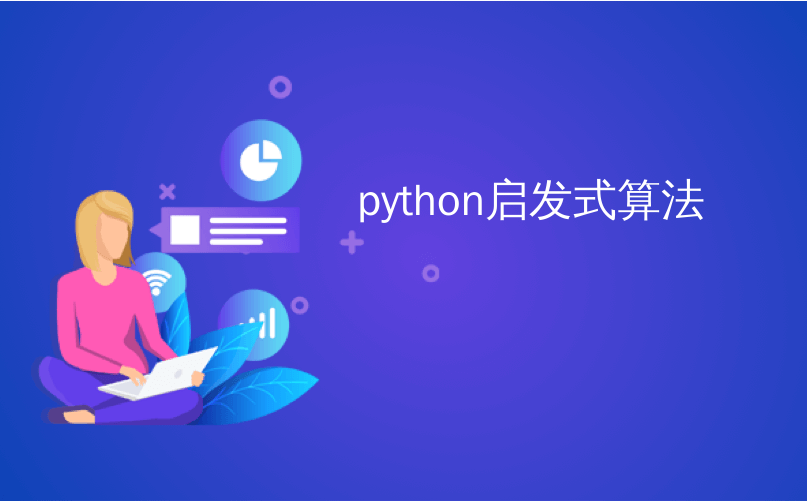
python启发式算法
带有Python的AI –启发式搜索 (AI with Python – Heuristic Search)
Heuristic search plays a key role in artificial intelligence. In this chapter, you will learn in detail about it.
启发式搜索在人工智能中起着关键作用。 在本章中,您将详细了解它。
人工智能中的启发式搜索概念 (Concept of Heuristic Search in AI)
Heuristic is a rule of thumb which leads us to the probable solution. Most problems in artificial intelligence are of exponential nature and have many possible solutions. You do not know exactly which solutions are correct and checking all the solutions would be very expensive.
启发式法则是一种经验法则,它使我们找到了可能的解决方案。 人工智能中的大多数问题都是指数性质的,并且有许多可能的解决方案。 您不确定确切的解决方案是正确的,因此检查所有解决方案将非常昂贵。
Thus, the use of heuristic narrows down the search for solution and eliminates the wrong options. The method of using heuristic to lead the search in search space is called Heuristic Search. Heuristic techniques are very useful because the search can be boosted when you use them.
因此,使用启发式方法缩小了对解决方案的搜索范围,并消除了错误的选择。 使用启发式在搜索空间中进行搜索的方法称为启发式搜索。 启发式技术非常有用,因为当您使用它们时可以增强搜索。
知情搜索和知情搜索之间的区别 (Difference between Uninformed and Informed Search)
There are two types of control strategies or search techniques: uninformed and informed. They are explained in detail as given here −
有两种类型的控制策略或搜索技术:无知和知情。 他们在这里详细解释-
不知情的搜索 (Uninformed Search)
It is also called blind search or blind control strategy. It is named so because there is information only about the problem definition, and no other extra information is available about the states. This kind of search techniques would search the whole state space for getting the solution. Breadth First Search (BFS) and Depth First Search (DFS) are the examples of uninformed search.
也称为盲目搜索或盲目控制策略。 之所以这样命名,是因为仅存在有关问题定义的信息,而没有其他有关状态的信息。 这种搜索技术将搜索整个状态空间以获得解决方案。 广度优先搜索(BFS)和深度优先搜索(DFS)是无信息搜索的示例。
知情搜索 (Informed Search)
It is also called heuristic search or heuristic control strategy. It is named so because there is some extra information about the states. This extra information is useful to compute the preference among the child nodes to explore and expand. There would be a heuristic function associated with each node. Best First Search (BFS), A*, Mean and Analysis are the examples of informed search.
也称为启发式搜索或启发式控制策略。 之所以这样命名,是因为有一些关于状态的额外信息。 此额外信息对于计算要探索和扩展的子节点之间的偏好很有用。 每个节点都会有一个启发式功能。 最佳优先搜索(BFS),A *,均值和分析是知情搜索的示例。
约束满足问题(CSP) (Constraint Satisfaction Problems (CSPs))
Constraint means restriction or limitation. In AI, constraint satisfaction problems are the problems which must be solved under some constraints. The focus must be on not to violate the constraint while solving such problems. Finally, when we reach the final solution, CSP must obey the restriction.
