#!usr/bin/python
# coding=UTF-8
#Python3字符串
''''''
'''
python字符串
1、使用单引号''或双引号""来创建字符串。
2、访问子字符串,使用方括号[]来截取字符串,变量[头下标:尾下标]
3、字符串索引值以0开始,-1为从末尾开始。
4、字符串以 + 进行拼接
'''
str1 = 'abcdefg'
str2 = str1[0:5]
print(str1[3],str1[:3],str2) #输出 d abc abcde
print(str1[2:-1],str1[3:-2]) #输出 cdef de
str3 = str1[2:5] + 'test'
print(str3) #输出 cdetest
"""
python转义字符
"""
# \ 续行符
# \\ 输出反斜杠符号
# \' 输出单引号
# \" 输出双引号
# \a 响铃
# \b 退格(Backspace),如果前面是字符串,会被退格删除掉
# \000 空
# \n 换行
# \v 纵向制表符
# \t 横向制表符
# \r 回车,将 \r 后面的内容移到字符串开头,并逐一替换开头部分的字符,直至将 \r 后面的内容完全替换完成。
# \f 换页
# \yyy 八进制数,y 代表 0~7 的字符,例如:\012 代表换行。
# \xyy 十六进制数,以 \x 开头,y 代表的字符,例如:\x0a 代表换行
# \other 其它的字符以普通格式输出
"""
"""
print('str\
str2\
str3') #输出 str str2 str3
print("test\\test") #输出 test\test
print('\'完美世界\'') #输出 '完美世界'
print('\"test\"') #输出 "test"
print('\a') #输出声音,不过要在cmd python环境中执行有声音
print('test \btest','abc\bdefg') #输出 testtest abdefg
print("test\000test",'111\000222') #输出 test test 111 222
print('abc\nefg')
'''
输出
abc
efg
'''
print('test\vtest') #输出 testtest
print('test\ttest') #输出 test test
print('python is good\r222')
#输出 222(在pycharm中执行结果)
#输出 222hon is good(在cmd python环境中执行结果)
print('test \f test') #输出 test test
print('\152') #输出 j
print('\x34') #输出 4
'''
python字符串运算符
+ 字符串连接
* 重复输出字符串,后面数字多少,则多少次输出
[] 通过索引获取字符串中某个字符
[:] 截取字符串中的一部分,遵循左闭右开原则,如str[0:2]是不包含第3个字符。
in 判断字符串是否包含给定的字符,有则返回True,没有则返回False
not in 判断字符串是否不包含给定的字符,没有则返回True,有则返回False
r/R 输出原始字符串
% 格式字符串
'''
str1 = 'Hello'
str2 = 'python'
print(str1 + str2) #输出 Hellopython
print(str1 * 3) #输出 HelloHelloHello
print(str2[3]) #输出 h
print(str1[0:2]) #输出 He
print('H' in str1) #输出 True
print('g' not in str1) #输出 True
print(r'\n\\"\'') #输出 \n\\"\'
print(R'\n\\"\'') #输出 \n\\"\'
'''
python字符串格式化输出
%s 格式化字符串
%d 格式化整数
%f 格式化浮点数字,可指定小数点后的精度
f-string 格式化字符串以 f 开头,后面跟着字符串,字符串中的表达式用大括号 {} 包起来,它会将变量或表达式计算后的值替换进去
'''
print('我是%s,已经%d年了,今天赚了%0.2f元' % ('tom',5,600.555)) #输出 我是tom,已经5年了,今天赚了600.55元
name = 'Tom'
list1 = ['test1','333','test555']
print(f'Hello {name}') #输出 Hello Tom
print(f'{3+5}') #输出 8
print(f'{3+5=}') #输出 3+5=8 使用 = 符号来拼接运算表达式与结果;
print(f'{list1[2]}') #输出 test555
'''
python字符串内置函数
capitalize() 将字符串第一个字符串转换为大写,其他字母变小写。
center() 返回一个指定的宽度 width 居中的字符串,如果 width 小于字符串宽度直接返回字符串,否则使用 fillchar 去填充。
center(width,fillchar)
width -- 字符串的总宽度。
fillchar -- 填充字符,默认为空格。
'''
str1 = 'abcdeFG'
print(str1.capitalize()) #输出 Abcdefg
print(str1.center(20,'*')) #输出 ******abcdeFG*******
print(str1.center(20)) #输出 abcdeFG
print(str1.center(3)) #输出 abcdeFG
'''
count() 返回子字符串在字符串中出现的次数,可选参数为在字符串搜索的开始与结束位置。
