# encoding: utf-8
# 版权所有 2023 涂聚文有限公司
# 许可信息查看:
# 描述:
# Author : geovindu,Geovin Du 涂聚文.
# IDE : PyCharm 2023.1 python 311
# Datetime : 2023/9/3 7:04
# User : geovindu
# Product : PyCharm
# Project : LukfookLeaveCalculation
# File : EmpLoyeeHolidaysGet.py
# explain : 学习
import sys
import os
import Common.Utils
import Model.Holiday
class EmpLoyeeHolidaysGet(object):
"""
抽取需要的数据
"""
def getYear(self,strsource):
"""
:param strsource:
:return:
"""
refloat = 0
if (strsource != None):
if ("年" in strsource):
refloat=Common.Utils.Utils.getYear(strsource)
return refloat
def getMonth(self, strsource):
"""
:param strsource:
:return:
"""
refloat = 0
if (strsource != None):
if ("月" in strsource):
refloat = Common.Utils.Utils.getMonth(strsource)
return refloat
def getDay(self, strsource):
"""
:param strsource:
:return:
"""
refloat = 0
if (strsource != None):
if ("日" in strsource):
refloat = Common.Utils.Utils.getDay(strsource)
return refloat
def getHolidays(self,strsource):
"""
獲得假期限列表
:param strsource:
:return:
"""
hday=[]
if (strsource != None):
if("星期" not in strsource):
if("nan" not in strsource):
spstr = strsource.split(' ') # 有两个,有换行符,两个假期,一定要这换行符 NaN
if (len(spstr) > 1):
for i in range(len(spstr)):
sk = spstr[i]
#print("for sk", sk)
hd = Model.Holiday.Holiday()
hd=EmpLoyeeHolidaysGet.checkHoliday(self,sk)
hday.append(hd)
elif (len(spstr) == 1):
sk = spstr[0]
#print("sk",sk)
hd = Model.Holiday.Holiday()
hd = EmpLoyeeHolidaysGet.checkHoliday(self,sk)
hday.append(hd)
else:
hday = []
else:
hday = []
return hday
def checkHoliday(self,strsource):
"""
检测是什么假期
:param strsource:
:return:
"""
hd=Model.Holiday.Holiday()
#print("chek")
if ("例休" in strsource):
#print("cehck例休")
hd.HolidayId=1
hd.HolidayName="例休"
hd.WorkTime=Common.Utils.Utils.getRegularHoliday(strsource)
if ("特假" in strsource):
hd.HolidayId=2
hd.HolidayName="特假"
hd.workTime=Common.Utils.Utils.getSpecialLeave(strsource)
if ("補休" in strsource):
hd.HolidayId=3
hd.HolidayName="補休"
hd.workTime = Common.Utils.Utils.getDeferredHoliday(strsource)
if ("加班" in strsource):
hd.HolidayId=4
hd.HolidayName="加班"
hd.workTime = Common.Utils.Utils.getOvertime(strsource)
if ("事假" in strsource):
hd.HolidayId=5
hd.HolidayName="事假"
hd.workTime = Common.Utils.Utils.getPersonalLeave(strsource)
if ("年假" in strsource):
hd.HolidayId = 6
hd.HolidayName = "年假"
hd.workTime = Common.Utils.Utils.getAnnualLeave(strsource)
if ("病假" in strsource):
hd.HolidayId = 7
hd.HolidayName = "病假"
hd.workTime = Common.Utils.Utils.getSickLeave(strsource)
if ("育兒假" in strsource):
hd.HolidayId = 8
hd.HolidayName = "育兒假"
hd.workTime = Common.Utils.Utils.getChildcareLeave(strsource)
if ("遲到" in strsource):
hd.HolidayId = 9
hd.HolidayName = "遲到"
hd.workTime = Common.Utils.Utils.getLateTime(strsource)
return hd
def getEmplee(self,Empleelist,id:int):
"""
從員工列表中找到對應ID員工的信息
:param Empleelist:
:param id:
:return:
"""
ee=[]
for em in Empleelist:
if(em.EmployeeId==id):
ee.append(em.EmployeeNo)
ee.append(em.EmployeeDep)
ee.append(em.EmployeeName)
