一:ajax请求页面数据
1:准备工作
(1)Controller层增加
@RequestMapping("getjson")
@ResponseBody
public News getjson(){
News news = new News();
news.setTitle("新闻标题");
news.setContent("新闻内容");
return news;
}
(2)html页面修改
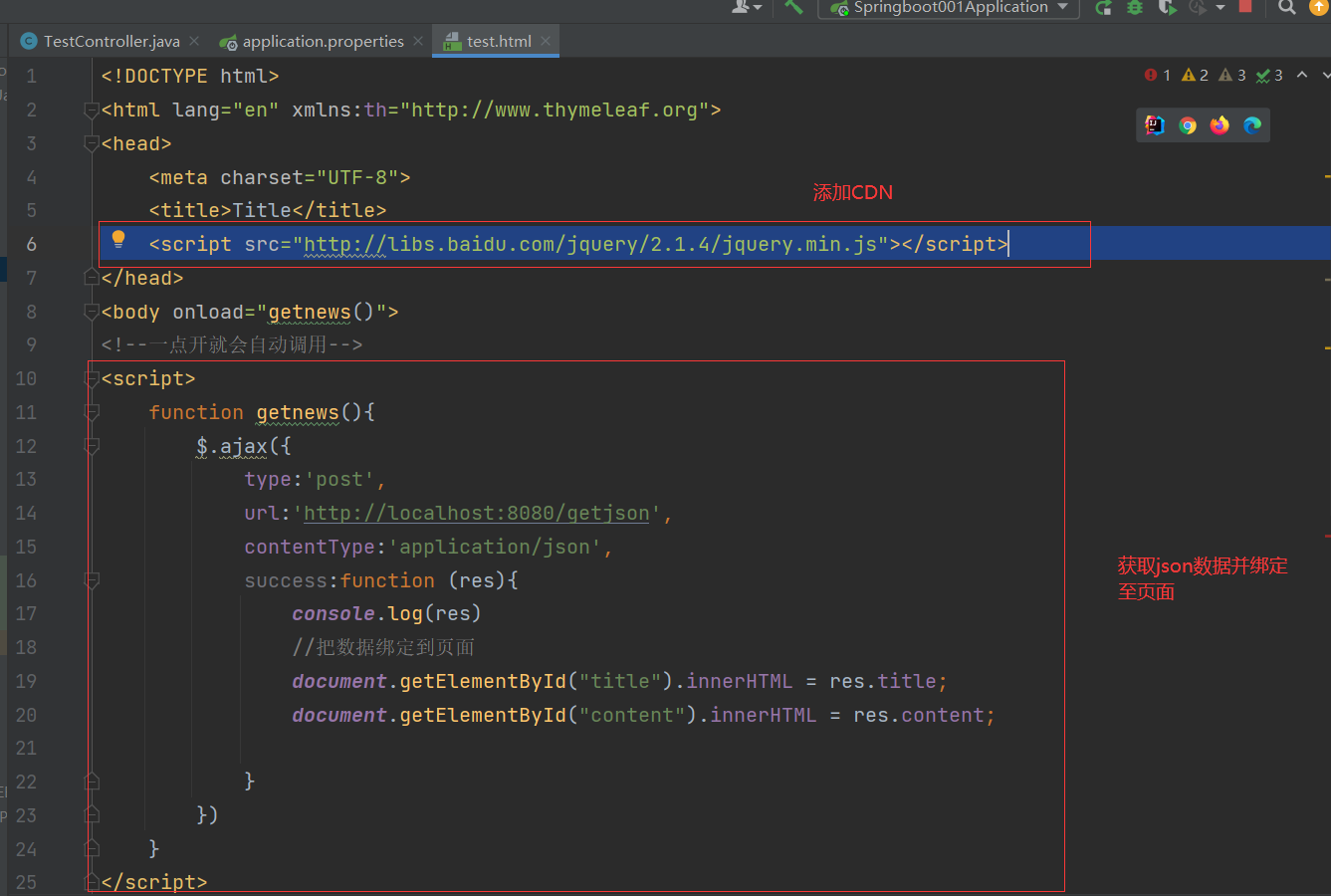
此时html完整代码如下
test.html
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>Title</title>
<script src="http://libs.baidu.com/jquery/2.1.4/jquery.min.js"></script>
</head>
<body onload="getnews()">
<!--一点开就会自动调用-->
<script>
function getnews(){
$.ajax({
type:'post',
url:'http://localhost:8080/getjson',
contentType:'application/json',
success:function (res){
console.log(res)
//把数据绑定到页面
document.getElementById("title").innerHTML = res.title;
document.getElementById("content").innerHTML = res.content;
}
})
}
</script>
<h1 th:text="${message}">hello world</h1>
<h2>这是一个html文件</h2>
<div id="title">title</div>
<div id="content">content</div>
<button onclick="getnews()">获取新闻</button>
<!--<div th:each="news:${newsList}">-->
<!--<!– 取别名 给newsList取名为news–>-->
<!-- <div th:text="${news.title}"></div>-->
<!-- <div th:text="${news.content}"></div>-->
<!--</div>-->
</body>
</html>
2:ajax和常规方法访问的区别
最主要的区别是访问的 先后顺序。
(1)常规方法进行访问
浏览器会先访问Controller层,然后Controller层处理后把数据传给Web层并填充好返回html给浏览器
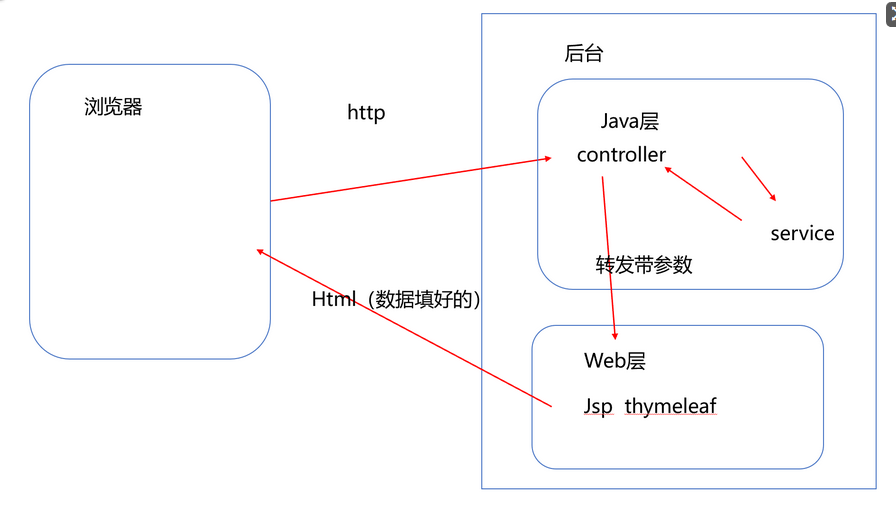
(2)ajax方法进行访问
使用ajax方法访问会先获得html页面的静态资源放回给浏览器,然后再访问Controller层获得数据进行填充。
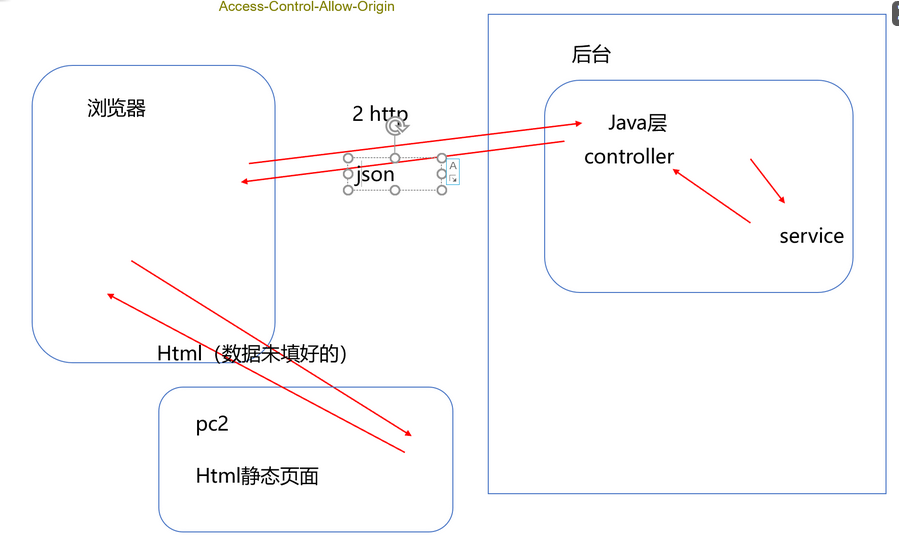
