#ifdef python基础
2021年 01月 24日 星期日 18:12:05 CST
1.python使用空格(tap or 空格),而不是括号,来使得代码结构化。
2.冒号:表示缩进代码块的开始,此后所有代码必须缩进相同的数量,直到该块结束。
示例code:
for x in townum:
if num1 < num2:
return num1
else:
return num2
3.python的每一个陈述不使用;来表示结束,但是若一行上有多个陈述,也可以使用;来分隔。
a = 5;b = 6; c = 7
4.python中的每一个数字、字符串、数据结构、函数、类都是python中的一个对象,每一个对象都有一个关联的类型和内部的数据。(这使得python语言非常的灵活)
5.注释(没见到批量注释的方法)
#
6.函数和对象方法调用
6.1 result = f(x,y,z)
6.2 g()
6.3 访问一个对象的方法(函数)的方法
obj.some_method(x,y,z)
6.4 函数
函数可以接受位置参数和关键字参数。
7.变量和参数传递
7.1 在python中,给一个变量赋值,其实是给右值代表的对象创建一个引用,而不是像c语言,会发生拷贝
a = [1,2,3]
b = a
在上述语句中,只是a和b引用了同一个对象。
示例:
#!/usr/bin/python
def main():
a = [1,2,3]
b = a
a.append(4)
print("a");print(a)
print("b");print(b)
c = b
print("c");print(c)
if __name__ == '__main__':
main()
输出:
a
[1, 2, 3, 4]
b
[1, 2, 3, 4]
c
[1, 2, 3, 4]
7.2 参数传递中的规则:
当把一个对象传递给函数,就在一个新的位置创建了一个对该对象的引用。
in:arg_rec.py
#!/usr/bin/python
def append_element(some_list,element):
some_list.append(element)
def main():
data = [1,2,3]
append_element(data,4)
print(data)
if __name__ == '__main__':
main()
out:
root@zyb-ubt:/home/zyb/python/two_chapter# ./arg_rec.py
[1, 2, 3, 4]
!!!弄清楚某种语言是传值调用还是传引用调用是非常重要的,搞不清楚就会碰到很多坑,由以上示例,显然python就是一个传引用调用的语言。
8.动态引用、强类型
in[dynamic_rec.py]:
#!/usr/bin/python
def main():
a = 5
type_a = type(a)
print ("a's type: ",end='')
print (type_a)
b = "type_string!"
type_b = type(b)
print ("b's type: ",end='')
print (type_b)
a+b
if __name__ == '__main__':
main()
out:
root@zyb-ubt:/home/zyb/python/two_chapter# ./dynamic_rec.py
a's type: <class 'int'>
b's type: <class 'str'>
Traceback (most recent call last):
File "/home/zyb/python/two_chapter/./dynamic_rec.py", line 16, in <module>
main()
File "/home/zyb/python/two_chapter/./dynamic_rec.py", line 13, in main
a+b
TypeError: unsupported operand type(s) for +: 'int' and 'str'
以上示例说明,
8.1 python是强类型相关的,也即是,每一个对象都有它自己的类型,类型信息存储在该对象的空间中。
8.2 且隐式类型转换只发生在确切的明显得环境下
in:
>>> a = 4.5
>>> b = 2
>>> print('a is {0},b is {1}'.format(type(a),type(b)))
out:
a is <class 'float'>,b is <class 'int'>
>>>
8.3 检查一个实例是否是某个类型的函数:isinstance(instance,type)
in:(检查一个实例是否是某个类型)
>>> a = 4.5
>>> b = 'string'
>>> isinstance(a,int);
False
>>> isinstance(b,str);
True
in:(检查一个实例是否都是某些类型)
>>> a = 4.5
>>> b = 2
>>> isinstance(a,(int,float))
True
>>> isinstance(a,(int,str))
False
>>>
9.属性和方法
属性:存储在对象内部
方法:用于访问对象内部的数据的函数
in:通过getattr获取某个属性或者方法
>>> a = 'foo'
>>> getattr(a,'split')
<built-in method split of str object at 0xb77a6aa0>
>>>
10.duck typing (鸭子类型:不关注对象本身的类型,只关注其可以使用的方法) (走路像鸭子、叫的像鸭子,那么就是鸭子)
in:
#!/usr/bin/python
def isiterable(obj):
try:
iter(obj)
return True
except TypeError:
return False
def main():
a = isiterable('a string')
print(a)
if __name__ == '__main__':
main()
out:
root@zyb-ubt:/home/zyb/python/two_chapter# ./duck_iterable.py
True
11.imports
python中,一个模块就是一个简单的以.py结尾的文件(包含着其实现的py代码)
11.1 我们自己构造一个module
in[1]:
root@zyb-ubt:/home/zyb/python/two_chapter# cat calc.py
PI = 3.1415926
def f(x):
return x +2
def g(a,b):
return a + b
in[2]:
>>> import calc
>>> result = calc.f(10)
>>> pi = calc.PI
>>> print(result)
12
>>> print(pi)
3.1415926
<==> from calc import f,g,PI
<==> import calc as clc
12.二进制操作符和比较
12.1 比较两个引用是否指向同一个对象
is
is not
in:
>>> a = [1,2,3]
>>> b = a