return ee
def getHoliday(self,holidays):
"""
返回假期
:param holidays:
:return:
"""
getname=""
if(len(holidays)>0):
for hd in holidays:
if(hd.HolidayId==1):
getname=getname+hd.HolidayName+str(hd.WorkTime)+"天 "
elif(hd.HolidayId==9):
getname = getname + hd.HolidayName + str(hd.WorkTime) + "分钟 "
else:
getname = getname + hd.HolidayName + str(hd.WorkTime) + "小时 "
return getname
def getHolidayAnnualLeave(self,holidays):
"""
返回年假数
:param holidays:
:return:
"""
getsum=0
if(len(holidays)>0):
for hd in holidays:
if(hd.HolidayId==6):
getsum=getsum+hd.WorkTime
#print("sum:",getsum,hd.HolidayName)
return getsum
def getHolidayOvertime(self,holidays):
"""
返回加班数
:param holidays:
:return:
"""
getsum=0
if(len(holidays)>0):
for hd in holidays:
if(hd.HolidayId==4):
getsum=getsum+hd.WorkTime
#print("sum:",getsum,hd.HolidayName)
return getsum
def getHolidayDeferred(self,holidays):
"""
返回補休数
:param holidays:
:return:
"""
getsum=0
if(len(holidays)>0):
for hd in holidays:
if(hd.HolidayId==3):
getsum=getsum+hd.WorkTime
#print("sum:",getsum,hd.HolidayName)
return getsum
def getEmpleeIdAnnualLeave(self, id:int,listEmp:list):
"""
年假ID
:param self:
:param listEmp:
:return:
"""
annual=0
#print(listEmp)
for sd in listEmp:
#print(sd[0],sd[1])
if(sd[0]==id):
#print(sd[1])
annual=annual+sd[1]
return annual
def getEmpleeIdOvertime(self, id:int,listEmp:list):
"""
加班时间ID
:param self:
:param listEmp:
:return:
"""
annual=0
#print(listEmp)
for sd in listEmp:
#print(sd[0],sd[1])
if(sd[0]==id):
#print(sd[1])
annual=annual+sd[1]
return annual
def getEmpleeIdeferred(self, id:int,listEmp:list):
"""
调休时间ID
:param self:
:param listEmp:
:return:
"""
annual=0
#print(listEmp)
for sd in listEmp:
#print(sd[0],sd[1])
if(sd[0]==id):
#print(sd[1])
annual=annual+sd[1]
return annual
# This is a sample Python script.
# encoding: utf-8
# 版权所有 2023 涂聚文有限公司
# 许可信息查看:
# 描述:
# Author : geovindu,Geovin Du 涂聚文.
# IDE : PyCharm 2023.1 python 311
# Datetime : 2023/9/2 20:20
# User : geovindu
# Product : PyCharm
# Project : LukfookLeaveCalculation
# File : main.py
# explain : 学习
# Press Shift+F10 to execute it or replace it with your code.
# Press Double Shift to search everywhere for classes, files, tool windows, actions, and settings.
import xlrd
import xlwt
import xlwings as xw
import xlsxwriter
import openpyxl as openws
import pandas as pd
import numpy as np
import pandasql
import os
import sys
import Common.Utils
import BLL.EmpLoyeeHolidaysGet
import Model.Employee
import Model.HolidayList
from openpyxl import load_workbook
def print_hi(name):
# Use a breakpoint in the code line below to debug your script.
print(f'Hi, {name}') # Press Ctrl+F8 to toggle the breakpoint.
# Press the green button in the gutter to run the script.
if __name__ == '__main__':
print_hi('PyCharm')
unstr=Common.Utils.Utils()
strtest="年假2小時 事假0.5小時" # 换行符
#strtest-strtest.replace('_x000D_', ' ', regex=True)
#strtest = "NaN"
spstr = strtest.split(" ")
thbll = BLL.EmpLoyeeHolidaysGet.EmpLoyeeHolidaysGet()