count(sub, start = 0,end = len(str))
sub 搜索的子字符串
start 字符串开始搜索的位置。默认为第一个字符,第一个字符索引值为0。
end 字符串中结束搜索的位置。默认为字符串的最后一个位置。
'''
str1 = "aabbccaabbcc"
sub = 'c'
print(str1.count(sub)) #输出 4
print(str1.count(sub,5)) #输出 3
print(str1.count(sub,5,11)) #输出 2
print(str1.count(sub,5,12)) #输出 3
print(str1.count(sub,5,122))#输出 3
'''
encode() 以指定的编码格式编码字符串,它是一个 bytes 对象。
str.encode(encoding='UTF-8', errors='strict')
encoding -- 要使用的编码,如: UTF-8,GBK。
errors -- 设置不同错误的处理方案。默认为 'strict',意为编码错误引起一个UnicodeError。
其他可能得值有 'ignore', 'replace', 'xmlcharrefreplace', 'backslashreplace'
以及通过 codecs.register_error() 注册的任何值。
decode() 以指定的编码格式解码 bytes 对象。默认编码为 'utf-8'。
bytes.decode(encoding="utf-8", errors="strict")
encoding -- 要使用的编码,如: UTF-8,GBK。
errors -- 设置不同错误的处理方案。默认为 'strict',意为编码错误引起一个UnicodeError。
其他可能得值有 'ignore', 'replace', 'xmlcharrefreplace', 'backslashreplace'
以及通过 codecs.register_error() 注册的任何值。
'''
str1 = "Python编程"
print(str1.encode('UTF-8')) #输出 b'Python\xe7\xbc\x96\xe7\xa8\x8b'
print(str1.encode('GBK')) #输出 b'Python\xb1\xe0\xb3\xcc'
print(str1.encode('UTF-8').decode('UTF-8','strict')) #输出 Python编程
print(str1.encode('GBK').decode('GBK','strict')) #输出 Python编程
'''
endswith() 判断字符串含有指定的后缀,有则返回 True,否则返回 False。
str.endswith(suffix,start,end)
suffix -- 可以是一个字符串或者是一个元素。
start -- 字符串中的开始位置。
end -- 字符中结束位置。
'''
str1 = '2021032912332.jpg'
print(str1.endswith('.jpg')) #输出 True
print(str1.endswith('.jpg',10)) #输出 True
print(str1.endswith('.png')) #输出 False
print(str1.endswith('.png',1,10)) #输出 False
'''
expandtabs() 返回字符串中的 tab 符号 \t 转为空格后生成的新字符串。tab 符号 \t 默认的空格数是 8。
在第 0、8、16...等处给出制表符位置,如果当前位置到开始位置或上一个制表符位置的字符数不足 8 的倍数则以空格代替。
str.expandtabs(tabsize=8)
tabsize -- 指定转换字符串中的 tab 符号 \t 转为空格的字符数。不足部分用空格代替
'''
str1 = 'aaa\tbbbb\tccc'
print(str1.expandtabs()) #输出 aaa bbbb ccc
print(str1.expandtabs(2)) #输出 aaa bbbb ccc
print(str1.expandtabs(6)) #输出 aaa bbbb ccc
str1 = 'aaa\tbbb\tccc'
print(str1.expandtabs()) #输出 aaa bbb ccc
print(str1.expandtabs(2)) #输出 aaa bbb ccc
print(str1.expandtabs(6)) #输出 aaa bbb ccc
str1 = 'aaabbbccc\tbbb\tccc'
print(str1.expandtabs()) #输出 aaabbbccc bbb ccc
print(str1.expandtabs(2)) #输出 aaabbbccc bbb ccc
print(str1.expandtabs(6)) #输出 aaabbbccc bbb ccc
'''
find() 检测字符串中是否包含子字符串
如果包含子字符串,返回开始的索引值。如果不包含,则返回-1。
str.find(str, beg=0, end=len(string))
str -- 指定检索的子字符串
beg -- 开始索引,默认为0。
end -- 结束索引,默认为字符串的长度。
'''
str1 = 'abcdefghijkdef'
str2 = 'def'
print(str1.find(str2)) #输出 3
print(str1.find(str2,5)) #输出 11
print(str1.find(str2,2,8)) #输出 3
print(str1.find(str2,12)) #输出 -1
