学生管理系统
采用python里面的Tkinter库,建立一个简单的图形交换界面,连接本地数据库,进行一些操作。
代码和数据库结构最后统一放。
下面这些库需要导入,哪个报错就下哪个。
from tkinter import * from tkinter import ttk import tkinter as tk from tkinter import messagebox import pickle import pymysql
登录界面
管理员界面
具体内容
学生界面
具体内容
程序代码如下:
1.sql语句代码:连接数据库的密码自己填,命名为sql_command.py文件,后面需要调用,名字乱了得改一下代码(如果你愿意)。注意,sql语句得和你数据库有关,我建立的数据库放在最后面了,有问题的话就别用我的数据库,用自己的也就只需要找到对应功能修改一下sql语句,工作量不大,而且sql语句不难。
import pymysql
from tkinter import *
from tkinter import ttk
import tkinter as tk
from tkinter import messagebox
db = pymysql.connect(host='localhost', user='root', password='mysql密码自己填', db='数据库名,自己创建一个,我的是course_work', port=3306)
#查询学号
def sel_num():
cur1 = db.cursor()
sql='select stu_num from stu'
cur1.execute(sql)
return cur1.fetchall()
#查询姓名
def select_name(stu_nums):
stu_nums=int(stu_nums)
cur1 = db.cursor()
sql = f"select stu_name from stu where stu_num='{stu_nums}'"
cur1.execute(sql)
return cur1.fetchone()
#查询性别
def select_sex(stu_nums):
stu_nums=int(stu_nums)
cur1 = db.cursor()
sql = f"select sex from stu where stu_num='{stu_nums}'"
cur1.execute(sql)
return cur1.fetchone()
#查询年龄
def select_age(stu_nums):
stu_nums=int(stu_nums)
cur1 = db.cursor()
sql = f"select age from stu where stu_num='{stu_nums}'"
cur1.execute(sql)
return cur1.fetchone()
#查询学生选课信息
def select_courses(stu_nums):
stu_nums=int(stu_nums)
cur1 = db.cursor()
sql = "select course_name,teacher_name,start_time,end_time from courses join stu_grades on \
stu_grades.course_id=courses.course_id where stu_grades.stu_num='{}' ".format(stu_nums)
cur1.execute(sql)
return cur1.fetchall()
#查找课程对应成绩
def select_courses1(stu_nums):
stu_nums=int(stu_nums)
cur1 = db.cursor()
sql = "select course_name,score,teacher_name,end_time from courses join stu_grades on \
stu_grades.course_id=courses.course_id where stu_grades.stu_num='{}'".format(stu_nums)
cur1.execute(sql)
return cur1.fetchall()
#查询所有学生信息
def select_stus():
cur1 = db.cursor()
sql = "select stu_num,stu_name,sex,age from stu"
cur1.execute(sql)
return cur1.fetchall()
#删除学生信息
def del_stus(stu_nums):
stu_nums=int(stu_nums)
cur1 = db.cursor()
sql="delete from stu where stu_num={} ".format(stu_nums)
cur1.execute(sql)
db.commit()
#查询学生python成绩
def sel_stus_python_grades():
cur1=db.cursor()
sql="SELECT stu.stu_num,stu_name,score from stu join stu_grades join courses on stu_grades.stu_num=stu.stu_num \
and stu_grades.course_id=courses.course_id where stu_grades.course_id=1;"
cur1.execute(sql)
return cur1.fetchall()
def sel_stus_java_grades():
cur1=db.cursor()
sql="SELECT stu.stu_num,stu_name,score from stu join stu_grades join courses on stu_grades.stu_num=stu.stu_num \
and stu_grades.course_id=courses.course_id where stu_grades.course_id=3;"
cur1.execute(sql)
return cur1.fetchall()
def sel_stus_mysql_grades():
cur1=db.cursor()
sql="SELECT stu.stu_num,stu_name,score from stu join stu_grades join courses on stu_grades.stu_num=stu.stu_num \
and stu_grades.course_id=courses.course_id where stu_grades.course_id=2;"
cur1.execute(sql)
return cur1.fetchall()
def sel_stus_django_grades():
cur1=db.cursor()
sql="SELECT stu.stu_num,stu_name,score from stu join stu_grades join courses on stu_grades.stu_num=stu.stu_num \
and stu_grades.course_id=courses.course_id where stu_grades.course_id=4;"
cur1.execute(sql)
return cur1.fetchall()
#添加学生信息
def add_student(num,name,sex,age):
