Spring Boot 概述
Spring Boot 特点
SpringBoot 是由 pivotal 团队开发的全新的Spring 框架,其设计初衷是简化 Spring 应用复杂的搭建和开发过程。该框架提供了一套简单的 Spring 模块依赖和管理工具,从而避免了开发人员处理复杂的模块依赖和版本冲突问题,同事提供打包即可用 Web 服务。
SpringBoot 特点如下:
- 快速创建独立的 Spring 应用程序
- 嵌入 Tomcat Web 容器,可快速部署
- 自动配置 JAR 包依赖和版本控制,简化 Maven 配置
- 自动装配 Spring 实例,不需要 XML 配置
- 提供诸如性能指标、健康检查、外部配置等线上监控和配置功能。
Spring Boot Application Starters
Starters 是一组资源依赖描述,用于为不同的 SpringBoot 应用提供一站式服务,而不必像传统的 Spring 项目那样,需要开发人员处理服务和服务之间的复杂依赖关系。例如,如果需要开发 REST 服务或 Web 应用程序时,只需只需添加一个 spring-boot-starter-web 依赖就可以了,具体的依赖细节由 Starters 统一处理,不需要应用程序分别处理各个 Jar 包之间的依赖关系。
<!-- 引入 spring-boot-starter-web 依赖 -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
Spring Boot 支持的内嵌 Servlet 容器
Spring Boot 支持的内嵌 Servlet 容器有:
- Tomcat 9.0
- Jetty 9.4
- Undertow 2.0
Spring Boot ( spring-boot-starter-web) 使用 Tomcat 作为默认的嵌入式 Servlet 容器,如果需要使用 Jetty 的话需要修改 pom.xml (Maven) 或者 build.gradle (Gradle) 文件配置。
<!-- 修改 pom.xml 文件配置 -->
<!--从Web启动器依赖中排除Tomcat-->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
<exclusions>
<exclusion>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-tomcat</artifactId>
</exclusion>
</exclusions>
</dependency>
## 标题
<!--添加Jetty依赖-->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-jetty</artifactId>
</dependency>
// 修改 build.gradle 文件配置(看上去更加简洁)
compile("org.springframework.boot:spring-boot-starter-web") {
exclude group: 'org.springframework.boot', module: 'spring-boot-starter-tomcat'
}
compile("org.springframework.boot:spring-boot-starter-jetty")
2_配置文件
配置文件
YAML 文件
我们可以通过 application.properties / application.yml 对 Spring Boot 程序进行简单的配置。
YAML 是 “YAML Ain’t a Markup Language”(YAML 不是一种标记语言)的递归缩写,是一种人类可读的数据序列化语言。相比于 properties 文件,YAML 配置的方式更加直观清晰,简介明了,有层次感。
properties 配置方式:
server.port=8330
spring.dubbo.applicaiton.name=dubbo-consumer
spring.dubbo.applicaiton.registry=zookeeper:127.0.0.1:2181
yaml 配置方式:
server:
prot:8330
spring:
dubbo:
application:
name:dubbo-consumer
registry:zookeeper:127.0.0.1:2181
但是 YAML 配置的方式有一个缺点,那就是不支持 @PropertySource 注解导入自定义的 YAML 配置。
读取配置文件
@Value 方式
@Value("${xxx}")
String xxx;
根据传入的 xxx 获取相应的值,@Value 方式一般同于读取比较简单的配置信息,但是并不建议使用。
@ConfigurationProperties 方式
定义一个 LibraryProperties 类:
import lombok.Getter;
import lombok.Setter;
import lombok.ToString;
import org.springframework.boot.context.properties.ConfigurationProperties;
import org.springframework.context.annotation.Configuration;
import org.springframework.stereotype.Component;
import java.util.List;
// 使用 @Component 注解声明,可以像使用普通 Bean 一样将其注入到类中使用。
@Component
@ConfigurationProperties(prefix = "library")
@Setter
@Getter
@ToString
class LibraryProperties {
private String location;
private List<Book> books;
@Setter
@Getter
@ToString
static class Book {
String name;
String description;
}
}
package cn.javaguide.readconfigproperties;
import org.springframework.beans.factory.InitializingBean;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class ReadConfigPropertiesApplication implements InitializingBean {
// 注入使用
private final LibraryProperties library;
public ReadConfigPropertiesApplication(LibraryProperties library) {
this.library = library;
}
public static void main(String[] args) {
SpringApplication.run(ReadConfigPropertiesApplication.class, args);
}
@Override
public void afterPropertiesSet() {
System.out.println(library.getLocation());
System.out.println(library.getBooks());
}
}
@ConfigurationProperties 方式
@ConfigurationProperties 方式不仅可以读取数据,还可以对读取的数据进行校验。
user:
name: xxx
email: 123455@
import lombok.Getter;
import lombok.Setter;
import lombok.ToString;
import org.springframework.boot.context.properties.ConfigurationProperties;
import org.springframework.stereotype.Component;
import org.springframework.validation.annotation.Validated;
import javax.validation.constraints.Email;
import javax.validation.constraints.NotEmpty;
// 注意:这里 ProfileProperties 类没有加 @Component 注解。
@Getter
@Setter
@ToString
@ConfigurationProperties("user")
@Validated
public class ProfileProperties {
@NotEmpty
private String name;
@Email
@NotEmpty
private String email;
// 配置文件中没有读取到的话就用默认值
private Boolean handsome = Boolean.TRUE;
}
import org.springframework.beans.factory.InitializingBean;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.boot.context.properties.EnableConfigurationProperties;
@SpringBootApplication
// 使用 @EnableConfigurationProperties 注册我们的配置 Bean。
@EnableConfigurationProperties(ProfileProperties.class)
public class ReadConfigPropertiesApplication implements InitializingBean {
private final ProfileProperties profileProperties;
public ReadConfigPropertiesApplication(ProfileProperties profileProperties) {
this.profileProperties = profileProperties;
}
public static void main(String[] args) {
SpringApplication.run(ReadConfigPropertiesApplication.class, args);
}
@Override
public void afterPropertiesSet() {
System.out.println(profileProperties.toString());
}
}
邮箱格式不正确,所以程序运行的时候就报错,也就保证了数据类型的安全性。
@PropertySource 方式
import lombok.Getter;
import lombok.Setter;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.context.annotation.PropertySource;
import org.springframework.stereotype.Component;
@Component
// @PropertySource 注解方式读取指定的 .properties 文件
@PropertySource("classpath:website.properties")
@Getter
@Setter
class WebSite {
@Value("${url}")
private String url;
}
使用:
@Autowired
private WebSite webSite;
System.out.println(webSite.getUrl());
Spring Boot 加载配置文件的优先级
Spring 读取配置文件也是有优先级的,其中:
- 项目目录下的 config 目录下的 applicaiton.yml 的优先级最高;
- resources 目录下的 config 目录下的 application.yml 的优先级次之;
- resources 目录下的 application.yml 优先级最低。
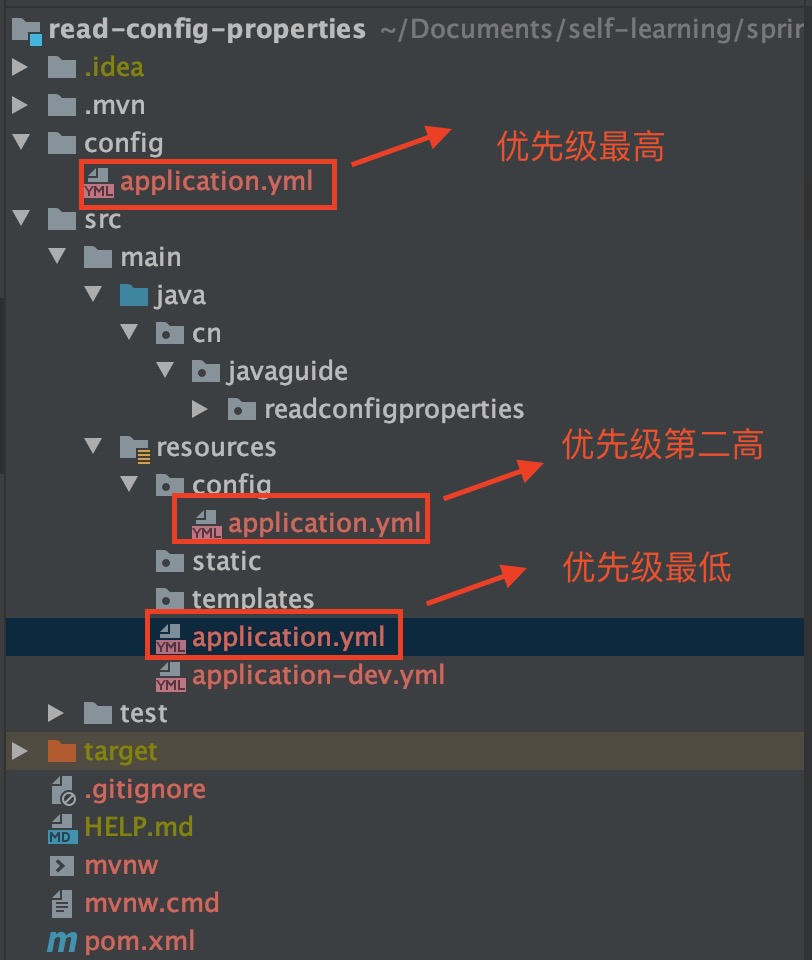
3_常用注解
常用注解
@SpringBootApplication
Spring Boot 项目的基石,创建 Spring Boot 项目之后会默认在主类加上。
@SpringBootApplication 可以认为是 @Configuration 、@EnableAutoConfiguration 和 @ComponentScan 注解的集合。这三个注解的具体作用如下:
- @EnableAutoConfiguration:启用 SpringBoot 的自动配置机制
- @ComponentScan: 扫描被 @Component / @Service / @Controller 注解的 Bean,注解默认会扫描该类所在的包下所有的类。
- @Configuration:允许在 Spring 上下文中注册额外的 Bean 或导入其他配置类
@EnableAutoConfiguration 注解是启动自动配置的关键,其源码如下:
import java.lang.annotation.Documented;
import java.lang.annotation.ElementType;
import java.lang.annotation.Inherited;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
import org.springframework.context.annotation.Import;
@Target({ElementType.TYPE})
@Retention(RetentionPolicy.RUNTIME)
@Documented
@Inherited
@AutoConfigurationPackage
@Import({AutoConfigurationImportSelector.class})
public @interface EnableAutoConfiguration {
String ENABLED_OVERRIDE_PROPERTY = "spring.boot.enableautoconfiguration";
Class<?>[] exclude() default {};
String[] excludeName() default {};
}
@EnableAutoConfiguration 注解通过 Spring 提供的 @Import 注解导入 AutoConfigurationImportSelector 类。AutoConfigurationImportSelector 类中 getCandidateConfigurations 方法会将所有自动配置类的信息以 List 的形式返回,这些配置信息会被 Spring 容器当作 Bean 来进行管理。
protected List<String> getCandidateConfigurations(AnnotationMetadata metadata, AnnotationAttributes attributes) {
List<String> configurations = SpringFactoriesLoader.loadFactoryNames(getSpringFactoriesLoaderFactoryClass(),
getBeanClassLoader());
Assert.notEmpty(configurations, "No auto configuration classes found in META-INF/spring.factories. If you "
+ "are using a custom packaging, make sure that file is correct.");
return configurations;
}
Spring Bean 相关注解
-
Autowired
自动导入对象到类中,被注入进的类同样要被 Spring 容器管理。
-
@RestController
@RestController 注解是 @Controller和 @ResponseBody 的合集,表示这是个控制器 Bean,并且是将函数的返回值直 接填入 HTTP 响应体中,是 REST 风格的控制器。
-
@Component
可标注任意类为 Spring 组件。如果一个 Bean 不知道属于哪个层,可以使用 @Component 注解标注。
-
@Repository
对应持久层即 Dao 层,主要用于数据库相关操作。
-
@Service
对应服务层,主要涉及一些复杂的逻辑,需要用到 Dao 层。
-
@Controller
对应 Spring MVC 控制层,主要用于接受用户请求并调用 Service 层返回数据给前端页面。
处理常见的 HTTP 请求类型
- @GetMapping:GET 请求
- @PostMapping:POST 请求
- @PutMapping:PUT 请求
- @DeleteMapping:DELETE 请求
前后端传值
-
@RequestParam & @Pathvairable
@PathVariable用于获取路径参数;
@RequestParam用于获取查询参数。
-
@RequestBody
用于读取 request 请求(可能是 POST、PUT、DELETE、GET 请求)的 Body 部分并且 Content-Type 为 application/json 格式的数据,接收到数据之后会自动将数据绑定到 Java 对象上去。系统会使用HttpMessageConverter 或者自定义的 HttpMessageConverter 将请求的 Body 中的 json 字符串转换为 java 对象。
4_请求参数校验
请求参数校验
我们需要对传入后端的数据再进行一遍校验,避免用户绕过浏览器直接通过一些 HTTP 工具直接向后端请求一些违法数据。Spring Boot 程序做请求参数校验,需要引入 spring-boot-starter-web 依赖。
常用校验注解
JSR 校验注解
-
@Null
被注释的元素必须为 null
-
@NotNull
被注释的元素必须不为 null
-
@AssertTrue
被注释的元素必须为 true
-
@AssertFalse
被注释的元素必须为 false
-
@Min(value)
被注释的元素必须是一个数字,其值必须大于等于指定的最小值
-
@Max(value)
被注释的元素必须是一个数字,其值必须小于等于指定的最大值
-
@DecimalMin(value)
被注释的元素必须是一个数字,其值必须大于等于指定的最小值
-
@DecimalMax(value)
被注释的元素必须是一个数字,其值必须小于等于指定的最大值
-
@Size(max=, min=)
被注释的元素的大小必须在指定的范围内
-
@Digits (integer, fraction)
