一、应用程序分层
上一篇文章中我们介绍了JDBC连接数据库的基本方法。但是当我们需要执行不同的sql语句时,代码就显得冗余。于是我们就有了分层的思想,应用程序通过创建不同的包来实现项目的分层,将项目中的代码根据功能做具体划分,并存放在不同的包下。
1.1、分层优点
分层结构将应用系统划分为若干层,每一层只解决问题的一部分,通过各层的协作提供整体解决方案。大的问题被分解为一系列相对独立的子问题,局部化在每一层中,这样就有效的降低了单个问 题的规模和复杂度,实现了复杂系统的第一步也是最为关键的一步分解。
分层结构具有良好的可扩展性,为应用系统的演化增长提供了一个灵活的支持,具有良好的可扩展性。增加新的功能时,无须对现有的代码做修改,业务逻辑可以得到最大限度的重用。
分层架构易于维护。在对系统进行分解后,不同的功能被封装在不同的层中,层与层之间的耦合显著降低。因此在修改某个层的代码时,只要不涉及层与层之间的接口,就不会对其他层造成严重影响。
1.2、层级划分
DAO: 把对数据库的所有操作定义成抽象方法,可以提供多种实现。
pojo: 数据库实体类
controller:控制层
service:业务逻辑层
二、Dao层(Database Access Object)数据访问对象
2.1、DAO是什么
DAO(Database Access Object)数据访问对象。主要的功能就是用于进行数据操作的,夹在业务逻辑与数据库资源中间。通俗来讲,就是将数据库操作都封装起来,对外提供相应的接口。
在面向对象设计过程中,有一些"套路”用于解决特定问题称为模式。DAO就是一种模式。
DAO 模式提供了访问关系型数据库系统所需操作的接口,将数据访问和业务逻辑分离对上层提供面向对象的数据访问接口。
2.2、DAO的优点
DAO模式的优势在于,实现了两次隔离。
隔离了数据访问代码和业务逻辑代码。业务逻辑代码直接调用DAO方法即可,完全感觉不到数据库表的存在。分工明确,数据访问层代码变化不影响业务逻辑代码,这符合单一职能原则,降低了藕合性,提高了可复用性。
隔离了不同数据库实现。采用面向接口编程,如果底层数据库变化,如由 MySQL 变成 Oracle 只要增加 DAO 接口的新实现类即可,原有 MySQ 实现不用修改。这符合 "开-闭" 原则。该原则降低了代码的藕合性,提高了代码扩展性和系统的可移植性。
2.3、DAO的组成
2.3.1、DAO基类:BaseDAO(连接数据库)
加载properties文件,实例化Connection,释放资源,执行公共方法。
public class BaseDao {
private static String driver;
private static String url;
private static String user;
private static String pwd;
static {
Properties properties = new Properties();
InputStream inputStream = BaseDao.class.getClassLoader().getResourceAsStream("database.properties");
try {
properties.load(inputStream);
} catch (IOException e) {
e.printStackTrace();
}
// 使用配置文件
driver=properties.getProperty("mysqldriver");
url=properties.getProperty("mysqlurl");
user=properties.getProperty("mysqluser");
pwd=properties.getProperty("mysqlpwd");
}
public Connection getConnection(){
Connection connection = null;
try {
Class.forName(driver);
connection = DriverManager.getConnection(url,user,pwd);
} catch (ClassNotFoundException e) {
e.printStackTrace();
} catch (SQLException e) {
e.printStackTrace();
}
return connection;
}
public void close(Connection connection){
this.close(null,null,connection);
}
public void close(PreparedStatement preparedStatement,Connection connection){
this.close(null,preparedStatement,connection);
}
public void close(ResultSet resultSet,PreparedStatement preparedStatement,Connection connection){
try{
if(null!=resultSet){
resultSet.close();
}
if(null!=preparedStatement){
preparedStatement.close();
}
if(null!=connection){
connection.close();
}
} catch (SQLException e) {
e.printStackTrace();
}
}
/**
* 将新增、修改、删除统一抽象到一个方法中
* @param sqlStr
* @param params
* @return
*/
public int executeUpdate(String sqlStr, Object... params){
Connection connection = this.getConnection();
PreparedStatement preparedStatement = null;
String sql = sqlStr;
int num = -1;
try {
preparedStatement = connection.prepareStatement(sql);
if(null!=params){
for(int i = 0; i < params.length;i++){
preparedStatement.setObject(i+1,params[i]);
}
}
num = preparedStatement.executeUpdate();
} catch (SQLException e){
e.printStackTrace();
} finally {
this.close(preparedStatement,connection);
}
return num;
}
public static void main(String[] args) {
BaseDao baseDao = new BaseDao();
Connection connection = baseDao.getConnection();
