1. Identifying the Syntax of CSS 认识CSS语法
selector
{
//Declaration
Block
property:
value;
}
<!DOCTYPE HTML>
<HTML>
<BODY>
<H1>Welcome to BookYourHotel website.</H1>
<H2>This site is very user friendly.</H2>
<H1>You can book your hotel while sitting at home.</H1>
</BODY>
</HTML>
Now, you want to display the text inside the <H1> tag in italics and red color. In addition, the text inside the<H2>tag should be displayed as bold. You can perform this task by defining CSS rules using the following code snippet:
h1 {color:red; font-style:italic;}
h2 {font-weight:bold;}
Example:
<!DOCTYPE HTML>
<HTML>
<head>
<title>This is CSS</title>
<style>
h1{
color:red;
font-style:italic;
}
h2 {
font-weight:bold;
}
</style>
</head>
<BODY>
<H1>Welcome to Genshin Impact website.</H1>
<H2>There are many dog users.</H2>
<H1>You can use this website to get yuan_stone.</H1>
</BODY>
</HTML>
2. Identifying the Types of Style Sheets认识样式表的类型
a. Inline style 内联样式
<!DOCTYPE HTML>
<HTML>
<BODY>
<P style="font-size: 24pt; color: red">Hotel booking from the comfort of your room.</P>
<P>Compare and book from more than 5000 hotels. </P>
</BODY>
</HTML>
b. Internal style sheet 内部样式表
<!DOCTYPE HTML>
<HTML>
<HEAD>
<STYLE type="text/css">
p
{
color:red;
font-size:20pt;
font-style:italic;
}
</STYLE>
</HEAD>
<BODY>
<P> Hotel booking from the comfort of your room.</P>
<P> Compare and book from more than 5000 hotels.</P>
</BODY>
</HTML>
c. External style sheet 外部样式表
<LINK type="text/css" rel="stylesheet" href="externalstylesheet.css" />
<!DOCTYPE HTML>
<HTML>
<HEAD>
<TITLE> An External Style Sheet </TITLE>
<LINK type="text/css" rel="stylesheet" href="externalstyle.css" />
</HEAD>
<BODY>
<H1> Hotel booking from the comfort of your room. </H1>
<P> Compare and book from more than 5000 hotels. </P>
</BODY>
</HTML>
p
{
color:red;
font-size:20pt;
font-style:italic;
}
h1
{
color:blue;
font-size:25pt;
font-weight:bold;
}
3. Applying Multiple Style Sheets 使用多个样式表
p{
color:blue;
font-size:12pt;
font-weight:bold;
}
Consider the following code to associate multiple style sheets with the HTML page:
<!DOCTYPE HTML>
<HTML>
<HEAD>
<LINK type="text/css" rel="stylesheet" href="externalstylesheet.css" />
<STYLE>
h1{
color:red;
font-size:12pt;
font-style:italic;
}
</STYLE>
</HEAD>
<BODY>
<P>Welcome to BookYourHotel website.</P>
<H1 style="font-size: 24pt; color: green"> Hotel booking from the
comfort of your room.</H1>
<H1> Compare and book from more than 5000 hotels. </H1></BODY>
</HTML>
In the preceding code, the first heading will appear in green with font size, 24, since the inline style defined for the first <H1> tag will override the internal style defined in the <STYLE> tag. However, the second heading will appear in italics, red color with font-size, 12, according to the internal style defined for the <H1> tag. The paragraph will be styled according to the external style sheet as no internal or inline style has been applied on it.
