一. 基础版本
在登陆界面有时需要保存已经登陆的账号信息,可以点开一个下拉菜单选择保存的账号,这里是用UIKit的表格组件编写一个下拉菜单组件demo,实现思路如下:
1.首先在一个UIViewController里放一个按钮或者标签框,用来显示当前选中的账号,右边放一个打开下拉菜单的小按钮,点击按钮时打开菜单,菜单是一个UITableView,设置好显示框后,将UITableViewController作为当前UIViewController的childviewcontroller,将表格视图作为当前根视图的子视图,尺寸根据显示框调整;
2.然后是菜单的显示和隐藏,将UITableView的背景设置为透明,去掉多余的表格分割线,使用表格的一个section的多个row来显示已有账号,隐藏菜单时返回row的数量为0这样刷新表格后会不显示,当显示菜单时,返回row的数量为数据源数组的长度然后reload表格即可;
3.最后要实现点击选中表格中的cell时将对应账号显示在显示框中,作为当前选中的账号,方法是在表格类内定义代理事件,并在跟视图中实现代理传入选中的cell的index将选中的账号更新显示在显示框内。
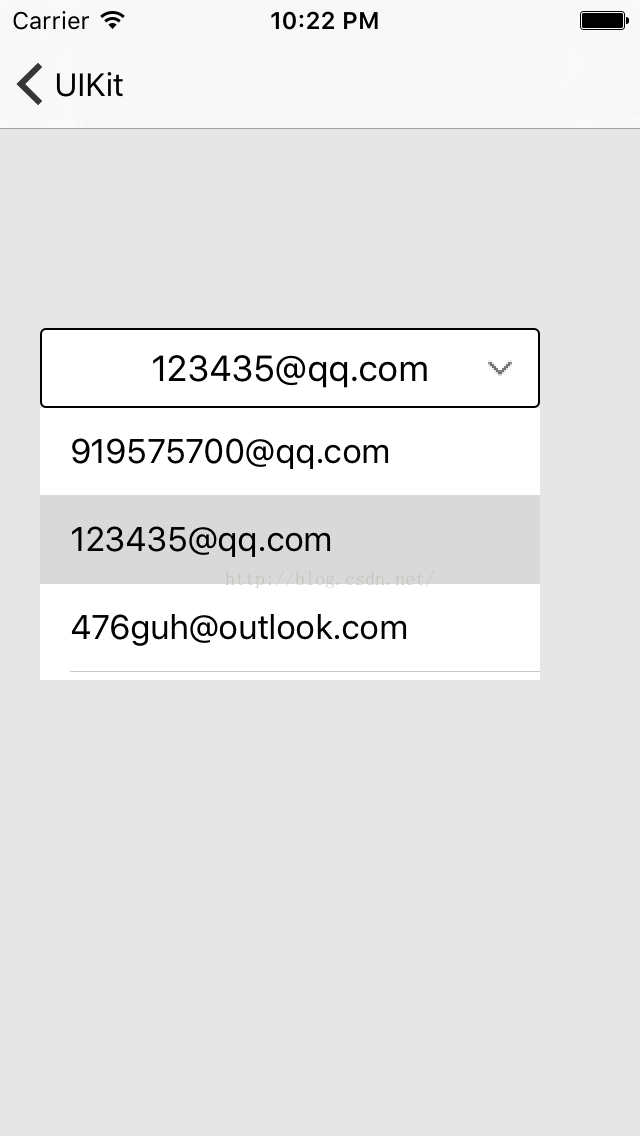
跟视图代码:
-
-
-
-
-
-
-
-
- #import <UIKit/UIKit.h>
-
- @interface AccountSelectViewController : UIViewController
-
- @end
-
-
-
-
-
-
-
- #define inputW 250 // 选择框宽度
- #import "AccountSelectViewController.h"
- #import "AccountTableViewController.h"
-
- @interface AccountSelectViewController ()<AccountDelegate>
-
-
-
-
- @property (nonatomic) NSArray *dataSource;
-
-
-
-
- @property (nonatomic, copy) UIButton *curAccount;
-
-
-
-
- @property (nonatomic)AccountTableViewController *accountList;
-
- @end
-
- @implementation AccountSelectViewController
-
- - (void)viewDidLoad {
- [super viewDidLoad];
-
-
- [self request];
-
-
- _accountList = [[AccountTableViewController alloc] init];
-
- _accountList.accountSource = _dataSource;
-
- _accountList.delegate = self;
-
- [self addChildViewController:_accountList];
- [self.view addSubview:_accountList.view];
-
-
- _curAccount = [[UIButton alloc]initWithFrame:CGRectMake(20, 100, 250, 40)];
-
- [_curAccount setTitle:[_dataSource objectAtIndex:0] forState:UIControlStateNormal];
-
- [_curAccount setTitleColor:[UIColor blackColor] forState:UIControlStateNormal];
-
- _curAccount.layer.borderWidth = 1.0;
-
- _curAccount.layer.cornerRadius = 3.0;
-
- [_curAccount setBackgroundColor:[UIColor whiteColor]];
-
- _accountList.view.frame = CGRectMake(_curAccount.frame.origin.x, _curAccount.frame.origin.y+_curAccount.frame.size.height, _curAccount.frame.size.width, 200);
- [self.view addSubview:_curAccount];
-
- UIButton *openBtn = [[UIButton alloc]initWithFrame:CGRectMake(inputW-30, 10, 20, 20)];
- [openBtn setImage:[UIImage imageNamed:@"down_arrow"] forState:UIControlStateNormal];
- [openBtn addTarget:self action:@selector(openAccountList) forControlEvents:UIControlEventTouchUpInside];
- [_curAccount addSubview:openBtn];
- }
-
-
-
-
- - (void)request {
- _dataSource = [[NSArray alloc]initWithObjects:@"919575700@qq.com", @"123435@qq.com", @"476guh@outlook.com", @"gvb84t48@163.com", nil nil];
- }
-
-
-
-
- - (void)openAccountList {
- _accountList.isOpen = !_accountList.isOpen;
- [_accountList.tableView reloadData];
- }
-
-
-
-
- - (void)selectedCell:(NSInteger)index {
-
- [_curAccount setTitle:[_dataSource objectAtIndex:index] forState:UIControlStateNormal];
- }
-
- @end
表格子视图代码: