关于帐户工具包
什么是无密码验证?
无密码身份验证会跳过使用密码进行注册或登录的过程。 而是使用发送到用户电话的一次性SMS验证码或指向其电子邮件地址的一次性链接来确认用户身份验证。
为什么要使用无密码身份验证?
- 用户的无缝登录和注册过程。
- 确保安全访问您的应用程序,因为用户将不会重复使用密码或使用容易猜到的密码(例如“密码”)。
- 避免使用户负担创建和记住唯一密码的麻烦
在此快速提示教程中,我将向您展示如何使用Facebook的Account Kit在Android应用中进行无密码身份验证。 您的用户将使用他们的电话号码或电子邮件地址进行注册和登录。 用户甚至不需要拥有Facebook帐户即可通过身份验证。
不仅如此,Account Kit还易于实施,使您不必繁琐地构建登录系统。
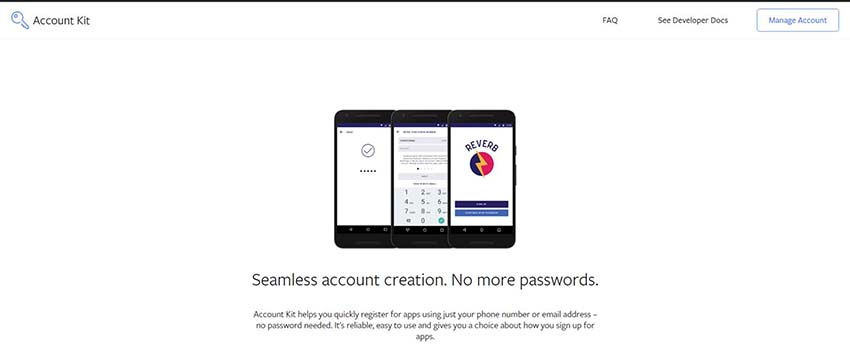
帐户工具包如何工作?
下图应阐明帐户工具包的工作方式。
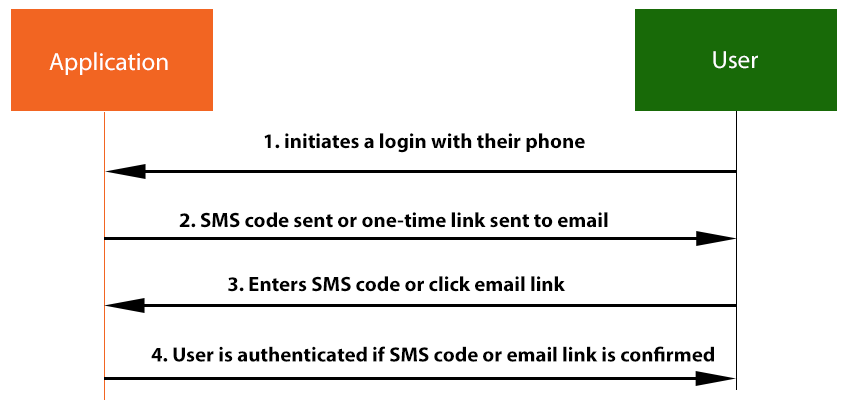
身份验证发生在用户和应用之间的一系列交换中。 首先,用户可以通过在手机上加载应用程序来启动登录。 然后,将验证码发送到用户的SMS,或通过电子邮件发送一次性链接。 之后,如果用户输入验证码或单击链接,则将对应用进行身份验证。
Facebook Account Kit的另一个很酷的功能是,当您的用户在应用程序中输入他或她的电话号码时,Account Kit将尝试使其与连接到用户的Facebook个人资料的电话号码匹配。 如果用户登录到Android Facebook应用程序,并且电话号码匹配,则“帐户工具包”将跳过发送SMS验证码的操作,从而使用户无缝登录。
使用帐户工具包
1.先决条件
要开始使用帐户工具包,您需要:
- 一个Facebook开发者帐户
- 与Account Kit 集成的应用程序
2.启用帐户工具包
转到您的应用程序信息中心,点击添加产品按钮,然后选择帐户 工具包 。 然后单击“ 入门”按钮以添加帐户工具包。 您将看到的是Account Kit的设置配置。
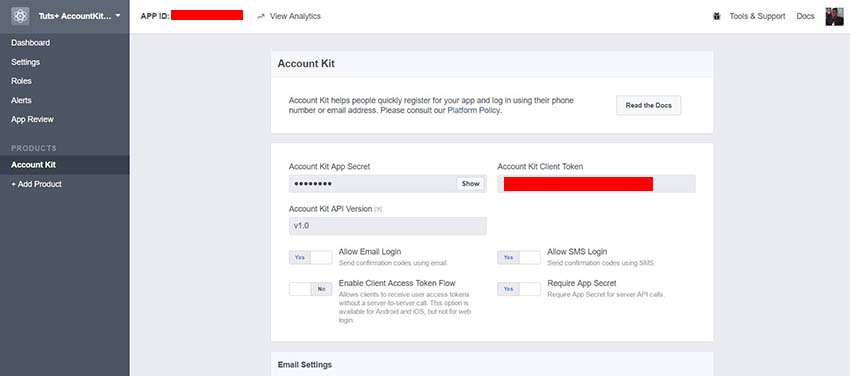
3.声明依赖
在build.gradle文件中将依赖项与最新版本的Account Kit SDK添加在一起,并同步您的项目。
repositories {
jcenter()
}
dependencies {
compile 'com.facebook.android:account-kit-sdk:4.+'
}
更新AndroidManifest.xml
添加您的Facebook应用ID,帐户工具包 客户令牌(可在“帐户工具包”设置信息中心中找到)和INTERNET
AndroidManifest的权限。 xml 。
<uses-permission android:name="android.permission.INTERNET" />
<meta-data android:name="com.facebook.accountkit.ApplicationName"
android:value="@string/app_name" />
<meta-data android:name="com.facebook.sdk.ApplicationId"
android:value="@string/FACEBOOK_APP_ID" />
<meta-data android:name="com.facebook.accountkit.ClientToken"
android:value="@string/ACCOUNT_KIT_CLIENT_TOKEN" />
<activity
android:name="com.facebook.accountkit.ui.AccountKitActivity"
android:theme="@style/AppLoginTheme"
tools:replace="android:theme"/>
更新资源文件
在您的strings.xml文件中包含您的应用ID和Account Kit客户令牌。
<string name="FACEBOOK_APP_ID">YourAPPId</string>
<string name="ACCOUNT_KIT_CLIENT_TOKEN">YourAccountKitClientToken</string>
还包括您样式中的Account Kit主题。 xml 。
<style name="AppLoginTheme" parent="Theme.AccountKit" />
6.初始化SDK
在您的Application类中,初始化SDK(请记住在AndroidManifest.xml中包含android:name
)。
public class MyApplication extends Application {
@Override
public void onCreate() {
super.onCreate();
AccountKit.initialize(getApplicationContext());
}
}
7.启动登录流程
我们必须为SMS和电子邮件登录身份验证流程编写一个单独的处理程序。
对于SMS,在第5行,我们指定登录类型LoginType.PHONE
。
public void onSMSLoginFlow(View view) {
final Intent intent = new Intent(this, AccountKitActivity.class);
AccountKitConfiguration.AccountKitConfigurationBuilder configurationBuilder =
new AccountKitConfiguration.AccountKitConfigurationBuilder(
LoginType.PHONE,
AccountKitActivity.ResponseType.CODE); // or .ResponseType.TOKEN
// ... perform additional configuration ...
intent.putExtra(
AccountKitActivity.ACCOUNT_KIT_ACTIVITY_CONFIGURATION,
configurationBuilder.build());
startActivityForResult(intent, 101);