>>> b is a
True
>>> c = [3,4,5]
>>> c is b
False
>>> c is not b
True
>>>
12.2 ==
和is 比起来,完全不是一回事,==是比较引用指向的对象的值否相同
in:
>>> a = [1,2,3]
>>> b = [1,2,3,4]
>>> c = [1,2,3]
>>> a == b
False
>>> a == c
True
>>>
12.3 二进制操作符汇总
a + b Add a and b
a - b Subtract b from a
a * b Multiply a by b
a / b Divide a by b
a // b 相除且不精确到小数
a ** b 求a的b次幂
>>> a = 2
>>> b = 3
>>> a ** b
8
>>>
a & b
a | b
a ^ b
a == b
a != b
a <= b, a < b
a > b, a >= b
a is b
a is not b
13. 可变和不可变的对象
13.1 可变:对象包含的值可以被改变
13.1.1 lists
in:
>>> a_list = ['foo',2,[4,5]]
>>> a_list[2] = (3,4)
>>> a_list
['foo', 2, (3, 4)]
>>>
dicts
NumPy arrarys
用户自定义类
13.2 不可变
strings(字符串)
tuples(元祖) ============================= 搞清楚数据结构的特性,避免日后使用的时候就各种"莫名奇妙"
>>> a_tuple = (3,5,(4,5))
>>> a_tuple[1] = 'four'
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: 'tuple' object does not support item assignment
>>>
14. 标量类型(标量类型只能有一个值,而复合类型可以包含多个值)
None python中的null值
str 字符串类型,其所属对象用于存储UTF-8编码的字符串
bytes Raw ASCII bytes (or Unicode encoded as bytes)
bool True/False
float 双精度64位的浮点数
int 任意精确度的整数
和c语言的int---由在32位或者64位架构来决定不同,python的int可以存储任意精度的整数,请仔细看以下示例:
in:
>>> ival = 17239871
>>> ival ** 6
26254519291092456596965462913230729701102721 #(远大于2^32的数)
>>> c = ival ** 6
>>> print(type(c))
<class 'int'>//还是int,但是是python的int
15.一些函数
15.1 print
15.1.1 输出不换行
print的默认输出是带有换行符的,可以这样使用以求不换行输出 print("output_content",end='')
15.1.2 print格式化输出 (format)
in:
>>> a = 4.5
>>> b = 2
>>> print('a is {0},b is {1}'.format(type(a),type(b)))
out:
a is <class 'float'>,b is <class 'int'>
>>>
15.2 str
将python对象转化为字符串对象
>>> a = 5.6
>>> s = str(a)
>>> print(type(s))
<class 'str'>
>>> print(s)
5.6
>>>
15.3 count() //计算字符串中某个字符或者单词出现的个数
in:
>>> b = 'this is a long string'
>>> b.count('l')
1
>>> b.count('i')
3
>>>
in:
>>> b = 'this is a long long long long string'
>>> b.count('long')
4
>>>
15.4 list(s) //针对字符串的,字符串就是一系列的unicode字符,所以可以被看作list和tuple
in:
>>> a = 5.6
>>> s = str(a)
>>> print(type(s))
<class 'str'>
>>> print(s)
5.6
>>>
in:
>>> a = 10000101
>>> list(a)
Traceback (most recent call last):
File "<pyshell#78>", line 1, in <module>
list(a)
TypeError: 'int' object is not iterable
>>> tuple(a)
Traceback (most recent call last):
File "<pyshell#79>", line 1, in <module>
tuple(a)
TypeError: 'int' object is not iterable
>>>
slicing 切片 ***
in:
>>> s = 'python'
>>> list(s)
['p', 'y', 't', 'h', 'o', 'n']
>>> s[:3]
'pyt'
>>> s[:5]
'pytho'
>>>
in:
>>> s = 'python'
>>> list(s)
['p', 'y', 't', 'h', 'o', 'n']
>>> s[:3]
'pyt'
>>> s[:5]
'pytho'
>>> s[2:3] =========================== 非常有用 ==========
't'
>>> s[1:4]
'yth'
>>>
15.5 若字符串中有很多\,为了不被隐式解释,则可以在字符串前使用r来转换。
in:
>>> s = r"this\has\no\special\character"
>>> print(s)
this\has\no\special\character
>>> s = "this\has\no\special\character"
>>> print(s)
this\has
o\special\character
>>>
15.6 模板化和格式化
template = '{0:.2f} {1:s} are worth US${2:d}'
模板解释:
• {0:.2f} 参数1格式化为一个待两位小数的浮点数
• {1:s} 参数2格式化为一个字符串
• {2:d} 参数3格式化为一个整数
in:
>>> template = '{0:.2f} {1:s} are worth US${2:d}'
>>> template.format(4.5678,'ARgent Pesos',1)
'4.57ARgent Pesos are worth US$1'
>>>
16. 字节和unicode
in:
>>> val = "espan"
>>> val
'espan'
>>> val_utf8 = val.encode('utf-8')
>>> val_utf8
b'espan'
>>> type(val_utf8)
<class 'bytes'>
