目录
八、Spring Boot自定义starters
**starters:**场景启动器
1. 导入依赖
<dependency>
<groupId>com.why</groupId>
<artifactId>why-spring-boot-starter</artifactId>
<version>1.0-SNAPSHOT</version>
</dependency>
2. 编写自动配置
指定配置类
@Configuration(
proxyBeanMethods = false
)
public @interface Configuration {
@AliasFor(
annotation = Component.class
)
String value() default "";
boolean proxyBeanMethods() default true;
}
在指定条件成立的条件下X自动配置类生效@ConditionalOnXxx
@ConditionalOnClass({Servlet.class, DispatcherServlet.class, WebMvcConfigurer.class})
@ConditionalOnMissingBean({WebMvcConfigurationSupport.class})
指定自动配置类的顺序
@AutoConfigureOrder
@AutoConfigureAfter
给容器中添加组件
@Bean
给容器添加组件时绑定相关配置
@ConditionalOnProperty(
prefix = "spring.mvc.hiddenmethod.filter",
name = {"enabled"},
matchIfMissing = false
)
public @interface ConditionalOnProperty {
String[] value() default {};
String prefix() default "";
String[] name() default {};
String havingValue() default "";
boolean matchIfMissing() default false;
}
加载自动配置类
将需要启动就加载的自动配置类,配置在META-INF/spring.factories中
如下列配置:
org.springframework.boot.autoconfigure.web.client.RestTemplateAutoConfiguration,\
org.springframework.boot.autoconfigure.web.embedded.EmbeddedWebServerFactoryCustomizerAutoConfiguration,\
3. 模式
启动器(Starter)
启动器模块是一个空的JAR文件,仅提供辅助性依赖管理,这些依赖可能用于自动装配或者其他类库
命名规范
官方命名空间:
前缀:spring-boot-starter-
模式:spring-boot-starter-模块名
举例:spring-boot-starter-web
自定义命名空间:
后缀:-spring-boot-starter
模式:模块-spring-boot-starter
举例:mybatis-spring-boot-starter
模式
自定义xxxx-starter,自动配置应该再编写xxxx-starter-autoconfigurer
4. 创建空项目
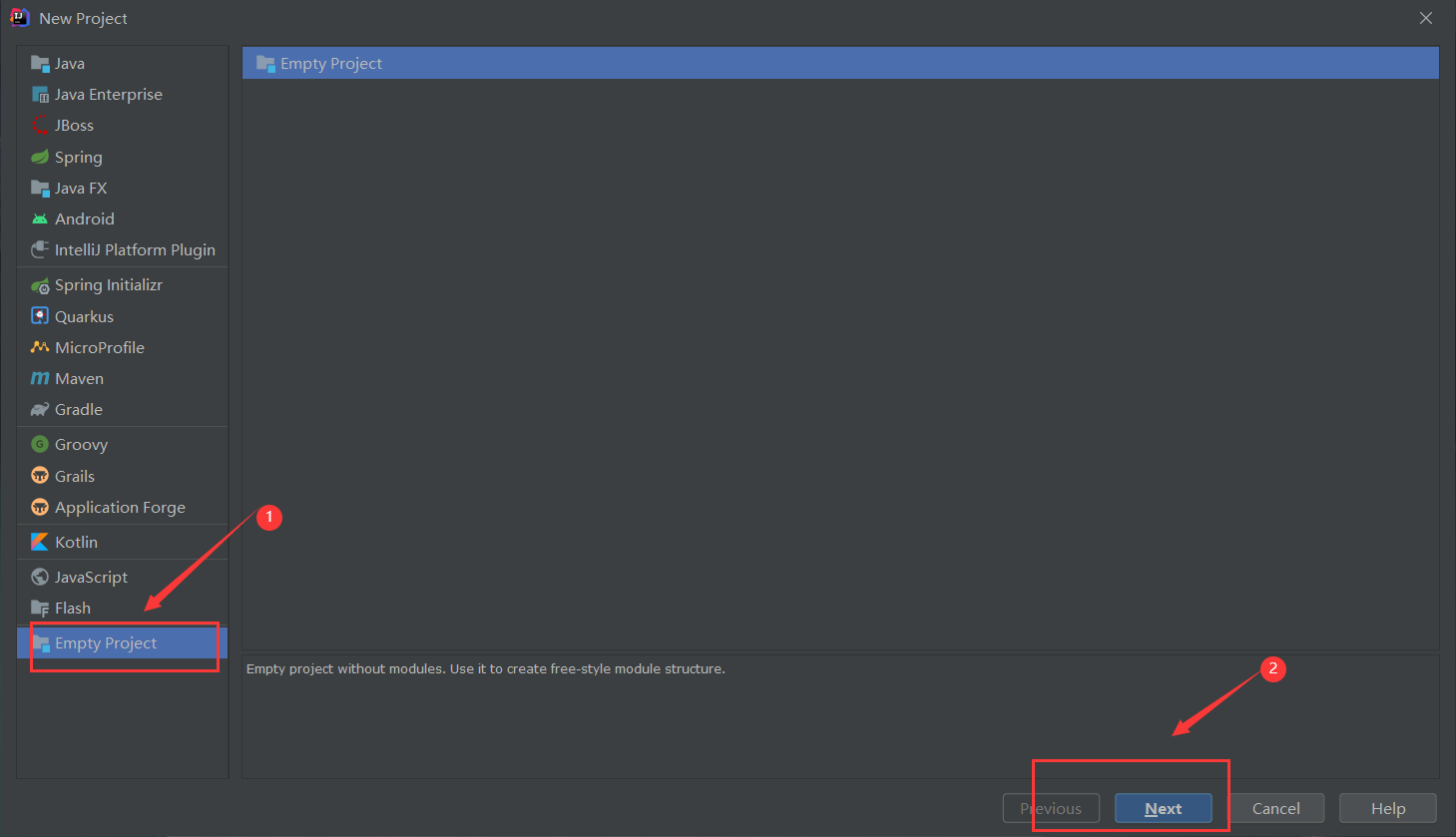
