angular svg
介绍 (Introduction)
We've all followed Angular tutorials, and the end result is usually a neatly-formatted "List component" screen showing a list of data, perhaps with a "Details component" to see further details about a chosen object. But wouldn't it be cool if we could get Angular to create beautiful, responsive diagrams instead? That's what we're going to create here.
我们都遵循了Angular教程,最终结果通常是格式整齐的“列表组件”屏幕,其中显示了数据列表,也许还带有“详细信息组件”以查看有关所选对象的更多详细信息。 但是,如果我们可以让Angular来创建漂亮的,响应式的图表,那不是很酷吗? 这就是我们要在这里创建的。
Our end result will look like this:
我们的最终结果将如下所示:
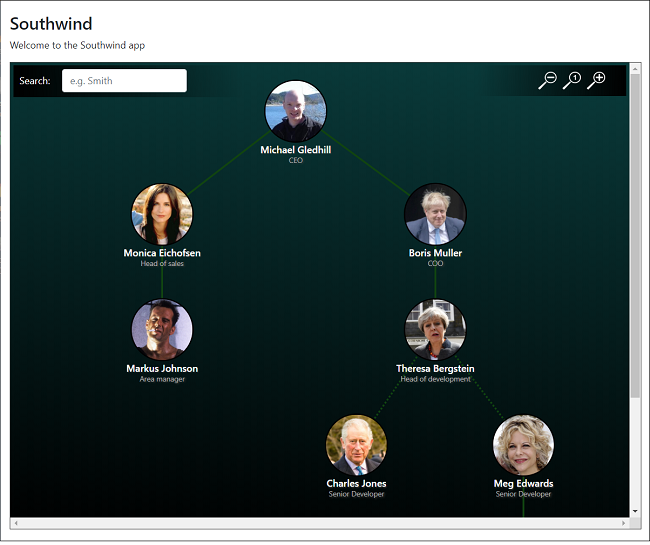
Now that looks cool!
现在看起来很酷!
Obviously, this looks great, but won't be suitable for all scenarios or types of data, but hopefully this article will teach you some useful techniques, and show you what is possible with Angular and SVG, without too much coding.
显然,这看起来不错,但并不适合所有场景或数据类型,但希望本文能教给您一些有用的技术,并向您展示Angular和SVG无需太多编码即可实现的工作。
We will read in some JSON data, and use Angular to bind to SVG elements to create a diagram using SVG. <svg>
elements have a big advantage over <canvas>
elements as they're much less memory hungry, and, because they are vector graphics, you can zoom in on them without losing image quality.
我们将读取一些JSON数据,并使用Angular绑定到SVG元素以使用SVG创建图。 <svg>
元素比<canvas>
<svg>
元素具有很大的优势,因为它们的内存消耗少得多,并且由于它们是矢量图形,因此可以放大它们而不损失图像质量。
You can see a "live" version of this website by clicking here. You'll see that, in this version, I've taken it further, allowing you to drag the SVG control around, and zoom in & out, either using the zoom icons, or your mouse wheel. You can also click on an employee to bring up their details.
通过单击此处,您可以查看此网站的“实时”版本。 您会看到,在此版本中,我将其进行了进一步的介绍,允许您使用缩放图标或鼠标滚轮来拖动SVG控件,以及放大和缩小。 您也可以单击员工以显示其详细信息。
范围 (Scope)
To write this application, you will need:
要编写此应用程序,您将需要:
- a copy of Visual Studio code Visual Studio代码的副本
- TypeScript, GIT, npm, and the Angular CLI installed 安装了TypeScript,GIT,npm和Angular CLI
the resources in the assets.zip file, which you can download at the top of this article
可以在本文顶部下载的assets.zip文件中的资源
- basic knowledge of Angular development Angular开发的基础知识
My original mock-up for this project also included a Web API project to read the data directly from SQL Server. I have replaced this with a hardcoded set of JSON data to keep this article more concise, but if you are interested in knowing how to create a Web API using ASP.NET Core 2, you can read my other Code Project article here.
