Java项目Maven配置操作Pdf
效果
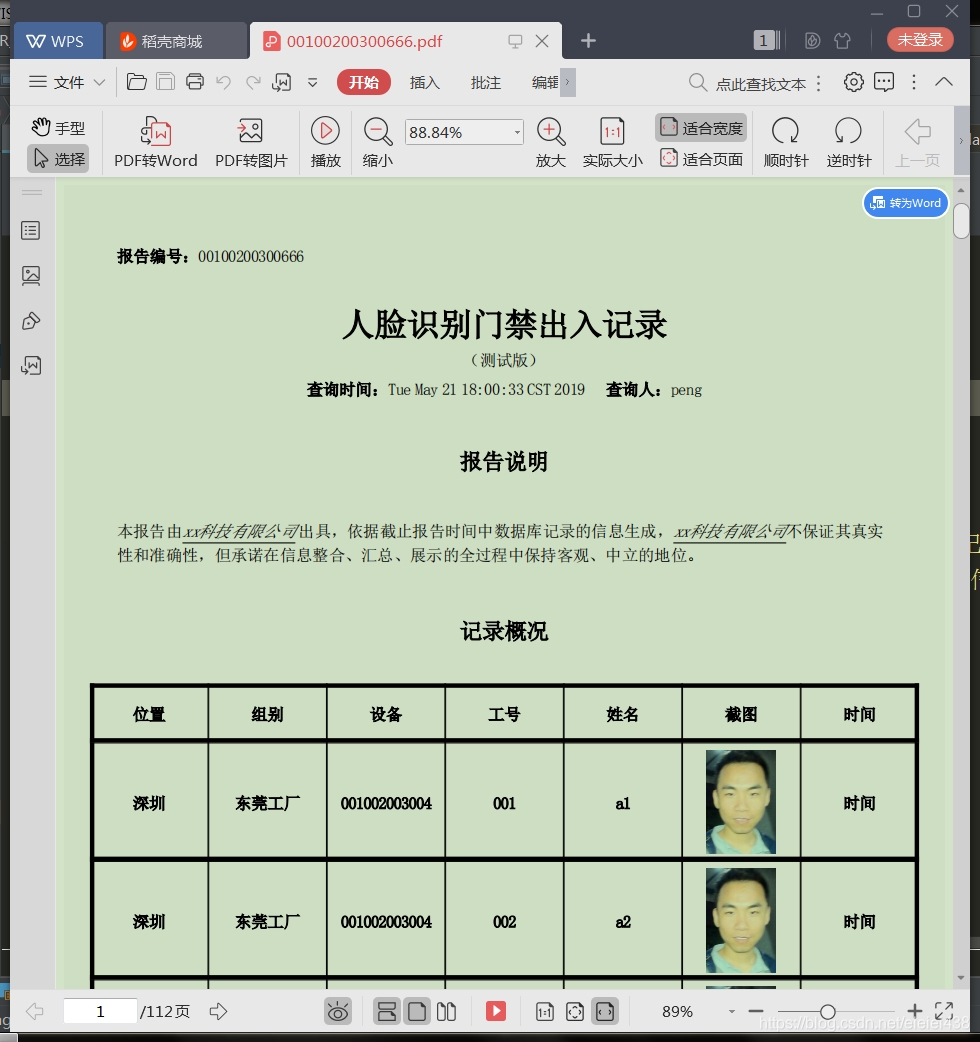
maven配置
- 【版本差异会出现STSong-Light' with 'UniGB-UCS2-H' is not recognized错误】
- 【下面的依赖配置解决了上述的问题】
<!-- pdf样式 -->
<dependency>
<groupId>com.itextpdf</groupId>
<artifactId>itext-asian</artifactId>
<version>5.2.0</version>
</dependency>
<!--pdf相关操作-->
<dependency>
<groupId>com.itextpdf</groupId>
<artifactId>itextpdf</artifactId>
<version>5.4.3</version>
</dependency>
工具类
public class DataUtil {
/**
* 分割路径
*/
public static String[] separatePath(String path) {
if (path == null || "".equals(path.trim())) {
return null;
}
String[] sep = path.split("\\.");
return new String[]{sep[0], sep[1]};
}
}
import com.itextpdf.text.*;
import com.itextpdf.text.pdf.*;
import com.visionin.framework.db.Util;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.util.Calendar;
public class PdfTempUtil {
public static void main(String[] args) throws Exception {
String toPath = "E://";
String pdfName = "00100200300666";//这里的名称随意
String suff_fix_name = ".pdf";
createPDF(toPath, pdfName, suff_fix_name);
}
/**
* 创建PDF文档
*/
public static String createPDF(String toPath, String pdfName, String suffFixName) throws Exception {
//输出路径
String outPath = toPath + pdfName + suffFixName;
//设置纸张
Rectangle rect = new Rectangle(PageSize.A4);
//创建文档实例
Document doc = new Document(rect);
//添加中文字体
BaseFont bfChinese = BaseFont.createFont("STSong-Light", "UniGB-UCS2-H", BaseFont.NOT_EMBEDDED);
//设置字体样式
Font textFont = new Font(bfChinese, 11, Font.NORMAL); //正常
Font redTextFont = new Font(bfChinese, 11, Font.NORMAL, BaseColor.RED); //正常,红色
Font boldFont = new Font(bfChinese, 11, Font.BOLD); //加粗
Font redBoldFont = new Font(bfChinese, 11, Font.BOLD, BaseColor.RED); //加粗,红色
Font firsetTitleFont = new Font(bfChinese, 22, Font.BOLD); //一级标题
Font secondTitleFont = new Font(bfChinese, 15, Font.BOLD); //二级标题
Font underlineFont = new Font(bfChinese, 11, Font.UNDERLINE); //下划线斜体
//创建输出流
PdfWriter.getInstance(doc, new FileOutputStream(new File(outPath)));
//打开文档
doc.open();
//创建新的一页
doc.newPage();
//段落
Paragraph p1 = new Paragraph();
//短语
Phrase ph1 = new Phrase();
//报告编号
Chunk c1 = new Chunk("报告编号:", boldFont);
Chunk c1_1 = new Chunk("" + pdfName, textFont);
ph1.add(c1);
ph1.add(c1_1);
p1.add(ph1);
doc.add(p1);
//报告标题
p1 = new Paragraph("人脸识别门禁出入记录", firsetTitleFont);
p1.setLeading(50);
p1.setAlignment(Element.ALIGN_CENTER);
doc.add(p1);
//报告版本
p1 = new Paragraph("(测试版)", textFont);
p1.setLeading(20);
p1.setAlignment(Element.ALIGN_CENTER);
doc.add(p1);
//报告时间和查询的账号
