题目:paltform总线中通过名字、名字列表和设备树三种方式实现driver和device匹配
方式一>>>名字:
#include <linux/init.h>
#include <linux/module.h>
#include <linux/of.h>
#include <linux/platform_device.h>
//对设备信息填充
struct resource res[]={
[0]={
.start = 0x12345678,
.end = 0x12345678+49,
.flags = IORESOURCE_MEM,
},
[1]={
.start = 71,
.end = 71,
.flags = IORESOURCE_IRQ,
},
};
//定义release函数
void pdev_release(struct device *dev)
{
printk("%s:%d\n",__func__,__LINE__);
}
//给对象赋值
struct platform_device pdev={
.name = "hello2",
.id = PLATFORM_DEVID_AUTO,
.dev = {
.release = pdev_release,
},
.resource = res,
.num_resources = ARRAY_SIZE(res),
};
static int __init mycdev_init(void)
{
platform_device_register(&pdev);
return 0;
}
static void __exit mycdev_exit(void)
{
//注销
platform_device_unregister(&pdev);
}
module_init(mycdev_init);
module_exit(mycdev_exit);
MODULE_LICENSE("GPL");
#include <linux/init.h>
#include <linux/module.h>
#include <linux/of.h>
#include <linux/platform_device.h>
struct resource *res;
int irqno;
//定义probe函数
int pdrv_probe(struct platform_device *pdev)
{
printk("%s\n",pdev->name);
//获取MEM类型资源
res = platform_get_resource(pdev,IORESOURCE_MEM,0);
if(res==NULL)
{
printk("获取men资源失败\n");
return ENODATA;
}
//获取中断类型资源
irqno = platform_get_irq(pdev,0);
if(irqno<0)
{
printk("获取中断资源失败\n");
return ENODATA;
}
printk("ADDR:%#llx,irqno:%d\n",res->start,irqno);
return 0;
}
//定义re函数
int pdrv_remove(struct platform_device *pdev)
{
printk("%s:%d\n",__func__,__LINE__);
return 0;
}
//给对象初始化
struct platform_driver pdrv={
.probe = pdrv_probe,
.remove = pdrv_remove,
.driver ={
.name = "abc",
},
};
module_platform_driver(pdrv);
MODULE_LICENSE("GPL");
测试结果: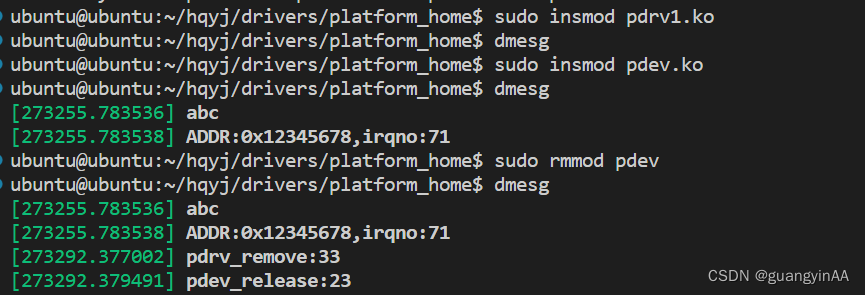
方式二>>>名字列表
#include <linux/init.h>
#include <linux/module.h>
#include <linux/of.h>
#include <linux/platform_device.h>
struct resource *res;
int irqno;
//定义probe函数
int pdrv_probe(struct platform_device *pdev)
{
printk("%s\n",pdev->name);
//获取MEM类型资源
res = platform_get_resource(pdev,IORESOURCE_MEM,0);
if(res==NULL)
{
printk("获取men资源失败\n");
return ENODATA;
}
//获取中断类型资源
irqno = platform_get_irq(pdev,0);
if(irqno<0)
{
printk("获取中断资源失败\n");
return ENODATA;
}
printk("ADDR:%#llx,irqno:%d\n",res->start,irqno);
return 0;
}
//定义re函数
int pdrv_remove(struct platform_device *pdev)
{
printk("%s:%d\n",__func__,__LINE__);
return 0;
}
struct platform_device_id idtable[]={
{"hello1",0},
{"hello2",1},
{"hello3",2},
{},
};
MODULE_DEVICE_TABLE(platform,idtable);
//给对象初始化
struct platform_driver pdrv={
.probe = pdrv_probe,
.remove = pdrv_remove,
.driver ={
.name = "abc",
},
.id_table = idtable,
};
module_platform_driver(pdrv);
MODULE_LICENSE("GPL");
测试结果: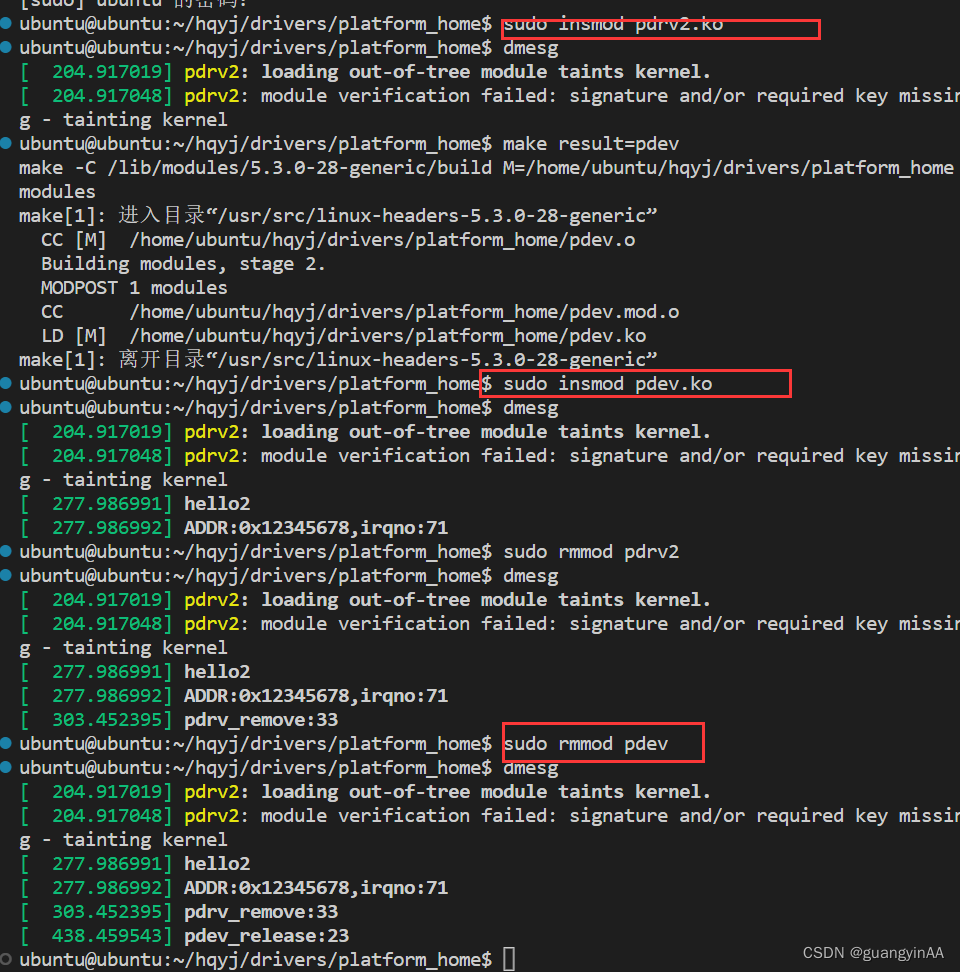
方式三>>>设备树
#include <linux/init.h>
#include <linux/module.h>
#include <linux/of.h>
#include <linux/platform_device.h>
#include <linux/of_gpio.h>
#include <linux/mod_devicetable.h>
struct resource *res;
int irqno;
struct gpio_desc *gpiono;
//定义probe函数
int pdrv_probe(struct platform_device *pdev)
{
printk("%s\n", pdev->name);
//获取MEM类型资源
res = platform_get_resource(pdev, IORESOURCE_MEM, 0);
if (res == NULL)
{
printk("获取men资源失败\n");
return ENODATA;
}
//获取中断类型资源
irqno = platform_get_irq(pdev, 0);
if (irqno < 0)
{
printk("获取中断资源失败\n");
return ENODATA;
}
printk("ADDR:%#x,irqno:%d\n", res->start, irqno);
//通过多重结构体获取GPIO编号
gpiono = gpiod_get_from_of_node(pdev->dev.of_node, "myled1", 0, GPIOD_OUT_HIGH, NULL);
if (IS_ERR(gpiono))
{
printk("获取gpio编号失败\n");
return PTR_ERR(gpiono);
}
printk("申请GPIO编号成功\n");
gpiod_set_value(gpiono, 1);
return 0;
}
//定义re函数
int pdrv_remove(struct platform_device *pdev)
{
gpiod_set_value(gpiono, 0);
gpiod_put(gpiono);
printk("%s:%d\n", __func__, __LINE__);
return 0;
}
//构建compatible表
struct of_device_id oftable[] = {
{.compatible = "hqyj,platform"},
{}
};
//热插拔宏
MODULE_DEVICE_TABLE(of, oftable);
//给对象初始化
struct platform_driver pdrv = {
.probe = pdrv_probe,
.remove = pdrv_remove,
.driver = {
.name = "abc",
.of_match_table = oftable,
},
};
module_platform_driver(pdrv);
MODULE_LICENSE("GPL");
测试结果: