1. 传统的错误处理
1.1 程序开发中常见的错误
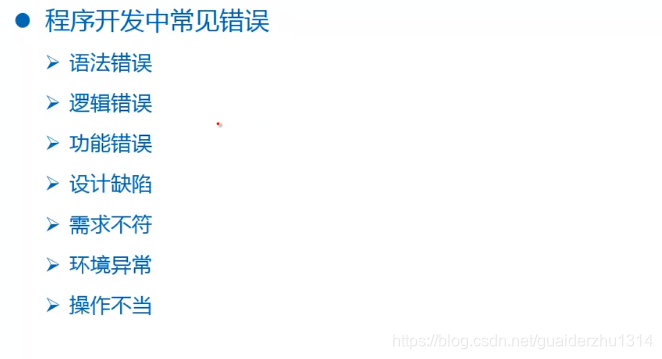
1.2 传统错误处理方式
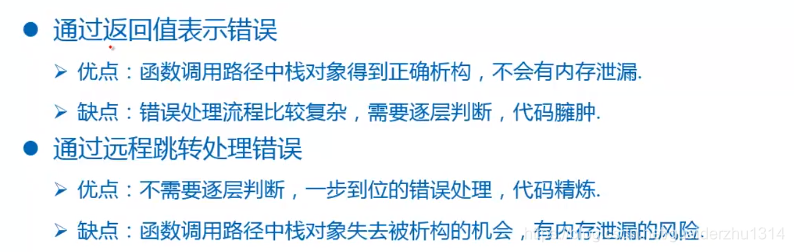
2. C++的异常机制
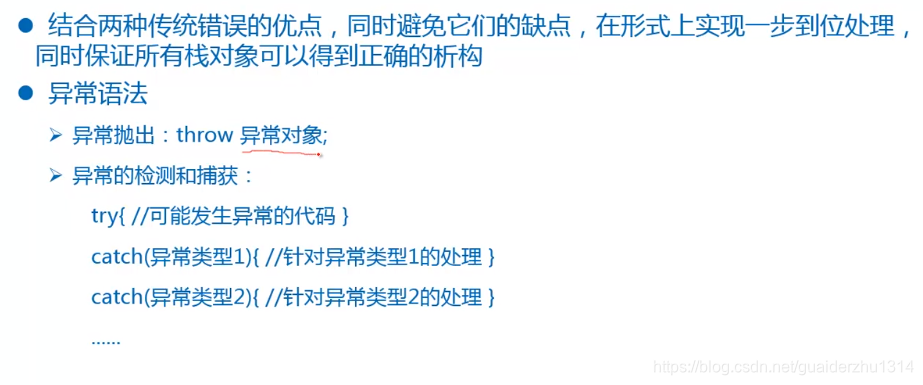
2.1 正常流程
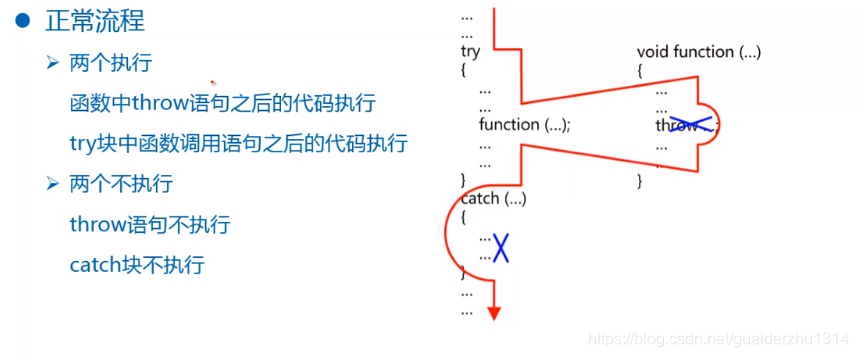
2.2 异常流程
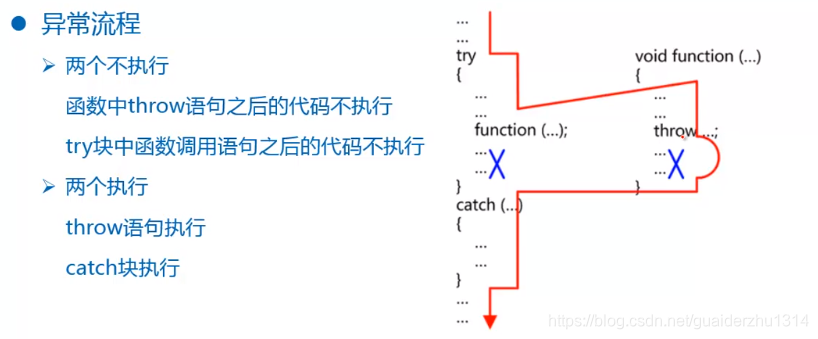
2.2.1 throw抛出基本类型:
#include <iostream>
#include <cstdio>
using namespace std;
class A{
public:
A(void){
cout << "A类构造" << endl;
}
~A(void){
cout << "A类析构" << endl;
}
};
int func3(void){
A a;
FILE *fp = fopen("x.txt", "r");
if(fp == NULL){
throw -1;
}
fclose(fp);
return 0;
}
int func2(void){
A a;
func3();
return 0;
}
int func1(void){
A a;
func2();
return 0;
}
int main(void){
try{
func1();
cout << "test" << endl;
}
catch(int ex){
cout << "文件打开失败" << endl;
return -1;
}
}
$ ./a.out
A类构造
A类构造
A类构造
A类析构
A类析构
A类析构
文件打开失败
2.2.2 throw抛出类类型:
#include <iostream>
#include <cstdio>
using namespace std;
class FileError{
public:
FileError(const string& file, int line):m_file(file), m_line(line){
cout <<"出错位置"<< m_file <<", 出错行号" << m_line << endl;
}
private:
string m_file;
int m_line;
};
class A{
public:
A(void){
cout << "A类构造" << endl;
}
~A(void){
cout << "A类析构" << endl;
}
};
int func3(void){
A a;
FILE *fp = fopen("x.txt", "r");
if(fp == NULL){
throw FileError(__FILE__, __LINE__);
throw -1;
}
fclose(fp);
return 0;
}