4. Identifying CSS Selectors 认识 CSS 选择器
a. Implementing the ID Selector 实现 ID 选择器
<!DOCTYPE HTML>
<HTML>
<HEAD>
<STYLE>
p
{
color:red;
}
#pname
{
color:green; font-size=20;
font-weight:bold;
}
</STYLE>
</HEAD>
<BODY>
<P ID="pname">Welcome to BookYourHotel Website.</P>
<P> Hotel booking facility at your doorstep.</P>
<p>this is one the best hotel</p>
</BODY>
<HTML>
b. Implementing the Class Selector 实现类选择器
<!DOCTYPE HTML>
<HTML>
<HEAD>
<STYLE>
. color1 { color:blue; }
. color2 { color:red; }
</STYLE>
</HEAD>
<BODY>
<H4 class="color1"> Welcome to BookYourHotel website.</H4>
<P class="color1">Provides online booking of domestic and international hotels.</P>
<P class="color2"> Avail great discounts and offers. </P>
</BODY>
</HTML>
5.Styling HTML Elements 设置 HTML 元素样式
<!DOCTYPE HTML>
<HTML>
<HEAD>
</HEAD>
<BODY>
<H1>About Us</H1><HR>
<P>BookYourHotel is the most trusted name in the destination management
industry. We are one of the pioneers for hotel booking. Currently we
are operating out of our head office in Chicago. In addition, we have
our presence across all the major cities in the US and Europe. Our
primary focus is on customer satisfaction and comfort.</P>
<P>With a manpower strength of over 400 employees spread across the US,
we ensure a quick and customized response to all your travel related
queries.</P>
<P>Our regional offices are situated at the following locations:
<UL>
<LI>Alabama</LI>
<LI>Florida</LI>
<LI>California</LI>
<LI>Colorado</LI>
<LI>Texas</LI>
<LI>New York</LI>
</UL>
<P>We are also planning to expand our services to other states.</P>
<P>Regarding any further queries or feedback, click at the
following link:</P> <A href="contactus.html">BookYourHotel</A>
</BODY>
</HTML>
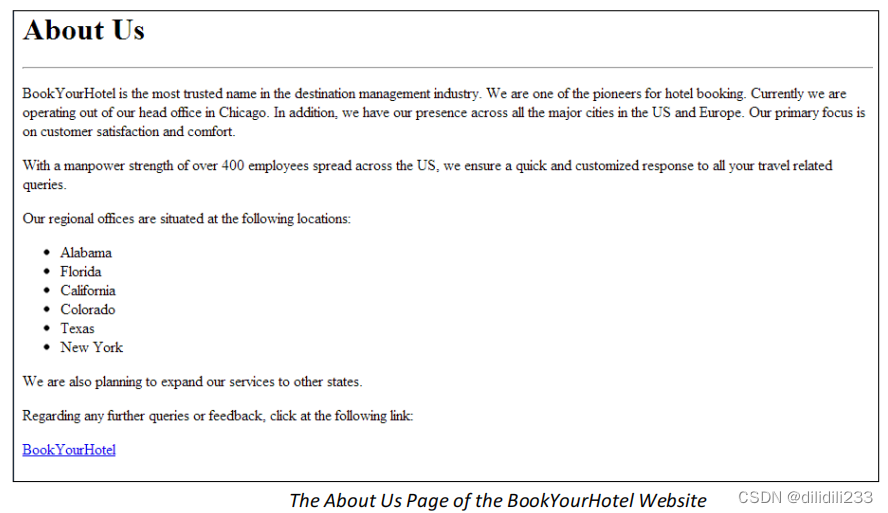
//The text in the paragraphs should have the font family as Helvetica and the font size as 20.
//段落中的文本的字体为 Helvetica,字体大小应为 20。
p{
font-family: "Helvetica"; Font-size:20px;
}
//The Heading level one should appear in small caps, it aligns the heading in red color and in centerand in Italic form.
//标题级别 1 应以小写字母显示,它以红色和中间和斜体形式对齐标题。
h1{
font-variant:small-caps;
font-style:italic;
}
h1{
Color: red;
text-align: center;
}
//The background should appear in lavender color.
//背景应以 lavender color 显示。
<STYLE>
body{
background-color:lavender;
}
</STYLE>
//The space between letters in a paragraph should be 2 points. Also, the line height should be 12 points.
//段落中字母之间的间距应为 2 磅。此外,行高应为 12 磅。
p{
letter-spacing:2pt; line-height:12pt;
}
//The symbol of the list bullets should appear as a square.