>>>
17,bool
True or False
18.类型转化 Type casting
in:
>>> s = '3.14159'
>>> fval = float(s)
>>> type(fval)
<class 'float'>
>>> fval
3.14159
>>> int(fval)
3
>>> bool(fval)
True
>>> bool(0)
False
>>>
19.None
python的null表示,也常用于函数参数中
20.日期和时间
太多,赞留
21.数的类型:整数和浮点数
python中表示数的只有两种类型,一种是int,一种是flaot,一个表示任意精度的整数,一个表示双精度64位的浮点数。
两种类型表示所有的数,化简为繁的思想。
22.字符串(不可改变)
使用''或者""表示的字符串,对于多行的字符串,可使用'''或者"""来表示
in:
>>> a = '''
... this is a multiline strings first
... akjcnasklcn second
... ascknaslckaslc123e123412 third
... '''
>>> print(a) #请注意,有4行
this is a multiline strings first
akjcnasklcn second
ascknaslckaslc123e123412 third
>>>
in:
>>> a = '''
... ascnmasklcnas coansc first
... a1239812812312 second
... ascvnkascnalkscav third
... '''
>>> print(a.count('\n'))
4
>>> a.count('\n')
4
>>>
字符串的不可改变的体现
>>> b = 'this is a long string'
>>> a = b.replace('string',"long")
>>> print(a)
this is a long long
>>> print(b) ===================== b依旧是'this is a long string',充分说明字符串的不可变,其实这一点非常好,我未学习python之前,以为其字符串和shell的变量类似,处于随时可改变的状态,r如果是这样,维护那些写的比较复杂的程序,将会是一场的艰难。(某个不熟悉业务的同事改了某个字符串,那么另一个人在不知情的情况下去寻找引起的问题....)
this is a long string
>>>
23.程序控制流
23.1 if,elif,else
in:
if x < 0:
print("x is less than 0!")
elif x == 0:
print("x is equal 0")
elif 0 < x < 5:
print("x is between 0 and 5")
else
print("x is equal or more than 5")
23.2 for循环
in:
root@zyb-ubt:/home/zyb/python/two_chapter# cat for_t.py
#!/usr/bin/python
def for_calc():
total = 0
sequence = [11,2,None,4,None,5]
for value in sequence:
if value is None:
continue
total += value
print(total)
def main():
for_calc()
if __name__ == "__main__":
main()
root@zyb-ubt:/home/zyb/python/two_chapter# ./for_t.py
22
23.3 while循环
root@zyb-ubt:/home/zyb/python/two_chapter# cat while_t.py
#!/usr/bin/python
def while_calc():
x = 256
total = 0
while x > 0:
if total > 500:
print("break up the while")
break
total += x
x = x//2
def main():
while_calc()
if __name__ == "__main__":
main()
root@zyb-ubt:/home/zyb/python/two_chapter# ./while_t.py
break up the while
root@zyb-ubt:/home/zyb/python/two_chapter#
23.4 无声明
pass
in:
if x < 0:
print("x is less than 0")
elif x == 0:
#目前啥也不干
pass
else
print("more than 0")
23.5 range
in:
>>> list(range(0,20,1))
[0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19]
>>> list(range(0,20))
[0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19]
>>> list(range(20,20,1))
[]
>>> list(range(20,0,1))
[]
>>> list(range(20,0,-1))
[20, 19, 18, 17, 16, 15, 14, 13, 12, 11, 10, 9, 8, 7, 6, 5, 4, 3, 2, 1]
>>> list[-1,-2]
list[-1, -2]
>>> list(range(20,0,-5))
[20, 15, 10, 5]
>>> list(range(20,0,-3))
[20, 17, 14, 11, 8, 5, 2]
>>> list(range(20,-9,-1))
[20, 19, 18, 17, 16, 15, 14, 13, 12, 11, 10, 9, 8, 7, 6, 5, 4, 3, 2, 1, 0, -1, -2, -3, -4, -5, -6, -7, -8]
>>>
23.6 三元表达式
in:
>>> x = 5
>>> "more than 0" if x > 0 else "less or equal than 0"
'more than 0'
>>>
2021年 01月 26日 星期二 22:43:33 CST
23.7 python内置数据结构
1.tuple 元组
in:创建元组
>>> tup = 4,5,6
>>> tup
(4, 5, 6)
>>> neset_tup = (4,5,6),(7,8)
>>> neset_tup
((4, 5, 6), (7, 8))
in:可以将任何序列或者迭代器转换为元组
>>> tuple([4,0,2])
(4, 0, 2)
>>>
in:
>>> tup = tuple("string")
>>> tup
('s', 't', 'r', 'i', 'n', 'g')
>>>
in:倍乘
In [14]: ('foo', 'bar') * 4