cur1=db.cursor()
sql="INSERT into stu VALUES ('%s','%s','%s','%s')"%(num,name,sex,age)
cur1.execute(sql)
db.commit()
cur1.close()
#添加课程信息
def add_course(num,course_num):
cur1 = db.cursor()
sql = "INSERT into stu_grades(stu_num,course_id) VALUES (%s,%s)" % (num, course_num)
cur1.execute(sql)
db.commit()
cur1.close()
# add_course(5011221723,1)
#修改学生成绩
def alter_course_score(num,course_num,grade):
cur1 = db.cursor()
sql="update stu_grades set score={} where stu_num={} and course_id={}".format(grade,num,course_num)
cur1.execute(sql)
db.commit()
2.管理员功能:py文件命名为admin_tk
from tkinter import *
from tkinter import ttk
import tkinter as tk
from tkinter import messagebox
import pymysql
from sql_command import select_stus,del_stus,sel_stus_python_grades,sel_stus_django_grades,sel_stus_java_grades
from sql_command import sel_stus_mysql_grades,add_student,add_course
from grade import sel_python,sel_java,sel_mysql,sel_django,choose_course,alter_course
db = pymysql.connect(host='localhost', user='root', password='同上', db='同上', port=3306)
#添加学生信息
def add_stu():
#退出功能
def destory_root():
add_msg.destroy()
#添加信息功能:
def add_msgs():
# global stu,stu_names,stu_sexs1,stu_ages
stu=stu_num.get()
stu_names=stu_name.get()
stu_sexs1=stu_sex.get()
stu_ages=stu_age.get()
print(stu_names)
print(stu)
add_student(stu,stu_names,stu_sexs1,stu_ages)
tk.messagebox.showinfo('提示!\n','添加成功')
#导入添加课程函数
from grade import choose_course
add_msg=Tk()
screenwidth = add_msg.winfo_screenwidth()
screenheight = add_msg.winfo_screenheight()
width = 350
height = 200
size = '%dx%d+%d+%d' % (width, height, (screenwidth - width) / 2, (screenheight - height) / 2)
add_msg.geometry(size)
# 设置窗口标题
add_msg.title('添加学生信息')
tk.Label(add_msg, text='学生学号:').place(x=10, y=20)
tk.Label(add_msg, text='学生名字:').place(x=10, y=60)
tk.Label(add_msg, text='学生性别:').place(x=10, y=100)
tk.Label(add_msg, text='学生年龄:').place(x=10, y=140)
global stu_num,stu_name,stu_sex,stu_age
stu_num = tk.IntVar(master=add_msg)
entry1= tk.Entry(add_msg, textvariable=stu_num).place(x=70, y=20)
stu_name = tk.StringVar(master=add_msg)
entry2= tk.Entry(add_msg, textvariable=stu_name).place(x=70, y=60)
stu_sex = tk.StringVar(master=add_msg)
entry3= tk.Entry(add_msg, textvariable=stu_sex).place(x=70, y=100)
stu_age = tk.IntVar(master=add_msg)
entry4= tk.Entry(add_msg, textvariable=stu_age).place(x=70, y=140)
add_courses_button=tk.Button(add_msg,text='添加课程信息',bg='green',command=choose_course).place(x=240,y=30)
add_courses_button = tk.Button(add_msg, text='修改成绩信息', bg='green', command=alter_course).place(x=240, y=75)
add_courses_button = tk.Button(add_msg, text='关闭', bg='green', command=destory_root).place(x=240, y=120)
add_courses_button = tk.Button(add_msg, text='保存', bg='green', command=add_msgs).place(x=285, y=120)
# add_msg.mainloop()
#删除学生信息
def del_stu():
#删除功能
def delete():
global num
num=stu_num.get()
print("hhhhh:"+str(num))
del_stus(num)
tk.messagebox.showinfo('提示!\n','删除成功')
# 退出功能
def destory_root():
root.destroy()
# 主窗口
root = Tk()
screenwidth = root.winfo_screenwidth()
screenheight = root.winfo_screenheight()
width = 240
height = 140
size = '%dx%d+%d+%d' % (width, height, (screenwidth - width) / 2, (screenheight - height) / 2)
root.geometry(size)
root.title('学生信息删除')
tk.Label(root,text='学生学号:').place(x=10,y=20)
#这里的问题,多个窗口IntVar()要用master绑定,caocaocao