//列表项目符号的符号应显示为正方形。
ul{
list-style-type:square;
list-style-position: inside;
}
6.The CSS Link properties CSS 链接属性
<STYLE>
a:link {
color:#FF0000;
}
a:visited {
color:#00FF00;
}
a: hover {
color: #0000FF;
}
a: active {
color: #FF00FF;
text-decoration:underline;
}
</STYLE>
7. Grouping and Nesting Styles 分组和嵌套样式
Grouping of Styles 样式分组
<!DOCTYPE HTML>
<HTML>
<HEAD>
<STYLE>
h1{
text-align:center;
}
</STYLE>
</HEAD>
<BODY>
<H1>BookYourHotel</H1>
<UL>
<LI><A href="aboutus.html">About Us</A></LI>
<LI><A href="rooms.html">Rooms</A></LI>
<LI><A href="facilities.hmtl">Facilities</A></LI>
<LI><A href="contactus.html">Contact Us</A></LI>
</UL>
<HR>
<H3> Welcome to the Home page</H3>
<P> BookYourHotel welcomes you with warmth and a feeling that each guest is truly special. It offers acozy and intimate experience amidst the glitz and glamour of South America. Our caring and courteous staffs are ever eager to ensure that all individual needs are cared for with professional expertise and a personal touch.
</P>
<P>The rooms have been designed with different floor plans to create individual spaces. We have Deluxe Rooms, Double and Single Rooms each with ensuite Bathrooms fitted with all modern conveniences. All room has Air- Conditioning and is equipped with well stocked Mini-bars, Television with Cable T.V. Conference facilities available for approximately 40 persons. Our multi-cuisine restaurant offers, a plethora of tasty local and international dishes to satisfy all palates. This with the elegant ambience makesfor a truly unique experience. </P>
</BODY>
</HTML>
Grouping Styles 分组样式
selector1, selector2
{
property:value;
}
h1,p
{
color:red;
}
Nesting of Styles 嵌套样式
li a{
color:red;
text-decoration:none;
}
You can apply various nesting styles on HTML elements. The following table lists the nesting styles.
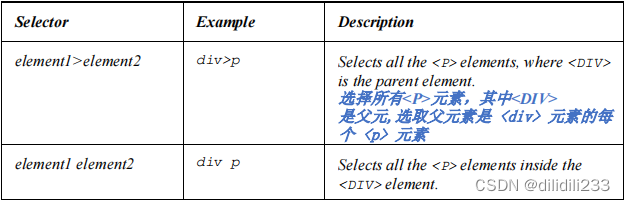
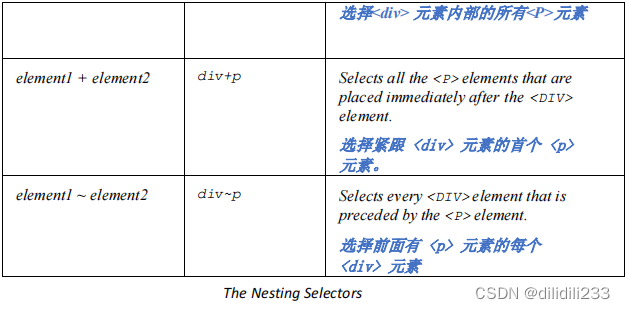
<!DOCTYPE HTML>
<HTML>
<HEAD>
<STYLE>
div+p
{
background-color:blue;
}
</STYLE>
</HEAD>
<BODY>
<H1>Welcome to the page.</H1>
<DIV>
<P>This is paragraph 1.</P>
</DIV>
<P>This is paragraph 2.</P>
<P>This is paragraph 3.</P>
</BODY>
</HTML>
In the preceding code, only the <P> tag that is placed immediately after the <DIV> tag gets stylized
8. Controlling the Visibility of Elements 控制元素的可见性
Display
li{
display: inline;
list-style-type:none;
}
In the preceding code snippet, links are horizontally displayed, as shown in the following figure.