约束表示限制或限制。 在AI中,约束满足问题是必须在某些约束下解决的问题。 在解决此类问题时,重点必须是不违反约束。 最后,当我们达成最终解决方案时,CSP必须遵守这一限制。
约束满足解决的现实世界问题 (Real World Problem Solved by Constraint Satisfaction)
The previous sections dealt with creating constraint satisfaction problems. Now, let us apply this to real world problems too. Some examples of real world problems solved by constraint satisfaction are as follows −
前面的部分涉及创建约束满足问题。 现在,让我们将其也应用于现实世界中的问题。 通过约束满足解决的现实世界问题的一些示例如下-
解决代数关系 (Solving algebraic relation)
With the help of constraint satisfaction problem, we can solve algebraic relations. In this example, we will try to solve a simple algebraic relation a*2 = b. It will return the value of a and b within the range that we would define.
借助于约束满足问题,我们可以解决代数关系。 在此示例中,我们将尝试求解简单的代数关系a * 2 = b 。 它将返回我们定义的范围内的a和b的值。
After completing this Python program, you would be able to understand the basics of solving problems with constraint satisfaction.
完成此Python程序后,您将能够了解使用约束满足条件解决问题的基础。
Note that before writing the program, we need to install Python package called python-constraint. You can install it with the help of the following command −
请注意,在编写程序之前,我们需要安装名为python-constraint的Python包。 您可以在以下命令的帮助下安装它-
pip install python-constraint
The following steps show you a Python program for solving algebraic relation using constraint satisfaction −
以下步骤显示了一个使用约束满足度解决代数关系的Python程序-
Import the constraint package using the following command −
使用以下命令导入约束包-
from constraint import *
Now, create an object of module named problem() as shown below −
现在,创建一个名为issue()的模块的对象,如下所示-
problem = Problem()
Now, define variables. Note that here we have two variables a and b, and we are defining 10 as their range, which means we got the solution within first 10 numbers.
现在,定义变量。 请注意,这里有两个变量a和b,我们将10定义为它们的范围,这意味着我们在前10个数字之内得到了解决方案。
problem.addVariable('a', range(10))
problem.addVariable('b', range(10))
Next, define the particular constraint that we want to apply on this problem. Observe that here we are using the constraint a*2 = b.
接下来,定义我们要对此问题应用的特定约束。 观察到这里我们使用约束a * 2 = b 。
problem.addConstraint(lambda a, b: a * 2 == b)
Now, create the object of getSolution() module using the following command −
现在,使用以下命令创建getSolution()模块的对象-
solutions = problem.getSolutions()
Lastly, print the output using the following command −
最后,使用以下命令打印输出-
print (solutions)
You can observe the output of the above program as follows −
您可以观察到上述程序的输出,如下所示:
[{'a': 4, 'b': 8}, {'a': 3, 'b': 6}, {'a': 2, 'b': 4}, {'a': 1, 'b': 2}, {'a': 0, 'b': 0}]
魔术广场 (Magic Square)
A magic square is an arrangement of distinct numbers, generally integers, in a square grid, where the numbers in each row , and in each column , and the numbers in the diagonal, all add up to the same number called the “magic constant”.
魔方是在方格中排列不同数字(通常是整数)的排列,其中每行,每列中的数字和对角线上的数字加起来等于一个相同的数字,称为“魔术常数” 。
The following is a stepwise execution of simple Python code for generating magic squares −
以下是用于生成魔术方块的简单Python代码的逐步执行-
Define a function named magic_square, as shown below −
定义一个名为magic_square的函数,如下所示-
def magic_square(matrix_ms):
iSize = len(matrix_ms[0])
sum_list = []
The following code shows the code for vertical of squares −
以下代码显示正方形垂直的代码-
for col in range(iSize):
sum_list.append(sum(row[col] for row in matrix_ms))
The following code shows the code for horizantal of squares −
以下代码显示了水平方格的代码-
sum_list.extend([sum (lines) for lines in matrix_ms])
The following code shows the code for horizontal of squares −
以下代码显示了水平正方形的代码-
dlResult = 0
for i in range(0,iSize):
dlResult +=matrix_ms[i][i]
sum_list.append(dlResult)
drResult = 0
for i in range(iSize-1,-1,-1):
drResult +=matrix_ms[i][i]
sum_list.append(drResult)
if len(set(sum_list))>1:
return False
return True
Now, give the value of the matrix and check the output −
现在,给出矩阵的值并检查输出-
print(magic_square([[1,2,3], [4,5,6], [7,8,9]]))
You can observe that the output would be False as the sum is not up to the same number.
您可以观察到输出将为False,因为总和不等于相同的数字。
print(magic_square([[3,9,2], [3,5,7], [9,1,6]]))
You can observe that the output would be True as the sum is the same number, that is 15 here.
您可以观察到输出为True,因为总和是相同的数字,此处为15 。
python启发式算法