stu_num= tk.IntVar(master=root) #输入框内容,stu_num.get()获取值
#你这直接就执行了呀
# stu_num.set(5011221723)
# print("rrrrrr:" + str(stu_num.get()))
# stu_num.set(5011221723)# 设置内容
# print(num)
num_entry = tk.Entry(root, textvariable=stu_num,width=20).place(x=75, y=20)
# num = stu_num.get()
# print(num) #0
#del_button用不上他的放回结果就不要接收啊,有时候用到检测是否按下啥的,加了好像也没很大问题
#pycharm这个好像也有有一个可以制作可视化tk代码界面的工具,你可以网上找下,支持分页等等操作
#弄完这个我也去帮另一个人弄java的这个mysql课设,我的基本完了,折磨完了终于,饭都快吃不下。
tk.Button(root,text='删除',command=delete).place(x=150,y=80)
colse_button=tk.Button(root,text='关闭',command=destory_root).place(x=50,y=80)
# root.mainloop()
#查询所有学生信息
def select_stu():
#退出功能
def destory_root():
root.destroy()
#主窗口
root = tk.Tk()
screenwidth = root.winfo_screenwidth()
screenheight = root.winfo_screenheight()
width = 360
height = 240
size = '%dx%d+%d+%d' % (width, height, (screenwidth - width) / 2, (screenheight - height) / 2)
root.geometry(size)
root.title('学生信息查询')
tk.Label(root, text='学生信息').pack()
area = ('学号', '姓名', '性别', '年龄')#列名
ac = ('sno', 'names', 'sex', 'age')#栏目
#数据交换处
data =list(select_stus())
tv = ttk.Treeview(root, columns=ac, show='headings',height = 6)
for i in range(len(ac)):
tv.column(ac[i], width=80, anchor='center')
tv.heading(ac[i], text=area[i])
tv.pack()
for i in range(len(data)):
tv.insert('', 'end', values=data[i])
#退出
exit_btn=tk.Button(root,text='关闭',command=destory_root).place(x=180,y=180)
root.mainloop()
#学生成绩管理
def grade_stu():
def destory_root():
win.destroy()
win=Tk()
screenwidth = win.winfo_screenwidth()
screenheight = win.winfo_screenheight()
width = 300
height = 200
size = '%dx%d+%d+%d' % (width, height, (screenwidth - width) / 2, (screenheight - height) / 2)
win.geometry(size)
win.title('学生成绩管理')
select_stu = tk.Button(win, text='学生python成绩查询', command=sel_python).place(x=50, y=10)
add_stu = tk.Button(win, text='学生java成绩查询', command=sel_java).place(x=50, y=50)
del_stu = tk.Button(win, text='学生mysql成绩查询', command=sel_mysql).place(x=50, y=90)
grade_stu = tk.Button(win, text='学生django成绩查询', command=sel_django).place(x=50, y=130)
exit_btn = tk.Button(win, text='关闭', command=destory_root).place(x=230, y=60)
3.功能完善:py文件命名为grade
from tkinter import *
from tkinter import ttk
import tkinter as tk
from tkinter import messagebox
import pymysql
from sql_command import sel_stus_python_grades,sel_stus_django_grades,sel_stus_java_grades
from sql_command import sel_stus_mysql_grades,add_course,alter_course_score
#每个文件里头最好都放过数据库连接,我没试过不写会咋样,反正写了总没事。
db = pymysql.connect(host='localhost', user='root', password='同上', db='同上', port=3306)
#查询python成绩
def sel_python():
def destory_root():
root.destroy()
# root = tk.Toplevel(win)
root=Tk()
screenwidth = root.winfo_screenwidth()
screenheight = root.winfo_screenheight()
width = 300
height = 240
size = '%dx%d+%d+%d' % (width, height, (screenwidth - width) / 2, (screenheight - height) / 2)
root.geometry(size)
root.title('学生成绩管理')
tk.Label(root, text='学生成绩').pack()
# area = ('学号', '姓名', 'java', 'python','mysql','django') # 列名
area = ('学号', '姓名', 'python') # 列名
# ac = ('sno', 'names', 'java_grade', 'python_grade','mysql_grade','django_grade') # 栏目
ac = ('sno', 'names', 'python_grade') # 栏目
