#include <iostream>
#include <vector>
using namespace std;
enum imageType
{
LSAT,
SPOT
};
class Image{
public:
virtual void draw() = 0;
static Image* findAndClone(imageType);
protected:
virtual imageType returnType() = 0;
virtual Image *clone() = 0;
static void addPrototype(Image *image)
{
_prototypes[_nextSlot++] = image;
}
private:
static Image* _prototypes[10];
static int _nextSlot;
};
Image* Image::_prototypes[];
int Image::_nextSlot;
Image* Image::findAndClone(imageType type)
{
for(int i = 0; i < _nextSlot; i++)
if(_prototypes[i]->returnType() == type)
return _prototypes[i]->clone();
}
class LandSatImage : public Image
{
public:
imageType returnType() override{
return LSAT;
}
void draw() override{
cout << "LandSatImage::draw" << endl;
}
Image * clone() override{
return new LandSatImage(1);
}
protected:
LandSatImage(int dummy) {
_id = _count++;
}
private:
static LandSatImage _landSatImage;
LandSatImage(){
addPrototype(this);
}
int _id;
static int _count;
};
LandSatImage LandSatImage::_landSatImage;
int LandSatImage::_count = 1;
int main()
{
return 0;
}
prototype pattern原型模式
于 2023-05-15 03:21:29 首次发布
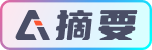