leetcode 101
题目链接
对称二叉树 symmetric tree,左右对称
思路:
- 先要考虑遍历方式——后序
- 因为要判断对称,肯定是从最底层开始判断,再返回父节点
class Solution {
public boolean isSymmetric(TreeNode root) {
Deque<TreeNode> q = new LinkedList<>();
q.addFirst(root.left);
q.addLast(root.right);
while (!q.isEmpty()) {
TreeNode l = q.removeFirst();
TreeNode r = q.removeLast();
if (l == null && r == null)
continue;
// 以上三个判断条件合并
if (l == null || r == null || l.val != r.val)
return false;
q.addFirst(l.left);
q.addFirst(l.right);
q.addLast(r.right);
q.addLast(r.left);
}
return true;
}
}
leetcode 104
题目链接
二叉树深度:任意一个节点到根节点的距离 ——前序遍历
二叉树高度:任意一个节点到叶子节点的距离 ——后序遍历合适
note: 根节点的高度就是二叉树的最大深度!!!
- 用迭代法,层序遍历求解结果
- 用后序遍历思路求解如下:
class Solution {
public int maxDepth(TreeNode root) {
int depth = postOrder(root);
return depth;
}
public int postOrder(TreeNode root) {
if (root == null)
return 0;
int a = postOrder(root.left);
int b = postOrder(root.right);
return Math.max(a, b) + 1;
}
}
leetcode 111
题目链接
最小深度——到叶子节点的最小距离
思路:
- like leetcode 104
- 也可以用求高度的方式去求
- 用迭代法,层序遍历求解结果
- 用后序遍历思路求解如下:
class Solution {
public int minDepth(TreeNode root) {
int res = postOrder(root);
return res;
}
public int postOrder(TreeNode root) {
if (root == null)
return 0;
int a = postOrder(root.left);
int b = postOrder(root.right);
if (root.left == null && root.right != null)
return b + 1;
if (root.left != null && root.right == null)
return a + 1;
return Math.min(a, b) + 1;
}
}
leetcode 222
题目链接
完全二叉树的节点个数
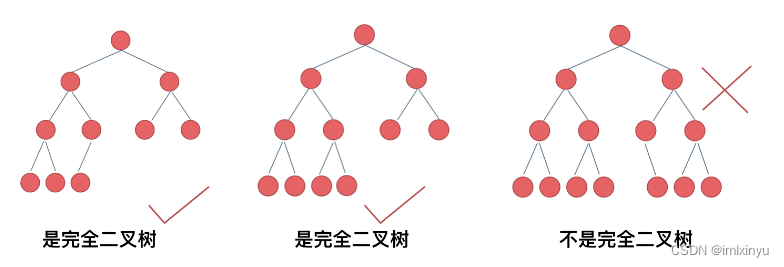
思路:
- 可以用求普通二叉树的节点个数的方法来求解
- 任意一种遍历方式都可以,层序、前中后
- 利用满二叉树的节点计算公式:
结点个数 = 2 h − 1 ( h 为树的高度 ) 结点个数 = 2^h-1(h为树的高度) 结点个数=2h−1(h为树的高度)- 利用后序遍历
- 判断是否为满二叉: 向左遍历深度 = 向右遍历深度 向左遍历深度 = 向右遍历深度 向左遍历深度=向右遍历深度
class Solution {
public int countNodes(TreeNode root) {
if (root == null)
return 0;
TreeNode l = root.left;
TreeNode r = root.right;
int leftDepth = 0, rightDepth = 0;
while (l != null) { //求向左遍历的深度
l = l.left;
++leftDepth;
}
while (r != null) {
r = r.right;
++rightDepth;
}
if (leftDepth == rightDepth) //是满二叉
return (2 << leftDepth) - 1; //depth = 2^h - 1
else //非满二叉,后序遍历
return countNodes(root.left) + countNodes(root.right) + 1;
}
}