1 初始化项目
react-native init mobxDemo
2 安装mobx
npm install mobx --save
3 安装mobx-react
npm install mobx-react --save
4.安装bable转码
npm install @babel/plugin-proposal-decorators
添加js文件
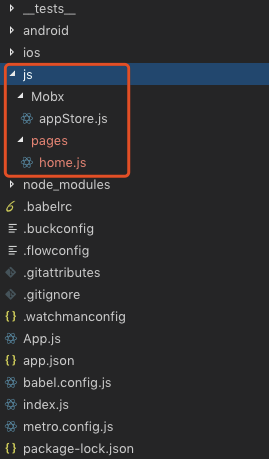
(1)appStre.js
import {observable,action} from 'mobx';
var appStore = observable({
counter: 0
});
appStore.addCount = action(()=>{
appStore.counter+=1;
});
appStore.subCount = action(()=>{
if(appStore.counter<=0){
return;
}
appStore.counter-=1;
});
appStore.changeCount = action((key)=>{
if(key<=0){
appStore.counter=0;
}
appStore.counter=parseInt(key);
});
export default appStore
(2)home.js
import React, {Component} from 'react';
import {Platform, StyleSheet, Text, View,TouchableOpacity,TextInput} from 'react-native';
import {observer, inject} from 'mobx-react';
@inject('store')
@observer
export default class Home extends Component{
constructor(props){
super(props);
this.state={
value:0
}
}
componentWillMount(){
console.log(this.props.store.counter)
}
sub=()=>{
let {store}=this.props;
store.subCount()
}
add=()=>{
let {store}=this.props;
store.addCount()
}
render() {
let {store}=this.props
return (
<View style={styles.container}>
<TouchableOpacity onPress={this.sub}>
<Text>-</Text>
</TouchableOpacity>
<TextInput style={{width:100,height:35,borderWidth:1}} value={store.counter.toString()}/>
<TouchableOpacity onPress={this.add}>
<Text>+</Text>
</TouchableOpacity>
</View>
);
}
}
const styles = StyleSheet.create({
container:{
flex:1,
flexDirection:'row',
alignItems:'center',
justifyContent:'center'
}
});
(3) app.js
import React, {Component} from 'react';
import {Platform, StyleSheet, Text, View} from 'react-native';
import {Provider} from 'mobx-react'
import AppStore from './js/Mobx/appStore'
import Home from './js/pages/home'
const instructions = Platform.select({
ios: 'Press Cmd+R to reload,\n' + 'Cmd+D or shake for dev menu',
android:
'Double tap R on your keyboard to reload,\n' +
'Shake or press menu button for dev menu',
});
export default class App extends Component{
render() {
return (
<Provider store={AppStore}>
<Home/>
</Provider>
);
}
}
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
backgroundColor: '#F5FCFF',
},
welcome: {
fontSize: 20,
textAlign: 'center',
margin: 10,
},
instructions: {
textAlign: 'center',
color: '#333333',
marginBottom: 5,
},
});
(4).babelrc(注意:react-native 0.57版本之后将全面升级babel 7,babel 6 将无法运行)
{
"presets": ["module:metro-react-native-babel-preset"],
"plugins": [
["@babel/plugin-proposal-decorators", { "legacy": true }],
["@babel/transform-runtime", {
"helpers": true,
"regenerator": false
}]
]
}
5 重新安装四个库(如果不重新安装这四个库会程序会报错)
yarn add @babel/core
yarn add @babel/plugin-proposal-decorators
yarn add @babel/plugin-transform-runtime
yarn add @babel/runtime
6 运行项目
react-native run-ios