# 数据替换
# data = \
# [
# ('张三', '90', '88', '95','35','34'),
# ('李四', '100', '92', '90','45','34'),
# ('王二', '88', '90', '91','67','67'),
# ('赵六', '25', 'wan', '21','87','23'),
# ('王琦', '23', 'man', '23','34','56'),
# ('老六', '48', '男', '19','34','23'),
# ('老八', '23', '女', '10','54','12')
# ]
data = list(sel_stus_python_grades())
tv = ttk.Treeview(root, columns=ac, show='headings', height=6)
for i in range(len(ac)):
tv.column(ac[i], width=80, anchor='center')
tv.heading(ac[i], text=area[i])
tv.pack()
for i in range(len(data)):
tv.insert('', 'end', values=data[i])
# 退出
exit_btn = tk.Button(root, text='关闭', command=destory_root).place(x=150, y=180)
#查询java成绩
def sel_java():
def destory_root():
root.destroy()
# root = tk.Toplevel(win)
root=Tk()
screenwidth = root.winfo_screenwidth()
screenheight = root.winfo_screenheight()
width = 300
height = 240
size = '%dx%d+%d+%d' % (width, height, (screenwidth - width) / 2, (screenheight - height) / 2)
root.geometry(size)
root.title('学生成绩管理')
tk.Label(root, text='学生成绩').pack()
# area = ('学号', '姓名', 'java', 'python','mysql','django') # 列名
area = ('学号', '姓名', 'java') # 列名
# ac = ('sno', 'names', 'java_grade', 'python_grade','mysql_grade','django_grade') # 栏目
ac = ('sno', 'names', 'java_grade') # 栏目
# 数据替换
# data = \
# [
# ('张三', '90', '88', '95','35','34'),
# ('李四', '100', '92', '90','45','34'),
# ('王二', '88', '90', '91','67','67'),
# ('赵六', '25', 'wan', '21','87','23'),
# ('王琦', '23', 'man', '23','34','56'),
# ('老六', '48', '男', '19','34','23'),
# ('老八', '23', '女', '10','54','12')
# ]
data = list(sel_stus_java_grades())
tv = ttk.Treeview(root, columns=ac, show='headings', height=6)
for i in range(len(ac)):
tv.column(ac[i], width=80, anchor='center')
tv.heading(ac[i], text=area[i])
tv.pack()
for i in range(len(data)):
tv.insert('', 'end', values=data[i])
# 退出
exit_btn = tk.Button(root, text='关闭', command=destory_root).place(x=150, y=180)
#查询mysql成绩
def sel_mysql():
def destory_root():
root.destroy()
# root = tk.Toplevel(win)
root = Tk()
screenwidth = root.winfo_screenwidth()
screenheight = root.winfo_screenheight()
width = 300
height = 240
size = '%dx%d+%d+%d' % (width, height, (screenwidth - width) / 2, (screenheight - height) / 2)
root.geometry(size)
root.title('学生成绩管理')
tk.Label(root, text='学生成绩').pack()
# area = ('学号', '姓名', 'java', 'python','mysql','django') # 列名
area = ('学号', '姓名', 'mysql') # 列名
# ac = ('sno', 'names', 'java_grade', 'python_grade','mysql_grade','django_grade') # 栏目
ac = ('sno', 'names', 'mysql_grade') # 栏目
data = list(sel_stus_mysql_grades())
tv = ttk.Treeview(root, columns=ac, show='headings', height=6)
for i in range(len(ac)):
tv.column(ac[i], width=80, anchor='center')
tv.heading(ac[i], text=area[i])
tv.pack()
for i in range(len(data)):
tv.insert('', 'end', values=data[i])
# 退出
exit_btn = tk.Button(root, text='关闭', command=destory_root).place(x=150, y=180)
#查询django成绩
def sel_django():
def destory_root():
root.destroy()
# root = tk.Toplevel(win)
root = Tk()
screenwidth = root.winfo_screenwidth()
screenheight = root.winfo_screenheight()
width = 300
height = 240
size = '%dx%d+%d+%d' % (width, height, (screenwidth - width) / 2, (screenheight - height) / 2)
root.geometry(size)
root.title('学生成绩管理')
tk.Label(root, text='学生成绩').pack()
# area = ('学号', '姓名', 'java', 'python','mysql','django') # 列名
area = ('学号', '姓名', 'django') # 列名
# ac = ('sno', 'names', 'java_grade', 'python_grade','mysql_grade','django_grade') # 栏目
ac = ('sno', 'names', 'django_grade') # 栏目
data = list(sel_stus_django_grades())
tv = ttk.Treeview(root, columns=ac, show='headings', height=6)
for i in range(len(ac)):
tv.column(ac[i], width=80, anchor='center')
tv.heading(ac[i], text=area[i])
tv.pack()
for i in range(len(data)):
tv.insert('', 'end', values=data[i])
# 退出
exit_btn = tk.Button(root, text='关闭', command=destory_root).place(x=150, y=180)
#选择课程
def choose_course():
#退出窗口
def destory_root():
add_msg.destroy()
#功能实现函数
def command_ok():
global Check1,Check2,Check3,Check4,stu_nums
stu_nums=stu_num.get()
if Check1.get()==1:
add_course(stu_nums,1)
if Check2.get()==1:
add_course(stu_nums, 2)
if Check3.get()==1:
add_course(stu_nums, 3)
if Check4.get() == 1:
add_course(stu_nums, 4)
tk.messagebox.showinfo('提示\n','添加成功')
add_msg = Tk()
screenwidth = add_msg.winfo_screenwidth()
screenheight = add_msg.winfo_screenheight()
width = 300
height = 200
size = '%dx%d+%d+%d' % (width, height, (screenwidth - width) / 2, (screenheight - height) / 2)
add_msg.geometry(size)
# 设置窗口标题
add_msg.title('添加学生选课信息')
#设置输入框
tk.Label(add_msg, text='学生学号:').place(x=40, y=20)
stu_num = tk.IntVar(master=add_msg)
entry1 = tk.Entry(add_msg, textvariable=stu_num).place(x=110, y=20)
# 新建整型变量
global Check1,Check2,Check3,Check4,stu_nums
Check1 = IntVar(master=add_msg)
Check2 = IntVar(master=add_msg)
Check3 = IntVar(master=add_msg)
Check4 = IntVar(master=add_msg)
# 设置4个复选框控件,使用variable参数来接收变量
check1 = Checkbutton(add_msg, text="python",variable = Check1).place(x=40,y=50)
check2 = Checkbutton(add_msg, text="mysql",variable = Check2).place(x=40,y=80)
check3 = Checkbutton(add_msg, text="java",variable = Check3).place(x=40,y=110)
check4 = Checkbutton(add_msg,text='django',variable=Check4).place(x=40,y=140)
choose_button=tk.Button(add_msg,text='确认',command=command_ok).place(x=200,y=90)
choose_button = tk.Button(add_msg, text='返回',command=destory_root).place(x=150, y=90)
#修改课程信息
def alter_course():
#退出窗口
def destory_root():
add_msg.destroy()
#确定修改函数
def alter_ok():
global python,mysql,java,django,num
python=python_grade.get()
mysql=mysql_grade.get()
java=java_grade.get()
django=django_grade.get()
num=stu_num.get()
# print(python)
# print(mysql)
# print(num)
if Check1.get()==1:
alter_course_score(num,1,python)
if Check2.get()==1:
alter_course_score(num,2,mysql)
if Check3.get()==1:
alter_course_score(num,3,java)
if Check4.get()==1:
alter_course_score(num,4,django)
tk.messagebox.showinfo('提示\n','修改成功')
add_msg = Tk()
screenwidth = add_msg.winfo_screenwidth()
screenheight = add_msg.winfo_screenheight()
width = 330
height = 200
size = '%dx%d+%d+%d' % (width, height, (screenwidth - width) / 2, (screenheight - height) / 2)
add_msg.geometry(size)
# 设置窗口标题
add_msg.title('修改学生成绩信息')
# 设置输入框
global python,mysql,java,django,num
tk.Label(add_msg, text='学生学号:').place(x=40, y=20)
stu_num = tk.IntVar(master=add_msg)
entry1 = tk.Entry(add_msg, textvariable=stu_num).place(x=110, y=15)
#设置提醒
tk.Label(add_msg,text='勾选成绩项后再修改成绩',bg='red').place(x=100,y=180)
#设置成绩输入框
tk.Label(add_msg, text='成绩修改:').place(x=110, y=50)
python_grade=tk.IntVar(master=add_msg)
entry1 = tk.Entry(add_msg, textvariable=python_grade,width=5).place(x=170, y=50)
tk.Label(add_msg, text='成绩修改:').place(x=110, y=80)
mysql_grade = tk.IntVar(master=add_msg)
entry1 = tk.Entry(add_msg, textvariable=mysql_grade,width=5).place(x=170, y=80)
