定义需要动态创建的组件-MessageComponent
<!-- message.component.html -->
<div>
<h4>message组件</h4>
<h5>类型: {{type}}</h5>
<h5>消息: {{message}}</h5>
</div>
<hr>
// message.component.ts
import { Component, OnInit } from '@angular/core';
@Component({
selector: 'app-message',
templateUrl: './message.component.html',
styleUrls: ['./message.component.less']
})
export class MessageComponent implements OnInit {
type = ''
message = ''
constructor() { }
ngOnInit(): void {
}
}
定义一个容器组件ContainerComponent
,用来存放动态生成的MessageComponent
组件。ng-template
是动态加载组件的最佳选择,因为它不会渲染任何额外的输出。
<!-- container.component.html -->
<div>
<h3>我是容器</h3>
<ng-template #messageContainer></ng-template>
</div>
<button (click)="createComponent()">新建</button>
<button (click)="clearComponent()">清空</button>
使用@ViewChild
获取具体的容器,使用read: ViewContainerRef
将其定义为视图容器类型。
@ViewChild('messageContainer', {read: ViewContainerRef}) container: ViewContainerRef | any
ViewContainerRef
是可以将一个或多个视图附着到组件中的容器。其createComponent
方法用来实例化一个 Component
并把它的宿主视图插入到本容器的指定 index
处。如果没有指定index
,就把新的视图追加到最后。第一个参数componentFactory
为必选参数,为ComponentFactory
的实例。
abstract class ViewContainerRef {
...
abstract createComponent<C>(componentFactory: ComponentFactory<C>, index?: number, injector?: Injector, projectableNodes?: any[][], ngModule?: NgModuleRef<any>): ComponentRef<C>
...
}
ComponentFactoryResolver
是一个服务,其resolveComponentFactory
方法能创建给定类型的组件的工厂对象ComponentFactory
,这个工厂对象可用于创建组件的实例。
constructor(private resolver: ComponentFactoryResolver) { }
const factory: ComponentFactory<MessageComponent> = this.resolver.resolveComponentFactory(MessageComponent)
这样,container
这个实例就可以使用createComponent
方法来创建组件。
const component: ComponentRef<MessageComponent> = this.container.createComponent(factory)
完整container.component.ts
代码
// container.component.ts
import { Component, OnInit, ViewChild, ViewContainerRef, ComponentFactoryResolver, ComponentFactory, ComponentRef } from '@angular/core';
import { MessageComponent } from '../message/message.component';
@Component({
selector: 'app-container',
templateUrl: './container.component.html',
styleUrls: ['./container.component.less']
})
export class ContainerComponent implements OnInit {
@ViewChild('messageContainer', {read: ViewContainerRef}) container: ViewContainerRef | any
constructor(private resolver: ComponentFactoryResolver) { }
count = 1
// 创建组件
createComponent() {
const factory: ComponentFactory<MessageComponent> = this.resolver.resolveComponentFactory(MessageComponent)
const component: ComponentRef<MessageComponent> = this.container.createComponent(factory)
console.log('component', component)
component.instance.type = `type${this.count++}` // 这里设置动态创建组件的属性值
component.instance.message = 'hello world!'
}
// 清空组件
clearComponent() {
this.count = 1
this.container.clear()
}
ngOnInit(): void {
}
}
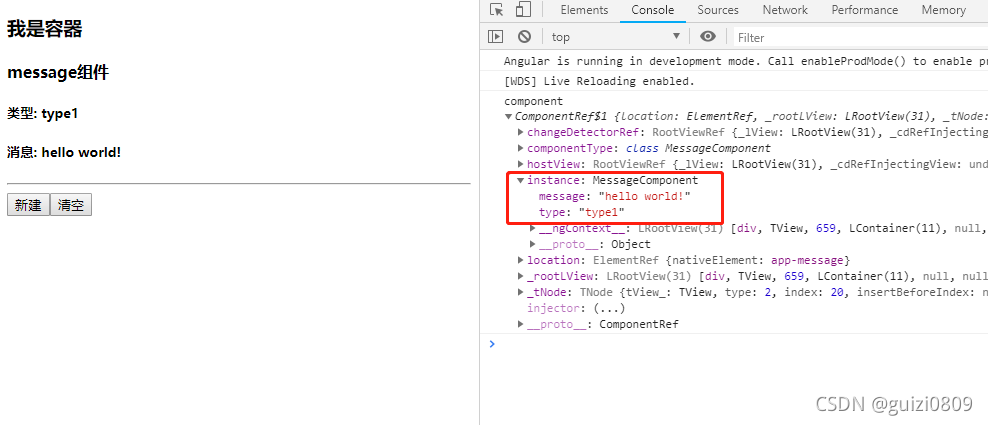