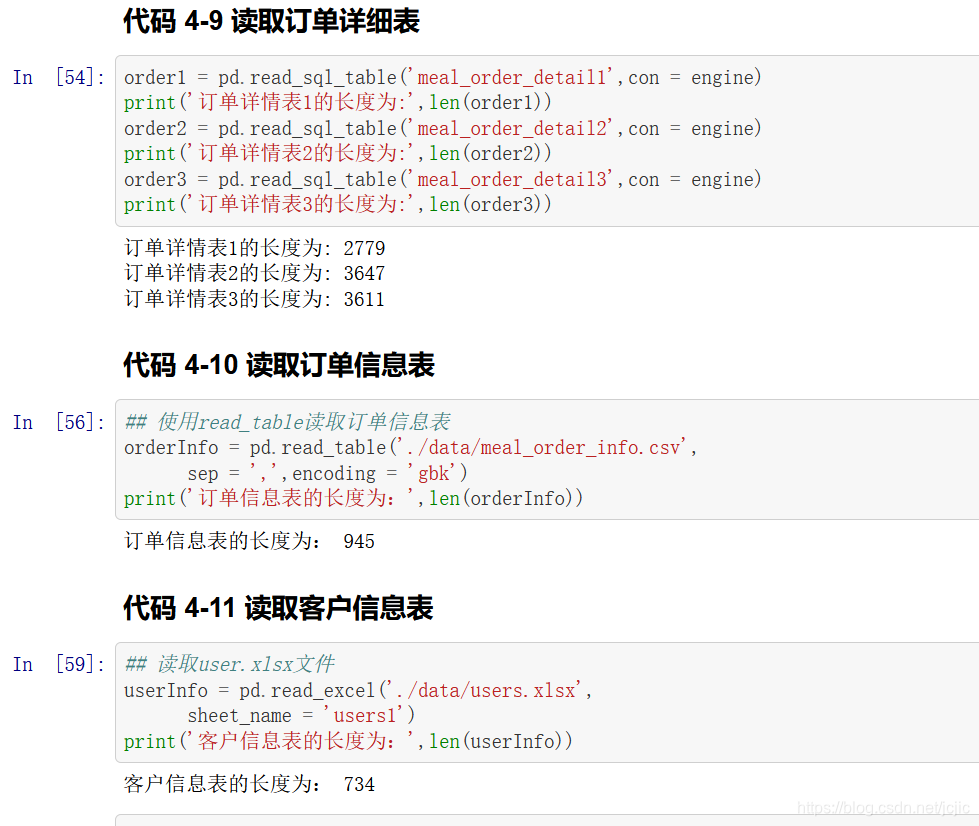
pandas_任务4.1 读写不同数据源的数据
ipynb格式浏览
代码4-1 SQLAlchemy连接MYSQL数据库的代码
from sqlalchemy import create_engine
import pandas as pd
engine = create_engine('mysql+pymysql://root:123456@localhost:3306/zuoye')
print(engine)
Engine(mysql+pymysql://root:***@localhost:3306/zuoye)
代码4-2 使用read_sql_table, read_sql_query,read_sql 函数读取数据库数据
formlist = pd.read_sql_query('show tables', con = engine)
print('testdb数据库数据表清单:','\n',formlist)
testdb数据库数据表清单:
Tables_in_zuoye
0 meal_order_detail1
1 meal_order_detail2
2 meal_order_detail3
D:\Study\anaconda\lib\site-packages\pymysql\cursors.py:170: Warning: (1366, "Incorrect string value: '\\xD6\\xD0\\xB9\\xFA\\xB1\\xEA...' for column 'VARIABLE_VALUE' at row 1")
result = self._query(query)
detail1 = pd.read_sql_table('meal_order_detail1',con=engine)
print('使用read_sql_table读取订单详情表的长度为:',len(detail1))
使用read_sql_table读取订单详情表的长度为: 2779
detail2 = pd.read_sql('select * from meal_order_detail2',
con = engine)
print('使用read_sql函数+sql语句读取的订单详情表长度为:',len(detail2))
detail3 = pd.read_sql('meal_order_detail3',con = engine)
print('使用read_sql函数+表格名称读取的订单详情表长度为:',
len(detail3))
使用read_sql函数+sql语句读取的订单详情表长度为: 3647
使用read_sql函数+表格名称读取的订单详情表长度为: 3611
代码4-3 使用to_sql方法写入数据
detail1.to_sql('test1',con = engine,index = False,
if_exists = 'replace')
formlist1 = pd.read_sql_query('show tables',con = engine)
print('新增一个表格后testdb数据库数据表清单为:','\n',formlist1)
新增一个表格后testdb数据库数据表清单为:
Tables_in_zuoye
0 meal_order_detail1
1 meal_order_detail2
2 meal_order_detail3
3 test1
代码4-4 使用read_table 和read_csv函数读取菜品订单信息表
order = pd.read_table('./data/meal_order_info.csv',
sep = ',',encoding = 'gbk')
print('使用read_table读取的订单信息表的长度为:',len(order))
使用read_table读取的订单信息表的长度为: 945
order1 = pd.read_csv('./data/meal_order_info.csv',
encoding = 'gbk')
print('使用read_csv读取的订单信息表的长度为:',len(order1))
使用read_csv读取的订单信息表的长度为: 945
4-5 更改参数读取菜品订单信息表
order2 = pd.read_table('./data/meal_order_info.csv',
sep = ';',encoding = 'gbk')
print('分隔符为;时订单信息表为:\n',order2)
分隔符为;时订单信息表为:
info_id,"emp_id","number_consumers","mode","dining_table_id","dining_table_name","expenditure","dishes_count","accounts_payable","use_start_time","check_closed","lock_time","cashier_id","pc_id","order_number","org_id","print_doc_bill_num","lock_table_info","order_status","phone","name"
0 417,1442,4,NA,1501,1022,165,5,165,"2016/8/1 11...
1 301,1095,3,NA,1430,1031,321,6,321,"2016/8/1 11...
2 413,1147,6,NA,1488,1009,854,15,854,"2016/8/1 1...
3 415,1166,4,NA,1502,1023,466,10,466,"2016/8/1 1...
4 392,1094,10,NA,1499,1020,704,24,704,"2016/8/1 ...
.. ...
940 641,1095,8,NA,1492,1013,679,12,679,"2016/8/31 ...
941 672,1089,6,NA,1489,1010,800,24,800,"2016/8/31 ...
942 692,1155,8,NA,1492,1013,735,10,735,"2016/8/31 ...
943 647,1094,4,NA,1485,1006,262,9,262,"2016/8/31 2...
944 570,1113,8,NA,1517,1038,589,13,589,"2016/8/31 ...
[945 rows x 1 columns]
order3 = pd.read_csv('./data/meal_order_info.csv',
sep = ',',header = None,encoding = 'gbk')
print('订单信息表为:','\n',order3)
订单信息表为:
0 1 2 3 4 \
0 info_id emp_id number_consumers mode dining_table_id
1 417 1442 4 NaN 1501
2 301 1095 3 NaN 1430
3 413 1147 6 NaN 1488
4 415 1166 4 NaN 1502
.. ... ... ... ... ...
941 641 1095 8 NaN 1492
942 672 1089 6 NaN 1489
943 692 1155 8 NaN 1492
944 647 1094 4 NaN 1485
945 570 1113 8 NaN 1517
5 6 7 8 \
0 dining_table_name expenditure dishes_count accounts_payable
1 1022 165 5 165
2 1031 321 6 321
3 1009 854 15 854
4 1023 466 10 466
.. ... ... ... ...
941 1013 679 12 679
942 1010 800 24 800
943 1013 735 10 735
944 1006 262 9 262
945 1038 589 13 589
9 ... 11 12 13 \
0 use_start_time ... lock_time cashier_id pc_id
1 2016/8/1 11:05:36 ... 2016/8/1 11:11:46 NaN NaN
2 2016/8/1 11:15:57 ... 2016/8/1 11:31:55 NaN NaN
3 2016/8/1 12:42:52 ... 2016/8/1 12:54:37 NaN NaN
4 2016/8/1 12:51:38 ... 2016/8/1 13:08:20 NaN NaN
.. ... ... ... ... ...
941 2016/8/31 21:23:48 ... 2016/8/31 21:31:48 NaN NaN
942 2016/8/31 21:24:12 ... 2016/8/31 21:56:12 NaN NaN
943 2016/8/31 21:25:18 ... 2016/8/31 21:33:34 NaN NaN
944 2016/8/31 21:37:39 ... 2016/8/31 21:55:39 NaN NaN
945 2016/8/31 21:41:56 ... 2016/8/31 21:32:56 NaN NaN
14 15 16 17 18 \
0 order_number org_id print_doc_bill_num lock_table_info order_status
1 NaN 330 NaN NaN 1
2 NaN 328 NaN NaN 1
3 NaN 330 NaN NaN 1
4 NaN 330 NaN NaN 1
.. ... ... ... ... ...
