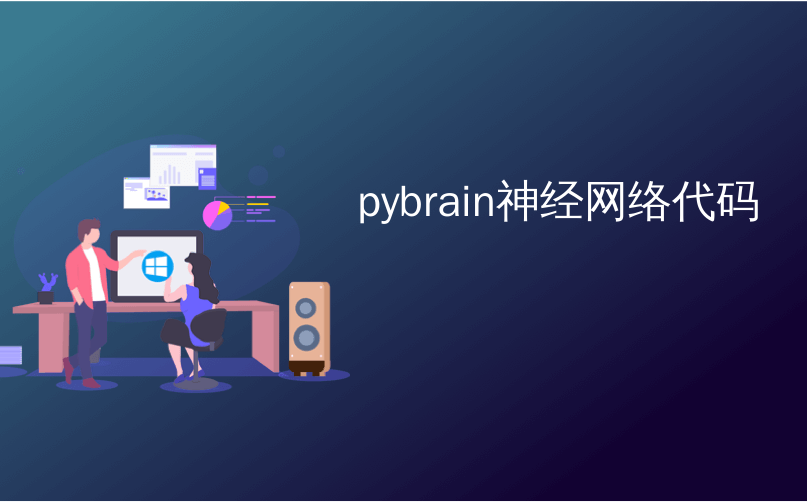
pybrain神经网络代码
PyBrain-网络训练数据集 (PyBrain - Training Datasets on Networks)
So far, we have seen how to create a network and a dataset. To work with datasets and networks together, we have to do it with the help of trainers.
到目前为止,我们已经看到了如何创建网络和数据集。 要一起使用数据集和网络,我们必须在培训师的帮助下进行。
Below is a working example to see how to add a dataset to the network created, and later trained and tested using trainers.
以下是一个工作示例,以了解如何将数据集添加到创建的网络中,并在以后使用培训师进行了培训和测试。
testnetwork.py (testnetwork.py)
from pybrain.tools.shortcuts import buildNetwork
from pybrain.structure import TanhLayer
from pybrain.datasets import SupervisedDataSet
from pybrain.supervised.trainers import BackpropTrainer
# Create a network with two inputs, three hidden, and one output
nn = buildNetwork(2, 3, 1, bias=True, hiddenclass=TanhLayer)
# Create a dataset that matches network input and output sizes:
norgate = SupervisedDataSet(2, 1)
# Create a dataset to be used for testing.
nortrain = SupervisedDataSet(2, 1)
# Add input and target values to dataset
# Values for NOR truth table
norgate.addSample((0, 0), (1,))
norgate.addSample((0, 1), (0,))
norgate.addSample((1, 0), (0,))
norgate.addSample((1, 1), (0,))
# Add input and target values to dataset
# Values for NOR truth table
nortrain.addSample((0, 0), (1,))
nortrain.addSample((0, 1), (0,))
nortrain.addSample((1, 0), (0,))
nortrain.addSample((1, 1), (0,))
#Training the network with dataset norgate.
trainer = BackpropTrainer(nn, norgate)
# will run the loop 1000 times to train it.
for epoch in range(1000):
trainer.train()
trainer.testOnData(dataset=nortrain, verbose = True)
To test the network and dataset, we need BackpropTrainer. BackpropTrainer is a trainer that trains the parameters of a module according to a supervised dataset (potentially sequential) by backpropagating the errors (through time).
要测试网络和数据集,我们需要BackpropTrainer。 BackpropTrainer是一种训练器,它通过对错误(通过时间)进行反向传播,根据受监督的数据集(可能是顺序的)训练模块的参数。
We have created 2 datasets of class - SupervisedDataSet. We are making use of NOR data model which is as follows −
我们创建了2个类的数据集-SupervisedDataSet。 我们正在使用NOR数据模型,如下所示-
A | B | A NOR B |
---|---|---|
0 | 0 | 1 |
0 | 1 | 0 |
1 | 0 | 0 |
1 | 1 | 0 |
一个 | 乙 | A NOR B |
---|---|---|
0 | 0 | 1个 |
0 | 1个 | 0 |
1个 | 0 | 0 |
1个 | 1个 | 0 |
The above data model is used to train the network.