System.out.println(connection);
}
}
2.3.2、DAO接口:XxxDao
/*
* 对数据库中Dog表的操作定义,比如,增删改操作
* 新增宠物信息
* 修改宠物信息
* 根据id删除宠物信息
* */
public interface DogDao {
Integer saveDog(Dog dog);
Integer updateDog(Dog dog,Integer id);
Integer delById(Integer id);
}
2.3.3、DAO实现类:XxxDaoImpl(实现DAO接口)
public class DogDaoImpl extends BaseDao implements DogDao{
// 新增宠物信息
public Integer saveDog(Dog dog) {
String sql = "insert into dog(name,health,love,strain,lytm) values(?,?,?,?,now())";
int num = super.executeUpdate(sql, dog.getName(), dog.getHealth(), dog.getLove(), dog.getStrain());
if(num>0){
System.out.println("修改成功");
}
return num;
}
// 修改宠物信息
public Integer updateDog(Dog dog) {
String sql = "update dog set name=?, health=?, love=?,strain=? where id=?";
int num = super.executeUpdate(sql, dog.getName(), dog.getHealth(), dog.getLove(), dog.getStrain(), dog.getId());
if(num>0){
System.out.println("修改成功");
}
return num;
}
// 根据id删除宠物信息
public Integer delById(Integer id) {
String sql = "delete from dog where id=?";
int num = super.executeUpdate(sql,id);
if(num>0){
System.out.println("id为"+id+"的狗子删除成功");
}
return num;
}
}
2.3.4、DAO测试类:XxxDaoTest
// 新增宠物信息
@Test
public void testDogDaoInsert(){
DogDaoImpl dogDao = new DogDaoImpl();
Dog dog = new Dog( "旺财", 100, 88, "中华田园狗");
dogDao.saveDog(dog);
}
// 修改宠物信息
@Test
public void testDogDaoUpdate(){
DogDaoImpl dogDao = new DogDaoImpl();
Dog dog = new Dog();
dog.setNname("可乐");
dog.sethealth(10);
dog.setlove(10);
dog.setId(2);
dogDao.updateDog(dog);
}
// 根据id删除宠物信息
@Test
public void testDogDaoDelete(){
DogDaoImpl dogDao = new DogDaoImpl();
Dog dog = new Dog();
dog.setId(4);
dogDao.deleteDog(dog.getId());
}
三、pojo层(Plain Ordinary Java Object)实体类
也有人称其为model、domain、bean等,pojo层是对应的数据库表的实体类。
根据表结构创建Dog类
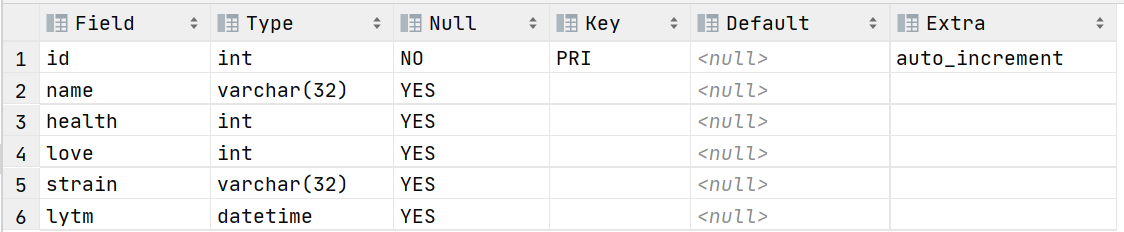
public class Dog {
private Integer id;
private String name;
private Integer health;
private Integer love;
private String strain;
private Date lytm;
// 重写toString函数
public String toString() {
return "Dog{" +
"id=" + id +
", name='" + name + '\'' +
", health=" + health +
", love=" + love +
", strain='" + strain + '\'' +
", lytm=" + lytm +
'}';
}
// 无参构造
public Dog() {
}
// 有参构造
public Dog(Integer id, String name, Integer health, Integer love, String strain, Date lytm) {
this.id = id;
this.name = name;
this.health = health;
this.love = love;
this.strain = strain;
this.lytm = lytm;
}
// 有参构造
public Dog(String name, Integer health, Integer love, String strain) {
this.name = name;
this.health = health;
this.love = love;
this.strain = strain;
}
// getter and setter方法
public Integer getId() {return id;}
public void setId(Integer id) {this.id = id;}
public String getName() {return name;}
public void setName(String name) {this.name = name;}
public Integer getHealth() {return health;}
public void setHealth(Integer health) {this.health = health;}
public Integer getLove() {return love;}
public void setLove(Integer love) {this.love = love;}
public String getStrain() {return strain;}
public void setStrain(String strain) {this.strain = strain;}
public Date getLytm() {return lytm;}
public void setLytm(Date lytm) {this.lytm = lytm;}
}