int func2(void){
A a;
func3();
return 0;
}
int func1(void){
A a;
func2();
return 0;
}
int main(void){
try{
func1();
cout << "test" << endl;
}
catch(int ex){
cout << "文件打开失败" << endl;
return -1;
}
catch(FileError& ex){
cout << "File open Error" << endl;
}
}
$ ./a.out
A类构造
A类构造
A类构造
出错位置a.cpp, 出错行号30
A类析构
A类析构
A类析构
File open Error
- 对于函数调用,只有知道函数中,throw会抛出的异常类型。才能去写catch语句, 所以就有了 函数的异常说明
3. 函数的异常说明
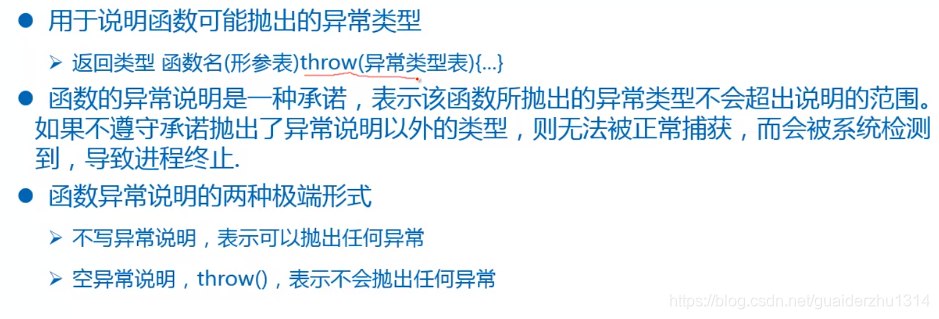
#include <iostream>
#include <cstdio>
using namespace std;
class FileError{};
class MemoryError{};
void func(void) throw (MemoryError){
throw MemoryError();
}
int main(void){
try{
func();
}
catch(MemoryError& ex){
cout << "MemoryError" << endl;
}
catch(FileError& ex){
cout << "FileError" << endl;
}
}
$ ./a.out
MemoryError
4. 标准异常类的说明
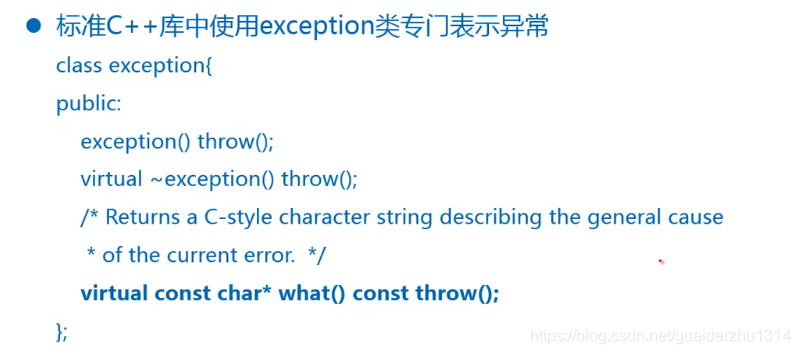
#include <iostream>
#include <cstdio>
using namespace std;
class FileError:public exception{
public:
const char* what() const throw(){
cout << "针对文件错误的处理" << endl;
return "FileError";
}
};
class MemoryError:public exception{
public:
const char* what() const throw(){
cout << "针对内存错误的处理" << endl;
return "MemoryError";
}
};
void func(void) throw (MemoryError, FileError){
throw MemoryError();
}
int main(void){
try{
func();
}
catch(exception& ex){
ex.what();
}
}
$ ./a.out
针对内存错误的处理