【项目2 - 建设“顺序表”算法库】
领会“0207将算法变程序”部分建议的方法,建设自己的专业基础设施算法库。这一周,建的是顺序表的算法库。
算法库包括两个文件:
头文件:list.h,包含定义顺序表数据结构的代码、宏定义、要实现算法的函数的声明;
源文件:list.cpp,包含实现各种算法的函数的定义
采用程序的多文件组织形式,在项目1的基础上,建立如上的两个文件,另外再建立一个源文件,编制main函数,完成相关的测试工作。
首先建立工程并添加应有的源文件和头文件,具体过程不罗列了,建好之后的文件视图如下:
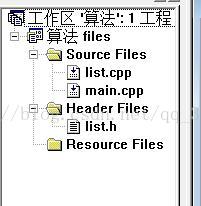
然后列出各文件的源代码:
list.h(用于存放线性表信息以及函数声明)
- #ifndef LIST_H_INCLUDED
- #define LIST_H_INCLUDED
-
- #define MaxSize 50
- typedef int ElemType;
- typedef struct
- {
- ElemType data[MaxSize];
- int length;
- } SqList;
- void CreateList(SqList *&L, ElemType a[], int n);
- void InitList(SqList *&L);
- void DestroyList(SqList *&L);
- bool ListEmpty(SqList *L);
- int ListLength(SqList *L);
- void DispList(SqList *L);
- bool GetElem(SqList *L,int i,ElemType &e);
- int LocateElem(SqList *L, ElemType e);
- bool ListInsert(SqList *&L,int i,ElemType e);
- bool ListDelete(SqList *&L,int i,ElemType &e);
- #endif
list.cpp(用于存放各类实现函数)
- #include <stdio.h>
- #include <malloc.h>
- #include "list.h"
-
-
- void CreateList(SqList *&L, ElemType a[], int n)
- {
- int i;
- L=(SqList *)malloc(sizeof(SqList));
- for (i=0; i<n; i++)
- L->data[i]=a[i];
- L->length=n;
- }
-
-
- void InitList(SqList *&L)
- {
- L=(SqList *)malloc(sizeof(SqList));
-
- L->length=0;
- }
-
-
- void DestroyList(SqList *&L)
- {
- free(L);
- }
-
-
- bool ListEmpty(SqList *L)
- {
- return(L->length==0);
- }
-
-
- int ListLength(SqList *L)
- {
- return(L->length);
- }
-
-
- void DispList(SqList *L)
- {
- int i;
- if (ListEmpty(L)) return;
- for (i=0; i<L->length; i++)
- printf("%d ",L->data[i]);
- printf("\n");
- }
-
-
- bool GetElem(SqList *L,int i,ElemType &e)
- {
- if (i<1 || i>L->length) return false;
- e=L->data[i-1];
- return true;
- }
-
-
- int LocateElem(SqList *L, ElemType e)
- {
- int i=0;
- while (i<L->length && L->data[i]!=e) i++;
- if (i>=L->length) return 0;
- else return i+1;
- }
-
-
- bool ListInsert(SqList *&L,int i,ElemType e)
- {
- int j;
- if (i<1 || i>L->length+1)
- return false;
- i--;
- for (j=L->length; j>i; j--)
- L->data[j]=L->data[j-1];
- L->data[i]=e;
- L->length++;
- return true;
- }
-
-
- bool ListDelete(SqList *&L,int i,ElemType &e)
- {
- int j;
- if (i<1 || i>L->length)
- return false;
- i--;
- e=L->data[i];
- for (j=i; j<L->length-1; j++)
- L->data[j]=L->data[j+1];
- L->length--;
- return true;
- }
main.cpp(测试函数的主函数)
- #include "list.h"
- int main()
- {
- SqList *sq;
- ElemType x[6]= {5,8,7,2,4,9};
- CreateList(sq, x, 6);
- DispList(sq);
- return 0;
- }
运行结果如下:
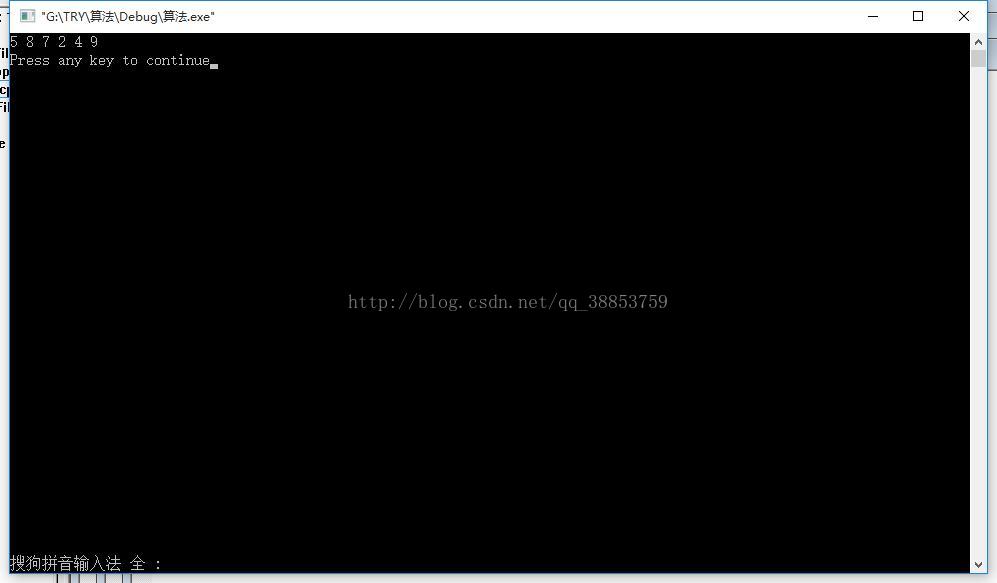