vue中vuex组件通信
讨论组件如何在彼此之间大规模共享数据,并使用示例代码使之在Vue / Vuex应用程序中起作用。
Vue是一个渐进式框架,在语法方面类似于Angular。 为了了解Vue中包含哪些组件以及Vuex出现在图片中的位置,首先,我们将介绍Vue如何提供在组件之间共享数据的能力。
什么是组件,为什么我们需要在组件之间共享数据?
如果您熟悉 Angular指令 ,就像一个简单的指令,我们可以编写自己的逻辑,提供模式(模板)并调用该组件(而不是将组件注册到根实例)。
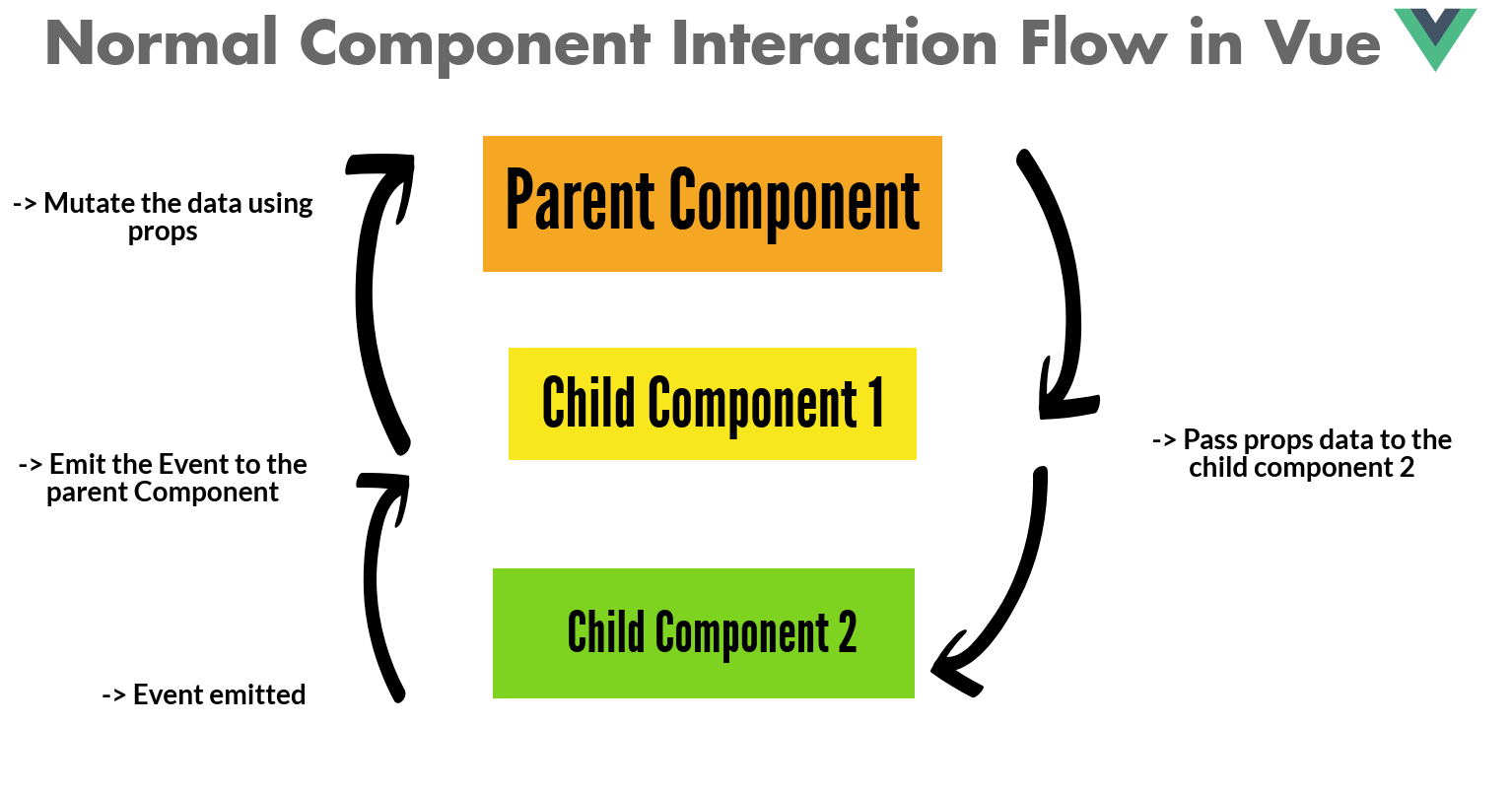
范例:
//Button directive component
Vue.component('button-directive', {
data: function () {
return {
counterValue: 0
}
}
template: ' < span > -Button Counter- </ span > < button v-on:click = "counterValue++" > {{ count } } </ button > '
});
< div id = "directive" >
< button-directive > </ button-directive >
</ div >
在上面的示例中, 按钮指令是一个组件,其中包括用于增加按钮计数器值的逻辑以及将实际呈现按钮的模板。 在顶层声明时,这些组件可以与其他组件共享。 要了解有关组件的更多信息,请访问Vue的组件文档。
上面的示例显示了一个组件,但是如果有多个组件且父组件和子组件彼此共享同一实例,该怎么办? 组件(子组件和父组件)之间的共享使用各种技术实现。
1.道具和活动
道具使您可以将任何数据类型传递给子组件,并允许您控制组件接收的数据类型。 这样,只要父级数据发生更改,子级组件就可以更新。 有关更多信息,请访问Vue的道具文档。 事件是将更新的信息从子组件传递到父组件的一种方式。 事件提供了一种方式来通知您的父母组件孩子的变化。
例:
<my-component @ eventExample =" parentHandler "></ my - component >
在这里,我的组件是带有eventExample的子组件,它将触发任何更改(内部使用-v-on:eventExample )。
可以使用类似的格式触发事件 :
export default {
methods : {
fireEvent() {
this .$emit( 'eventExample' , eventValueOne);
}
}
}
prop和event均可与v-modal一起用于双向绑定,其中输入值的更改将调用triggerEvent方法。
例:
<template>< div >
<input type="text" :value="value" @input="triggerEvent"/>
</div>
</ template >
<script>
export default {
props : {
value : String
},
methods : {
triggerEvent(event) {
this .$emit( 'input' , event.target.value);
}
}
}
< /script>
2. 提供/注入
这允许将数据或方法从祖先组件选择性地展示给其所有后代。 尽管provide / inject本身不是React性的,但可以用来传递React性对象。
示例:考虑两个组件,并且这两个组件共享。
const Provider = {
provide: {
foo:'bar'
},
template: ` <div> <slot> <slot> </div>`
}
const Child = {
injec t: [ 'foo' ],
template: ` <div> {{ foo }} injected. <slot> <slot> </div>`,
}
new Vue({
e l: '#app' ,