Out[14]: ('foo', 'bar', 'foo', 'bar', 'foo', 'bar', 'foo', 'bar')
in:打开元组
>>> tup
('s', 't', 'r', 'i', 'n', 'g')
>>> tup[1]
't'
>>> tup[0]
's'
>>> a,b,c = tup #说明此种打开元组的方法是错误的,必须一对一
Traceback (most recent call last):
File "<pyshell#19>", line 1, in <module>
a,b,c = tup
ValueError: too many values to unpack (expected 3)#注意报错,too Many
>>> a,b,c,d,e,f = tup
>>> a
's'
>>> b
't'
in:
>>> tup = 4,5,(6,7)
>>> a,b,(c,d) = tup
>>> d
7
>>> A,B,C,D = tup #说明此种打开元组的方法是错误的,还是必须一对一 <<<<=====================================|
Traceback (most recent call last): |
File "<pyshell#29>", line 1, in <module> |
A,B,C,D = tup |
ValueError: not enough values to unpack (expected 4, got 3)#注意报错,not enough |
>>> |
|
in:利用元组反转成员 |
|
In [21]: a, b = 1, 2 |
|
In [22]: a |
Out[22]: 1 |
|
In [23]: b |
Out[23]: 2 |
|
In [24]: b, a = a, b |
|
In [25]: a |
Out[25]: 2 |
|
In [26]: b |
Out[26]: 1 |
|
in:利用变量的解包特性来遍历元组或者链表 |
|
>>> seq = [(1,2,3),(4,5,6),(7,8,9)] |
>>> for a,b,c in seq: |
print("a{0},b{1},c{2}".format(a,b,c)) |
a1,b2,c3 |
a4,b5,c6 |
a7,b8,c9 |
>>> |
|
>>> seq = [[1,2,3],[4,5,6],[7,8,9]] |
>>> for a,b,c in seq: |
print("a{0},b{1},c{2}".format(a,b,c)) |
a1,b2,c3 |
a4,b5,c6 |
a7,b8,c9 |
>>> |
in: |
>>> a,b,*c = values =============================================================================================-
*的用法解决了上面的必须一对一问题
>>> c
[3, 4, 5]
>>>
>>> e,f,g = c
>>> e,f,g
(3, 4, 5)
>>>
1.1 访问元组成员 [] tup[0]
1.2 属性:
(1) 创建元组后,就无法修改每个插槽中存储的对象。
(2) 若元组中的某个对象是可更改的,则即使该对象是元组的成员也是可以更改的。
以上两个性质并不矛盾,元组一旦创建不可改,指的创建元组以后的每一个对象就固定了。
而该对象可改,是指在该对象不变成另一个对象的情况下可改变其内容。
1.3 方法
由tuple的不可修改属性决定,所以tuple可用的方法就很少。
tuple.count()
>>> a = ("aaa","bbb","ccc")
>>> a
('aaa', 'bbb', 'ccc')
>>> type(a)
<class 'tuple'>
>>> a.count(1)
0
>>> a.count("aaa")
1
>>>
2.List 链表是可变长度、内容可改可替换的。
1.list的创建
in:
>>> a_list = [2,3,7,None]
in:
>>> tup = ("foo","bar","baz")
>>> b_list = list(tup)
>>> b_list
['foo', 'bar', 'baz']
>>> b_list[1]
'bar'
2.list的增删查改函数
(1) insert(pos,"word") 在list中的pos位序插入一个元素
in:
>>> b_list.insert(2,"four")
>>> b_list
['foo', 'bar', 'four', 'baz']
>>>
(2) append() 尾插入一个元素
in:
>>> b_list.append("five")
>>> b_list
['foo', 'bar', 'four', 'baz', 'five']
>>>
(3) pop(pos) 将pos位序的元素移除list并返回
in:
>>> b_list.pop(0)
'foo'
>>> b_list
['bar', 'four', 'baz', 'five']
>>>
(4) remove("word") 移除list中为"word"的元素,
pop是以位置来遍历和移除,remove是以内容来遍历和移除,所以remove在具有多个相同的元素的情况下如果我们只想删除其中一个,那么remove一定不能使用。
in:
>>> b_list
['bar', 'baz', 'five', 'aaa', 'bbb', 'ccc', 'aaa'] #具有相同元素的情况下
>>> b_list.remove("aaa")
>>> b_list
['bar', 'baz', 'five', 'bbb', 'ccc', 'aaa']
>>>
in:
>>> b_list
['bar', 'four', 'baz', 'five']
>>> b_list.remove("four")
>>> b_list
['bar', 'baz', 'five']
>>>
(5) in 判断元素是否在list中
in:
>>> 'abc' in b_list
False
(6) not in
同上
(7) + 合并list
in:
>>> a_list
[2, 3, 7, None]
>>> b_list
['bar', 'baz', 'five']
>>> a_list + b_list
[2, 3, 7, None, 'bar', 'baz', 'five']
>>>
(8) 扩展list
in:
>>> b_list
['bar', 'baz', 'five']
>>> b_list.extend(["aaa","bbb","ccc"])
>>> b_list
['bar', 'baz', 'five', 'aaa', 'bbb', 'ccc']
>>>
(9)
in:
>>> list_arrary = [[1,2,3],[4,5,6],[7,8,9]]
>>> list_arrary[0]
[1, 2, 3]
>>> list_arrary[1]
[4, 5, 6]
>>> list_arrary[2]
[7, 8, 9]
>>> for chunk in list_arrary:
... everything = everything + chunk
...