tt=thbll.getHolidays(spstr[0])
print("tt",tt[0].WorkTime,spstr)
dataframe1 = pd.read_excel('2023年7月.xlsx')
#print(dataframe1)
#for idx, data in dataframe1.iterrows():
#print("[{}]: {}".format(idx, data))
# 第1列为日期,其他列是员工对应的列的考勤
print("*************")
print(dataframe1.index)
print("*************")
print(dataframe1.shape) #行列
datarowcol=dataframe1.shape
print("行:",datarowcol[0],",列:",datarowcol[1])
excelRows=datarowcol[0]
excelColumns=datarowcol[1]
print("*********列标题****")
print(dataframe1.columns) #第一行标题 pandas.core.indexes.base.Index
titls=dataframe1.columns.to_list()
print(titls)
print("标题:",titls[1])
year=thbll.getYear(titls[1])
print("*************",year)
dmps = []
# 姓名,工号,序号,部门 0行至3行为员工资料 第一行为标题,不算行的内容 可以切片方式GET数据
#序号
idlist =[]
dataId = dataframe1.loc[0:0]
for idx, datadd in dataId.iterrows():
print("[{}]: {}".format(idx, datadd))
idlist=datadd.to_list()
for idd in range(1,len(idlist)):
#employvee=Model.Employee.employee()
#employvee.EmployeeId=idd
#dmps.append(employvee)
print(idd)
print("*******序号******")
#工号
nolist=[]
dataNo = dataframe1.loc[1:1]
for idx, dataoo in dataNo.iterrows():
print("[{}]: {}".format(idx, dataoo))
nolist=dataoo.to_list()
print("*******工号******")
#部门
deplist=[]
dataDep = dataframe1.loc[2:2]
for idx, datapp in dataDep.iterrows():
print("[{}]: {}".format(idx, datapp))
deplist=datapp.to_list()
print("*******部门******")
#姓名
namelist=[]
dataName = dataframe1.loc[3:3]
for idx, datann in dataName.iterrows():
print("[{}]: {}".format(idx, datann))
namelist=datann.to_list()
print("*******姓名******")
for i in range(1,len(namelist)):
employvee = Model.Employee.employee()
employvee.EmployeeId=idlist[i]
employvee.EmployeeNo=nolist[i]
employvee.EmployeeName=namelist[i]
employvee.EmployeeDep=deplist[i]
dmps.append(employvee)
for ob in dmps:
print(ob.EmployeeId,ob.EmployeeNo,ob.EmployeeName,ob.EmployeeDep)
#data1 = dataframe1.loc[0:3]
#print(type(data1))
#print(data1)
#for idx, data in data1.iterrows():
#print("[{}]: {}".format(idx, data))
#print("*************")
AnnualLeave=0
Overtime=0
DeferredHoliday=0
SpecialLeave=0
PersonalLeave=0
SickLeave=0
ChildcareLeave=0
LateTime=0
RegularHoliday=0
# 内容
mon=0
day=0
getdate=[]
getHolidays=[]
readrows=(excelRows-1)-3 #索此值从零开始,所以总数减一,再减三行统计的
data4 = dataframe1.loc[4:readrows] #第四行开始内空
getrow=1
emplist=[]
for idx, datavalue in data4.iterrows():
#strnum=Common.Utils.Utils.getAnnualLeave(data)
employs = Model.Employee.employee()
print("[{}]: {}".format(idx, datavalue))
slist=datavalue.tolist()
hbll=BLL.EmpLoyeeHolidaysGet.EmpLoyeeHolidaysGet() #病假4小時_x000D_事假0.5小時 未处理
#print(slist) #这是读取第1列的日期数据
for i in range(0, 1):
strvalue = str(slist[i])
print("get value:", strvalue.replace('nan', ''), type(strvalue))
mon=hbll.getMonth(strvalue)
day=hbll.getDay(strvalue)
print("*******时间************",mon,day)
getdate.append(str(year)+'-'+str(mon)+'-'+str(day))
print(slist)
hid=1
for i in range(1,len(slist)):
getdd = Model.HolidayList.HolidayList()
getdd.HolidayDate = str(year) + '-' + str(mon) + '-' + str(day)