component s: {
Child,
Provider
}
})
Vuex出现在哪里?
如上所述,我们可以在两个组件之间共享实例,或者说,在三个组件之间共享实例。 当一个人进入一个组件在两个或三个或更多组件之间共享时,就会出现问题。 假设有一个大型应用程序,其中包含数百个组件,而一个组件中的一个prop需要在50个其他组件之间共享; 维护该组件的状态不是一个有问题的问题吗? 为了解决这个问题,Vuex会维护状态,并且使用突变处理状态中的任何更改,并且可以使用提交来调用该突变。
简而言之,Vuex会被动地更新正在从存储中读取数据的所有组件。
- 动作 -状态收到允许值以更改状态的位置。
- 突变 -实际状态发生变化的地方。
考虑以下示例。
注意:为了初始化存储,我正在创建Vues.Store的新实例。
/* Store where thestate is initialized and the values are mutated depending upon the values */
var state Manager = new Vuex.Store {
state : {
state1: '', // Initialize the state1
state2: '' // Initialize the state2
}
actions:{
// API's can be called here and then accordingly can be passed for mutation
},
mutations: {
changeStateOne( state , payload) {
state .state1 += payload; // Recieved the payload from the action at the bottom
},
changeStateTwo( state , payload) {
state .state2 += payload; // Recieved the payload from the action at the bottom.
}
}
}
// Component one
const componentOne = {
template: ` <div class="componentOne"> {{ state ManagerComponent }}</div>`,
computed: {
state ManagerComponent() {
return {
state Manager. state .state1
state Manager. state .state2
}
}
}
}
// Component Two
const componentTwo = {
template: ` <div class="componentTwo"> {{ stastateManagerComponentteManagerComponent }}</div>`,
computed: {
state ManagerComponent() {
return {
state Manager. state .state1
state Manager. state .state2
}
}
}
}
new Vue({
el: ' #app-container',
components: {
componentOne,
componentTwo
}
methods: {
increaseStatesValue() {
state Manager.commit('changeStateOne', 1 ); // Action with value one that is sent to the store
state Manager.commit('changeStateTwo', 2 ); // Action with value two that is sent to the store
}
}
})
// Thus resulting to a shared state between two components.
