.
实现编辑器所需的组件(js,css,img)必须添加到项目中。 这是用于将ckeditor4
HTML编辑器添加到您的项目的客户端依赖项的文件树状结构。
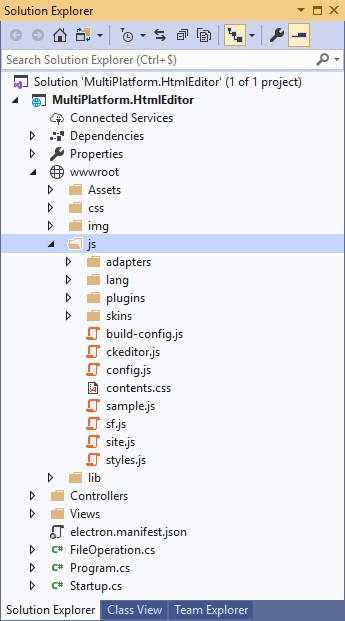
6.在主线程和渲染线程之间建立通信 (6. Establishing Communication Between the Main Thread and the Render Thread)
There are two functions which require communication between the C# (main process) and the Html/Js (Render
process).
有两个功能需要C#(主进程)和Html / Js( Render
进程)之间进行通信。
Open File
打开文件
Save File
保存存档
Here is the schema of the communication between the two-processes needed for the implementation of these two features:
这是实现这两个功能所需的两个过程之间的通信模式:
Open File
![]() |
Save File |
打开文件
![]() |
保存存档 |
7.使用文件的操作对象 (7. Use the File’s Operations Object)
The editor integrates the basic file’s operations: Open, Save. A dedicated class is implemented for these operations: FileOperations.cs. This static
object integrates the two matching methods required for the example.
编辑器集成了基本文件的操作:打开,保存。 为这些操作实现了一个专用类: FileOperations.cs 。 该static
对象集成了示例所需的两种匹配方法。
Once each of these steps has been done successfully (and tested), the application is finished, well almost, you have to build the project and it could be a little bit tricky regarding the type of executable you want to produce.
一旦成功完成了每个步骤(并测试了这些步骤),应用程序就完成了,几乎,您必须构建项目,并且对于要生成的可执行文件的类型可能有些棘手。
8.生成.NET Core 3 / Electron.NET应用程序 (8. Build the .NET Core 3 / Electron.NET Application)
For the first release of this article, the most obvious (actually the basic) executable deploiement type is exposed.
对于本文的第一个版本,公开了最明显的(实际上是基本的)可执行文件部署类型。
This part is very well documented on the ElectronNET GitHub web site.
这部分在ElectronNET GitHub网站上有很好的记录。
First, you have to create the Electron configuration file for the ASP.NET MVC application with the command:
首先,您必须使用以下命令为ASP.NET MVC应用程序创建Electron配置文件:
electronize init
Thereafter, you can execute the application with the command:
之后,您可以使用以下命令执行应用程序:
electronize start
This command will do the build, and the restore for the project, it could take a little time the first time it is executed (the Electron wrapper has to be loaded).
此命令将完成构建以及项目的还原,第一次执行可能会花费一些时间(必须加载Electron包装器)。
目前的问题 (Current Issues)
- I have not managed to update the icon of the application (working on it) 我尚未设法更新应用程序的图标(正在使用该图标)
The menu display “Electron” and not “File” in the menu bar on Mac OS X.
在Mac OS X的菜单栏中,菜单显示“ Electron ”而不是“ File ”。
结论 (Conclusion)
The usage of Electron.NET appears to be a solution of choice for the ASP.NET developers who want to develop multiplatform desktop application (for Windows, Linux and MacOS X). Electron.NET makes it possible.
对于想要开发多平台桌面应用程序(适用于Windows,Linux和MacOS X)的ASP.NET开发人员,Electron.NET的使用似乎是一种选择的解决方案。 Electron.NET使之成为可能。
With the encapsulation of a web application into a desktop application, many JavaScript components can be reused or recycled for a desktop development and that is a strong point of this way of doing. There are plenty of JavaScript components that can be a great addition to a desktop application (for the navigation, GUI effects …).
通过将Web应用程序封装到桌面应用程序中,可以将许多JavaScript组件重复使用或回收用于桌面开发,这是这种方法的强项。 有很多JavaScript组件可以很好地添加到桌面应用程序中(用于导航,GUI效果……)。
Nevertheless, the use of Electron.NET is not optimized for the Windows platform, and many methods of the original project are not available in the .NET Core wrapper. Besides, Elentron.NET requires you to master the JavaScript programing more than actually ASP.NET C# knowledge.
尽管如此,Electron.NET的使用并未针对Windows平台进行优化,并且.NET Core包装器中没有原始项目的许多方法。 此外,Elentron.NET要求您比实际的ASP.NET C#知识更精通JavaScript编程。
But Electron.NET is a great wrapper that can be used if you want to produce desktop application from your web site development.
但是Electron.NET是一个很好的包装器,如果您想从网站开发中生成桌面应用程序,可以使用它。
If Electron.NET does not match your expectations, there are many other solutions for coding a multi-platform GUI desktop application with C# .NET Core 3, check the book which inspired this article here.
如果Electron.NET不符合您的期望,那么还有许多其他解决方案可以使用C#.NET Core 3编码多平台GUI桌面应用程序,请在此处查阅启发本文的书。
历史 (History)
6th January, 2020: First version
2020年1月6 日 :第一版
翻译自: https://www.codeproject.com/Articles/5255569/Multiplatform-Desktop-HTML-Editor-with-NET-Core-3