tk.Label(add_msg, text='成绩修改:').place(x=110, y=110)
java_grade = tk.IntVar(master=add_msg)
entry1 = tk.Entry(add_msg, textvariable=java_grade,width=5).place(x=170, y=110)
tk.Label(add_msg, text='成绩修改:').place(x=110, y=140)
django_grade = tk.IntVar(master=add_msg)
entry1 = tk.Entry(add_msg, textvariable=django_grade,width=5).place(x=170, y=140)
# 新建整型变量
global Check1, Check2, Check3, Check4, stu_nums
Check1 = IntVar(master=add_msg)
Check2 = IntVar(master=add_msg)
Check3 = IntVar(master=add_msg)
Check4 = IntVar(master=add_msg)
# 设置4个复选框控件,使用variable参数来接收变量
check1 = Checkbutton(add_msg, text="python", variable=Check1).place(x=40, y=50)
check2 = Checkbutton(add_msg, text="mysql", variable=Check2).place(x=40, y=80)
check3 = Checkbutton(add_msg, text="java", variable=Check3).place(x=40, y=110)
check4 = Checkbutton(add_msg, text='django', variable=Check4).place(x=40, y=140)
choose_button = tk.Button(add_msg, text='确认', command=alter_ok).place(x=270, y=140)
choose_button = tk.Button(add_msg, text='返回', command=destory_root).place(x=270, y=100)
# add_msg.mainloop()
4.主窗口和功能实现:
from sql_command import select_courses1
from sql_command import select_stus,del_stus,sel_stus_python_grades,sel_stus_django_grades,sel_stus_java_grades
from sql_command import sel_stus_mysql_grades,add_student,add_course
from grade import sel_python,sel_java,sel_mysql,sel_django,choose_course,alter_course
#收工收工,历时一个星期。从tk复习各个组件开始,最开始第一版只有输入框等,界面输出不美观,后期添加各种列表框和按钮提示框。
#数据库连接最开始本地,后期想到远端使用,便学了一下方法,弄了内网渗透,问题便解决了。
#同时通过pyinstaller将py文件打包成exe文件,即使没有编译器也能远端运行学生管理系统,十分感谢制作过程中的测试人员。
#该课设有不少可以优化的地方,可以添加老师信息和课程信息,界面交换设计挺累的,人多就弄的完善些,不过下次一定。
#函数的命名,由于相似功能太多,函数命名有些不好弄,所有设置了不少提示。设置管理员账号(本次是使用默认admin账号)。
db = pymysql.connect(host='localhost', user='root', password='同上', db='同上', port=3306)
#管理员界面
def control_stu():
# #添加学生信息
# def add_stu():
# pass
# #删除学生信息
# def del_stu():
# pass
#查询所有学生信息
# def select_stu():
# pass
#学生成绩管理
# def grade_stu():
# pass
from admin_tk import add_stu, del_stu, select_stu, grade_stu
con_stu= tk.Toplevel(win)
width = 350
height = 200
size = '%dx%d+%d+%d' % (width, height, (screenwidth - width) / 2, (screenheight - height) / 2)
con_stu.geometry(size)
con_stu.title('管理员模式')
select_stu= tk.Button(con_stu,text='学生信息查询',command=select_stu).place(x=130, y=10)
add_stu=tk.Button(con_stu,text='添加学生信息',command=add_stu).place(x=130,y=50)
del_stu= tk.Button(con_stu,text='删除学生信息',command=del_stu).place(x=130, y=90)
grade_stu= tk.Button(con_stu,text='学生成绩管理',command=grade_stu).place(x=130, y=130)
#学生信息界面
global usr_name
def stu_information():
#连接数据库
# db = pymysql.connect(host='localhost', user='root', password='', db='', port=3306)
# cur1 = db.cursor()
# sql = "select sname from stu where stu_num=545703"
# cur1.execute(sql)
# print(cur1.fetchone()) # 查询信息
#退出系统
def exit_items():
tk.messagebox.showinfo('退出成功!\n', '欢迎下次使用')
stu_ifm.destroy()
#查询成绩功能
def select_grade():
#退出功能
def distory():
grade_win.destroy()
global usr_name
usr_name=username.get()
grade_win =tk.Toplevel(win)
width = 380
height = 240
size = '%dx%d+%d+%d' % (width, height, (screenwidth - width) / 2, (screenheight - height) / 2)
grade_win.geometry(size)
#设置窗口标题
grade_win.title('学生成绩查询')
tk.Label(grade_win, text='学生成绩').pack()
area = ('科目', '成绩', '课程老师', '时间') # 列名
ac = ('courses', 'grade', 'teacher', 'time') # 栏目
# 插入合理数据
data = list(select_courses1(usr_name))
