1.功能描述
1.在某个组件下面显示popupwindow,并且背景是灰色。
2.根据内容多少显示popupwindow,当内容过多时,固定popupwindow的高度。
2.废话少说,先看东西
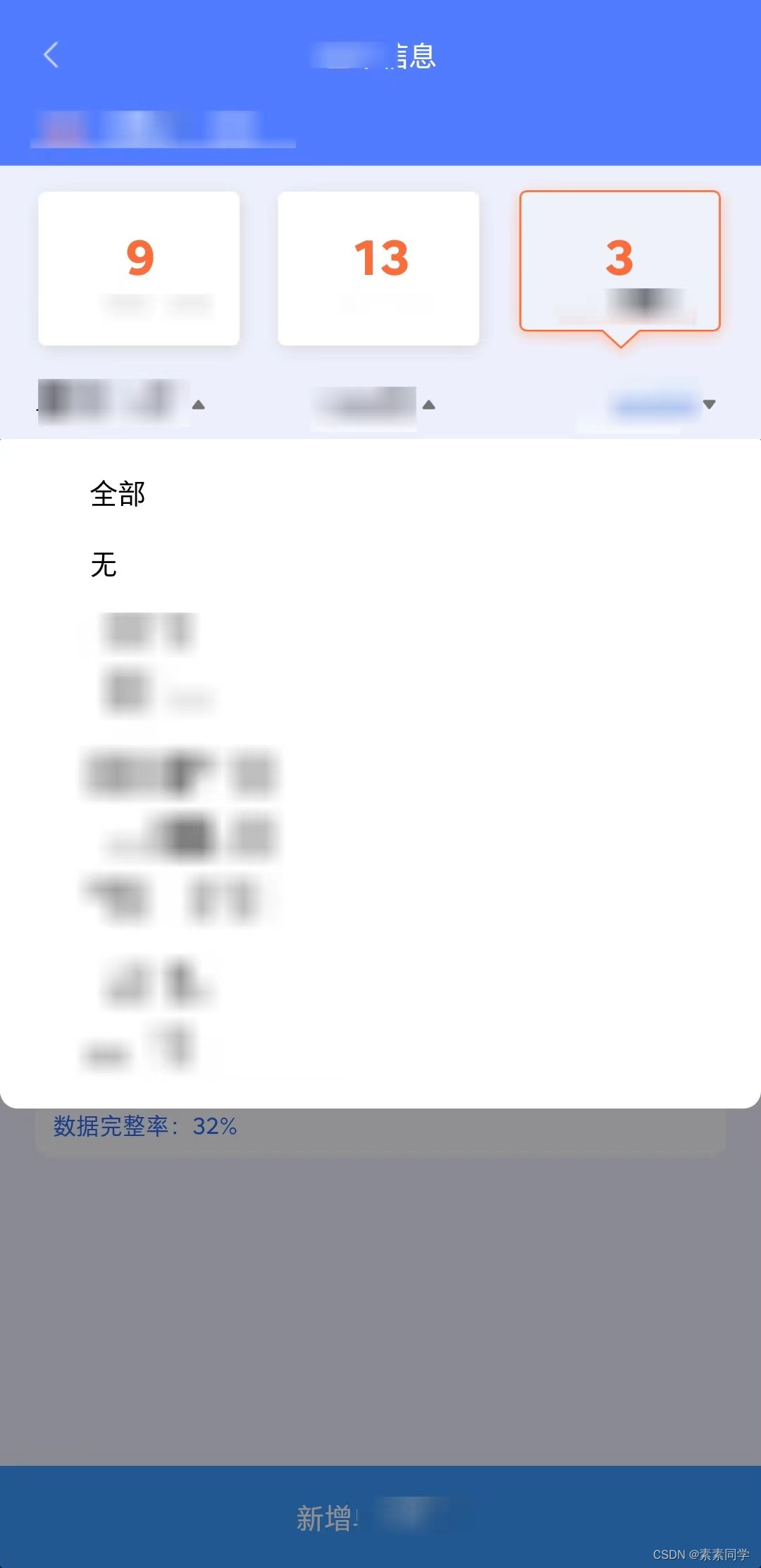
3.使用方法
private List<MyPopupWindowUtilsBean> mPopCenterDataList = new ArrayList<>();
if (mPopCenterDataList != null && mPopCenterDataList.size() > 0) {
cbCenter.setChecked(true);
MyPopupWindowUtils.showMassesReportHandState(mActivity, llSelectorBg, mSelectorBg, mPopCenterDataList, new OnMyPopupWindowUtilsOnItemClickListener() {
@Override
public void onItemClick(String label, String value) {
cbCenter.setChecked(false);
if (!TextUtils.isEmpty(label)) {
if (label.equals("全部")) {
cbCenter.setText("场所分类");
} else {
cbCenter.setText(label);
}
placeClassify = value;
mPullToRefreshLayout.autoRefresh();
}
}
});
} else {
if (sList != null && sList.size() > 0) {
mPopCenterDataList.add(new MyPopupWindowUtilsBean("全部", "", false));
for (DictBean item : sList) {
mPopCenterDataList.add(new MyPopupWindowUtilsBean(item.getLabel(), item.getValue(), false));
}
cbCenter.setChecked(true);
MyPopupWindowUtils.showMassesReportHandState(mActivity, llSelectorBg, mSelectorBg, mPopCenterDataList, new OnMyPopupWindowUtilsOnItemClickListener() {
@Override
public void onItemClick(String label, String value) {
cbCenter.setChecked(false);
if (!TextUtils.isEmpty(label)) {
if (label.equals("全部")) {
cbCenter.setText("默认文案");
} else {
cbCenter.setText(label);
}
placeClassify = value;
mPullToRefreshLayout.autoRefresh();
}
}
});
} else {
ToastUtils.showShort("暂无数据");
}
}
源码 MyPopupWindowUtils.java
public class MyPopupWindowUtils {
private static PopupWindow mPopupWindow;
public static void show(Activity mActivity, View mainView, View bgView, List<MyPopupWindowUtilsBean> list, OnMyPopupWindowUtilsOnItemClickListener listener) {
if (list == null) {
ToastUtils.showShort("数据为空");
return;
}
if (mainView == null) {
ToastUtils.showShort("标题view为空");
return;
}
if (bgView == null) {
ToastUtils.showShort("灰色背景view为空");
return;
}
bgView.setVisibility(View.VISIBLE);
View viewPercentage = LayoutInflater.from(mActivity).inflate(R.layout.my_popup_window_utils_list, null);
RecyclerView mRecyclerView = viewPercentage.findViewById(R.id.mRecyclerView);
mRecyclerView.setLayoutManager(new LinearLayoutManager(mActivity));
MyPopupWindowUtilsAdapter sAdapter = new MyPopupWindowUtilsAdapter();
mRecyclerView.setAdapter(sAdapter);
sAdapter.setOnItemClickListener(new OnItemClickListener() {
@Override
public void onItemClick(BaseQuickAdapter adapter, View view, int position) {
List<MyPopupWindowUtilsBean> data = sAdapter.getData();
