1、设计相关页面
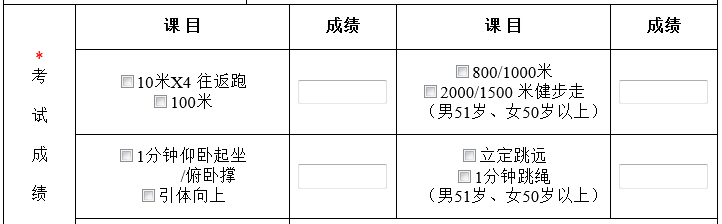
<table class="baseTable bottom-baseTable" style="width: 90%;height:720px;margin: 0 auto;table-layout: fixed">
<tr style="height: 45px;">
<td style="width:10%;" rowspan="4";><span style="color: red;">*</span><br/>考<br/><br/>试<br/><br/>成<br/><br/>绩</td>
<td style="width:30%;font-weight: bold;">课 目</td>
<td style="width:15%;font-weight: bold;">成绩</td>
<td style="width:30%;font-weight: bold;">课 目</td>
<td style="width:15%;font-weight: bold;">成绩</td>
</tr>
<tr>
<td>
<input type="checkbox" id="WFP" name="topic" value="WFP" onClick="checkSelected(1,this.id)" />10米X4 往返跑<br />
<input type="checkbox" id="DP" name="topic" value="DP" onClick="checkSelected(1,this.id)" />100米
</td>
<td><input type="text" id="score1" class="unnamed1" style="width:80%;"></td>
<td>
<input type="checkbox" id="CP" name="topic" value="CP" onClick="checkSelected(2,this.id)" />800/1000米<br />
<input type="checkbox" id="JBZ" name="topic" value="JBZ" onClick="checkSelected(2,this.id)" />2000/1500 米健步走
<
br
/><
span
style
="
padding-left
:
10%
;"
>
(男51岁、女50岁以上)
</
span
>
</td>
<td><input type="text" id="score2" class="unnamed1" style="width:80%;"></td>
</tr>
<tr>
<td>
<input type="checkbox" id="YWQZ" name="topic" value="YWQZ" onClick="checkSelected(3,this.id)" />1分钟仰卧起坐
<
br
/><
span
style
="
padding-left
:
25%
;"
>
/俯卧撑
</
span
><
br
/>
<input type="checkbox" id="YTXS" name="topic" value="YTXS" onClick="checkSelected(3,this.id)" />引体向上
</td>
<td><input type="text" id="score3" class="unnamed1" style="width:80%;"></td>
<td>
<input type="checkbox" id="LDTY" name="topic" value="LDTY" onClick="checkSelected(4,this.id)" />立定跳远<br />
<input type="checkbox" id="TS" name="topic" value="TS" onClick="checkSelected(4,this.id)" />1分钟跳绳
<br /><span style="padding-left: 10%;">(男51岁、女50岁以上)</span>
</td>
<td><input type="text" id="score4" class="unnamed1" style="width:80%;"></td>
</tr>
</table>
2、验证每组中各项是否同时选择,保证每组最多只能选择一项
//核对checkbox约定组是否同时选择
function checkSelected(group,id){
if(group == 1){
if(id == "WFP"){
$("#DP").attr("checked",false);
}else if(id == "DP"){
$("#WFP").attr("checked",false);
}
}else if(group == 2){
if(id == "CP"){
$("#JBZ").attr("checked",false);
}else if(id == "JBZ"){
$("#CP").attr("checked",false);
}
}else if(group == 3){
if(id == "YWQZ"){
$("#YTXS").attr("checked",false);
}else if(id == "YTXS"){
$("#YWQZ").attr("checked",false);
}
}else{
if(id == "LDTY"){
$("#TS").attr("checked",false);
}else if(id == "TS"){
$("#LDTY").attr("checked",false);
}
}
}
3、验证各科成绩是否未空
//验证各科目成绩是否为空
function checkScoreIsNull(){
var topic = [];
var msg = null;
$("input[name='topic']:checked").each(function(){
topic.push($(this).val());
});
if(topic.length > 0){
for(var i=0;i<topic.length;i++){
var tmp = topic[i];
if(tmp == "WFP" && $("#score1").val().trim() == ""){
$("#score1").focus();
msg = "(10米X4 往返跑)成绩不能为空!";
}else if(tmp == "DP" && $("#score1").val().trim() == ""){
$("#score1").focus();
msg = "(100米)成绩不能为空!";
}else if(tmp == "CP" && $("#score2").val().trim() == ""){
$("#score2").focus();
msg = "(800/1000米)成绩不能为空!";
}else if(tmp == "JBZ" && $("#score2").val().trim() == ""){
$("#score2").focus();
msg = "(2000/1500 米健步走)成绩不能为空!";
}else if(tmp == "YWQZ" && $("#score3").val().trim() == ""){
$("#score3").focus();
msg = "(1分钟仰卧起坐/俯卧撑)成绩不能为空!";
}else if(tmp == "YTXS" && $("#score3").val().trim() == ""){
$("#score3").focus();
msg = "(引体向上)成绩不能为空!";
}else if(tmp == "LDTY" && $("#score4").val().trim() == ""){
$("#score4").focus();
msg = "(立定跳远)成绩不能为空!";
}else if(tmp == "TS" && $("#score4").val().trim() == ""){
$("#score4").focus();
msg = "(1分钟跳绳)成绩不能为空!";
}else{
msg = "ok";
}
}
}else{
msg = "请选择课目!"
}
return msg;
}
4、通过JS获取各科目成绩并拼接成字符串当作成绩传入后台
//获取各课目成绩
function getFitnesstestScore(){
var achievement = "";
var topic = [];
$("input[name='topic']:checked").each(function(){
topic.push($(this).val());
});
if(topic.length > 0){
for(var i=0;i<topic.length;i++){
var tmp = topic[i];
var score = "";
if(tmp == "WFP" || tmp == "DP"){
score = $("#score1").val();
}else if(tmp == "CP" || tmp == "JBZ"){
score = $("#score2").val();
}else if(tmp == "YWQZ" || tmp == "YTXS"){
score = $("#score3").val();
}else{
score = $("#score4").val();
}
achievement = achievement + tmp + "/" + score + ","
}
}
return achievement;
}
5、后台解析该字符串并循环保存
/**
* 保存各项体能成绩
* @author HuangHua
* @param scoreList 各项体能成绩列表
* @throws ServiceException
*/
private void saveFitnesstestScore(Integer fitnesstestId,String fitnesstestScores) throws ServiceException{
try{
if(fitnesstestId != null && fitnesstestScores != null && fitnesstestScores.length() > 0){
String[] achievement = fitnesstestScores.split(",");
for(int i=0; i< achievement.length; i++){
FitnesstestScore fs = new FitnesstestScore();
String[] scoreArray = achievement[i].split("/");
String subject = scoreArray[0];
String score = scoreArray[1];
fs.setFitnesstestId(fitnesstestId);
fs.setSubject(subject);fs.setScore(score);
fitnesstestScoreService.createFitnesstestScore(fs);
}
}else{
throw new ServiceException("保存各科体能成绩失败,体能达标成绩id不能为空!");
}
}catch (Exception e) {
throw new ServiceException("保存各科体能成绩失败",e);
}
}