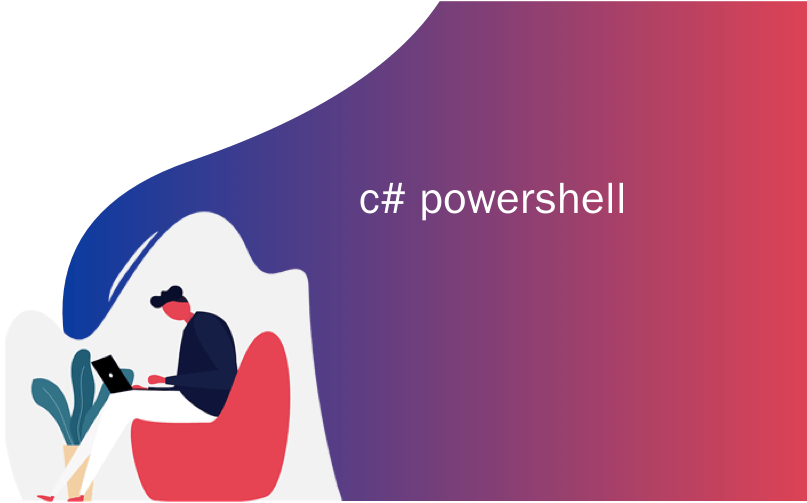
c# powershell
Variables are an important part of the programming languages. Variables are used to store information for basic types like name, age, label, address, count, etc. Variables are named accordingly related to the information or data we want to save.
变量是编程语言的重要组成部分。 变量用于存储基本类型的信息,例如名称,年龄,标签,地址,计数等。变量的命名与我们要保存的信息或数据相关。
变量类型 (Variable Types)
As there are different data types we generally use variables for specific types. Different programming languages provide very similar data types. Here list of the variable types.
由于存在不同的数据类型,因此我们通常将变量用于特定类型。 不同的编程语言提供了非常相似的数据类型。 这里列出了变量类型。
- Integer Variable Type 整数变量类型
- Character Variable Type字符变量类型
- String Variable Type字符串变量类型
- Boolean Variable Type布尔变量类型
- Float Variable Type浮动变量类型
- Double Variable Type双变量类型
整数变量类型(Integer Variable Type)
Integer variable type is used to store numeric types which can be used for calculations. We generally use decimal numbers like 5, 12124, -342 as integer variable types. If we do not use 5 for calculation we can store it as a different type named string variable type. Here are some examples of integer variable type.
整数变量类型用于存储可用于计算的数字类型。 我们通常使用5、12124,-342等十进制数字作为整数变量类型。 如果我们不使用5进行计算,则可以将其存储为名为字符串变量类型的其他类型。 以下是整数变量类型的一些示例。
12
-24234
1
0
13241235123512
-5
字符变量类型 (Character Variable Type)
The character type is very similar to the String variable type where the String variable consists of single or multiple character variables. Some programming languages provide character variable type as string variable type.a
, 5
,z
are stored as character variable type. Here are some examples of character variable type.
字符类型与String变量类型非常相似,其中String变量由单个或多个字符变量组成。 一些编程语言提供字符变量类型作为字符串变量类型。 a
, 5
, z
被存储为字符变量类型。 以下是字符变量类型的一些示例。
"a"
"<"
"b"
"5"
"0"
"?"
字符串变量类型 (String Variable Type)
A string variable is used to store text data or values. A string can consist of single or multiple characters. String variable types are used like name, address, comments, etc.
字符串变量用于存储文本数据或值。 字符串可以包含单个或多个字符。 使用字符串变量类型,例如名称,地址,注释等。
"poftut.com"
"2234234"
"abc"
"Ahmet Ali Baydan"
布尔变量类型 (Boolean Variable Type)
Programming languages also provides logic operations and logic data types True
and False
. Logic variables are named as boolean
variables. and
1
can be also used for boolean values.
编程语言还提供逻辑运算和逻辑数据类型True
和False
。 逻辑变量被称为boolean
变量。 和
1
也可以用于布尔值。
True
False
1
0
浮动变量类型 (Float Variable Type)
Float variables are used to store non-decimal numeric values. We can use more precise values with a float data type. Floating types generally consumes more memory or ram.
浮点变量用于存储非十进制数字值。 我们可以对浮点数据类型使用更精确的值。 浮动类型通常会占用更多内存或内存。
1.2342352
0.12124
42342.1
-12124.433
双变量类型 (Double Variable Type)
Float variable type store some floating point numbers. If we need more details and precision we should use double variable types.
浮点变量类型存储一些浮点数。 如果需要更多细节和精度,则应使用双变量类型。
123.23213453242345
-0.24123512351345345345
通用变量定义语法 (Generic Variable Definition Syntax)
Where different programming languages provide different variable definition syntax. We can provide some generic syntax about variable definition in different programming languages.
不同的编程语言提供不同的变量定义语法。 我们可以提供不同编程语言中有关变量定义的一些通用语法。
TYPE VARIABLE_NAME=VARIABLE_DATA
- TYPE is the variable type TYPE是变量类型
- VARIABLE_NAME is the name or label of the variableVARIABLE_NAME是变量的名称或标签
- VARIABLE_DATA is the data we want to store in the variableVARIABLE_DATA是我们要存储在变量中的数据
在PHP中定义和使用变量(Define and Use Variables In PHP)
在PHP中定义变量(Define Variable In PHP)
We can define a PHP variable just prefixing the variable named with the $
sign. We can also set the variable value with the =
sign.