>>>
>>> everything
[1, 2, 3, 4, 5, 6, 7, 8, 9]
>>>
(10) 排序
sort
in: 按照位序排序
>>> b_list
['bar', 'baz', 'five', 'bbb', 'ccc', 'aaa']
>>> b_list.sort()
>>> b_list #由此可见,排序会彻底改变链表中元素的当前书讯
['aaa', 'bar', 'baz', 'bbb', 'ccc', 'five']
>>>
in: sort(key=len) 按照字符串长度排序
(1)
>>> num_list = [1000,200,323,12,1,23,123]
>>> num_list
[1000, 200, 323, 12, 1, 23, 123]
>>> num_list.sort(key=len)
Traceback (most recent call last): #sort(key=len)只针对str类型的list
File "<stdin>", line 1, in <module>
TypeError: object of type 'int' has no len()
(2)
>>> string_list = ['aaa','asncasckjn','bb','879023']
>>> string_list.sort()
>>> string_list
['879023', 'aaa', 'asncasckjn', 'bb']
>>> string_list.sort(key=len)
>>> string_list
['bb', 'aaa', '879023', 'asncasckjn']
>>>
(3) import bisect #补充了对有序链表进行二分查找和插入有序链表的方法
#import bisect是用于有序链表的,若用于无序链表那么就可能达不到我们想要的结果
in:
>>> import bisect
>>> c = [1,2,2,2,3,4,7]
>>> bisect.bisect(c,2) #插入c中的一个值为2的元素,并返回其插入位置
4
>>> c
[1, 2, 2, 2, 3, 4, 7]
>>>
in:
>>> c
[1, 2, 2, 2, 3, 4, 6, 7]
>>> bisect.insort(c,10)
>>> c
[1, 2, 2, 2, 3, 4, 6, 7, 10]
>>>
(11) slice 切片
list[start:end] 列出从start开始到end-1位置的所有元素
>>> c
[1, 2, 2, 2, 3, 4, 6, 7, 10]
>>> c[1:5]
[2, 2, 2, 3]
>>> c[1:8]
[2, 2, 2, 3, 4, 6, 7]
>>>
使用组合:
list[:end]
list[start:]
list[-start:-end] #从后往前数
list[::increment] #以increment为位序的递加因子的list
in:
>>> c[::]
[1, 2, 2, 2, 3, 4, 6, 7, 10]
>>> c[::2]
[1, 2, 3, 6, 10]
>>> c[::5]
[1, 4]
>>>
当increment == -1时,能起到逆转list的作用
in:
>>> c[::-1]
[10, 7, 6, 4, 3, 2, 2, 2, 1]
>>>
(12) 一些有用的序列函数
12.1 enumerate
我们写程序员时经常有for循环遍历的用法,而enumerate
创建元素位序和元素值得映射关系
in:
>>> mapping = {}
>>> some_list
['foo', 'bar', 'baz']
>>> for i, v in enumerate(some_list):
... mapping[v] = i
...
>>> mapping
{'foo': 0, 'bar': 1, 'baz': 2}
>>>
in:
>>> mapping.clear()
>>> mapping
{}
>>> for i, v in enumerate(some_list):
... mapping[i] = v
...