}
对于电子邮件,在第5行,我们指定登录类型LoginType.EMAIL
。
public void onEmailLoginFlow(View view) {
final Intent intent = new Intent(this, AccountKitActivity.class);
AccountKitConfiguration.AccountKitConfigurationBuilder configurationBuilder =
new AccountKitConfiguration.AccountKitConfigurationBuilder(
LoginType.EMAIL,
AccountKitActivity.ResponseType.CODE); // or .ResponseType.TOKEN
// ... perform additional configuration ...
intent.putExtra(
AccountKitActivity.ACCOUNT_KIT_ACTIVITY_CONFIGURATION,
configurationBuilder.build());
startActivityForResult(intent, 101);
}
8.布置登录屏幕
这是一个简单的屏幕布局,其中显示了用于通过SMS或电子邮件登录的按钮。
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout
xmlns:android="https://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/activity_main"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
android:paddingBottom="@dimen/activity_vertical_margin"
android:orientation="vertical"
tools:context="com.chikeandroid.tutsplus_facebook_accountkit.MainActivity">
<Button
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Login By SMS"
android:onClick="onSMSLoginFlow"/>
<Button
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Login By Email"
android:onClick="onEmailLoginFlow"/>
</LinearLayout>
9.处理来自登录流程的响应
现在,当用户尝试登录时,我们将在onActivityResult()
方法中获得响应。 在这种方法中,我们可以处理成功,取消和失败的身份验证。
@Override
protected void onActivityResult(final int requestCode, final int resultCode, final Intent data) {
super.onActivityResult(requestCode, resultCode, data);
if (requestCode == 101) { // confirm that this response matches your request
AccountKitLoginResult loginResult = data.getParcelableExtra(AccountKitLoginResult.RESULT_KEY);
String toastMessage;
if (loginResult.getError() != null) {
toastMessage = loginResult.getError().getErrorType().getMessage();
showErrorActivity(loginResult.getError());
} else if (loginResult.wasCancelled()) {
toastMessage = "Login Cancelled";
} else {
if (loginResult.getAccessToken() != null) {
toastMessage = "Success:" + loginResult.getAccessToken().getAccountId();
} else {
toastMessage = String.format(
"Success:%s...",
loginResult.getAuthorizationCode().substring(0, 10));
}
// If you have an authorization code, retrieve it from
// loginResult.getAuthorizationCode()
// and pass it to your server and exchange it for an access token.
// Success! Start your next activity...
goToMyLoggedInActivity();
}
// Surface the result to your user in an appropriate way.
Toast.makeText(this, toastMessage, Toast.LENGTH_LONG).show();
}
}
完成的应用程序
现在,我们可以运行我们的应用程序以测试SMS和电子邮件登录流程!
请注意,Account Kit JavaScript SDK不支持WebView登录,因此您不能从带有Account Kit的WebView中登录。 您必须使用本机代码编写Account Kit登录界面。
结论
在本快速提示教程中,您了解了使用Facebook Account Kit进行无密码身份验证的含义,含义,为什么要考虑使用它以及如何在Android应用程序中实现它。
不过要提一个警告:有些人认为无密码身份验证的安全性较低。 当安全性成为优先事项时,例如银行应用程序,大多数人不会使用它。 因此,对于何时使用它以及何时使用更传统的身份验证方案,应谨慎考虑。