p1 = new Paragraph();
p1.setLeading(20);
p1.setAlignment(Element.ALIGN_CENTER);
ph1 = new Phrase();
Chunk c2 = new Chunk("查询时间:", boldFont);
Chunk c2_1 = new Chunk(Calendar.getInstance().getTime() + "", textFont);
Chunk c3 = new Chunk(leftPad("查询人:", 10), boldFont);
Chunk c3_1 = new Chunk("peng", textFont);
ph1.add(c2);
ph1.add(c2_1);
ph1.add(c3);
ph1.add(c3_1);
p1.add(ph1);
doc.add(p1);
//报告说明
p1 = new Paragraph("报告说明", secondTitleFont);
p1.setLeading(50);
p1.setAlignment(Element.ALIGN_CENTER);
doc.add(p1);
p1 = new Paragraph(" ");
p1.setLeading(30);
doc.add(p1);
p1 = new Paragraph();
ph1 = new Phrase();
Chunk c4 = new Chunk("本报告由", textFont);
Chunk c5 = new Chunk("XX科技有限公司", underlineFont);
c5.setSkew(0, 30);
Chunk c6 = new Chunk(" 出具,依据截止报告时间中数据库记录的信息生成,", textFont);
Chunk c7 = new Chunk("不保证其真实性和准确性,但承诺在信息整合、汇总、展示的全过程中保持客观、中立的地位。", textFont);
ph1.add(c4);
ph1.add(c5);
ph1.add(c6);
ph1.add(c5);
ph1.add(c7);
p1.add(ph1);
doc.add(p1);
//记录概况
p1 = new Paragraph("记录概况", secondTitleFont);
p1.setSpacingBefore(30);
p1.setSpacingAfter(30);
p1.setAlignment(Element.ALIGN_CENTER);
doc.add(p1);
// 创建一个有4列的表格
PdfPTable table = new PdfPTable(7);
table.setTotalWidth(new float[]{80, 80, 80, 80, 80, 80, 80}); //设置列宽
table.setLockedWidth(true); //锁定列宽
table = createCell(table, new String[]{"位置", "组别", "设备", "工号", "姓名", "截图", "时间"}, 1, 7, -1);
for (int i = 1; i < 1000; i++) {
table = createCell(table, new String[]{"深圳", "东莞工厂", "001002003004", "00" + i, "a" + i, "E://demo.jpg", "时间"}, 1, 7, 5);
}
doc.add(table);
doc.close();
return outPath;
}
/**
* 创建单元格
*/
private static PdfPTable createCell(PdfPTable table, String[] title, int row, int cols, int img_col_num) throws DocumentException, IOException {
//添加中文字体
BaseFont bfChinese = BaseFont.createFont("STSong-Light", "UniGB-UCS2-H", BaseFont.NOT_EMBEDDED);
Font font = new Font(bfChinese, 11, Font.BOLD);
for (int i = 0; i < row; i++) {
for (int j = 0; j < cols; j++) {
PdfPCell cell = new PdfPCell();
if (i == 0 && title != null) {//设置表头
if (img_col_num == j) {//添加图片列
Image img = Image.getInstance(title[img_col_num]);
img.setBorderWidth(0);
img.scaleToFit(50, 70);// 大小
cell = new PdfPCell(img);
cell.setPadding(5);
} else {
cell = new PdfPCell(new Phrase(title[j], font)); //这样表头才能居中
}
if (table.getRows().size() == 0) {
cell.setBorderWidthTop(3);
}
}
if (row == 1 && cols == 1) { //只有一行一列
cell.setBorderWidthTop(3);
}
if (j == 0) {//设置左边的边框宽度
cell.setBorderWidthLeft(3);
}
if (j == (cols - 1)) {//设置右边的边框宽度
cell.setBorderWidthRight(3);
}
