在models.py中创建数据库表
from django.db import models # Create your models here. class Book(models.Model): name = models.CharField(max_length=20) price = models.IntegerField pub_date = models.DateField
在terminal中 :python manage.py makemigrations
返回结果:No changes detected
创建失败。
原因可能如下:
- migration folder You need a migrations package in your app.
- INSTALLED_APPS You need your app to be specified in the INSTALLED_APPS .dict
- Verbosity start by running makemigrations -v 3 for verbosity. This might shed some light on the problem.
- Full path In INSTALLED_APPS it is recommended to specify the full module app config path 'apply.apps.MyAppConfig'
- --settings you might want to make sure the correct settings file is set: manage.py makemigrations --settings mysite.settings
- specify app name explicitly put the app name in manage.py makemigrations myapp- that narrows down the migrations for the app alone and helps you isolate the problem.
- model meta check you have the right app_label in your model meta
- Debug django debug django core script. makemigrations command is pretty much straight forward. Here's how to do it in pycharm. change your script definition accordingly (ex: makemigrations --traceback myapp)
而我的情况是第二种 :setting.py内的INSTALLED_APPS未添加 app名字


INSTALLED_APPS = [ 'django.contrib.admin', 'django.contrib.auth', 'django.contrib.contenttypes', 'django.contrib.sessions', 'django.contrib.messages', 'django.contrib.staticfiles', 'app1', ]
添加上后,创建成功
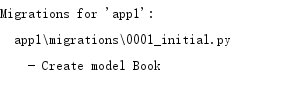