本篇内容: ArtTS系统能力-通知的学习(3.1)
一、 知识储备
1. 基础类型通知
按内容分成四类:
类型 | 描述 |
---|
NOTIFICATION_CONTENT_BASIC_TEXT | 普通文本类型 |
NOTIFICATION_CONTENT_LONG_TEXT | 长文本类型 |
NOTIFICATION_CONTENT_MULTILINE | 多行文本类型 |
NOTIFICATION_CONTENT_PICTURE | 图片类型 |
2. 带进度类型通知
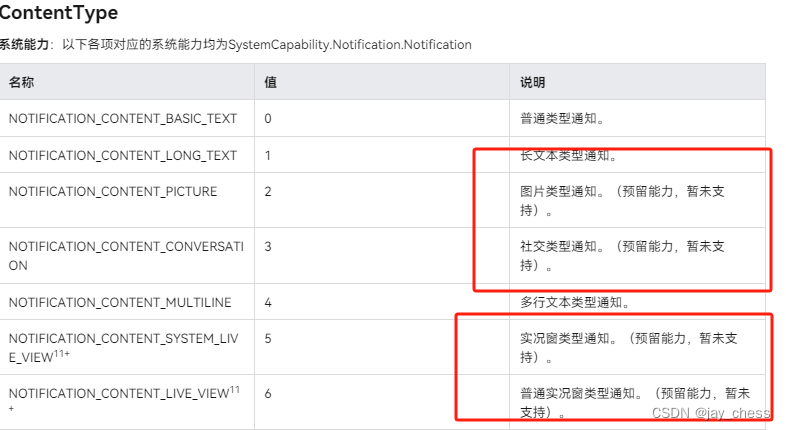
3. 带事件响应类型通知
二、 效果一览
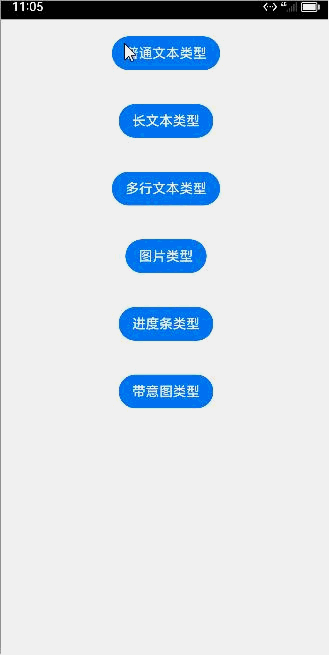
三、 源码剖析
import notificationManager from '@ohos.notificationManager'
import http from '@ohos.net.http'
import ResponseCode from '@ohos.net.http'
import image from '@ohos.multimedia.image'
import wantAgent from '@ohos.app.ability.wantAgent'
function basicText() {
let notificationRequest = {
id: 1,
content: {
contentType: notificationManager.ContentType.NOTIFICATION_CONTENT_BASIC_TEXT,
normal: {
title: '通知类型',
text: '普通文本类型',
additionalText: '我是补充标题'
}
}
}
notificationManager.publish(notificationRequest, err => {
if (err) {
console.error(`普通文本类型通知发布失败: ${err}`)
return;
}
console.info('普通文本类型通知发布成功')
})
}
function longText() {
let notificationRequest = {
id: 2,
content: {
contentType: notificationManager.ContentType.NOTIFICATION_CONTENT_LONG_TEXT,
longText: {
title: '通知类型', //无效
text: '长文本类型', //无效
additionalText: '我是补充标题 ',
longText: '我是长文本我是长文本我是长文本',
briefText: '我是简明信息', //无效
expandedTitle: '我是扩展文本'
}
}
}
notificationManager.publish(notificationRequest, err => {
if (err) {
console.error('长文本类型通知发布失败:' + err)
return;
}
console.info('长文本类型通知发布成功')
})
}
function multiline() {
let notificationRequest = {
id: 3,
content: {
contentType: notificationManager.ContentType.NOTIFICATION_CONTENT_MULTILINE,
multiLine: {
title: '通知类型', //无效
additionalText: '我是补充标题',
text: '多行文本类型', //无效
briefText: '我是简明信息', //无效
longTitle: '我是长文本标题',
lines: ['第一行', '第二行', '第三行', '第四行', '第五行']
}
}
}
notificationManager.publish(notificationRequest, err => {
if (err) {
console.error(`多行文本通知发布失败: ${err}`)
return;
}
console.info('多行文本通知发布成功')
})
}
function picture() {
let imgUrl: string = 'https://img1.baidu.com/it/u=3241660985,1063915045&fm=253&fmt=auto&app=138&f=JPEG?w=800&h=1194';
http.createHttp().request(imgUrl, (err, data) => {
if (err) {
console.error(`err is ${JSON.stringify(err)}`)
} else {
let code = data.responseCode;
if (ResponseCode.ResponseCode.OK == code) {
let res: any = data.result;
let imageSource = image.createImageSource(res)
let options = {
alphaTye: 0, //透明度
editable: false, //是否可编辑
pixelFormat: 3, //像素格式
scaleMode: 1, //缩略值
size: { height: 100, wight: 100 } //创建图片大小
}
imageSource.createPixelMap(options).then(pixelMap => {
let imagePixelMap: PixelMap = undefined;
imagePixelMap = pixelMap;
let notificationRequest = {
id: 4,
content: {