'''
rfind() 返回字符串最后一次出现的位置,如果没有匹配项则返回-1。
str.rfind(str,begin=0, end=len(string))
str -- 查找的字符串
begin -- 开始查找的位置,默认为0
end -- 结束查找位置,默认为字符串的长度。
'''
str1 = "Python is good"
str2 = "is"
print(str1.rfind(str2)) #输出 7
print(str1.rfind('o')) #输出 12
print(str1.rfind('o', 1, 10)) #输出 4
print(str1.rfind('o', 1, 2)) #输出 -1
'''
index() 检测字符串中是否包含子字符串
如果包含子字符串,返回开始的索引值。如果不包含,则抛出异常。
str.index(str, beg=0, end=len(string))
str -- 指定检索的子字符串
beg -- 开始索引,默认为0。
end -- 结束索引,默认为字符串的长度。
'''
str1 = 'abcdefghijkdef'
str2 = 'def'
print(str1.index(str2)) # 输出 3
print(str1.index(str2, 5)) # 输出 11
print(str1.index(str2, 2, 8)) # 输出 3
#print(str1.index(str2, 12)) # 输出 ValueError: substring not found
'''
rindex() 返回子字符串 str 在字符串中最后出现的位置,如果没有匹配的字符串会报异常。
str.rindex(str, begin=0, end=len(string))
str -- 查找的字符串
begin -- 开始查找的位置,默认为0
end -- 结束查找位置,默认为字符串的长度。
'''
str1 = "Python is good"
str2 = "is"
print(str1.rindex(str2)) #输出 7
print(str1.rindex('o')) #输出 12
print(str1.rindex('o', 1, 10)) #输出 4
#print(str1.rindex('aa')) #输出 ValueError: substring not found
'''
isalnum() 检测字符串是否由字母和数字组成。
如果 string 至少有一个字符并且所有字符都是字母或数字则返回 True,否则返回 False
'''
str1 = 'abcdefghijkdef'
str2 = 'def2222'
str3 = 'www.baid.com'
print(str1.isalnum()) # 输出 True
print(str2.isalnum()) # 输出 True
print(str3.isalnum()) # 输出 False
'''
isalpha() 检测字符串是否只由字母或文字组成。
如果字符串至少有一个字符并且所有字符都是字母或文字则返回 True,否则返回 False。
'''
str1 = 'python教程'
str2 = 'paython2222'
str3 = '中文教程'
print(str1.isalpha()) # 输出 True
print(str2.isalpha()) # 输出 False
print(str3.isalpha()) # 输出 True
'''
isdigit() 检测字符串是否只由数字组成。
如果字符串只包含数字则返回 True 否则返回 False。
'''
str1 = '2992512'
str2 = 'paython2222'
str3 = '中文教程'
print(str1.isdigit()) # 输出 True
print(str2.isdigit()) # 输出 False
print(str3.isdigit()) # 输出 False
'''
islower() 检测字符串是否由小写字母组成。
如果字符串中包含至少一个区分大小写的字符,
并且所有这些(区分大小写的)字符都是小写,则返回 True,否则返回 False
'''
str1 = '2992512'
str2 = 'paython2222'
str3 = 'Gtkdfg'
print(str1.islower()) # 输出 False
print(str2.islower()) # 输出 True
print(str3.islower()) # 输出 False
'''
isnumeric() 检测字符串是否只由数字组成,
数字可以是: Unicode 数字,全角数字(双字节),罗马数字,汉字数字。
指数类似 ² 与分数类似 ½ 也属于数字。
字符串中只包含数字字符,则返回 True,否则返回 False
'''
str1 = '2992512'
str2 = '一二2222'
str3 = 'Gtkdfg'
print(str1.isnumeric()) # 输出 True
print(str2.isnumeric()) # 输出 True
print(str3.isnumeric()) # 输出 False
'''
isspace() 检测字符串是否只由空白字符组成。
如果字符串中只包含空格,则返回 True,否则返回 False.
'''
str1 = ' '
str2 = 'test test'
print(str1.isspace()) # 输出 True
print(str2.isspace()) # 输出 False
'''
istitle() 检测字符串中所有的单词拼写首字母是否为大写,且其他字母为小写。
如果字符串中所有的单词拼写首字母为大写,且其他字母为小写则返回 True,否则返回 False.
