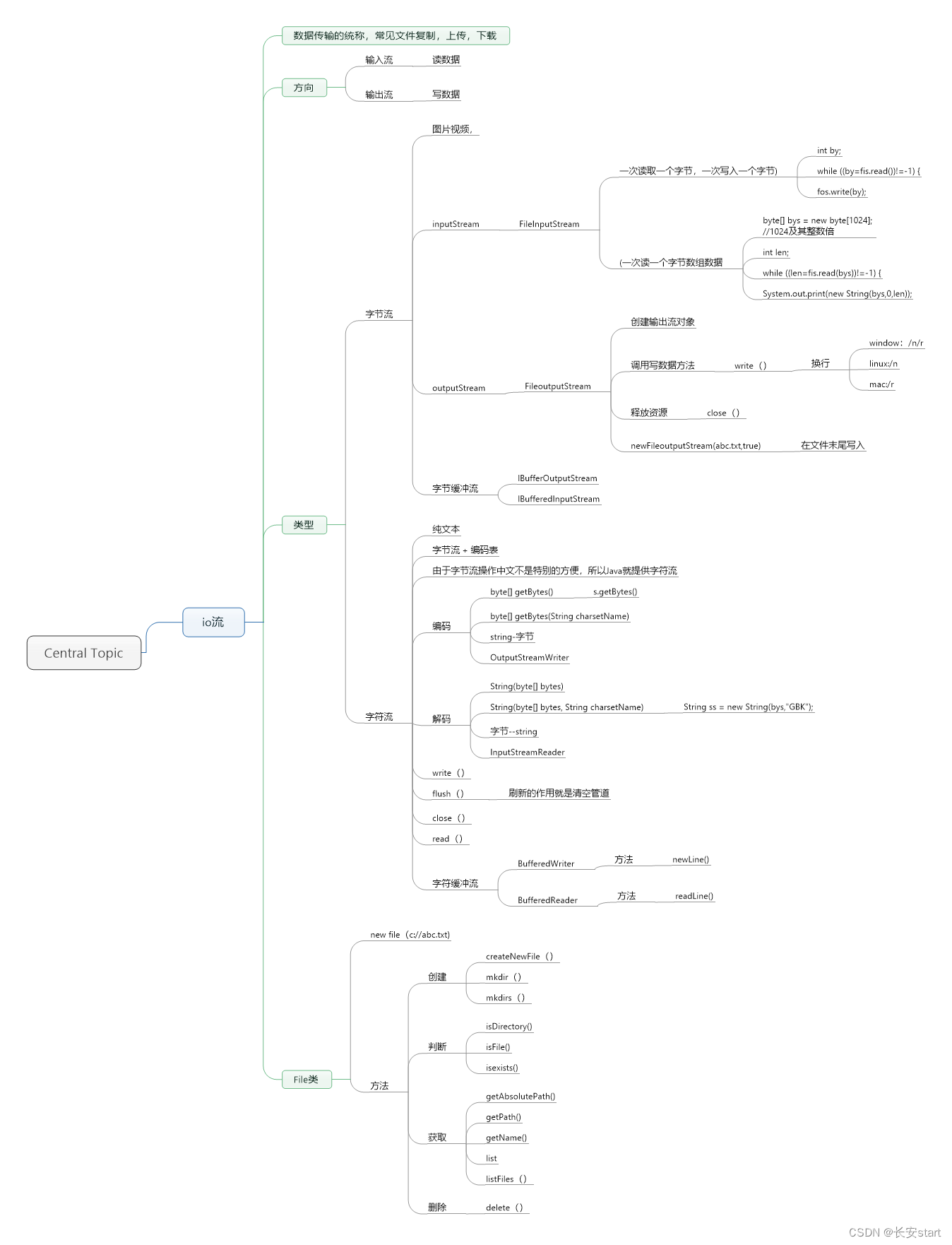
流
把内存中的数据写到硬盘或网络等或把硬盘或网络中的数据输入到内存的过程就是流。
流是抽象出来的概念,如同内存与硬盘之间的一个架起的一个管道,连通彼此,通过操作流控制数据的流入或流出,
流体系图
流的主要操作方法,
输入内存read(),内存输出到硬盘为write( ),和flush(),共有的方法close( ),close()方法中含有flush()方法,但最好push()一下
字节流读的时候,是一个字节一个字节读的,直到最后一个字节,read()返回的是读到的字节数据,读完之后返回-1
字节输入流FileInputStream
public class FileInputStreamss {
public static void main(String[] args) {
FileInputStream hao = null;
try {
//创建输入流
hao = new FileInputStream("src/javajinjie/ioliu/nihao.txt");
System.out.println(hao);
System.out.println(hao.getClass());
int b = 0;
for(int i = 0;i<3;i++){
b=hao.read();
System.out.print((char)b);
}
} catch (FileNotFoundException ee) {
ee.printStackTrace();
} catch (IOException aa) {
aa.printStackTrace();
}
}
字节输出流FileOutputStream
public class Fileoutss {
public static void main(String[] args) {
FileOutputStream ta = null;
try {
ta = new FileOutputStream("src/javajinjie/ioliu/one.txt");
ta.write(Integer.parseInt("123"));
}catch (NumberFormatException e) {
e.printStackTrace();
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
finally {
if(ta!=null){
try {
ta.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
字符流读的时候,是一次读一个字符,也就是2个字节,同样有read()方法,读完之后返回-1,
字符输入流FileReader
public class Filereadss {
public static void main(String[] args) {
Reader one = null;
try{
one = new FileReader("src/javajinjie/ioliu/one.txt");
int b = 0;
while((b = one.read()) !=-1){
System.out.print((char)b);
}
}catch(FileNotFoundException cc) {
cc.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
}
字符输出流FileWriter
public class Filewriter {
public static void main(String[] args) {
Writer two = null;
try {
two = new FileWriter("src/javajinjie/ioliu/two.txt");
two.write("小康zho小ng小康");
} catch (IOException e) {
e.printStackTrace();
}finally {
if(two != null){
try {
two.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
缓冲流
就是把读到,或写入的字节或字符先放到缓存中,然后一次写入硬盘或写入内存。目的就是减少硬盘的读取次数,提高效率。
注意bufferedReader中有ReadLine()方法,只是读取一行字符,读完之后返回null,要读取完整的文件内容,要判断读到最后的是否是“q”,“q”是读完整个文件返回的,bufferedWriter,提供了newline()可以写换行符。
字节输入缓冲流BufferedInputStream,字节输出缓冲流BufferedOutputStream
public class Huancunio {
public static void main(String[] args) {
BufferedInputStream bis = null;
BufferedOutputStream bos = null;
try {
bis = new BufferedInputStream(new FileInputStream("src/javajinjie/ioliu/nihao.txt"));
bos = new BufferedOutputStream(new FileOutputStream("src/javajinjie/ioliu/one.txt"));
int b = 0;
while((b=bis.read()) !=-1){
bos.write((char)b);
}
bos.flush();
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}finally{