#strvalue=str(slist[i]).replace(r'\s+|\\n', ' ', regex=True) _x000D_
#isnan=np.isnan(slist[i])
#if(isnan):
strvalue = str(slist[i]).replace('_x000D_', ' ') # 规换单元格的换行符,否则处理不了正确数据
#strvalue = str(slist[i]).replace('nan', ' ')
#print("get value:",strvalue.replace('nan',''),type(strvalue))
strnums = hbll.getHolidays(strvalue) # float str
print("hid:",hid)
getdd.HolidayEmpId = hid
if(len(strnums)>0):
for sn in range(len(strnums)):
print("str:",strnums[sn].HolidayId,strnums[sn].HolidayName,strnums[sn].WorkTime)
if strnums[sn].HolidayId==6:
AnnualLeave=AnnualLeave+strnums[sn].WorkTime
if strnums[sn].HolidayId == 4:
Overtime=Overtime+strnums[sn].WorkTime
if strnums[sn].HolidayId == 3:
DeferredHoliday = DeferredHoliday + strnums[sn].WorkTime
if strnums[sn].HolidayId == 2:
SpecialLeave=SpecialLeave+strnums[sn].WorkTime
if strnums[sn].HolidayId == 5:
PersonalLeave=PersonalLeave+strnums[sn].WorkTime
if strnums[sn].HolidayId == 7:
SickLeave=SickLeave+strnums[sn].WorkTime
if strnums[sn].HolidayId == 8:
ChildcareLeave=ChildcareLeave+strnums[sn].WorkTime
if strnums[sn].HolidayId == 9:
LateTime=LateTime+strnums[sn].WorkTime
if strnums[sn].HolidayId == 1:
RegularHoliday=RegularHoliday+strnums[sn].WorkTime
getdd.Holdays = strnums
else:
print("0")
getdd.Holdays =[]
hid+=1
getHolidays.append((getdd))
employs.EmployeeHolidays=getHolidays
employs.EmployeeActiveDate=getdate
getrow+=1
# getHolidays.append(strnums)
print("类型:", type(datavalue))
emplist.append((employs))
flist=[]
getIdAnnualLeave=[]
fdata=('ID','工号','部门','姓名','日期','假期')
flist.append(fdata)
print("date:",getdate,len(getdate))
print(len(getHolidays))
#for dddd in emplist:
#print(dddd.EmployeeId,len(dddd.EmployeeHolidays)) #emplist[0].EmployeeHolidays:
for sd in emplist[0].EmployeeHolidays:
fdata=(sd.HolidayEmpId,thbll.getEmplee(dmps,sd.HolidayEmpId)[0],thbll.getEmplee(dmps,sd.HolidayEmpId)[1],thbll.getEmplee(dmps,sd.HolidayEmpId)[2],sd.HolidayDate,thbll.getHoliday(sd.Holdays))
flist.append(fdata)
#ananuaint=thbll.getEmpleeIdAnnualLeave(sd.HolidayEmpId,emplist[0].EmployeeHolidays)
#getIdAnnualLeave.append(ananuaint)
print(sd.HolidayEmpId,thbll.getEmplee(dmps,sd.HolidayEmpId)[0],thbll.getEmplee(dmps,sd.HolidayEmpId)[1],thbll.getEmplee(dmps,sd.HolidayEmpId)[2],sd.HolidayDate,thbll.getHoliday(sd.Holdays))
print("**************")
print(getIdAnnualLeave)
print("*************",year,"年",mon,"月","考勤合计*************")#,day,"日"
print("年假合计:",AnnualLeave,"小时,加班合计:",Overtime,"小时,补休合计:",DeferredHoliday,"小时,特假合计:",SpecialLeave,"小时")
print("事假合计",PersonalLeave,"小时,病假合计:",SickLeave,"小时,迟到合计:",LateTime,"分钟,育儿假合计:",ChildcareLeave,"小时")
print("例休:",RegularHoliday,"天")
print("*****************************************************************")
#年假
for sd in emplist[0].EmployeeHolidays:
ananuaint=[sd.HolidayEmpId,thbll.getHolidayAnnualLeave(sd.Holdays)]
getIdAnnualLeave.append(ananuaint)
print(getIdAnnualLeave)
dd=thbll.getEmpleeIdAnnualLeave(1,getIdAnnualLeave)
print("年假 id=1 sum:",dd)
#加班
getIDOvertimm=[]
for sd in emplist[0].EmployeeHolidays:
overtimeint=[sd.HolidayEmpId,thbll.getHolidayOvertime(sd.Holdays)]
getIDOvertimm.append(overtimeint)
print(getIDOvertimm)
dov=thbll.getEmpleeIdOvertime(1,getIDOvertimm)
print("加班 id=1 sum:",dov)
#补休
getIdDeferred=[]
for sd in emplist[0].EmployeeHolidays:
defint=[sd.HolidayEmpId,thbll.getHolidayDeferred(sd.Holdays)]
getIdDeferred.append(defint)
print(getIdDeferred)
doef=thbll.getEmpleeIdeferred(1,getIdDeferred)
print("补休 id=1 sum:",doef)
'''
'''
taum= []
tva=("名称","合计")
taum.append(tva)
tva=("年假", str(AnnualLeave) + "小时")
taum.append(tva)
tva=("加班",str(Overtime)+"小时")
taum.append(tva)
tva=("补休",str(DeferredHoliday)+"小时")
taum.append(tva)
tva=("特假",str(SpecialLeave)+"小时")
taum.append(tva)
tva=("事假",str(PersonalLeave)+"小时")
taum.append(tva)
tva=("病假",str(SickLeave)+"小时")
taum.append(tva)
tva=("迟到",str(LateTime)+"分钟")
taum.append(tva)
tva=("育儿假",str(ChildcareLeave)+"小时")
taum.append(tva)
tva=("例休",str(RegularHoliday)+"天")
taum.append(tva)
sheetname1 = str(year) + "年" + str(mon) + "月假期"
writer = pd.ExcelWriter(sheetname1+"方案.xlsx") # 这里是创建了可写入不同sheet的文件
text1 = pd.DataFrame(flist,columns=['ID','工号','部门','姓名','日期','假期'])
text1.to_excel(writer, sheet_name=sheetname1,header=0, index=False) # sheet命名为
sheetname2=str(year)+"年"+str(mon)+"月合计"
text2 = pd.DataFrame(taum) #,columns=['名称','合计']
text2.to_excel(writer, sheet_name=sheetname2, header=0, index=False) # sheet命名为
text3=dataframe1;
sheetname3=str(year) + "年" + str(mon) + "月明細"
text3.to_excel(writer,sheet_name=sheetname3, header=0, index=False)
#writer.sheets.update()
writer.close()
dataframe2 = openws.load_workbook("2023年7月.xlsx")
# Define variable to read sheet
sheet = dataframe2.active
setrow=datarowcol[0] #总共行
setcol=datarowcol[1] #总共列
#每个人的合计
for idd in range(1, len(idlist)):
sheet.cell(row=setrow-1, column=1+idd).value = str(thbll.getEmpleeIdAnnualLeave(idd,getIdAnnualLeave)) + "小时"
sheet.cell(row=setrow - 0, column=1+idd).value = str(thbll.getEmpleeIdOvertime(idd,getIDOvertimm))+"小时"
sheet.cell(row=setrow +1, column=1+idd).value = str(thbll.getEmpleeIdeferred(idd,getIdDeferred))+"小时"
#合部汇总
sheet.cell(row=setrow+2, column=1).value = "年假汇总"
sheet.cell(row=setrow +3, column=1).value = "加班汇总"
sheet.cell(row=setrow +4, column=1).value = "补休汇总"
sheet.cell(row=setrow+2, column=2).value = str(AnnualLeave) + "小时"
sheet.cell(row=setrow +3, column=2).value = str(Overtime)+"小时"
sheet.cell(row=setrow +4, column=2).value = str(DeferredHoliday)+"小时"
dataframe2.save(r'demo.xlsx')
# Iterate the loop to read the cell values
#for row in range(0, dataframe2.max_row):
#for col in dataframe2.iter_cols(1, dataframe2.max_column):
#print(col[row].value)
# a single cell
#v1 = xw.range("A1:A7").value
#v2 = xw.range("F5").value
#print("Result:", v1)
# https://www.geeksforgeeks.org/how-to-add-one-row-in-an-existing-pandas-dataframe/ 新添加一行
# See PyCharm help at https://www.jetbrains.com/help/pycharm/
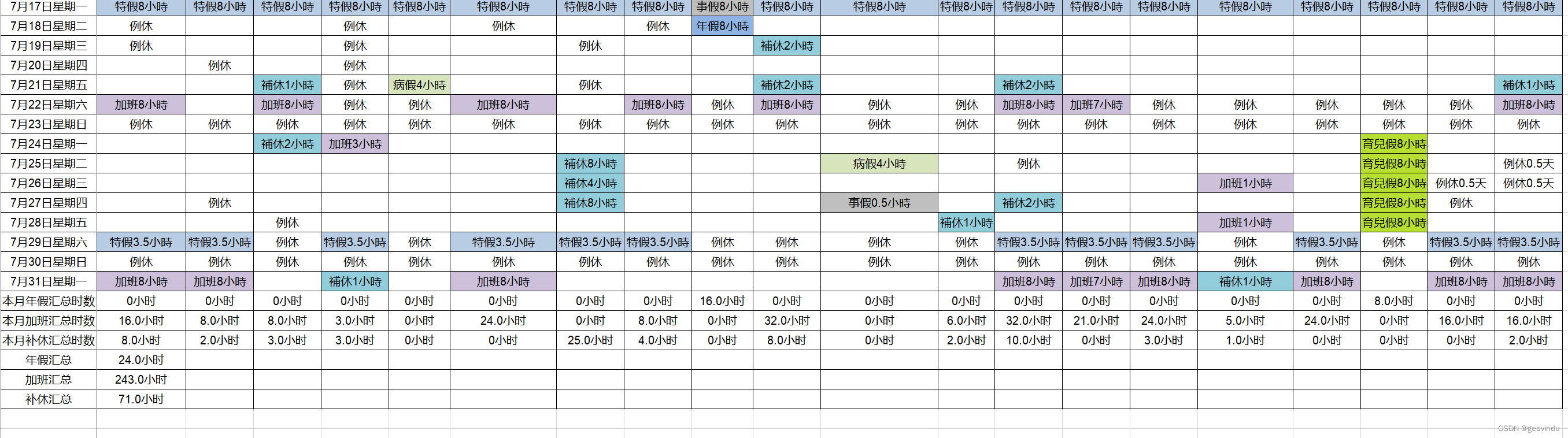