ajax这种工作方式使得前后端分离,被称为 前后端分离技术 前端和后台可以存在于 不同的服务器
也是目前主流的工作模式。
但这种工作方式会出现Access-Control-Allow-Origin的问题(跨域问题)
就是访问后台时,服务器发现域名不一致(不同的服务器),禁止访问里面的方法来获得数据,所以浏览器只会得到静态页面而不会获得数据。
解决方法是在Controller层添加注解,解除跨域的限制
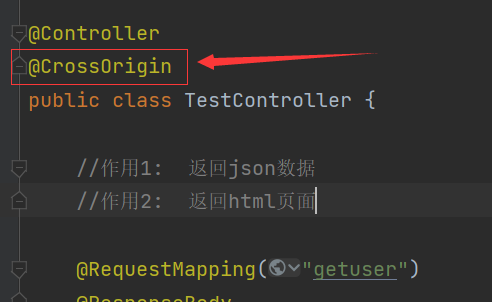
然后就可以跨域进行访问了
(3)ajaxa实际案例
在Controller层添加语句
@RequestMapping("commit")
@ResponseBody
public Map<String,Object> commit(String username, String password){
System.out.println("username");
System.out.println("password");
Map<String,Object>result = new HashMap<>();
if(username.equals("admin")&&password.equals("123456")){
result.put("code",0);
}else {
result.put("code",-1);
result.put("msg","登陆失败");
}
return result;
}
编写html
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>Title</title>
<script src="http://libs.baidu.com/jquery/2.1.4/jquery.min.js"></script>
</head>
<body">
<!--一点开就会自动调用-->
<script>
function getnews(){
$.ajax({
type:'post',
url:'http://localhost:8080/commit',
data:$('form').serialize(),
// contentType:'application/json',
success:function (res){
console.log(res)
if(res.code == 0){
window.location.href = 'success.html'
}else{
window.location.href = 'fail.html'
}
}
})
}
</script>
<form id="form" action="##" onsubmit="return false" method="post">
<div>用户名:<input name="username"/></div>
<div>密码:<input name="password"/></div>
<div><input type="submit" value="提交" onclick="getnews()"/></div>
</form>
</body>
</html>
success.html和fail.html自行编写,只是用来做显示而已。