<div id="app-container">
<div class="componentOne"> {{ state ManagerComponent }}</div>
<div class="componentTwo"> {{ state ManagerComponent }}</div>
</div>
在上面的示例中,我们看到两个组件彼此共享相同的状态,其中,这些组件彼此共享相同的状态,并且通过一个存储将值附加到两个组件中(在上面的示例中是stateManager ,国家的商店)。
关于动作的注意事项:在上面的示例中,该动作是gainStatesValue ()函数,该函数为突变部分的changeStateOne和changeStateTwo函数提供有效负载。
Vuex的上述示例可以映射到下图中。
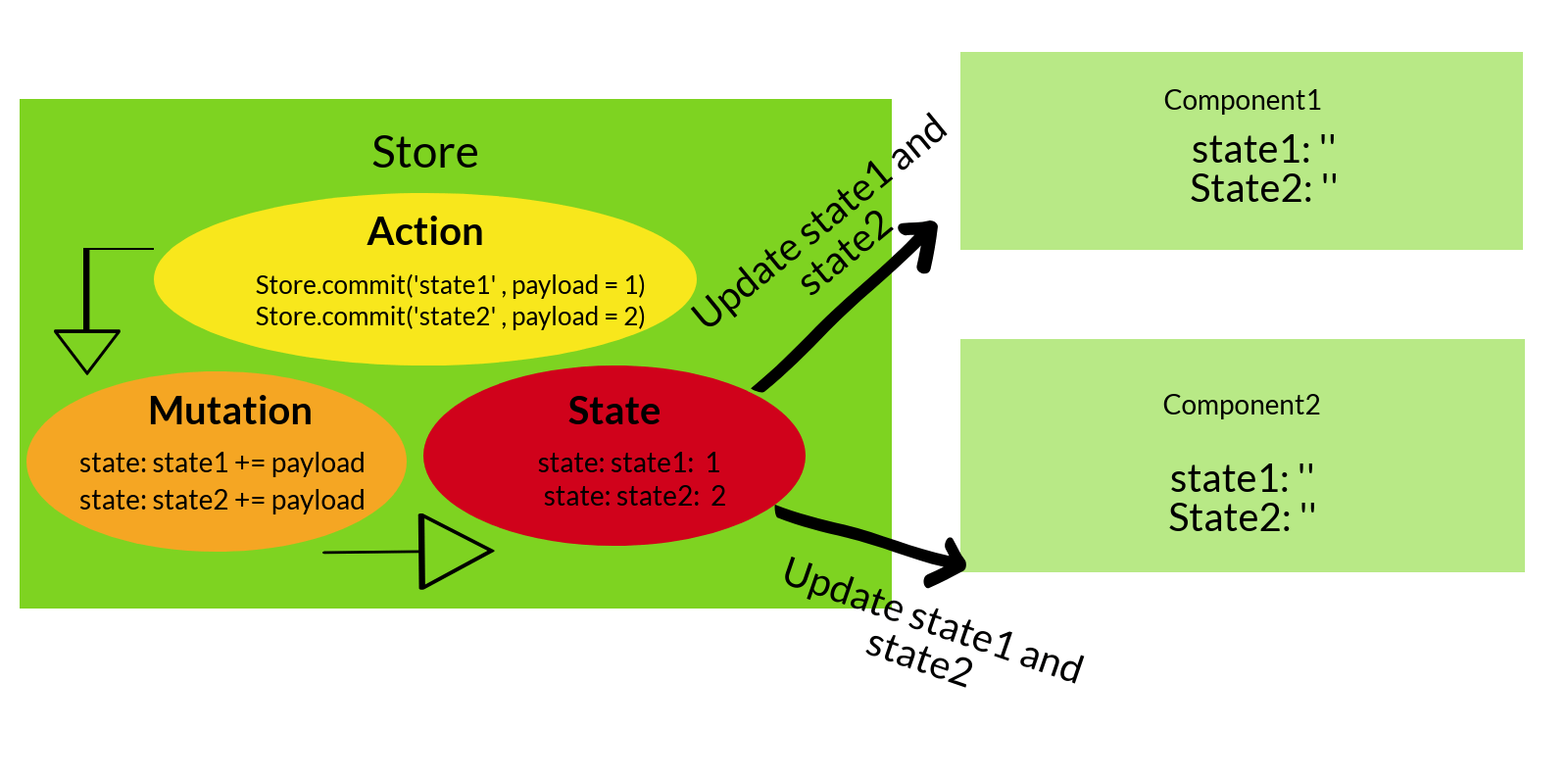
在架构方面,Vuex使用磁通作为其方法。 要了解有关通量的更多信息,请查看通量模式的文档 。
我希望本文能提供一些关于Vuex为什么在Vue应用程序中使用的想法,以及它如何在两个组件之间提供流畅的通信并促进状态之间的值更改容易。
如果您在任何类型的开发项目中需要帮助,我也可以为您提供有关项目的咨询。 我是最受好评的自由职业者。 您可以直接在 Upwork 上雇用我 。 你也可以 雇用我 自由职业者 。 如果您有任何评论,问题或建议,请随时在下面的评论部分中发布它们!
翻译自: https://hackernoon.com/components-and-how-they-interact-in-vue-and-vuex-35s32q8
vue中vuex组件通信