我们可以定义一个PHP变量,只是在变量前面加上$
符号。 我们还可以使用=
符号设置变量值。
//Integer Variable Type
$age=25;
//String Variable Type
$name="Ahmet";
//Float Variable Type
$rate=12.4;
在PHP中使用变量 (Use Variable In PHP)
We can use these different variables in PHP like below. In this example, we will print the variable values to the standard output or screen.
我们可以在PHP中使用这些不同的变量,如下所示。 在此示例中,我们将变量值打印到标准输出或屏幕上。
//Integer Variable Type
echo $age;
//String Variable Type
echo $name;
//Float Variable Type
echo $rate;
在Python中定义和使用变量 (Define and Use Variables In Python)
We can define Python variables easily without using any special sign or type keyword.
我们可以轻松定义Python变量,而无需使用任何特殊的sign或type关键字。
在Python中定义变量 (Define Variable In Python)
We can define Python variables without a special sign for the variable name. We will also use =
to assign variable value.
我们可以定义Python变量,而变量名称不带特殊符号。 我们还将使用=
分配变量值。
//Integer Variable Type
age=25
//String Variable Type
name="Ahmet"
//Float Variable Type
rate=12.4
在Python中使用变量 (Use Variable In Python)
We can use the Python variable like below.
我们可以使用如下所示的Python变量。
//Integer Variable Type
print(age)
//String Variable Type
print(name)
//Float Variable Type
print(rate)
在C / C ++中定义和使用变量 (Define and Use Variables In C/C++)
C and C++ programming languages uses the same syntax for variable definition.
C和C ++编程语言对变量定义使用相同的语法。
在C / C ++中定义变量 (Define Variable In C/C++)
In C/C++ we use variable type before specifying the name of the variable.
在C / C ++中,我们在指定变量名称之前使用变量类型。
//Integer Variable Type
int age=25;
//String Variable Type
char name[]="Ahmet";
//Float Variable Type
float rate=12.4;
在C / C ++中使用变量 (Use Variable In C/C++)
While using the variables in C/C++ we should specify the variable name.
在C / C ++中使用变量时,我们应指定变量名称。
//Integer Variable Type
printf("%d",&age);
//String Variable Type
printf("%s",&name);
//Float Variable Type
printf("%f",&rate);
在Java中定义和使用变量 (Define and Use Variables In Java)
Java variables are similar to the C# and C/C++ programming langues.
Java变量类似于C#和C / C ++编程语言。
在Java中定义变量 (Define Variable In Java)
We will specify the variable types before the variable names.
我们将在变量名称之前指定变量类型。
//Integer Variable Type
int age=25;
//String Variable Type
String name="Ahmet";
//Float Variable Type
float rate=12.4;
在C#中定义和使用变量 (Define and Use Variables In C#)
C# variables are similar to the Java variables.
C#变量类似于Java变量。
在C#中定义变量 (Define Variable In C#)
We will specify the variable types before the variable names.
我们将在变量名称之前指定变量类型。
//Integer Variable Type
int age=25;
//String Variable Type
string name="Ahmet";
//Float Variable Type
float rate=12.4;
在JavaScript中定义和使用变量 (Define and Use Variables In JavaScript)
JavaScript is a dynamic and interpreted language where everything is an object.
JavaScript是一种动态且可解释的语言,其中的一切都是对象。
在JavaScript中定义变量(Define Variable In JavaScript)
We can use var
keyword before the variable name but its optional which makes the definition readable.
我们可以在变量名之前使用var
关键字,但是可以使用var
关键字,这使定义易于阅读。
//Integer Variable Type
var age=25;
//String Variable Type
var name="Ahmet";
//Float Variable Type
var rate=12.4;
在PowerShell中定义和使用变量 (Define and Use Variables In PowerShell)
PowerShell is a dynamic language where types are set automatically.
PowerShell是一种动态语言,可以自动设置类型。
在PowerShell中定义变量 (Define Variable In PowerShell)
We will use $
sign by prefixing the variable name and set the value.
我们将在变量名前使用$
符号并设置值。
//Integer Variable Type
$age=25;
//String Variable Type
var name="Ahmet";
//Float Variable Type
var rate=12.4;

在Bash中定义和使用变量(Define and Use Variables In Bash)
Bash is a shell environment for Linux distributions. It provides some programming features and supports variables.
Bash是Linux发行版的Shell环境。 它提供了一些编程功能并支持变量。
在Bash中定义和使用变量 (Define and Use Variable In Bash)
We can create a variable for Bash like below.
我们可以为Bash创建一个变量,如下所示。
//Integer Variable Type
age=25
//String Variable Type
name="Ahmet"
//Float Variable Type
rate=12.4
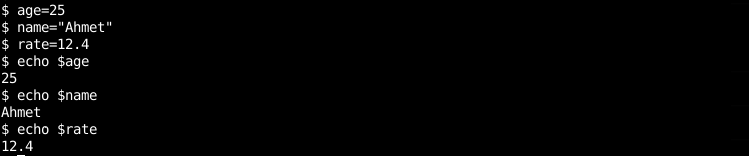
c# powershell