我对该项目的原始模型还包括一个Web API项目,可直接从SQL Server读取数据。 为了使本文更加简洁,我将其替换为一组硬编码的JSON数据,但是如果您想了解如何使用ASP.NET Core 2创建Web API,可以在这里阅读我的其他Code Project文章。
You'll also notice that I'm cheating a little bit: each of the Employee
records already has an x and y coordinate. We're not going to write code to take a set of hierarchical data, and work out where to place it on a new diagram. Everyone's data will look different, and this wouldn't add much value to the article.
您还会注意到我在作弊:每个Employee
记录已经具有x和y坐标。 我们不会编写代码来获取一组分层数据,也不会确定将其放置在新图上的位置。 每个人的数据看起来都将有所不同,这不会为文章增加太多价值。
This article is more to give you creative ideas which you can use in your own projects, rather than being a step-by-step introduction to Angular. And there seem to be very few examples showing how to use Angular and SVG together. I hope you find this interesting article useful, please remember to leave a comment if you do!
本文的主要目的是为您提供可以在自己的项目中使用的创意,而不是逐步介绍Angular。 似乎很少有示例显示如何一起使用Angular和SVG。 希望这篇有趣的文章对您有所帮助,请记住发表评论!
让我们创建我们的应用程序! (Let's Create Our App!)
1.创建Angular Barebones应用程序 (1. Create the Angular Barebones App)
Okay, on my Windows machine, I have opened up a Command Prompt, and go into my c:\repos directory. To create the Angular app, I will now run:
好的,在Windows计算机上,我打开了一个命令提示符,并进入我的c:\ repos目录。 要创建Angular应用,我现在将运行:
ng new Southwind --routing=true --style=scss
A few minutes later, I have a c:\repos\Southwind folder, containing 31,000 files. Okay, let's go to that folder, and start Visual Studio Code:
几分钟后,我有了一个c:\ repos \ Southwind文件夹,其中包含31,000个文件。 好的,我们转到该文件夹,然后启动Visual Studio Code:
cd Southwind
code .
Now, by default, all Angular apps will run on port 4200. I like to change this, with each new project, just to make sure there's no clashes. To do this, let's open up package.json in Visual Studio Code, open the "package.json" file, and change the "start
" script to specify a different port number:
现在,默认情况下,所有Angular应用程序都将在端口4200上运行。对于每个新项目,我都希望对此进行更改,以确保没有冲突。 要做到这一点,在Visual Studio代码让我们打开了的package.json,打开“ 的package.json”文件,并修改“ start
”脚本以指定不同的端口号:
"scripts": {
"ng": "ng",
"start": "ng serve --port 4401",
If we were to now go back to our Command Prompt, we would be able to run our new Angular project:
如果现在返回到命令提示符,则可以运行新的Angular项目:
npm start
...and we could open http://localhost:4401
in a browser to see the default Angular webpage.
...我们可以在浏览器中打开http://localhost:4401
来查看默认的Angular网页。
2.将Bootstrap添加到Angular应用 (2. Add Bootstrap to the Angular App)
Next up, let's add Bootstrap. Back in the Command Prompt, run the following command:
接下来,让我们添加Bootstrap。 返回命令提示符,运行以下命令:
npm install ngx-bootstrap bootstrap --save
In Visual Studio code, open the file src\styles.scss and add the following line:
在Visual Studio代码中,打开文件src \ styles.scss并添加以下行:
@import '../node_modules/bootstrap/dist/css/bootstrap.min.css';
Okay, let's quickly check that it worked okay. In the src\index.html file, I'm going to wrap the <body>
tag in a Bootstrap-friendly container class:
好的,让我们快速检查它是否正常。 在src \ index.html文件中,我将<body>
标记包装在对Bootstrap友好的容器类中:
<body>
<div class="container-fluid">
<h3>Southwind</h3>
<app-root></app-root>
</div>
</body>
Next, in the src\app\app.component.html, let's delete all the code, and replace it with this:
接下来,在src \ app \ app.component.html中 ,让我们删除所有代码,并将其替换为:
<p>Welcome to the Southwind app</p>
<div class="row">
<div class="col-md-3">1st column</div>
<div class="col-md-3">2nd column</div>
<div class="col-md-3">3rd column</div>
<div class="col-md-3">4th column</div>
</div>
If you were to now run the app, you'd see a fairly dull screen, showing four columns. When you resize the browser window to a smaller width, the columns will stack one on top of another.
