/**
* 书本:《Thinking In Java》
* 功能:对象随机地出现,并且要求由数量有限的服务器提供随机数量的服务时间。
* 每个银行顾客要求一定数量的服务时间,这是出纳员必须花费在顾客身上,以服务顾客需求的时间单位的数量。服务时间的数量对每个顾客来说都是不同的,并且是随机确定的。
* 另外,你不知道在每个时间间隔内有多少顾客会到达,因此这也是随机确定的:
* 文件:BankTellerSimulation.java
* 时间:2015年5月10日08:36:06
* 作者:cutter_point
*/
package Lesson21Concurency;
import java.util.LinkedList;
import java.util.PriorityQueue;
import java.util.Queue;
import java.util.Random;
import java.util.concurrent.ArrayBlockingQueue;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import java.util.concurrent.TimeUnit;
//客户类
class Customer
{
private final int serviceTime; //客户要求被服务的时间
public Customer(int serviceTime)
{
this.serviceTime = serviceTime;
}
public int getServiceTime()
{
return serviceTime;
}
@Override
public String toString()
{
return "顾客要求的 [服务时间 =" + serviceTime + "]";
}
}
//顾客队列
/*
* 一个由数组支持的有界阻塞队列。此队列按 FIFO(先进先出)原则对元素进行排序。队列的头部 是在队列中存在时间最长的元素。队列的尾部 是在队列中存在时间最短的元素。
* 新元素插入到队列的尾部,队列获取操作则是从队列头部开始获得元素。
这是一个典型的“有界缓存区”,固定大小的数组在其中保持生产者插入的元素和使用者提取的元素。一旦创建了这样的缓存区,就不能再增加其容量。
试图向已满队列中放入元素会导致操作受阻塞;试图从空队列中提取元素将导致类似阻塞。
*/
class CustomerLine extends ArrayBlockingQueue<Customer>
{
public CustomerLine(int maxLineSize) //数组的最大长度
{
super(maxLineSize);
}
@Override
public String toString()
{
if(this.size() == 0)
return "[没有顾客,为空]";
StringBuilder result = new StringBuilder();
for(Customer customer : this)
{
//吧数组里面的顾客全部取出来,放到string里面去
result.append(customer);
}
return result.toString();
}
}
//顾客产生器,随机产生顾客
class CustomerGenerator implements Runnable
{
//得到顾客的队列
private CustomerLine customers;
//产生随机数
Random rand = new Random(998);
public CustomerGenerator(CustomerLine customers)
{
this.customers = customers;
}
@Override
public void run()
{
try
{
//只要当前线程没有被打断,那么我们就不断地产生新的顾客
while(!Thread.interrupted())
{
//为了随机时间里面产生顾客,产生的顾客需求也是随机地
//随机一段时间 nextInt是产生一个在0到指定的数据之间的数
TimeUnit.MILLISECONDS.sleep(rand.nextInt(300));
//然后队列里面添加一个随机地顾客
customers.put(new Customer(rand.nextInt(1000)));
}
}
catch (InterruptedException e)
{
System.out.println("顾客产生器线程被打断了");
e.printStackTrace();
}
System.out.println("顾客产生器 终结(terminating)");
}
}
//银行出纳员类
class Teller implements Runnable, Comparable<Teller>
{
private static int count = 0; //计数,银行有几个出纳员
private final int id = count++; //当前出纳员的员工id号
private int customersServed = 0; //当前出纳员服务了多少顾客
private CustomerLine customers; //顾客队列
private boolean servingCustome
【ThinkingInJava】64、银行出纳员仿真
最新推荐文章于 2024-03-05 11:44:37 发布
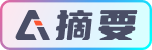