1 <template> 2 <!-- 生成二维码开放接口: 3 二维码内容[通常为url] 4 二维码大小[限制为正方形] 二维码下方显示:文字 5 二维码中间显示:图片--> 6 <div id="meQrcode" :title="qrText"> 7 <div class="qrcode_box"> 8 <img 9 class="qrcode_canvas" 10 id="qrcode_canvas" 11 ref="qrcode_canvas" 12 alt="二维码本图" 13 /> 14 <img 15 v-if="qrLogo" 16 class="qrcode_logo" 17 ref="qrcode_logo" 18 :src="qrLogo" 19 alt="公司logo" 20 /> 21 <canvas 22 :width="qrSize" 23 :height="qrSize" 24 class="canvas" 25 ref="canvas" 26 ></canvas> 27 </div> 28 </div> 29 </template> 30 <script> 31 import QRCode from "qrcode"; 32 import logo from "./logo11.png"; 33 export default { 34 props: { 35 qrUrl: { 36 type: String, 37 default: "http://www.baidu.com/" 38 }, 39 qrSize: { 40 type: Number, 41 default: 300 42 }, 43 qrText: { 44 default: "百度一下,也不知道" 45 }, 46 qrLogo: { 47 type: String, 48 default: logo 49 }, 50 qrLogoSize: { 51 type: Number, 52 default: 40 53 }, 54 qrTextSize: { 55 type: Number, 56 default: 14 57 } 58 }, 59 data() { 60 return {}; 61 }, 62 methods: { 63 /** 64 * @argument qrUrl 二维码内容 65 * @argument qrSize 二维码大小 66 * @argument qrText 二维码中间显示文字 67 * @argument qrTextSize 二维码中间显示文字大小(默认16px) 68 * @argument qrLogo 二维码中间显示图片 69 * @argument qrLogoSize 二维码中间显示图片大小(默认为80) 70 */ 71 handleQrcodeToDataUrl() { 72 let qrcode_canvas = this.$refs.qrcode_canvas; 73 let qrcode_logo = this.$refs.qrcode_logo; 74 let canvas = this.$refs.canvas; 75 const that = this; 76 QRCode.toDataURL( 77 this.qrUrl, 78 { errorCorrectionLevel: "H" }, 79 (err, url) => { 80 qrcode_canvas.src = url; 81 // 画二维码里的logo// 在canvas里进行拼接 82 let ctx = canvas.getContext("2d"); 83 84 setTimeout(() => { 85 //获取图片 86 ctx.drawImage(qrcode_canvas, 0, 0, that.qrSize, that.qrSize); 87 if (that.qrLogo) { 88 //设置logo大小 89 //设置获取的logo将其变为圆角以及添加白色背景 90 ctx.fillStyle = "#fff"; 91 ctx.beginPath(); 92 let logoPosition = (that.qrSize - that.qrLogoSize) / 2; //logo相对于canvas居中定位 93 let h = that.qrLogoSize + 10; //圆角高 10为基数(logo四周白色背景为10/2) 94 let w = that.qrLogoSize + 10; //圆角宽 95 let x = logoPosition - 5; 96 let y = logoPosition - 5; 97 let r = 5; //圆角半径 98 ctx.moveTo(x + r, y); 99 ctx.arcTo(x + w, y, x + w, y + h, r); 100 ctx.arcTo(x + w, y + h, x, y + h, r); 101 ctx.arcTo(x, y + h, x, y, r); 102 ctx.arcTo(x, y, x + w, y, r); 103 ctx.closePath(); 104 ctx.fill(); 105 ctx.drawImage( 106 qrcode_logo, 107 logoPosition, 108 logoPosition, 109 that.qrLogoSize, 110 that.qrLogoSize 111 ); 112 } 113 if (that.qrText) { 114 //设置字体 115 let fpadd = 10; //规定内间距 116 ctx.font = "bold " + that.qrTextSize + "px Arial"; 117 let tw = ctx.measureText(that.qrText).width; //文字真实宽度 118 let ftop = that.qrSize - that.qrTextSize; //根据字体大小计算文字top 119 let fleft = (that.qrSize - tw) / 2; //根据字体大小计算文字left 120 let tp = that.qrTextSize / 2; //字体边距为字体大小的一半可以自己设置 121 ctx.fillStyle = "#fff"; 122 ctx.fillRect( 123 fleft - tp / 2, 124 ftop - tp / 2, 125 tw + tp, 126 that.qrTextSize + tp 127 ); 128 ctx.textBaseline = "top"; //设置绘制文本时的文本基线。 129 ctx.fillStyle = "#333"; 130 ctx.fillText(that.qrText, fleft, ftop); 131 } 132 canvas.style.display = "none"; 133 qrcode_canvas.src = canvas.toDataURL(); 134 qrcode_canvas.style.display = "inline-block"; 135 }, 0); 136 } 137 ); 138 } 139 }, 141 mounted() { 142 this.handleQrcodeToDataUrl(); 143 }, 144 updated() { 145 this.handleQrcodeToDataUrl(); 146 }, 147 }; 148 </script> 149 <style scoped> 150 .qrcode_box, 151 #meQrcode { 152 display: inline-block; 153 } 154 .qrcode_box img { 155 display: none; 156 } 157 </style>
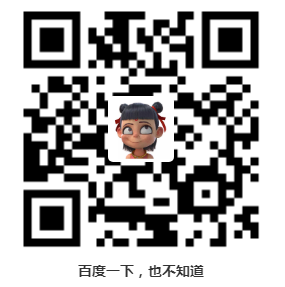
源码地址:https://gitee.com/yolanda624/coffer/tree/master/src/components/a-qrcode