tv = ttk.Treeview(grade_win, columns=ac, show='headings', height=6)
for i in range(len(ac)):
tv.column(ac[i], width=80, anchor='center')
tv.heading(ac[i], text=area[i])
tv.pack()
for i in range(len(data)):
tv.insert('', 'end', values=data[i])
# 退出
exit_btn = tk.Button(grade_win, text='关闭', command=distory).place(x=190, y=180)
#查询课程功能
def select_course():
def distory():
course_win.destroy()
global usr_name
usr_name=username.get()
course_win =tk.Toplevel(win)
width = 400
height = 240
size = '%dx%d+%d+%d' % (width, height, (screenwidth - width) / 2, (screenheight - height) / 2)
course_win.geometry(size)
course_win.title('学生选课查询')
tk.Label(course_win, text='学生选修课程').pack()
area = ('科目', '课程老师', '开始时间','结课时间') # 列名
ac = ('courses', 'teacher', 'start_time', 'end_time') # 栏目
#插入合理数据
data=list(select_courses(usr_name))
tv = ttk.Treeview(course_win, columns=ac, show='headings', height=6)
for i in range(len(ac)):
tv.column(ac[i], width=90, anchor='center')
tv.heading(ac[i], text=area[i])
tv.pack()
for i in range(len(data)):
tv.insert('', 'end', values=data[i])
# 退出
exit_btn = tk.Button(course_win, text='关闭', command=distory).place(x=200, y=180)
#
# tk.Label(course_win, text='所选课程:').place(x=10, y=20)
# classes= tk.StringVar()
# classes.set(select_courses(usr_name)) #设置内容
# class_entry = tk.Entry(course_win, textvariable=classes).place(x=70, y=20)
#
# tk.Label(course_win, text='课程老师:').place(x=10, y=80)
# teachers= tk.StringVar()
# teachers.set(select_teachers(usr_name)) # 设置内容
# teacher_entry= tk.Entry(course_win, textvariable=teachers).place(x=70, y=80)
# tk.Button(course_win,text='关闭',command=distory).place(x=100,y=130)
global usr_name
usr_name = username.get()
stu_ifm= tk.Toplevel(win)
width = 350
height = 200
size = '%dx%d+%d+%d' % (width, height, (screenwidth - width) / 2, (screenheight - height) / 2)
stu_ifm.geometry(size)
stu_ifm.title('学生信息查询')
# #根据学号查出姓名性别年龄
# sel_name='select sname from stu where stu_num=usr_name'
# sel_sex='select sex from stu where stu_num=usr_name'
# sel_age='select age from stu where stu_num=usr_name'
# cur1.execute(sel_name)
tk.Label(stu_ifm, text='学号:').place(x=10, y=10)
num=tk.StringVar()
num.set(usr_name)#设置内容
entry_name = tk.Entry(stu_ifm, textvariable=num).place(x=50, y=10)
name=tk.StringVar()
name.set(select_name(usr_name))#设置内容学生姓名
tk.Label(stu_ifm, text='姓名:').place(x=10, y=50)
entry_name = tk.Entry(stu_ifm, textvariable=name).place(x=50, y=50)
tk.Label(stu_ifm, text='性别:').place(x=10, y=90)
sex=tk.StringVar()
sex.set(select_sex(usr_name))#设置内容学生性别
entry_name = tk.Entry(stu_ifm, textvariable=sex).place(x=50, y=90)
tk.Label(stu_ifm, text='年龄:').place(x=10, y=130)
age=tk.StringVar()
age.set(select_age(usr_name))#设置内容学生年龄
entry_name = tk.Entry(stu_ifm, textvariable=age).place(x=50, y=130)
grade_button=tk.Button(stu_ifm,text='成绩查询',command=select_grade).place(x=250,y=10)
course_button = tk.Button(stu_ifm,text='课程查询',command=select_course).place(x=250, y=70)
exit_button=tk.Button(stu_ifm,text='退出登录',command=exit_items).place(x=250, y=130)
#登录功能
def login():
global usr_name
usr_name = username.get()
usr_psw = user_password.get()
# with open('usrs_info.pickle', 'wb') as usr_file:
# usrs_info = {'admin': 'admin'}
# pickle.dump(usrs_info, usr_file)
try:
with open('usrs_info.pickle', 'rb') as usr_file:
usrs_info = pickle.load(usr_file)