contentType: notificationManager.ContentType.NOTIFICATION_CONTENT_PICTURE,
picture: {
title: '通知类型',
text: '图片通知',
additionalText: '我是补充标题',
briefText: '我是简明信息',
expandedTitle: '扩展消息',
picture: imagePixelMap
}
}
}
notificationManager.publish(notificationRequest, err => { // 官方解释 :2024.06.30 图片类型通知。(预留能力,暂未支持)。
if (err) {
console.error(`图片类型通知发布失败: ${err}`)
return;
}
console.info('图片类型通知发布成功')
})
})
}
}
})
}
function progress() {
let progress = 1;
// for (let i = 0; i < 100; i++) {
// setTimeout(() => {
// progress += 1;
//
// }, 100);
// }
//需要先查询系统是否支持进度条模板
notificationManager.isSupportTemplate('downloadTemplate').then((data) => {
let isSupport: boolean = data;
if (isSupport) {
let notificationRequest = {
id: 5,
content: {
contentType: notificationManager.ContentType.NOTIFICATION_CONTENT_BASIC_TEXT,
normal: {
title: '下载通知',
text: '我正在下载',
additionalText: '下载通知标题'
}
},
template: { // 构造进度条模板,name字段当前需要固定配置为 downloadTemplate
name: 'downloadTemplate',
data: {
title: '文档下载',
fileName: '阿吉',
progressValue: 22
}
}
}
notificationManager.publish(notificationRequest, err => {
if (err) {
console.error(`下载进度提醒失败 ${err}`)
return;
}
console.info('下载进度提醒正常')
})
}
}).catch(err => {
console.error(`暂不支持:${err.message}`)
})
}
function action() {
let wantAgentObj = null; //用于保存创建成功的wantAgent对象,后续使用其完成触发的动作
let wantAgentInfo = { //通过 wantAgentInfo的operationType设置动作类型
wants: [
{
deviceId: '',
bundleName: 'com.aji.first',
abilityName: 'com.aji.first.SecondAbility',
action: '',
entities: [],
uri: '',
parameters: {}
}
],
operationType: wantAgent.OperationType.START_ABILITY,
requestCode: 0,
wantAgentFlags: [wantAgent.WantAgentFlags.CONSTANT_FLAG]
}
wantAgent.getWantAgent(wantAgentInfo, (err, data) => {
if (err) {
console.error('失败: ' + JSON.stringify(err))
} else {
wantAgentObj = data;
}
})
let notificationRequest = {6
id: 6,
content: {
contentType: notificationManager.ContentType.NOTIFICATION_CONTENT_BASIC_TEXT,
normal: {
title: '标题',
text: '进入应用',
additionalText: '欢迎再次使用'
},
label: '阿吉',
wantAgent: wantAgentObj
}
}
notificationManager.publish(notificationRequest, err => {
if (err) {
console.error('发布失败' + err)
return;
}
console.info('发布成功')
})
}
@Entry
@Component
struct Index {
build() {
Column() {
Button('普通文本类型').onClick(() => {
basicText();
})
.margin(20)
Button('长文本类型').onClick(() => {
longText();
})
.margin(20)
Button('多行文本类型').onClick(() => {
multiline();
})
.margin(20)
Button('图片类型').onClick(() => {
picture();
})
.margin(20)
Button('进度条类型').onClick(() => {
progress();
})
.margin(20)
Button('带意图类型').onClick(() => {
action();
})
.margin(20)
}
.width('100%')
.height('100%')
}
}