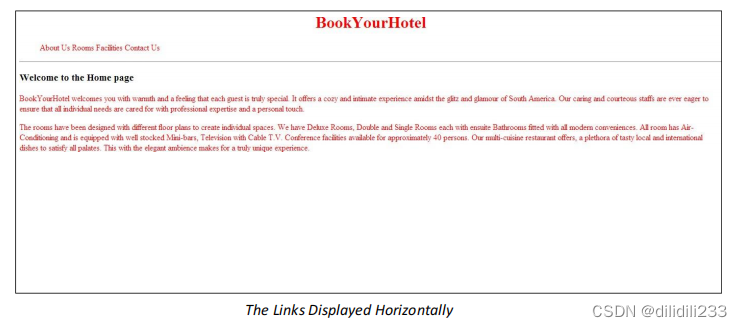
Visibility可见度
<!DOCTYPE HTML>
<HTML>
<HEAD>
<STYLE>
h2{
visibility:hidden;
}
</STYLE>
</HEAD>
<BODY>
<H1>Welcome to BookYourHotel website. </H1>
<H2>This site is very user friendly.</H2>
<H3>You can book your hotel while sitting at home.</H3>
</BODY>
</HTML>
见性属性的输出,如下图所示。
9.Positioning HTML Elements 定位 HTML 元素
Position 位置
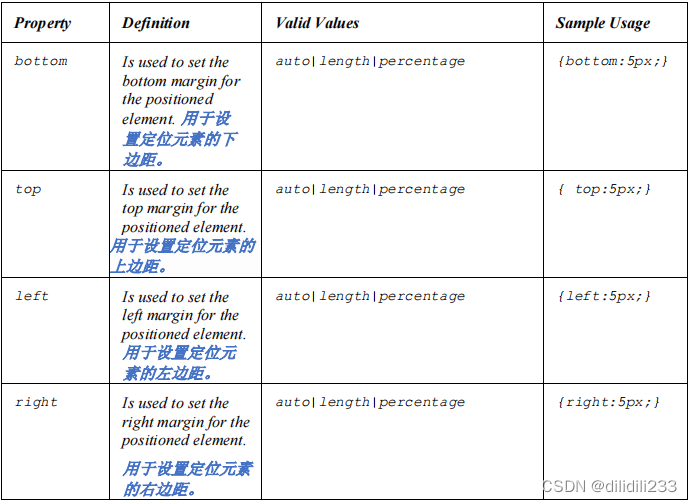
h3{
position: absolute;
top:0px;
}
Source Code
<!DOCTYPE HTML>
<HTML>
<HEAD>
<STYLE>
h1{
text-align:center;
}
h3{
position: absolute;
top:0px;
}
li{
display: inline;
list-style-type:none;
}
li a{
color:red;
text-decoration:none;
}
h1,p{
color:red;
}
</STYLE>
</HEAD>
<BODY>
<H1>BookYourHotel</H1>
<UL>
<LI><A href="aboutus.html">About Us</A></LI>
<LI><A href="rooms.html">Rooms</A></LI>
<LI><A href="facilities.hmtl">Facilities</A></LI>
<LI><A href="contactus.html">Contact Us</A></LI>
</UL>
<HR>
<H3> Welcome to the Home page</H3>
<P> BookYourHotel welcomes you with warmth and a feeling that each guest is truly special. It offers a cozy and intimate experience amidst the glitz and glamour of South America. Our caring and courteous staffs are ever eager to
ensure that all individual needs are cared for with professional expertise and
a personal touch.</P>
<P>The rooms have been designed with different floor plans to create
individual spaces. We have Deluxe Rooms, Double and Single Rooms each with
ensuite Bathrooms fitted with all modern conveniences. All room has AirConditioning and is equipped with well stocked Mini-bars, Television with
Cable T.V. Conference facilities available for approximately 40 persons. Our
multi-cuisine restaurant offers, a plethora of tasty local and international
dishes to satisfy all palates. This with the elegant ambience makes for a
truly unique experience.</P>
</BODY>
</HTML>
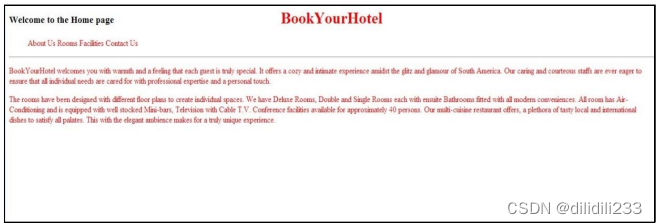
Float 浮动
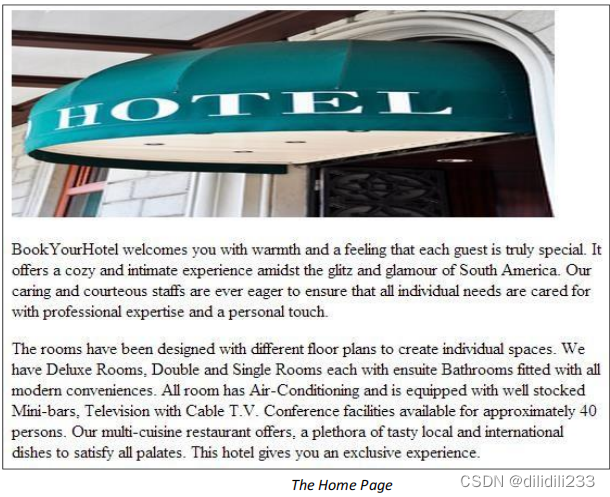
<!DOCTYPE HTML>
<HTML>
<HEAD>
<STYLE>
img {
float: left;
}
</STYLE>
</HEAD>
<BODY>
<IMG src= "dep_6777368-Hotel-entrance.jpg" width=500 height=200>