except FileNotFoundError:
with open('usrs_info.pickle', 'wb') as usr_file:
usrs_info = {'admin': 'admin'}
pickle.dump(usrs_info, usr_file)
# 判断是否为管理员
if usr_name in usrs_info and usr_name == 'admin':
if usr_name == 'admin' and usr_psw == 'admin':
success_login = tk.messagebox.showinfo('Welcom\n', '管理员账号' + usr_name + '登录成功')
# 管理员信息界面
if success_login:
control_stu()
else:
tk.messagebox.showerror('Error\n', 'Pass word is wrong!')
# 判断是否是学生
elif usr_name in usrs_info and usr_name!='admin':
if usr_psw == usrs_info[usr_name]:
success_login = tk.messagebox.showinfo('Welcom\n', '账号' + usr_name + '登录成功')
# 信息界面
if success_login:
stu_information()
else:
tk.messagebox.showerror('Error\n', 'Pass word is wrong!')
else:
is_sign_up = tk.messagebox.showinfo('Welcom\n', '你还没有注册,是否注册?')
if is_sign_up:
usr_sign_up()
#注册功能
def usr_sign_up():
#注册用户功能
def sign_to_test():
#获取注册的信息并保存
npa=new_psw_again.get()
np=new_psw.get()
nn=new_name.get()
#判断学号是否在学生信息里
# if nn not in cur1.fetchall():
# tk.messagebox.showerror('Error\n','不存在该学号')
# else:
with open('usrs_info.pickle','rb') as usr_file:
exit_usr_ifno=pickle.load(usr_file)
if np!=npa:
tk.messagebox.showerror('Error\n','密码不一致')
elif nn in exit_usr_ifno:
tk.messagebox.showerror('Error\n','用户已存在')
else:
exit_usr_ifno[nn]=np
with open('usrs_info.pickle','wb') as usr_file:
pickle.dump(exit_usr_ifno,usr_file)
tk.messagebox.showinfo('Welcome\n','注册成功')
win_sign_up.destroy()
#在主页面上建立另一个页面
win_sign_up=tk.Toplevel(win)
width = 350
height = 200
size = '%dx%d+%d+%d' % (width, height, (screenwidth - width) / 2, (screenheight - height) / 2)
win_sign_up.geometry(size)
win_sign_up.title('注册窗口')
new_name=tk.StringVar()
tk.Label(win_sign_up,text='学号:').place(x=10,y=10)
entry_name=tk.Entry(win_sign_up,textvariable=new_name).place(x=130,y=10)
new_psw=tk.StringVar()
tk.Label(win_sign_up,text='password:').place(x=10,y=50)
entry_psw=tk.Entry(win_sign_up,textvariable=new_psw,show="*").place(x=130,y=50)
new_psw_again=tk.StringVar()
tk.Label(win_sign_up,text='repeat password:').place(x=10,y=90)
entry_psw=tk.Entry(win_sign_up,textvariable=new_psw_again,show='*').place(x=130,y=90)
btn_sign_up=tk.Button(win_sign_up,text='注册',command=sign_to_test).place(x=270,y=130)
#主登录窗口
win=Tk()
win.title('欢迎来到学生管理系统')
screenwidth = win.winfo_screenwidth()
screenheight = win.winfo_screenheight()
width = 450
height = 300
size = '%dx%d+%d+%d' % (width, height, (screenwidth - width) / 2, (screenheight - height) / 2)
win.geometry(size)
canvas=tk.Canvas(win,height=200,width=500)
image_file=tk.PhotoImage(file='welcome.gif')#插入图片
image=canvas.create_image(0,0,anchor='nw',image=image_file)
canvas.pack(side='top')
tk.Label(win,text='学号').place(x=100,y=150)
tk.Label(win,text='密码').place(x=100,y=190)
username=StringVar()
stunum=tk.Entry(win,textvariable=username).place(x=150,y=150)
user_password=StringVar()
password=tk.Entry(win,textvariable=user_password,show='*').place(x=150,y=190)
bt_login=tk.Button(win,text='登录',command=login).place(x=250,y=230)
bt_signup=tk.Button(win,text='注册',command=usr_sign_up).place(x=150,y=230)
#关闭数据库
db.close()
win.mainloop()
5.贴图:需放在项目同一目录(网上扣的,然后自己改了一下大小)
注意注意:数据库如果出错的话找到错误修改一下sql_command.py文件里面的sql语句。
数据库设计:本人采用navicat,没有sql语句,这里就把表基本结构列出来。(最好别乱改名字)
1.学生表:stu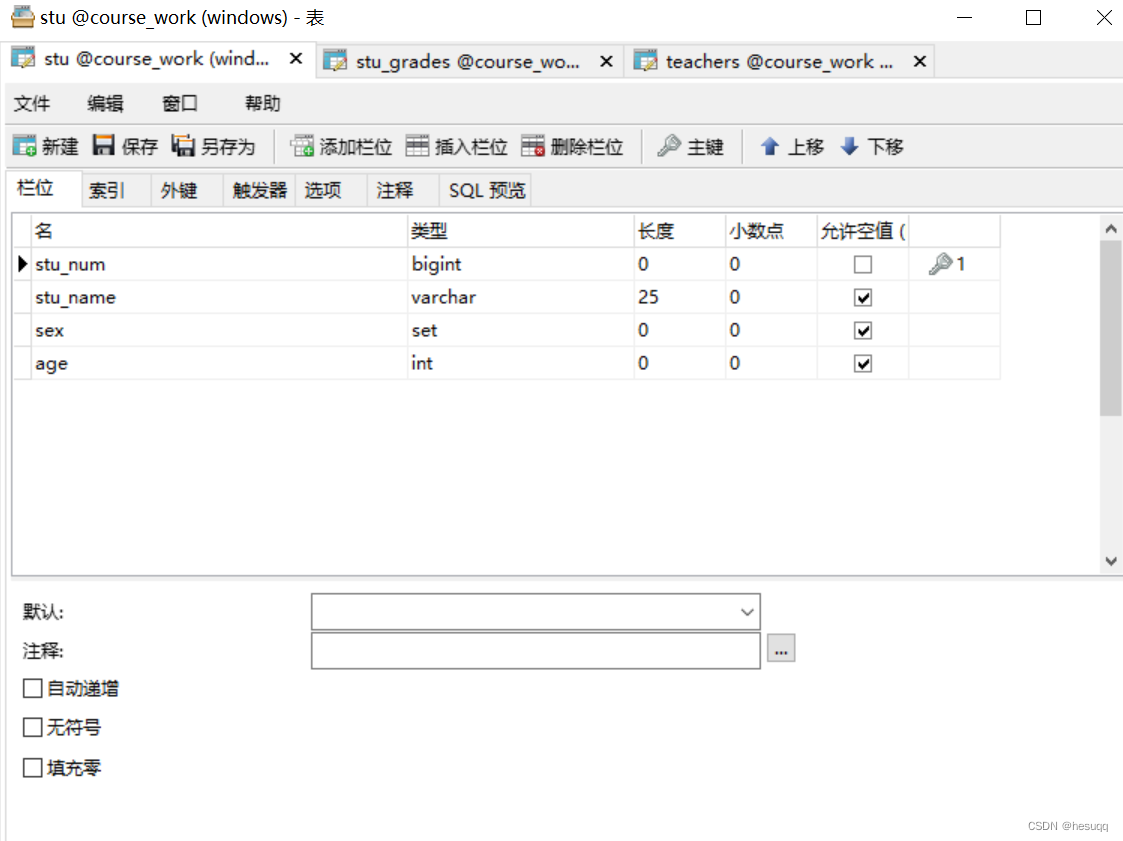
2. 学生成绩表:stu_grade
3.课程表:courses
课设说明书:后续发放。
有问题可以留言评论,尽量解答。