被注释的元素必须是一个数字,其值必须在可接受的范围内
-
@Past
被注释的元素必须是一个过去的日期
-
@Future
被注释的元素必须是一个将来的日期
-
@Pattern(regex=,flag=)
被注释的元素必须符合指定的正则表达式
Hibernate Validator 校验注解
-
@NotBlank(message =)
验证字符串非 null,且长度必须大于 0
-
@Email
被注释的元素必须是电子邮箱地址
-
@Length(min=,max=)
被注释的字符串的大小必须在指定的范围内
-
@NotEmpty
被注释的字符串的必须非空
-
@Range(min=,max=,message=)
被注释的元素必须在合适的范围内
验证请求参数 Path Variables 和 Request Parameters
@RestController
@RequestMapping("/api")
// 使用 @Validated 注解,告诉 Spring 去校验方法参数。
@Validated
public class PersonController {
@GetMapping("/person/{id}")
// 校验请求参数 Path Variables
public ResponseEntity<Integer> getPersonByID(@Valid @PathVariable("id") @Max(value = 5,message = "超过 id 的范围了") Integer id) {
return ResponseEntity.ok().body(id);
}
@PutMapping("/person")
// 校验请求参数 Request Parameters
public ResponseEntity<String> getPersonByName(@Valid @RequestParam("name") @Size(max = 6,message = "超过 name 的范围了") String name) {
return ResponseEntity.ok().body(name);
}
}
验证请求体
在需要验证的参数上加上了@Valid 注解,如果验证失败,它将抛出 MethodArgumentNotValidException。默认情况下,Spring 会将此异常转换为 HTTP Status 400(错误请求)。
@RestController
@RequestMapping("/api")
public class PersonController {
@PostMapping("/person")
public ResponseEntity<Person> getPerson(@RequestBody @Valid Person person) {
return ResponseEntity.ok().body(person);
}
}
实现定时任务
基于 SpringBoot 的 @Scheduled 注解就能很方便地创建一个定时任务。
@Component
public class ScheduledTasks {
private static final Logger log = LoggerFactory.getLogger(ScheduledTasks.class);
private static final SimpleDateFormat dateFormat = new SimpleDateFormat("HH:mm:ss");
/**
* fixedRate:即固定速率执行,单位是毫秒,这里 5000 表示每 5 秒执行一次。
*/
@Scheduled(fixedRate = 5000)
public void reportCurrentTimeWithFixedRate() {
log.info("Current Thread : {}", Thread.currentThread().getName());
log.info("Fixed Rate Task : The time is now {}", dateFormat.format(new Date()));
}
}
然后需要在启动类中加上 @EnableScheduling 注解,这样才可以启动定时任务。@EnableScheduling 注解的作用是发现注解 @Scheduled 的任务并在后台执行该任务。
SpringBoot
简介
SpringBoot 是由 pivotal 团队开发的全新的Spring 框架,其设计初衷是简化 Spring 应用复杂的搭建和开发过程。该框架提供了一套简单的 Spring 模块依赖和管理工具,从而避免了开发人员处理复杂的模块依赖和版本冲突问题,同事提供打包即可用 Web 服务。
SpringBoot 特点如下:
- 快速创建独立的 Spring 应用程序
- 嵌入 Tomcat Web 容器,可快速部署。
- 自动配置 JAR 包依赖和版本控制,简化 Maven 配置
- 自动装配 Spring 实例,不需要 XML 配置
- 提供诸如性能指标、健康检查、外部配置等线上监控和配置功能。
常用注解
@SpringBootApplication
Spring Boot 项目的基石,创建 SpringBoot 项目之后会默认在主类加上。
@SpringBootApplication 可以认为是 @Configuration 、@EnableAutoConfiguration 和 @ComponentScan 注解的集合。其中
- @EnableAutoConfiguration:启用 SpringBoot 的自动配置机制
- @ComponentScan: 扫描被 @Component / @Service / @Controller 注解的 Bean,注解默认会扫描该类所在的包下所有的类。
- @Configuration:允许在 Spring 上下文中注册额外的 Bean 或导入其他配置类
@Value & @ ConfigurationProperties & @PropertySource
通过 @Value(“${property}”) 读取比较简单的配置信息;
通过 @ConfigurationProperties 读取配置信息并与 bean 绑定;
通过 @PropertySource 读取指定 properties 文件。
@ControllerAdvice & @ExceptionHandler
@ControllerAdvice:注解定义全局异常处理类
@ExceptionHandler:注解声明异常处理方法