如果现在要运行该应用程序,则会看到一个相当乏味的屏幕,其中显示四列。 当您将浏览器窗口调整为较小的宽度时,这些列将一个堆叠在另一个之上。
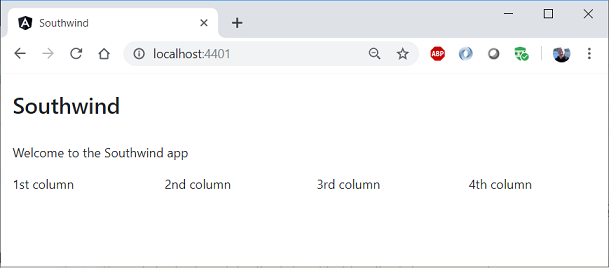
Yeah, it's dumb... we're meant to be learning SVG here (!), but honestly, Angular has been changing so rapidly and dramatically over the past few years, I always like to create apps step-by-step and test them along the way, to make sure nothing's quietly been changed.
是的,这很愚蠢...我们本来打算在这里学习SVG(!),但是老实说,Angular在过去几年中变化如此之快,而且非常引人注目,我一直喜欢逐步开发和测试应用程序一路走来,以确保没有任何更改。
3.将资源添加到我们的应用程序 (3. Add the Resources to Our App)
Okay, assuming this is all working fine, let's add some resources to the app. Our little app will contain some employees data, and photos for each of them. I've also included some icons that I've used in the "full version" of this app, which you can find online.
好的,假设一切正常,让我们向应用程序添加一些资源。 我们的小应用程序将包含一些员工数据以及每个员工的照片。 我还提供了一些我在此应用程序“完整版”中使用过的图标,您可以在网上找到它们。
At the top of this tutorial, you'll find an assets.zip file. Go ahead and unzip this into your src\assets folder. You should then see these 3 folders and their files, in Visual Studio code:
在本教程的顶部,您将找到assets.zip文件。 继续并将其解压缩到您的src \ assets文件夹中。 然后,您应该在Visual Studio代码中看到这3个文件夹及其文件:
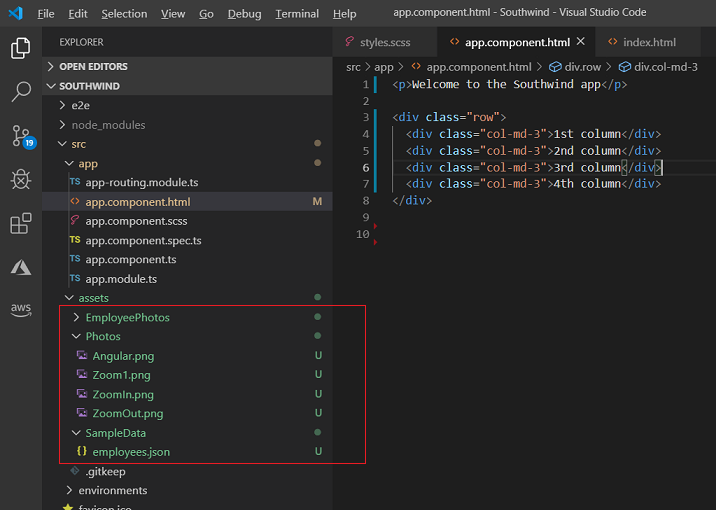
Okay, let's load some data!
好吧,让我们加载一些数据!
4.加载JSON数据 (4. Loading JSON Data)
In the assets\SampleData folder, you'll see a new employees.json file. This contains the list of employee
records, and all a list of relationship
records - which employees are the managers or sub-managers of other employees? The structure looks like this:
在assets \ Sampl