>>> mapping
{0: 'foo', 1: 'bar', 2: 'baz'}
>>>
12.2:sorted
将任何序列进行排序并返回一个新的list。和之前的sort不可逆不同
in:
>>> a = [912,12,12312123,2312,1,23,2,312]
>>> sorted(a)
[1, 2, 12, 23, 312, 912, 2312, 12312123]
>>> a
[912, 12, 12312123, 2312, 1, 23, 2, 312]
>>> a.sort()
>>> a
[1, 2, 12, 23, 312, 912, 2312, 12312123]
>>>
12.3:zip
将多个list、多个元祖、或其他类型的多个序列构造成一个由多个元祖组成的list,构造规则按照多个list中的元素一对一的方式。最终生成的元素成员为tuple的list,list成员个数序列中成员数最少的序列的个数决定。
in:
>>> seq1 = ['foo','bar','baz']
>>> seq2 = ['one','two','three','four']
>>> zipped = zip(seq1,seq2)
>>> list(zipped)
[('foo', 'one'), ('bar', 'two'), ('baz', 'three')]
12.4:reversed 反转链表
3.dict (hash map 或者关联数组)
插入:
in:
empty_dict = {}
>>> d1 = {'a':'some value','b':[1,2,3,4]}
>>> d1['a']
'some value'
>>> d1['some value'] #不能这样访问
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
KeyError: 'some value'
>>> d1[7] = 'an integer' #插入新映射关系
>>> d1
{'a': 'some value', 'b': [1, 2, 3, 4], 7: 'an integer'}
>>>
删除:
del:
in:
>>> del d1['a']
>>> d1
{'b': [1, 2, 3, 4], 7: 'an integer'}
>>>
pop
in:
>>> d1
{'b': [1, 2, 3, 4], 7: 'an integer'}
>>> d1.pop('b')
[1, 2, 3, 4]
>>> d1
{7: 'an integer'}
>>>
keys: 得到键
values: 得到值
in:
>>> d1 = {'a':'some value','b':[1,2,3,4]}
>>> list(d1.keys())
['a', 'b']
>>> list(d1.values())
['some value', [1, 2, 3, 4]]
>>>
update(): 更新或者插入新的键值对
in:
>>> d1 = {'a':'some value','b':[1,2,3,4]}
>>> list(d1.keys())
['a', 'b']
>>> list(d1.values())
['some value', [1, 2, 3, 4]]
>>> d1.update({'b':'foo','c':12})
>>> d1
{'a': 'some value', 'b': 'foo', 'c': 12} #请注意,b的value变了
>>>
从序列创建dicts:
1.一个使用情形:你需要将两个链表创建为一个键值对。
in:
>>> mapping = {}
>>> key_list = {1,2,3,4,5}
>>> values_list = {"one","two","three","four"}
>>> for key,value in zip(key_list,values_list):
... mapping[key] = value
...
>>> mapping
{1: 'one', 2: 'three', 3: 'four', 4: 'two'}
>>>
获取默认值
in:
>>> mapping
{1: 'one', 2: 'three', 3: 'four', 4: 'two'}
>>> key_list
{1, 2, 3, 4, 5}
>>> value = mapping.get(5,"no") #指定查找map中key为5的元素,查找不到就返回默认值"no"
>>> value
'no'
>>>
setdefault hash_map.setdefault(letter,default):在hash_map中查找键lette对应的值,若该key不存在,则创建新的键值对.
1.一个使用情形:将一个成员为str的list,根据其str成员的首字母,构建一个dict
in:
>>> words = ['apple','bat','bar','atom','book']
>>> by_letter = {}
>>> for word in words:
... letter = word[0]
... by_letter.setdefault(letter,[]).append(word)
...