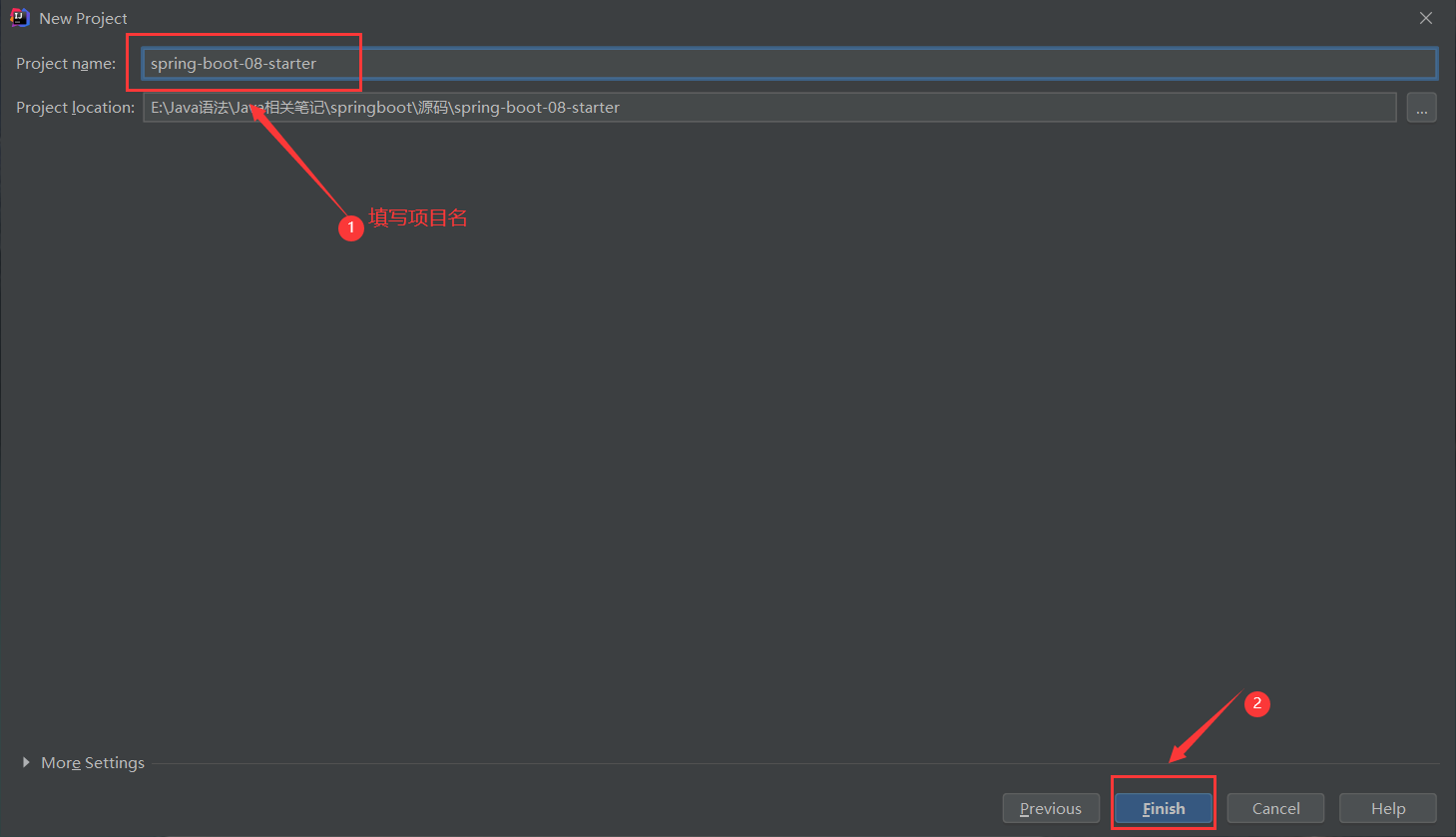
file - > Project Settings - > Modules
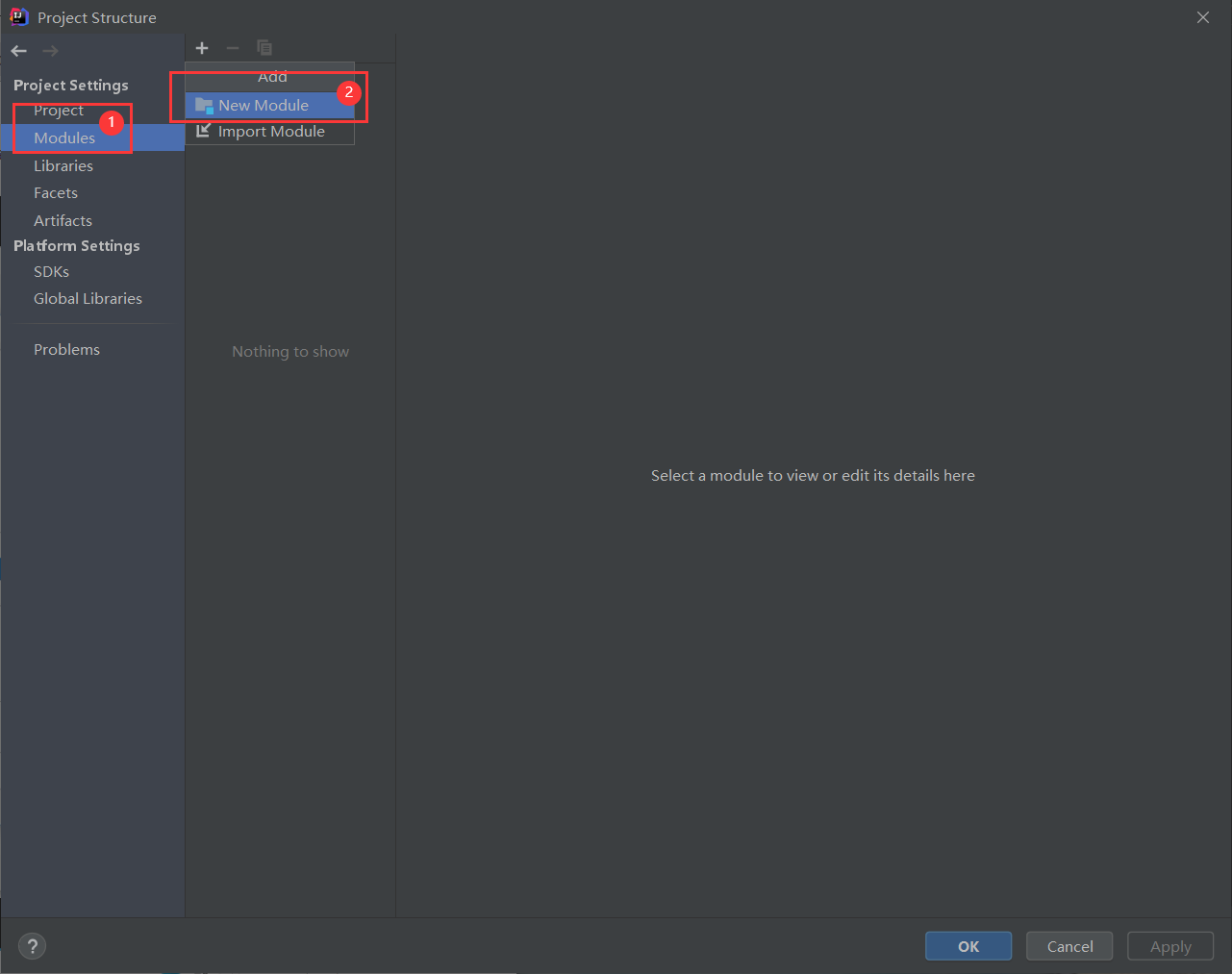
创建新工程,创建Maven工程,用于做启动器
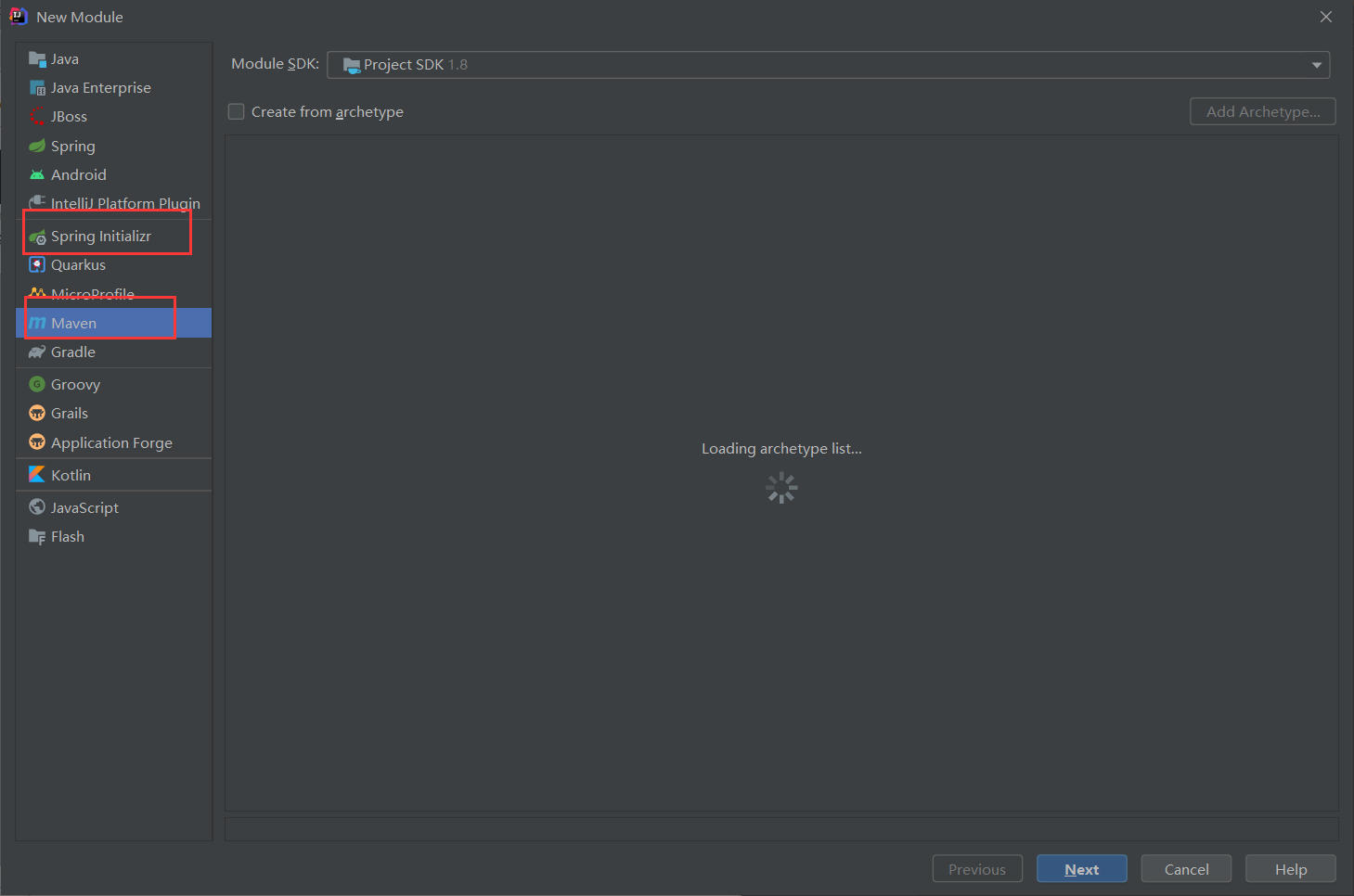
自定义Starter名
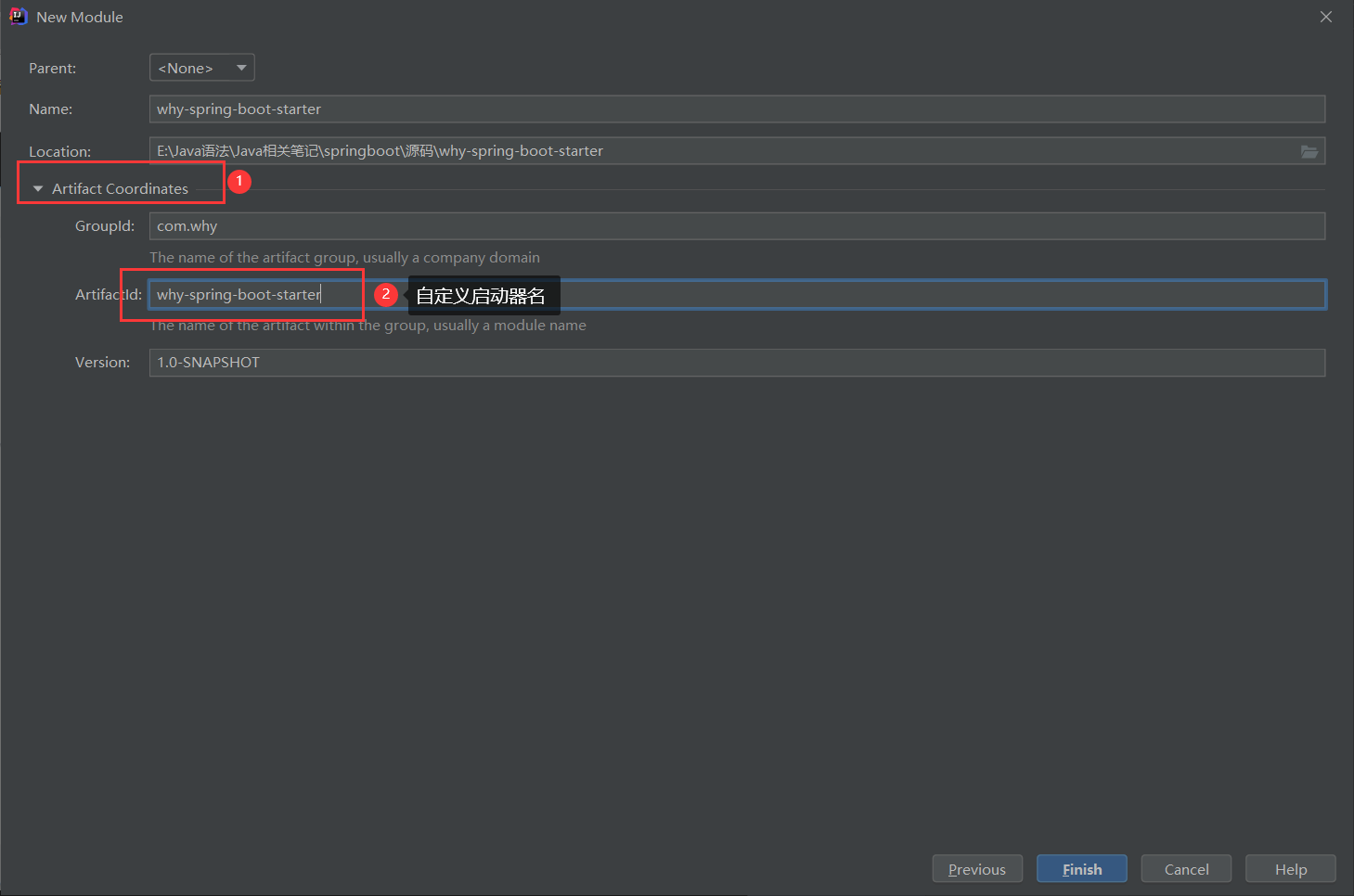
利用Spring Boot初始化器创建新模块,用作自动配置
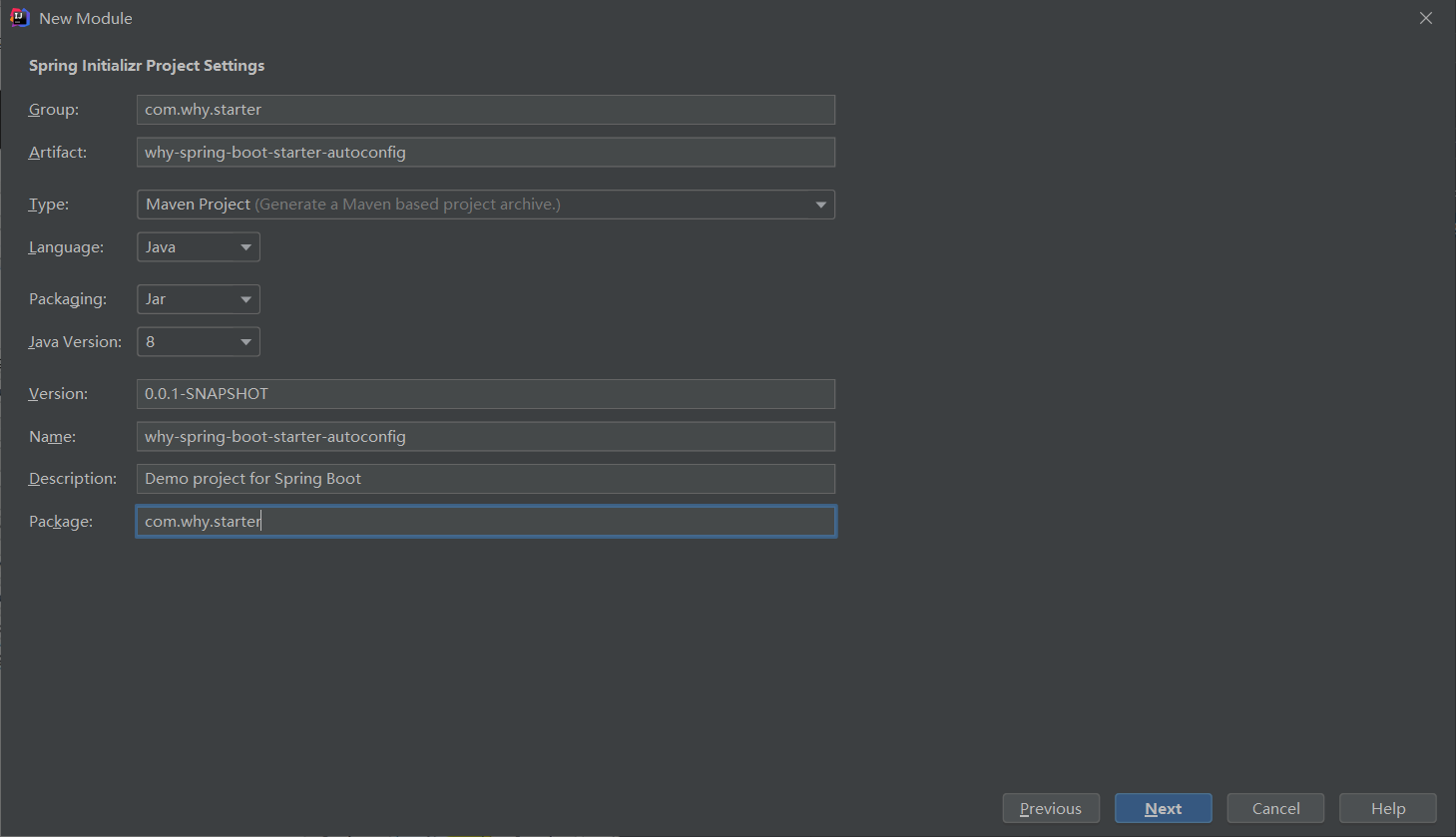
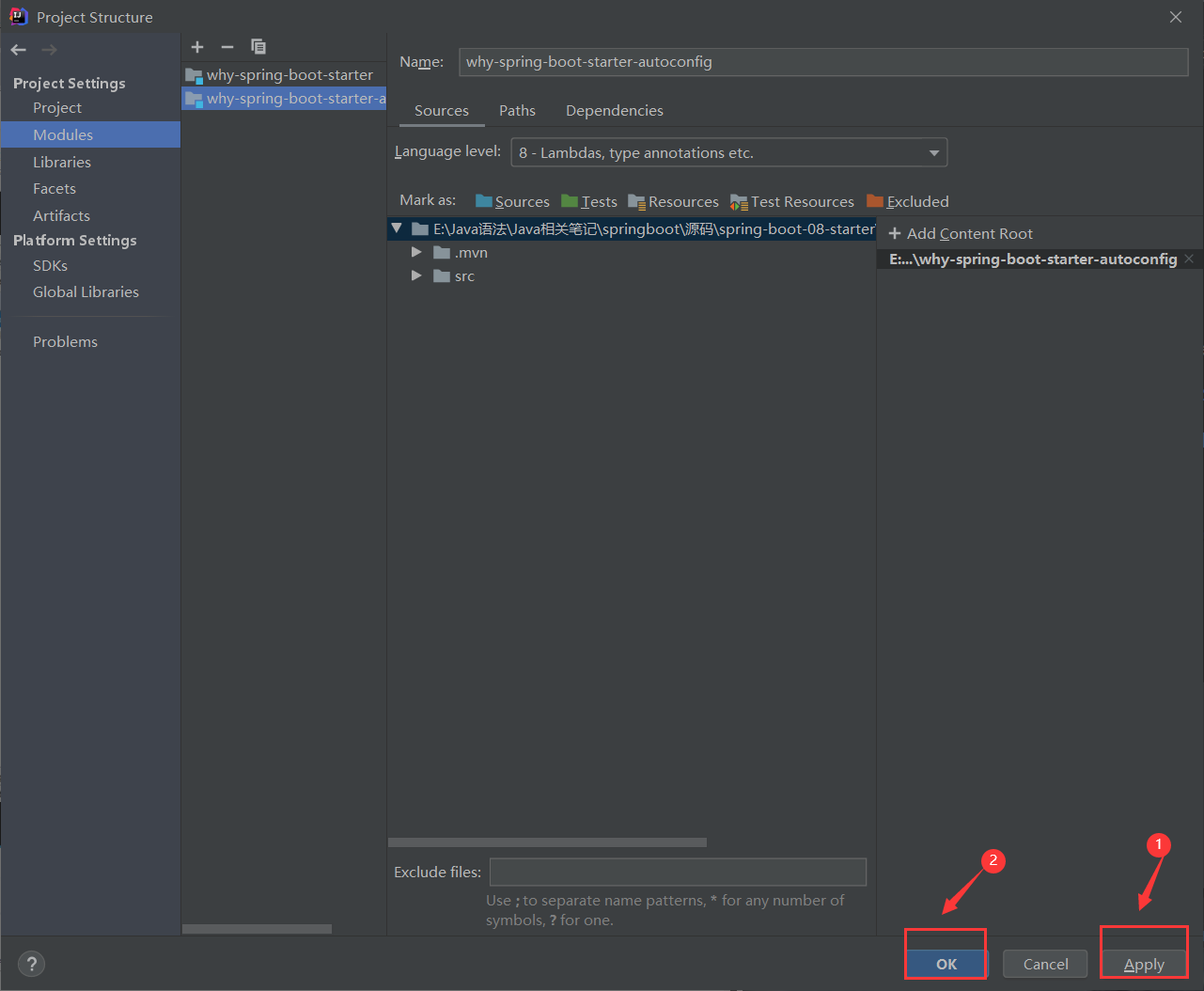
5. 自定义Starter
创建的目录结构
5.1 why-spring-boot-starter设置
引入why-spring-boot-autoconfig中的groupId、artifactId和version
<!-- 启动器-->
<dependencies>
<!-- 引入自动配置模块-->
