首先你要下载一个AFNetworking框架,不好意思这里没链接^_^
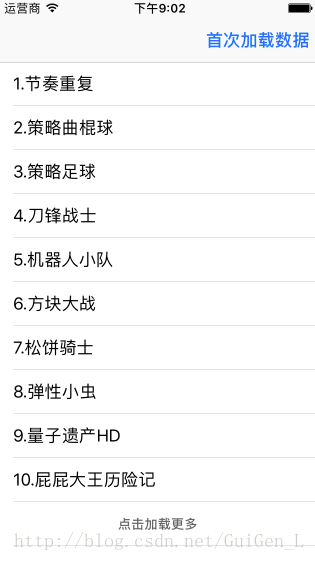
上拉下加载下一页
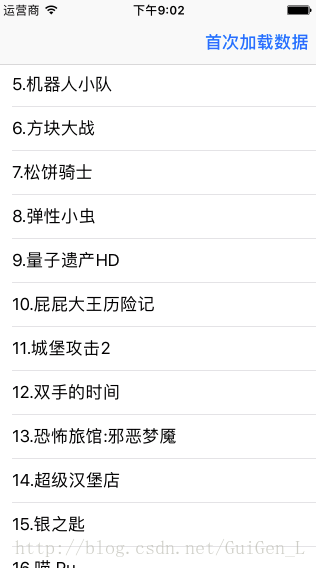
我封装的MyAFNetworking
#import <Foundation/Foundation.h>
#import "AFHTTPRequestOperationManager.h"
typedef void (^FinishBlock)(id dataString);
typedef void (^ErrorBlock)(NSError* error);
@interface MyAFNetworking : NSObject
@property (strong, nonatomic) FinishBlock finishBlock;
@property (strong, nonatomic) ErrorBlock errorBlock;
+(void)getUrlStr:(NSString*)urlStr finish:(FinishBlock)finish andError:(ErrorBlock)errors;
+(void)postUrlStr1:(NSString*)urlSte1 postDic:(NSMutableDictionary*)dic finish1:(FinishBlock)finish1 andError:(ErrorBlock)errors;
@end
#import "MyAFNetworking.h"
@implementation MyAFNetworking
+(void)getUrlStr:(NSString*)urlStr finish:(FinishBlock)finish andError:(ErrorBlock)errors
{
AFHTTPRequestOperationManager* manger = [AFHTTPRequestOperationManager manager];
manger.responseSerializer = [AFHTTPResponseSerializer serializer];
[manger GET:urlStr parameters:nil success:^(AFHTTPRequestOperation *operation, id responseObject) {
finish(responseObject);
} failure:^(AFHTTPRequestOperation *operation, NSError *error) {
errors(error);
}];
}
+(void)postUrlStr1:(NSString*)urlSte1 postDic:(NSMutableDictionary*)dic finish1:(FinishBlock)finish1 andError:(ErrorBlock)errors
{
AFHTTPRequestOperationManager* manger = [AFHTTPRequestOperationManager manager];
manger.responseSerializer = [AFHTTPResponseSerializer serializer];
[manger POST:urlSte1 parameters:dic success:^(AFHTTPRequestOperation *operation, id responseObject) {
finish1(responseObject);
} failure:^(AFHTTPRequestOperation *operation, NSError *error) {
errors(error);
}];
}
@end
ViewController.m中
#import "ViewController.h"
#import "AFHTTPRequestOperationManager.h"
#import "MJRefresh.h"
#import "First_ViewController.h"
#import "MyAFNetworking.h"
#define AppURL @"http://iappfree.candou.com:8080/free/applications/limited?currency=rmb&page=%ld"
@interface ViewController ()<UITableViewDelegate,UITableViewDataSource>
@property (retain) UITableView* tableView;
@property (retain) NSMutableArray* dataSource;
@property (assign) NSInteger page;
@end
@implementation ViewController
- (void)viewDidLoad {
[super viewDidLoad];
self.view.backgroundColor = [UIColor whiteColor];
_page=1;
self.automaticallyAdjustsScrollViewInsets = NO;
_dataSource = [NSMutableArray new];
_tableView = [[UITableView alloc]initWithFrame:CGRectMake(0, 64, [UIScreen mainScreen].bounds.size.width, [UIScreen mainScreen].bounds.size.height-64) style:UITableViewStylePlain];
_tableView.delegate = self;
_tableView.dataSource = self;
[self.view addSubview:_tableView];
self.navigationItem.rightBarButtonItem = [[UIBarButtonItem alloc]initWithTitle:@"首次加载数据" style:UIBarButtonItemStyleDone target:self action:@selector(rightAction:)];
[self rightAction:nil];
__weak __typeof(&*self)weakSelf = self;
[_tableView addLegendHeaderWithRefreshingBlock:^{
_page=1;
[weakSelf.tableView.header beginRefreshing];
[weakSelf rightAction:nil];
}];
[_tableView addLegendFooterWithRefreshingBlock:^{
weakSelf.page++;
[weakSelf.tableView.footer beginRefreshing];
[weakSelf rightAction:nil];
}];
}
-(void)rightAction:(UIBarButtonItem*)sender
{
if (sender) {
_page = 1;
}
NSLog(@"首次加载");
[MyAFNetworking getUrlStr:[NSString stringWithFormat:AppURL,_page] finish:^(id dataString) {
NSDictionary* dic = [NSJSONSerialization JSONObjectWithData:dataString options:NSJSONReadingMutableContainers error:nil];
NSLog(@"========dic:%@", dic);
if (_page==1) {
[_dataSource removeAllObjects];
}
[_dataSource addObjectsFromArray:[dic objectForKey:@"applications"]];
[_tableView reloadData];
[_tableView.header endRefreshing];
[_tableView.footer endRefreshing];
} andError:^(NSError *error) {
NSLog(@"========error:%@", error);
}];
}
#pragma mark - 表格视图协议
-(NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section
{