>>> by_letter
{'a': ['apple', 'atom'], 'b': ['bat', 'bar', 'book']}
>>>
2.hash 检测一个hash函数是否可以进行hash。也就是具有映射关系
in:
>>> hash('string')
-251606679
>>> hash((1,2,(2,3)))
-1552955886
>>> hash((1,2,[2,3]))
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: unhashable type: 'list'
>>>
4.set set是一些无序的、值唯一的元素的集合
创建:
set([2,3,3,8,5,6,7]) 或者 {2,3,3,8,5,6,7}
in:
>>> set([2,3,3,8,5,6,7])
{2, 3, 5, 6, 7, 8}
>>> {2,3,3,8,5,6,7}
{2, 3, 5, 6, 7, 8}
>>>
union 合并两个集合为一个集合 a_set | b_set
intersection 返回两个集合的交集 a_set.intersection(b_set) a_set & b_set
相关操作:
a.add(x)
a.clear()
a.remove(x)
a.pop() N/A Remove an arbitrary element from the set a, raising
KeyError if the set is empty
a.union(b)
a.update(b) a |= b Set the contents of a to be the union of the
elements in a and b
a.intersection(b)
a.intersection_update(b) a &= b Set the contents of a to be the intersection of the
elements in a and b
a.difference(b) a - b The elements in a that are not in b
a.difference_update(b) a -= b Set a to the elements in a that are not in b
a.symmetric_difference(b) a ^ b All of the elements in either a or b but not both
a.symmetric_difference_update(b) a ^= b Set a to contain the elements in either a or b but
not both
a.issubset(b) 如果集合a是b的子集,则返回True。
a.issuperset(b) 如果集合b是a的子集,则返回True。
a.isdisjoint(b) 如果集合a和b没有公共元素则返回True。
5.理解list、set、dict
list可以通过精准的描述来构建 [expr for val in collection if condition]
in:
>>> strings = ['a','as','bat','car','dove','python']
>>> [x.upper() for x in strings if len(x) > 2]
['BAT', 'CAR', 'DOVE', 'PYTHON']
>>>
dict dict_comp = {key-expr : value_expr for value in collection if condition}
set set_com = {expr for value in collection if condition}
in:
>>> some_tuples = [(1, 2, 3), (4, 5, 6), (7, 8, 9)]
>>> flattened = [x for tup in some_tuples for x in tup]
>>> flattened
[1, 2, 3, 4, 5, 6, 7, 8, 9]
>>>
这个示例表明,无论list递归了几次,我们都可以采取从外到内来地来构造表达式从而获取到元素
23.8 函数 python中,函数是主要和重要的代码组织和重用的方法。
1.声明: def
2.返回: return 可以返回多个声明,若一个函数没有return,则会自动返回None
in[1]:返回多个参数
root@zyb-ubt:/home/zyb/python/two_chapter# ./ret_multi.py
(5, 6, 7)
root@zyb-ubt:/home/zyb/python/two_chapter# cat ret_multi.py
#!/usr/bin/python
def f():
a = 5
b = 6
c = 7
return a,b,c
if __name__ == "__main__":
print(f())
root@zyb-ubt:/home/zyb/python/two_chapter#
in[2]: 返回list类型的数据
root@zyb-ubt:/home/zyb/python/two_chapter# cat ret_multi.py
#!/usr/bin/python
def f():
a = 5
b = 6
c = 7
return a,b,c
def ret_list():
a = []
for i in range(20):
a.append(i)
return a
if __name__ == "__main__":
#print(f())
print(ret_list())
root@zyb-ubt:/home/zyb/python/two_chapter# ./ret_multi.py
[0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19]
root@zyb-ubt:/home/zyb/python/two_chapter#
以上两个示例充分说明,python中的函数,可以灵活返回
3.参数:
位置参数
关键词参数:关键词参数用于确认默认值或者可选参数
1.若函数的参数中既有关键值参数也有位置参数,则关键词参数必须跟在位置参数之后。
2.关键词参数的声明顺序和使用顺序可以不一致,说明清楚就行
my_function(x=5,y=6,z=7)
my_function(y=6,x=5,z=7)
示例:
def my_function(x,y,z=0.7)
使用 my_function(5,7,z=0.7) or my_function(3.14,7,3.5) or my_function(10,20)
4.命名空间namespace:
1.函数可以访问两个空间的变量:全局空间、局部空间,说的更明白点,python使用namespace来描述一个变量的范围。
2.默认情况下,在函数内分配的所有变量都分配给局部命名空间。
3.当函数被调用后,局部命名空间立即被创建,并被函数的参数给填充(函数参数本来就是函数内部的变量),在函数调用结束,局部namespace就立即被释放。
4.可以在函数内给外部的变量赋值,但是该外部的变量必须先被声明。
in:
root@zyb-ubt:/home/zyb/python/two_chapter# cat func_t.py
#!/usr/bin/python
a = None
def func():
#a = []
global a
a = [] # 这一步也是必不可少的,如果缺少这一步,将报错“AttributeError: 'NoneType' object has no attribute 'append'”,就是不确定a的对象类型
for i in range(5):
a.append(i)
return a
def main():
b = func()
print(b)
if __name__ == "__main__":
main()
root@zyb-ubt:/home/zyb/python/two_chapter# ./func_t.py
[0, 1, 2, 3, 4]
root@zyb-ubt:/home/zyb/python/two_chapter#
5.函数也是对象:所以我们也可以使用函数对象的许多方法。
in:数据清洗
我们要将成员类型为str的list进行一系列操作清洗操作:去空格、去除特殊字符、统一大小写等。
(待处理字符串)
states = [' Alabama ', 'Georgia!', 'Georgia', 'georgia', 'FlOrIda','south carolina##', 'West virginia?']
按照正常逻辑:
in:
root@zyb-ubt:/home/zyb/python/two_chapter# cat clean_list.py
#!/usr/bin/python
import re
states = [' Alabama ', 'Georgia!', 'Georgia', 'georgia', 'FlOrIda','south carolina##', 'West virginia?']
def clean_strings(strings):
result = []
for value in strings:
value = value.strip();
value = re.sub('[!#?]','',value) #将字符串中包含有!、#、?的字符替换为无
value = value.title()
result.append(value)
return result
if __name__=="__main__":
print(clean_strings(states))
root@zyb-ubt:/home/zyb/python/two_chapter# ./clean_list.py
['Alabama', 'Georgia', 'Georgia', 'Georgia', 'Florida', 'South Carolina', 'West Virginia']
将函数当做对象的逻辑:
root@zyb-ubt:/home/zyb/python/two_chapter# cat fun_obj.py
#!/usr/bin/python
import re
def remove_punctuation(value):
return re.sub('[!#?]','',value)
clear_ops = [str.strip,remove_punctuation,str.title]
def clean_stirngs(strings,ops):
result = []
for value in strings:
for function in ops:
value = function(value)
result.append(value)
return result
states = [' Alabama ', 'Georgia!', 'Georgia', 'georgia', 'FlOrIda','south carolina##', 'West virginia?']
if __name__=="__main__":
print(clean_stirngs(states,clear_ops))
root@zyb-ubt:/home/zyb/python/two_chapter# ./fun_obj.py
['Alabama', 'Georgia', 'Georgia', 'Georgia', 'Florida', 'South Carolina', 'West Virginia']
root@zyb-ubt:/home/zyb/python/two_chapter#
这种写法,能够很方便的扩展你对字符串的操作,万一以后你对数据的操作要求变了,比如你要增加一个功能:将字符串全部转化为小写,那么只需要在clear_ops中增加str.lower即可完成。
这里说一下python中的函数,若str.lower()是调用lower函数,而str.lower是定义一个函数指针,该函数指针指向函数lower()
5.lambda函数: 一个匿名函数的声明方式,只能有单独的一条语句。
def short_functino(x):
return x * 2
<==>
equi_anon = lamba x:x * 2
适用于那种在函数中使用函数作为参数的函数。
>>> strings = ['foo', 'card', 'bar', 'aaaa', 'abab']
>>> strings.sort(key=lambda x:len(set(list(x))))
>>> strings
['aaaa', 'foo', 'abab', 'bar', 'card']
6.函数派生:
看不出有什么用且陌生,后续用到再看
7.Generator: 能遍历序列、文本行,是pyhton中的一个很重要的特征,随之,我们应具有一些使得对象可转化为能遍历的函数。(我们需要生成序列)
(迭代器是一个对象,它将它指向的对象传递给Python解释器)
创建一个生成器,那么就要使用yield而不是return.
def squares(n=10)
print("Generating squares from 1 to {0}".format(n **2))
for i in range(1,n+1):
yield i **2
当调用生成器时,并不会有代码被执行,而当你访问生成器中的成员时,生成器中的代码才会被执行。
in:
root@zyb-ubt:/home/zyb/python/three_chapter# ./generator_t.py
<generator object squares at 0xb7892290>
start.....................
Generating squares from 1 to 100
1 4 9 16 25 36 49 64 81 100
root@zyb-ubt:/home/zyb/python/three_chapter# cat generator_t.py
#!/usr/bin/python
def squares(n=10):
print("Generating squares from 1 to {0}".format(n **2))
for i in range(1,n+1):
yield i **2
def main():
gen = squares()
print(gen)
print("start.....................")
for x in gen:
print(x,end=' ')
print()
if __name__=="__main__":
main()
root@zyb-ubt:/home/zyb/python/three_chapter#
8.标准库itertools
combinations(iterable, k)
permutations(iterable, k)
groupby(iterable[, keyfunc])
product(*iterables, repeat=1)
9.出错和异常处理
in1:
def attempt_float(x):
try:
return float(x)
except: #若出现异常情况,就将参数返回
return x
in2:
def attempt_float(x):
try:
return float(x)
except ValueError: #只处理ValueError这种情况
return x
in3:
def attempt_float(x):
try:
return float(x)
except (TypeError,ValueError): #处理某个错误集合中的任何一种情况
return x
不处理任何错误
in4:
def open_file(path):
f = open(path,'w')
try:
write_to_file(f)
finally:
f.close()
23.9 文件和操作系统
read([size]) 返回读到的字符串,该长度为size
readlines([size]) Return list of lines in the file, with optional size argument
write(str) Write passed string to file
writelines(strings) Write passed sequence of strings to the file
close() Close the handle
flush() Flush the internal I/O buffer to disk
seek(pos) Move to indicated file position (integer)
tell() Return current file position as in
in:
root@zyb-ubt:/home/zyb/python/three_chapter# cat file_ops.py
#!/usr/bin/python
def main():
f = open('./readme.txt','r')
line = f.read(10)
print(line)
f.close()
if __name__=="__main__":
main()
root@zyb-ubt:/home/zyb/python/three_chapter# ./file_ops.py
2021年 02月
#endif
python基础
最新推荐文章于 2022-10-21 18:35:00 发布
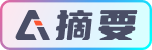