try {
bis.close();
bos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
字符输入缓冲流FileReader
public class BufferedReaderTest01 {
public static void main(String[] args) throws Exception{
FileReader reader = new FileReader("C:\\Users\\linzi\\Desktop\\javalearn\\src\\io\\laodu_io\\io\\www.txt");
// 当一个流的构造方法中需要一个流的时候,这个被传进来的流叫做:节点流。
// 外部负责包装的这个流,叫做:包装流,还有一个名字叫做:处理流。
// 像当前这个程序来说:FileReader就是一个节点流。BufferedReader就是包装流/处理流。
BufferedReader br = new BufferedReader(reader);
// 读一行
/*String firstLine = br.readLine();
System.out.println(firstLine);
String secondLine = br.readLine();
System.out.println(secondLine);
String line3 = br.readLine();
System.out.println(line3);*/
// br.readLine()方法读取一个文本行,但不带换行符。
String s = null;
while((s = br.readLine()) != null){
System.out.print(s);
}
// 关闭流
// 对于包装流来说,只需要关闭最外层流就行,里面的节点流会自动关闭。(可以看源代码。)
br.close();
}
}
字符输出缓冲流BufferedWriter
public class BufferedWriterTest {
public static void main(String[] args) throws Exception{
// 带有缓冲区的字符输出流
BufferedWriter out = new BufferedWriter(new FileWriter("C:\\Users\\linzi\\Desktop\\javalearn\\src\\io\\laodu_io\\io\\eee.txt"));
// BufferedWriter out = new BufferedWriter(new OutputStreamWriter(new FileOutputStream("copy", true)));
// 开始写。
out.write("hello world!");
out.write("\n");
out.write("hello kitty!");
// 刷新
out.flush();
// 关闭最外层
out.close();
}
}
转换流
将字节流转换为字符流
字节输入流转换为字符输入流InputStreamReader
public class Zhuanhuanliu {
public static void main(String[] args) {
BufferedReader zinput = null;
try {
zinput = new BufferedReader(
new InputStreamReader(
new FileInputStream("src/javajinjie/ioliu/nihao.txt")));
// int b = 0;
// while((b=zinput.read()) !=-1){
// System.out.print((char)b);
// }
String ss = null;
while((ss=zinput.readLine()) != null){
System.out.println(ss);
}
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}finally{
if(zinput !=null) {
try {
zinput.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
字节输出流转为字符输出流OutputStreamWriter
public class Zhuanliuchu {
public static void main(String[] args) {
BufferedWriter bw = null;
try {
bw = new BufferedWriter(
new OutputStreamWriter(
new FileOutputStream("src/javajinjie/ioliu/two.txt")
)
);
bw.write("我们小康");
bw.newLine();
bw.write("qizhongguo");
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
bw.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
打印流 printStream,
system.out.println默认输入到控制台,如果system使用了printStream,—system.setout(new printStream(文件路径))就是输入到文件。
接收屏幕输入 inputStreamReader(system.in))
public class Jieshou {
public static void main(String[] args) {
BufferedReader br = null;
BufferedWriter bw = null;
try {
br = new BufferedReader(new InputStreamReader(System.in));
bw = new BufferedWriter(new FileWriter("src/javajinjie/ioliu/nihao.txt"));
String cc = null;
while ((cc =br.readLine()) != null) {
System.out.println(cc);
bw.write(cc);
bw.flush();
System.out.println();
}
}catch (IOException e) {
e.printStackTrace();
}finally {
if(br!=null){
try {
br.close();
bw.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
对象流
就是把内存中的对象写入硬盘或网络(序列化),或是把硬盘中的对象信息写入内存(反序列化)
要写入的对象必须实现serializable接口,这个接口是空的,实现了就表示可序列化。类实现了serializable接口后,类中变量如果不想被序列化,可用transient修饰。
当把内存中的对象写入硬盘时,系统会自动分配一个SerialVersionUID,此时如果把类中属性修改,系统会再分配一个serialVersionUID,造成不兼容,解决办法在类中设定 私有静态常量 private static final long serialVersionUID = -1111111111111111111L; 18 个1;
硬盘中的对象写入内存ObjectInputStream
public class Xuliein {
public static void main(String[] args) {
ObjectInputStream ois = null;
try {
ois = new ObjectInputStream(new FileInputStream("src/javajinjie/ioliu/xulie/du.txt"));
Father father = (Father) ois.readObject();
System.out.println(father.name);
} catch (IOException | ClassNotFoundException e) {
e.printStackTrace();
} finally {
try {
ois.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
内存中的对象写入硬盘ObjectOutputStream
public class Xulieout {
public static void main(String[] args) {
ObjectOutputStream oos = null;
try {
oos = new ObjectOutputStream(
new FileOutputStream("src/javajinjie/ioliu/xulie/du.txt"));
Father father = new Father();
father.name = "zhong";
oos.writeObject(father);
}catch(FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}finally{
try {
oos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
class Father implements Serializable {
// private static final long SerialVersionUID = -1111111411111111111L;
String name;
// int age;
}
File类
File 提供了大量的文件操作:删除文件,修改文件,得到文件修改日期,建立目录、列表文件等等,与io流无关,只是对文件操作
public class FileTest02 {
public static void main(String[] args) {
File f1 = new File("D:\\course\\01-开课\\开学典礼.ppt");
// 获取文件名
System.out.println("文件名:" + f1.getName());
// 判断是否是一个目录
System.out.println(f1.isDirectory()); // false
// 判断是否是一个文件
System.out.println(f1.isFile()); // true
// 获取文件最后一次修改时间
long haoMiao = f1.lastModified(); // 这个毫秒是从1970年到现在的总毫秒数。
// 将总毫秒数转换成日期?????
Date time = new Date(haoMiao);
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss SSS");
String strTime = sdf.format(time);
System.out.println(strTime);
// 获取文件大小
System.out.println(f1.length()); //216064字节。
}
}