'''
str1 = 'Kicle Tom'
str2 = 'Test test'
print(str1.istitle()) # 输出 True
print(str2.istitle()) # 输出 False
'''
isupper() 检测字符串中所有的字母是否都为大写。
如果字符串中包含至少一个区分大小写的字符,
并且所有这些(区分大小写的)字符都是大写,则返回 True,否则返回 False
'''
str1 = 'Kicle Tom'
str2 = 'TEST 123'
str3 = 'TEST TEST'
print(str1.isupper()) # 输出 False
print(str2.isupper()) # 输出 True
print(str3.isupper()) # 输出 True
'''
join() 将序列中的元素以指定的字符连接生成一个新的字符串。
str.join(sequence)
sequence -- 要连接的元素序列。
返回通过指定字符连接序列中元素后生成的新字符串。
'''
str1 = " "
str2 = "-"
sequence = ['Python','is','the','best']
print(str1.join(sequence)) #输出 Python is the best
print(str2.join(sequence)) #输出 Python-is-the-best
'''
len() 返回对象(字符、列表、元组等)长度或项目个数。
返回对象长度。
'''
list1 = ['Python','is','the','best']
str1 = 'pythonisthebest'
tuple1 = ('test','test')
set1 = {'test','is','test1'}
set2 = {'test','is','test'}
print(len(list1)) #输出 4
print(len(str1)) #输出 15
print(len(tuple1)) #输出 2
print(len(set1)) #输出 3
print(len(set2)) #输出 2
'''
ljust() 返回一个原字符串左对齐,并使用空格填充至指定长度的新字符串。
如果指定的长度小于原字符串的长度则返回原字符串。
str.ljust(width, fillchar)
width -- 指定字符串长度。
fillchar -- 填充字符,默认为空格。
'''
str1 = "Python is the best"
print(str1.ljust(30,'*')) #输出 Python is the best************
print(str1.ljust(10,'*')) #输出 Python is the best
'''
rjust() 返回一个原字符串右对齐,并使用空格填充至长度 width 的新字符串。
如果指定的长度小于字符串的长度则返回原字符串。
str.rjust(width, fillchar)
width -- 指定填充指定字符后中字符串的总长度.
fillchar -- 填充的字符,默认为空格。
'''
str1 = "Python is good"
print(str1.rjust(20, '*')) #输出 ******Python is good
print(str1.rjust(10)) #输出 Python is good
'''
lower() 将字符串中所有大写字符转为小写字母。
返回将字符串中所有大写字符转换为小写后生成的字符串。
upper() 将字符串中所有小写字母转为大写字母。
返回小写字母转为大写字母的字符串。
'''
str1 = "Python Is The Best"
print('lower():'+str1.lower()) #输出 lower():python is the best
print('upper():'+str1.upper()) #输出 upper():PYTHON IS THE BEST
'''
lstrip() 截掉字符串左边的空格或指定字符。
返回截掉字符串左边的空格或指定字符后生成的新字符串。
'''
str1 = " Python Is The Best"
str2 = "cccPython Is The Best"
print(str1.lstrip()) #输出 Python Is The Best
print(str2.lstrip('c')) #输出 Python Is The Best
'''
rstrip() 删除 string 字符串末尾的指定字符(默认为空格).
str.rstrip(chars)
chars -- 指定删除的字符(默认为空格)
'''
str1 = "str1Python is good "
str2 = "str2Python is good22222"
print(str1.rstrip()) #输出 str1Python is good
print(str2.rstrip('2')) #输出 str2Python is good
'''
strip() 移除字符串头尾指定的字符(默认为空格)或字符序列。
只能删除开头或是结尾的字符,不能删除中间部分的字符。
'''
str1 = "===Python==is==good==="
print(str1.strip('=')) #输出 Python==is==good
'''
maketrans() 用于创建字符映射的转换表,对于接受两个参数的最简单的调用方式,
第一个参数是字符串,表示需要转换的字符,第二个参数也是字符串表示转换的目标。
两个字符串的长度必须相同,为一一对应的关系。
str.maketrans(intab,outtab)
intab -- 字符串中要替代的字符组成的字符串。
outtab -- 相应的映射字符的字符串。
translate() 根据参数table给出的表(包含 256 个字符)转换字符串的字符,要过滤掉的字符放到 deletechars 参数中。
str.translate(table)
bytes.translate(table[, delete])
bytearray.translate(table[, delete]
table -- 翻译表,翻译表是通过 maketrans() 方法转换而来。
deletechars -- 字符串中要过滤的字符列表。
返回翻译后的字符串,若给出了 delete 参数,
则将原来的bytes中的属于delete的字符删除,剩下的字符要按照table中给出的映射来进行映射 。
'''
str1 = "Python Is The Best"
intab = "Ps"
outtab = "12"
trantab = str1.maketrans(intab,outtab)
print (str1.translate(trantab)) #输出 1ython I2 The Be2t
'''
max() 返回字符串中最大的字母。
min() 返回字符串中最小的字母。
'''
str1 = 'abcedGtkilfok'
print(max(str1)) #输出 t
print(min(str1)) #输出 G
print(min('dtertdgfderso')) #输出 d
'''
replace() 把字符串中的 old(旧字符串)替换成 new(新字符串),如果指定第三个参数max,则替换不超过 max 次。
str.replace(old,new,max)
old -- 将被替换的子字符串。
new -- 新字符串,用于替换old子字符串。
max -- 可选字符串, 替换不超过 max 次
'''
str1 = 'www.baidu.com'
print(str1.replace('w','b')) #输出 bbb.baidu.com
print(str1.replace('w','b',2)) #输出 bbw.baidu.com
'''
split() 通过指定分隔符对字符串进行切片,如果第二个参数 num 有指定值,则分割为 num+1 个子字符串。
str.split(str="", num=string.count(str))
str -- 分隔符,默认为所有的空字符,包括空格、换行(\n)、制表符(\t)等。
num -- 分割次数。默认为 -1, 即分隔所有。
'''
str1 = "Python is good"
print(str1.split()) #输出 ['Python', 'is', 'good']
print(str1.split('is')) #输出 ['Python ', ' good']
print(str1.split('o',1)) #输出 ['Pyth', 'n is good']
'''
splitlines() 按照行('\r', '\r\n', \n')分隔,返回一个包含各行作为元素的列表,
如果参数 keepends 为 False,不包含换行符,如果为 True,则保留换行符。
str.splitlines(keepends)
keepends -- 在输出结果里是否去掉换行符('\r', '\r\n', \n'),
默认为 False,不包含换行符,如果为 True,则保留换行符。
返回一个包含各行作为元素的列表。
'''
str1 = "Python \nis \r\ngood\r\n"
print(str1.splitlines()) #输出 ['Python ', 'is ', 'good']
print(str1.splitlines(True)) #输出 ['Python \n', 'is \r\n', 'good\r\n']
'''
startswith() 用于检查字符串是否是以指定子字符串开头,
如果是则返回 True,否则返回 False。
如果参数 beg 和 end 指定值,则在指定范围内检查。
str.startswith(substr, begin=0, end=len(string));
str -- 检测的字符串。
substr -- 指定的子字符串。
begin -- 可选参数用于设置字符串检测的起始位置。
end -- 可选参数用于设置字符串检测的结束位置。
'''
str1 = "Python is good"
print(str1.startswith('Python')) #输出 True
print(str1.startswith('test')) #输出 False
print(str1.startswith('Python', 1, 10)) #输出 False
'''
swapcase() 对字符串的大小写字母进行转换。
'''
str1 = "Python Is The Best"
print(str1.swapcase()) #输出 pYTHON iS tHE bEST
'''
title() 返回"标题化"的字符串,就是说所有单词的首字母都转化为大写。
#非字母后的第一个字母将转换为大写字母:
'''
str1 = "Python is the best"
str2 = "Hello 3c3c3c n2n2n2"
print(str1.title()) #输出 Python Is The Best
print(str2.title()) #输出 Hello 3C3C3C N2N2N2
'''
zfill(width) 返回指定长度的字符串,原字符串右对齐,前面填充0。
width -- 指定字符串的长度。原字符串右对齐,前面填充0。
'''
str1 = "Python is the best"
print(str1.zfill(10)) #输出 Python is the best
print(str1.zfill(20)) #输出 00Python is the best
'''
isdecimal() 检查字符串是否只包含十进制字符。这种方法只存在于unicode对象。
定义一个十进制字符串,只需要在字符串前添加 'u' 前缀即可。
如果字符串是否只包含十进制字符返回True,否则返回False。
'''
str1 = "234234"
str2 = "Python"
print(str1.isdecimal()) #输出 True
print(str2.isdecimal()) #输出 False
Python3字符串
最新推荐文章于 2024-01-15 07:57:43 发布
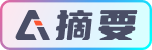