941 NaN 330 NaN NaN 1
942 NaN 330 NaN NaN 1
943 NaN 330 NaN NaN 1
944 NaN 330 NaN NaN 1
945 NaN 330 NaN NaN 1
19 20
0 phone name
1 18688880641 苗宇怡
2 18688880174 赵颖
3 18688880276 徐毅凡
4 18688880231 张大鹏
.. ... ...
941 18688880307 李靖
942 18688880305 莫言
943 18688880327 习一冰
944 18688880207 章春华
945 18688880313 唐雅嘉
[946 rows x 21 columns]
order4 = pd.read_csv('./data/meal_order_info1.csv',
sep = ',',encoding = 'utf-8')
代码4-6 使用to_csv函数将数据写入CSV文件中
import os
print('订单信息表写入文本文件前目录内文件列表为:\n',
os.listdir('./tmp'))
order.to_csv('./tmp/orderInfo.csv',sep = ';',index = False)
print('订单信息表写入文本文件后目录内文件列表为:\n',
os.listdir('./tmp'))
订单信息表写入文本文件前目录内文件列表为:
['1996~2015年人口数据特征间关系箱线图.png', '1996~2015年末人口分布特征散点图和折线图.png.png', '1996与2015年人口数据特征间关系直方图.png', '1996与2015年人口数据特征间关系饼状图.png', 'orderInfo.csv', '人口与时间的散点图.png']
订单信息表写入文本文件后目录内文件列表为:
['1996~2015年人口数据特征间关系箱线图.png', '1996~2015年末人口分布特征散点图和折线图.png.png', '1996与2015年人口数据特征间关系直方图.png', '1996与2015年人口数据特征间关系饼状图.png', 'orderInfo.csv', '人口与时间的散点图.png']
代码4-7 使用read_excel函数读取菜品订单信息表
user = pd.read_excel('./data/users.xlsx')
print('客户信息表长度为:',len(user))
客户信息表长度为: 734
代码 4-8 使用to_excel函数将数据存储为Excel文件
print('客户信息表写入excel文件前目录内文件列表为:\n',
os.listdir('./tmp'))
user.to_excel('./tmp/userInfo.xlsx')
print('客户信息表写入excel文件后目录内文件列表为:\n',
os.listdir('./tmp'))
客户信息表写入excel文件前目录内文件列表为:
['1996~2015年人口数据特征间关系箱线图.png', '1996~2015年末人口分布特征散点图和折线图.png.png', '1996与2015年人口数据特征间关系直方图.png', '1996与2015年人口数据特征间关系饼状图.png', 'orderInfo.csv', '人口与时间的散点图.png']
客户信息表写入excel文件后目录内文件列表为:
['1996~2015年人口数据特征间关系箱线图.png', '1996~2015年末人口分布特征散点图和折线图.png.png', '1996与2015年人口数据特征间关系直方图.png', '1996与2015年人口数据特征间关系饼状图.png', 'orderInfo.csv', 'userInfo.xlsx', '人口与时间的散点图.png']
代码 4-9 读取订单详细表
order1 = pd.read_sql_table('meal_order_detail1',con = engine)
print('订单详情表1的长度为:',len(order1))
order2 = pd.read_sql_table('meal_order_detail2',con = engine)
print('订单详情表2的长度为:',len(order2))
order3 = pd.read_sql_table('meal_order_detail3',con = engine)
print('订单详情表3的长度为:',len(order3))
订单详情表1的长度为: 2779
订单详情表2的长度为: 3647
订单详情表3的长度为: 3611
代码 4-10 读取订单信息表
orderInfo = pd.read_table('./data/meal_order_info.csv',
sep = ',',encoding = 'gbk')
print('订单信息表的长度为:',len(orderInfo))
订单信息表的长度为: 945
代码 4-11 读取客户信息表
userInfo = pd.read_excel('./data/users.xlsx',
sheet_name = 'users1')
print('客户信息表的长度为:',len(userInfo))
客户信息表的长度为: 734