<P> BookYourHotel welcomes you with warmth and a feeling that each guest is truly special. It offers a cozy and intimate experience amidst the glitz and glamour of South America. Our caring and courteous staffs are ever eager to ensure that all individual needs are cared for with professional expertise and a personal touch.</P>
<P>The rooms have been designed with different floor plans to create individual spaces. We have Deluxe Rooms, Double and Single Rooms each with ensuite Bathrooms fitted with all modern conveniences. All room has Air- Conditioning and is equipped with well stocked Minibars, Television with Cable T.V. Conference facilities available for approximately 40 persons. Our multi-cuisine restaurant offers, a plethora of tasty local and international dishes to satisfy all palates. This hotel gives you an exclusive experience.</P>
</BODY>
</HTML>
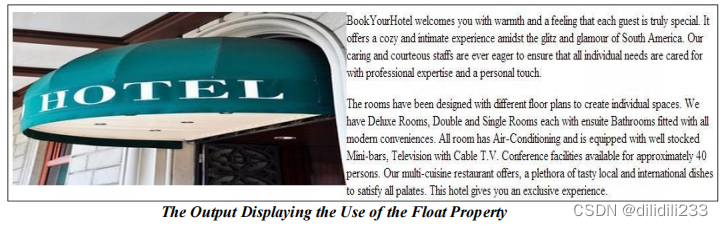
Clear
clear 属性:
<!DOCTYPE HTML>
<HTML>
<HEAD>
<STYLE>
img {
float: left;
}
.auto-style{
clear:both;
}
</STYLE>
</HEAD>
<BODY>
<IMG src= "dep_6777368-Hotel-entrance.jpg" width=500 height=200>
<P> BookYourHotel welcomes you with warmth and a feeling that each guest is truly special. It offers a cozy and intimate experience amidst the glitz and glamour of South America. Our caring and courteous staffs are ever eager to ensure that all individual needs are cared for with professional expertise and a personal
touch.</P>
<P class="auto-style">The rooms have been designed with different floor plans to create individual spaces. We have Deluxe Rooms, Double and Single Rooms each with ensuite Bathrooms fitted with all modern
conveniences. All room has Air-Conditioning and is equipped with well stocked Mini-bars, Television with Cable T.V. Conference facilities available for approximately 40 persons. Our multi-cuisine restaurant offers, a plethora of tasty local and international dishes to satisfy all palates. This hotel gives you an exclusive
experience.</P>
</BODY>
</HTML>
The preceding code displays a paragraph on the right side and another paragraph below the image on the Web page.