题意:这道题用广搜,与hdu 1241 Oil Deposits题,是一样的。
java代码:
import java.util.ArrayList;
import java.util.Scanner;
public class Main {
/*
* t表示测试事件的个数
* h表示行
* w表示列
* Node2952保存数据的对象数组,相当于c++的结构体。
* dir表示四个方向,上下左右
*/
static int t, h, w;
static Node2952[][] map = new Node2952[101][101];
static int dir[][] = { { -1, 0 }, { 1, 0 }, { 0, -1 }, { 0, 1 } };
static MyQueue2952<Node2952> mq = new MyQueue2952<Node2952>();
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
t = sc.nextInt();
while (t-- > 0) {
h = sc.nextInt();
w = sc.nextInt();
for (int i = 0; i < h; i++) {
String str = sc.next();
for (int j = 0; j < w; j++) {
map[i][j] = new Node2952(i, j, str.charAt(j));
}
}
int count = 0;
for (int i = 0; i < h; i++) {
for (int j = 0; j < w; j++) {
if (map[i][j].c == '#') {
map[i][j].c = '.';
breadthFirstSearch(i, j);
count++;
}
}
}
System.out.println(count);
}
}
/*
* breadthFirstSearch广度优先搜索
* x,y表示搜索源的坐标。
*/
private static void breadthFirstSearch(int x, int y) {
mq.add(map[x][y]);
while (!mq.isEmpty()) {
Node2952 no1 = mq.pop();
for (int i = 0; i < dir.length; i++) {
int a = no1.x + dir[i][0];
int b = no1.y + dir[i][1];
// 越界
if (a < 0 || b < 0 || a >= h || b >= w) {
continue;
}
// 遇到障碍物
if (map[a][b].c == '.') {
continue;
}
map[a][b].c = '.';
mq.add(map[a][b]);
}
}
}
}
/*
* Node表示结点
*/
class Node2952 {
int x;
int y;
char c;
public Node2952(int x, int y, char c) {
this.x = x;
this.y = y;
this.c = c;
}
}
/*
* 加泛型的队列。
* 用来做广搜题。
*/
class MyQueue2952<E> {
ArrayList<E> al = new ArrayList<E>();
int index;
public MyQueue2952() {
index = 0;
}
public void add(E no) {
al.add(no);
index++;
}
public E pop() {
if (al.isEmpty()) {
return null;
}
E nod = al.remove(0);
index--;
return nod;
}
public boolean isEmpty() {
return al.isEmpty();
}
}
Counting Sheep
数羊
Time Limit: 2000/1000 MS (Java/Others) Memory Limit: 32768/32768 K (Java/Others)Total Submission(s): 2603 Accepted Submission(s): 1719
Problem Description
A while ago I had trouble sleeping.
前一段时间我有睡眠问题。
I used to lie awake, staring at the ceiling, for hours and hours.
我曾经彻夜不睡,盯着天花板,一小时又一小时。
Then one day my grandmother suggested I tried counting sheep after I'd gone to bed.
然后有一天,我的祖母建议我数羊,然后才上床睡觉。
As always when my grandmother suggests things, I decided to try it out.
我每天坚持数羊。
The only problem was, there were no sheep around to be counted when I went to bed.
问题只有一个,那么就是我的身边什么时候才没有羊。
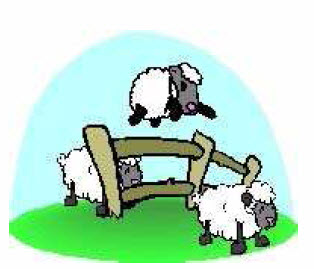
Creative as I am, that wasn't going to stop me.
大家都是聪明人,没有什么事可以阻扰我们。
I sat down and wrote a computer program that made a grid of characters, where # represents a sheep, while . is grass (or whatever you like, just not sheep).
我坐下来,写了一个计算机程序,这个程序可以处理字符网格,'#'表示一只羊,然而'。'表示一片草地(也可以表示他不是羊)。
To make the counting a little more interesting, I also decided I wanted to count flocks of sheep instead of single sheep.
为了使数羊活动变的更加有趣,我决定数羊群的个数,而不是单个羊。
Two sheep are in the same flock if they share a common side (up, down, right or left).
如果两只羊所在的图中有邻边,那么就表示这两只羊是一个羊群(四种相邻方式分别为,上下左右)。
Also, if sheep A is in the same flock as sheep B, and sheep B is in the same flock as sheep C, then sheeps A and C are in the same flock.
如果A和B是一个羊群,且B和C是一个羊群,那么A和C也是一个羊群,就是说ABC三个是一个羊群的。
Now, I've got a new problem.
如果A和B是一个羊群,且B和C是一个羊群,那么A和C也是一个羊群,就是说ABC三个是一个羊群的。
Now, I've got a new problem.
现在我遇到了一个问题。
Though counting these sheep actually helps me fall asleep, I find that it is extremely boring.
我发现这个题极其无聊,想让你帮我计算羊群的个数,来减轻我的随眠问题。
To solve this, I've decided I need another computer program that does the counting for me.
为了解决这个问题,我决定找另外的一台计算机来帮我计算。
Then I'll be able to just start both these programs before I go to bed, and I'll sleep tight until the morning without any disturbances.
这样就可以方便我一边睡觉(人睡觉),一边数羊(计算机数羊)。
I need you to write this program for me.
勇士我需要你为我写一个程序。
Input
The first line of input contains a single number T, the number of test cases to follow.
输入的第一行包含一个整数T,表示测试事件的个数。
Each test case begins with a line containing two numbers, H and W, the height and width of the sheep grid.
输入的第一行包含一个整数T,表示测试事件的个数。
Each test case begins with a line containing two numbers, H and W, the height and width of the sheep grid.
每一个测试事件的起始行包含两个整数H和W分表表示单词height(高),width(宽),这两个参数表示羊栏的大小。
Then follows H lines, each containing W characters (either # or .), describing that part of the grid.
接下来有H行数据,每一行包含W的字符,每一个字符表示一个羊圈,“#”表示羊圈有羊,“。”表示没有羊。
Output
For each test case, output a line containing a single number,
对于每一个测试事件,在单独的一行输出结果,
the amount of sheep flock son that grid according to the rules stated in the problem description.
按照问题的标准格式,输出羊群的个数。
Notes and Constraints
注意和条件
0 < T <= 100
0 < H,W <= 100
0 < T <= 100
0 < H,W <= 100
Sample Input
2 4 4 #.#. .#.# #.## .#.# 3 5 ###.# ..#.. #.###
Sample Output
6 3
Source