for (int i = 0; i < data.size(); i++) {
data.get(i).setSelect(false);
}
MyPopupWindowUtilsBean item = (MyPopupWindowUtilsBean) adapter.getItem(position);
String label = item.getLabel();
String value = item.getValue();
item.setSelect(true);
bgView.setVisibility(View.GONE);
if (listener != null) {
listener.onItemClick(label, value);
}
if (mPopupWindow != null) {
mPopupWindow.dismiss();
}
}
});
sAdapter.setNewInstance(list);
mPopupWindow = new PopupWindow(mActivity);
mPopupWindow.setWidth(ViewGroup.LayoutParams.MATCH_PARENT);
int dataCount = sAdapter.getData().size();
if (dataCount > (400 / 40)) {
mPopupWindow.setHeight(MyDpUtil.dp2px(mActivity, 400));
} else {
mPopupWindow.setHeight(ViewGroup.LayoutParams.WRAP_CONTENT);
}
mPopupWindow.setAnimationStyle(R.style.PopupWindowAnimStyle);
mPopupWindow.setFocusable(true);
mPopupWindow.setTouchable(true);
ColorDrawable dw = new ColorDrawable(0x0000000000);
mPopupWindow.setBackgroundDrawable(dw);
mPopupWindow.setContentView(viewPercentage);
int[] location = new int[2];
mainView.getLocationInWindow(location);
int viewY = location[1];
mPopupWindow.showAtLocation(mainView, Gravity.NO_GRAVITY, 0, viewY + mainView.getMeasuredHeight());
mPopupWindow.setOnDismissListener(new PopupWindow.OnDismissListener() {
@Override
public void onDismiss() {
bgView.setVisibility(View.GONE);
if (listener != null) {
listener.onItemClick("", "");
}
}
});
}
}
源码 OnMyPopupWindowUtilsOnItemClickListener.java
public interface OnMyPopupWindowUtilsOnItemClickListener {
void onItemClick(String label, String value);
}
源码 MyPopupWindowUtilsBean.java
public class MyPopupWindowUtilsBean {
private String label;
private String value;
private boolean select;
public MyPopupWindowUtilsBean() {
}
public MyPopupWindowUtilsBean(String label, boolean select) {
this.label = label;
this.select = select;
}
public MyPopupWindowUtilsBean(String label, String value, boolean select) {
this.label = label;
this.value = value;
this.select = select;
}
public boolean isSelect() {
return select;
}
public void setSelect(boolean select) {
this.select = select;
}
public String getLabel() {
return label;
}
public void setLabel(String label) {
this.label = label;
}
public String getValue() {
return value;
}
public void setValue(String value) {
this.value = value;
}
}
源码 MyPopupWindowUtilsAdapter.java
public class MyPopupWindowUtilsAdapter extends BaseQuickAdapter<MyPopupWindowUtilsBean, BaseViewHolder> {
public MyPopupWindowUtilsAdapter() {
super(R.layout.my_popup_window_utils_list_item, null);
}
@Override
protected void convert(@NonNull BaseViewHolder holder, MyPopupWindowUtilsBean item) {
try {
CheckBox cbLabel = holder.getView(R.id.cbLabel);
cbLabel.setClickable(false);
cbLabel.setEnabled(false);
cbLabel.setText(item.getLabel());
if (item.isSelect()) {
cbLabel.setChecked(true);
} else {
cbLabel.setChecked(false);
}
} catch (Exception e) {
ToastUtils.showShort("数据异常");
}
}
}
布局 my_popup_window_utils_list.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="@drawable/bg_wr_002"
android:orientation="vertical">
<androidx.recyclerview.widget.RecyclerView
android:id="@+id/mRecyclerView"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:listitem="@layout/my_popup_window_utils_list_item" />
</LinearLayout>
布局 my_popup_window_utils_list_item.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="40dp"
android:background="@drawable/btn_bg_transparent_04_r0"
android:orientation="vertical">
<CheckBox
android:checked="false"
android:id="@+id/cbLabel"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:button="@null"
android:drawableRight="@drawable/select_base_bg002"
android:overScrollMode="never"
android:paddingLeft="50dp"
android:paddingRight="50dp"
android:text="全部"
android:textColor="@drawable/select_suwaylead_003"
android:textSize="16sp" />
</LinearLayout>
布局 bg_wr_002.xml
<?xml version="1.0" encoding="utf-8"?>
<shape xmlns:android="http://schemas.android.com/apk/res/android"
android:shape="rectangle">
<solid android:color="#FFFFFF" />
<corners
android:bottomLeftRadius="10dp"
android:bottomRightRadius="10dp"
android:topLeftRadius="1dp"
android:topRightRadius="1dp" />
</shape>
布局 btn_bg_transparent_04_r0.xml
<?xml version="1.0" encoding="utf-8"?>
<selector xmlns:android="http://schemas.android.com/apk/res/android">
<item android:state_pressed="true">
<shape>
<solid android:color="#225985F6" />
</shape>
</item>
<item android:state_pressed="false">
<shape>
<solid android:color="#00FFFFFF" />
</shape>
</item>
</selector>
布局 select_base_bg002.xml
<?xml version="1.0" encoding="utf-8"?>
<selector xmlns:android="http://schemas.android.com/apk/res/android">
<item android:drawable="@mipmap/home_checkmark" android:state_checked="true"></item>
<item android:drawable="@mipmap/home_checkmark1"></item>
</selector>
注意:这里的两张图片可以自行设置(建议去iconfont上自行下载所需样式,这里提供一个库,里面有:
https://www.iconfont.cn/collections/detail?spm=a313x.7781069.0.da5a778a4&cid=1537 )
布局 select_suwaylead_003.xml
<selector xmlns:android="http://schemas.android.com/apk/res/android">
<item android:color="#000000" android:state_checked="false" />
<item android:color="#0056F9" android:state_checked="true" />
</selector>