上面的数据模型用于训练网络。
norgate = SupervisedDataSet(2, 1)
# Add input and target values to dataset
# Values for NOR truth table
norgate.addSample((0, 0), (1,))
norgate.addSample((0, 1), (0,))
norgate.addSample((1, 0), (0,))
norgate.addSample((1, 1), (0,))
Following is the dataset used to test −
以下是用于测试的数据集-
# Create a dataset to be used for testing.
nortrain = SupervisedDataSet(2, 1)
# Add input and target values to dataset
# Values for NOR truth table
norgate.addSample((0, 0), (1,))
norgate.addSample((0, 1), (0,))
norgate.addSample((1, 0), (0,))
norgate.addSample((1, 1), (0,))
The trainer is used as follows −
教练的用法如下-
#Training the network with dataset norgate.
trainer = BackpropTrainer(nn, norgate)
# will run the loop 1000 times to train it.
for epoch in range(1000):
trainer.train()
To test on the dataset, we can use the below code −
要测试数据集,我们可以使用以下代码-
trainer.testOnData(dataset=nortrain, verbose = True)
输出量 (Output)
python testnetwork.py (python testnetwork.py)
C:\pybrain\pybrain\src>python testnetwork.py
Testing on data:
('out: ', '[0.887 ]')
('correct:', '[1 ]')
error: 0.00637334
('out: ', '[0.149 ]')
('correct:', '[0 ]')
error: 0.01110338
('out: ', '[0.102 ]')
('correct:', '[0 ]')
error: 0.00522736
('out: ', '[-0.163]')
('correct:', '[0 ]')
error: 0.01328650
('All errors:', [0.006373344564625953, 0.01110338071737218, 0.005227359234093431
, 0.01328649974219942])
('Average error:', 0.008997646064572746)
('Max error:', 0.01328649974219942, 'Median error:', 0.01110338071737218)
If you check the output, the test data almost matches with the dataset we have provided and hence the error is 0.008.
如果检查输出,则测试数据几乎与我们提供的数据集匹配,因此错误为0.008。
Let us now change the test data and see an average error. We have changed the output as shown below −
现在让我们更改测试数据并查看平均误差。 我们更改了输出,如下所示-
Following is the dataset used to test −
以下是用于测试的数据集-
# Create a dataset to be used for testing.
nortrain = SupervisedDataSet(2, 1)
# Add input and target values to dataset
# Values for NOR truth table
norgate.addSample((0, 0), (0,))
norgate.addSample((0, 1), (1,))
norgate.addSample((1, 0), (1,))
norgate.addSample((1, 1), (0,))
Let us now test it.
现在让我们对其进行测试。
输出量 (Output)
python testnework.py (python testnework.py)
C:\pybrain\pybrain\src>python testnetwork.py
Testing on data:
('out: ', '[0.988 ]')
('correct:', '[0 ]')
error: 0.48842978
('out: ', '[0.027 ]')
('correct:', '[1 ]')
error: 0.47382097
('out: ', '[0.021 ]')
('correct:', '[1 ]')
error: 0.47876379
('out: ', '[-0.04 ]')
('correct:', '[0 ]')
error: 0.00079160
('All errors:', [0.4884297811030845, 0.47382096780393873, 0.47876378995939756, 0
.0007915982149002194])
('Average error:', 0.3604515342703303)
('Max error:', 0.4884297811030845, 'Median error:', 0.47876378995939756)
We are getting the error as 0.36, which shows that our test data is not completely matching with the network trained.
我们得到的误差为0.36,这表明我们的测试数据与受过训练的网络不完全匹配。
翻译自: https://www.tutorialspoint.com/pybrain/pybrain_training_datasets_on_networks.htm
pybrain神经网络代码