if (i == (row - 1)) {//设置底部的边框宽度
cell.setBorderWidthBottom(3);
}
cell.setMinimumHeight(40); //设置单元格高度
cell.setUseAscender(true); //设置可以居中
cell.setHorizontalAlignment(PdfPCell.ALIGN_CENTER); //设置水平居中
cell.setVerticalAlignment(PdfPCell.ALIGN_MIDDLE); //设置垂直居中
table.addCell(cell);
}
}
return table;
}
/**
* 加水印(字符串)
*/
public static void stringWaterMark(String inputFile, String waterMarkName) {
try {
String[] spe = DataUtil.separatePath(inputFile);
String outputFile = spe[0] + "_WM." + spe[1];
PdfReader reader = new PdfReader(inputFile);
PdfStamper stamper = new PdfStamper(reader, new FileOutputStream(outputFile));
//添加中文字体
BaseFont bfChinese = BaseFont.createFont("STSong-Light", "UniGB-UCS2-H", BaseFont.NOT_EMBEDDED);
int total = reader.getNumberOfPages() + 1;
PdfContentByte under;
int j = waterMarkName.length();
char c = 0;
int rise = 0;
//给每一页加水印
for (int i = 1; i < total; i++) {
rise = 400;
under = stamper.getUnderContent(i);
under.beginText();
under.setFontAndSize(bfChinese, 30);
under.setTextMatrix(200, 120);
for (int k = 0; k < j; k++) {
under.setTextRise(rise);
c = waterMarkName.charAt(k);
under.showText(c + "");
}
// 添加水印文字
under.endText();
}
stamper.close();
} catch (Exception e) {
e.printStackTrace();
}
}
/**
* 加水印(图片)
*/
public static void imageWaterMark(String inputFile, String imageFile) {
try {
String[] spe = DataUtil.separatePath(inputFile);
String outputFile = spe[0] + "_WM." + spe[1];
PdfReader reader = new PdfReader(inputFile);
PdfStamper stamper = new PdfStamper(reader, new FileOutputStream(outputFile));
int total = reader.getNumberOfPages() + 1;
Image image = Image.getInstance(imageFile);
image.setAbsolutePosition(-100, 0);//坐标
image.scaleAbsolute(800, 1000);//自定义大小
//image.setRotation(-20);//旋转 弧度
//image.setRotationDegrees(-45);//旋转 角度
//image.scalePercent(50);//依照比例缩放
PdfGState gs = new PdfGState();
gs.setFillOpacity(0.2f);// 设置透明度为0.2
PdfContentByte under;
//给每一页加水印
for (int i = 1; i < total; i++) {
under = stamper.getUnderContent(i);
under.beginText();
// 添加水印图片
under.addImage(image);
under.setGState(gs);
}
stamper.close();
} catch (Exception e) {
e.printStackTrace();
}
}
/**
* 设置左边距
*/
public static String leftPad(String str, int i) {
int addSpaceNo = i - str.length();
String space = "";
for (int k = 0; k < addSpaceNo; k++) {
space = " " + space;
}
String result = space + str;
return result;
}
/**
* 设置间距
*/
public static String printBlank(int tmp) {
String space = "";
for (int m = 0; m < tmp; m++) {
space = space + " ";
}
return space;
}
}