-
问题概述:
- 需要合并两个字符串。
- 从
word1
开始,交替添加字母。 - 如果一个字符串比另一个字符串长,将多出来的字母追加到合并后字符串的末尾。
-
示例分析:
- 示例 1:
word1 = "abc", word2 = "pqr"
。合并过程如上述解释。 - 示例 2:
word1 = "ab", word2 = "pqrs"
。合并过程如上述解释。 - 示例 3:
word1 = "abcd", word2 = "pq"
。合并过程如上述解释。
- 示例 1:
-
解决思路:
- 使用两个指针
i
和j
分别指向两个字符串的起始位置。 - 通过循环,交替添加
word1[i]
和word2[j]
到合并后的字符串,同时移动指针。 - 处理两个字符串长度不同的情况,将多出来的字符追加到合并后字符串的末尾。
- 使用两个指针
-
代码实现:
- 使用
StringBuilder
作为结果字符串的容器。 - 循环条件是
i
和j
同时不超出对应字符串的长度。 - 在循环中,依次添加
word1[i]
和word2[j]
到结果字符串,并移动指针。 - 处理长度不同的情况,将多出来的字符追加到结果字符串的末尾。
- 使用
- 我的代码:
class Solution { public String mergeAlternately(String word1, String word2) { int i = 0; int j = 0; int m = word1.length(); int n = word2.length(); StringBuilder ans = new StringBuilder(); while ((i < m) && (j < n)) { ans.append(word1.charAt(i)); ans.append(word2.charAt(j)); i++; j++; } if (j == n && i != m-1) { while (i < m) { ans.append(word1.charAt(i)); i++; } } if (i == m && j != n-1) { while (j < n) { ans.append(word2.charAt(j)); j++; } } return ans.toString(); } }
但是始终报错,问题出在第二三个while循环上,排查问题,最终发现问题出在if的判断条件上,
-
if (i == m && j != n-1 ) {
while (j < n) {
ans.append(word2.charAt(j));
j++;
}
}
-
Word1=ab ,word2=pqrs 初始状态:i = 0, j = 0, m = 2, n = 4, ans = "" 第一次循环: ans.append(word1.charAt(i)),ans = "a" ans.append(word2.charAt(j)),ans = "ap" i++,j++,此时 i = 1, j =1 ans.append(word1.charAt(i)),ans = "apb" ans.append(word2.charAt(j)),ans = "apbq" i++,j++,此时 i = 1, j =1 第二次循环: ans.append(word1.charAt(i)),ans = "ab" ans.append(word2.charAt(j)),ans = "abq" i++,j++,此时 i = 2, j = 2 跳出主循环 进入第一个剩余字符处理的 if,不符合条件,: 由于 i == m == 2,我们进入第3个if语句, 此时j不等于3,ans.append(word2.charAt(j)),ans = "apbqr" j++,j=4,不符合循环判断条件,无法继续进行,跳出循环,输出结果
改正如下:
class Solution { public String mergeAlternately(String word1, String word2) { int i = 0; int j = 0; int m = word1.length(); int n = word2.length(); StringBuilder ans = new StringBuilder(); while ((i < m) && (j < n)) { ans.append(word1.charAt(i)); ans.append(word2.charAt(j)); i++; j++; } if (j == n && i != m) { while (i < m) { ans.append(word1.charAt(i)); i++; } } if (i == m && j != n) { while (j < n) { ans.append(word2.charAt(j)); j++; } } return ans.toString(); } }
优化如下:
-
class Solution { public String mergeAlternately(String word1, String word2) { int i = 0; int j = 0; int m = word1.length(); int n = word2.length(); StringBuilder ans = new StringBuilder(); while ((i < m) && (j < n)) { ans.append(word1.charAt(i)); ans.append(word2.charAt(j)); i++; j++; while (i < m) { ans.append(word1.charAt(i)); i++; } while (j < n) { ans.append(word2.charAt(j)); j++; } return ans.toString(); } }
LC(day1)要求合并两个字符串,规则是从 word1 开始,交替添加字母,如果一个字符串比另一个字符串长,就将多出来的字母追加到合并后字符串的末尾。
最新推荐文章于 2024-11-16 21:36:47 发布
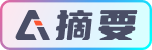