<dependency>
<groupId>com.why.starter</groupId>
<artifactId>why-spring-boot-starter-autoconfig</artifactId>
<version>0.0.1-SNAPSHOT</version>
</dependency>
</dependencies>
5.2 why-spring-boot-autoconfig中的设置
删除并新建相关文件或文件夹
目录结构:
pom.xml文件中删除多余依赖
保留的依赖:
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
</dependency>
</dependencies>
创建HelloService服务
public class HelloService {
@Autowired
HelloProperties helloProperties;
public String sayHelloWhy(String name){
return helloProperties.getPrefix()+"-"+name + helloProperties.getSuffix();
}
}
创建属性类
import org.springframework.boot.context.properties.ConfigurationProperties;
/**
* @Description TODO 属性类
* @Author why
* @Date 2020/12/29 16:51
* Version 1.0
**/
@ConfigurationProperties(prefix = "why.hello")
public class HelloProperties {
private String prefix;
private String suffix;
public String getPrefix() {
return prefix;
}
public void setPrefix(String prefix) {
this.prefix = prefix;
}
public String getSuffix() {
return suffix;
}
public void setSuffix(String suffix) {
this.suffix = suffix;
}
}
创建自动配置类
@Configuration
@ConditionalOnMissingBean(HelloService.class)//容器中没有HelloService时才生效
@EnableConfigurationProperties(HelloProperties.class)//让配置类生效,开启属性文件绑定功能,默认放在容器中
public class HelloServiceAutoConfiguration {
@Autowired
HelloProperties helloProperties;
@Bean
public HelloService helloService(){
HelloService service = new HelloService();
return service;
}
}
创建配置factories文件
org.springframework.boot.autoconfigure.EnableAutoConfiguration=\
com.why.starter.HelloServiceAutoConfiguration
5.3 将自动配置模块和启动器模块导入Maven仓库
将自动配置类导入Maven仓库
按住ctrl键同时 选中clean和install运行
将Starter模块导入Maven仓库
操作同上
引用自定义的Starter
pom.xml文件中添加依赖
<dependency>
<groupId>com.why</groupId>
<artifactId>why-spring-boot-starter</artifactId>
<version>1.0-SNAPSHOT</version>
</dependency>
创建测试实例
RestController
public class HelloController {
@Autowired
HelloService helloService;
@GetMapping("/starter/hello")
public String sayHello(){
String wyy = helloService.sayHelloWhy("wyy");
return wyy;
}
}
配置文件中配置前后缀
why.hello.prefix="why"
why.hello.suffix="666"