return _dataSource.count;
}
- (UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath
{
NSLog(@"哈哈哈哈哈哈哈哈");
static NSString* cellID = @"cell";
UITableViewCell* cell = [tableView dequeueReusableCellWithIdentifier:cellID];
if (cell == nil) {
cell = [[UITableViewCell alloc]initWithStyle:UITableViewCellStyleDefault reuseIdentifier:cellID];
}
NSDictionary* dic = _dataSource[indexPath.row];
NSLog(@"====%@====",dic);
cell.textLabel.text = [NSString stringWithFormat:@"%ld.%@",indexPath.row+1,[dic objectForKey:@"name"]];
return cell;
}
-(void)tableView:(UITableView *)tableView didSelectRowAtIndexPath:(NSIndexPath *)indexPath
{
[tableView deselectRowAtIndexPath:indexPath animated:YES];
First_ViewController* firstVC = [[First_ViewController alloc]init];
[self.navigationController pushViewController:firstVC animated:YES];
}
- (void)didReceiveMemoryWarning {
[super didReceiveMemoryWarning];
}
@end
First_ViewController.m中
#import "First_ViewController.h"
#import "AFHTTPRequestOperationManager.h"
#import "MyAFNetworking.h"
#define AppURL @"http://api.cy.daoyoudao.com/app/dishlist.do"
@interface First_ViewController ()<UITableViewDelegate,UITableViewDataSource>
@property (retain) UITableView* tableView;
@property (retain) NSMutableArray* dataSource;
@end
@implementation First_ViewController
- (void)viewDidLoad {
[super viewDidLoad];
self.view.backgroundColor = [UIColor whiteColor];
_dataSource = [NSMutableArray new];
self.automaticallyAdjustsScrollViewInsets = NO;
_tableView = [[UITableView alloc]initWithFrame:CGRectMake(0, 64, [UIScreen mainScreen].bounds.size.width, [UIScreen mainScreen].bounds.size.height-64) style:UITableViewStylePlain];
_tableView.delegate = self;
_tableView.dataSource = self;
[self.view addSubview:_tableView];
[self downLoadData];
}
-(void)downLoadData
{
NSMutableDictionary* dataDic = [NSMutableDictionary new];
[dataDic setValue:@"100" forKey:@"apkversion"];
[dataDic setValue:@"醉江月" forKey:@"appname"];
[dataDic setValue:@"v2.2.1.0703" forKey:@"appversion"];
[dataDic setValue:@"670" forKey:@"categorycode"];
[dataDic setValue:@"33285601411781238031" forKey:@"clientid"];
[dataDic setValue:@"1" forKey:@"curpage"];
[dataDic setValue:@"0" forKey:@"filtertype"];
[dataDic setValue:@"0" forKey:@"filtervalue"];
[dataDic setValue:@"10986" forKey:@"groupid"];
[dataDic setValue:@"864502023192438" forKey:@"imei"];
[dataDic setValue:@"460001491957881" forKey:@"imsi"];
[dataDic setValue:@"com.dd.zjyue" forKey:@"packagename"];
[dataDic setValue:@"15" forKey:@"pagesize"];
[dataDic setValue:@"720*1280" forKey:@"resolution"];
[dataDic setValue:@"11814" forKey:@"shopid"];
[dataDic setValue:@"4f891256" forKey:@"signature"];
[dataDic setValue:@"0" forKey:@"unpackid"];
[dataDic setValue:@"a_4.4.2" forKey:@"versionrelease"];
[dataDic setValue:@"116.337033,40.045998" forKey:@"xylocation"];
[MyAFNetworking postUrlStr1:AppURL postDic:dataDic finish1:^(id dataString) {
NSDictionary* dic = [NSJSONSerialization JSONObjectWithData:dataString options:NSJSONReadingMutableContainers error:nil];
[_dataSource addObjectsFromArray:[dic objectForKey:@"getDishList"]];
[_tableView reloadData];
NSLog(@"===========dic===========:%@", dic);
} andError:^(NSError *error) {
NSLog(@"==error:%@", error);
}];
}
#pragma mark - 表格协议
-(NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section
{
return _dataSource.count;
}
-(UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath
{
static NSString* cellID = @"cell";
UITableViewCell* cell = [tableView dequeueReusableCellWithIdentifier:cellID];
if (cell == nil) {
cell = [[UITableViewCell alloc]initWithStyle:UITableViewCellStyleDefault reuseIdentifier:cellID];
}
NSDictionary* dic = _dataSource[indexPath.row];
NSLog(@"====%@====",dic);
cell.textLabel.text = [dic objectForKey:@"productinfo"];
return cell;
}
- (void)didReceiveMemoryWarning {
[super didReceiveMemoryWarning];
}
@end
谢谢大家看到我博客,新手多多关照