八,项目开发实现步骤
(七)创建数据访问接口实现类
- 在net.xiaomi.student.dao里创建子包impl:implementation
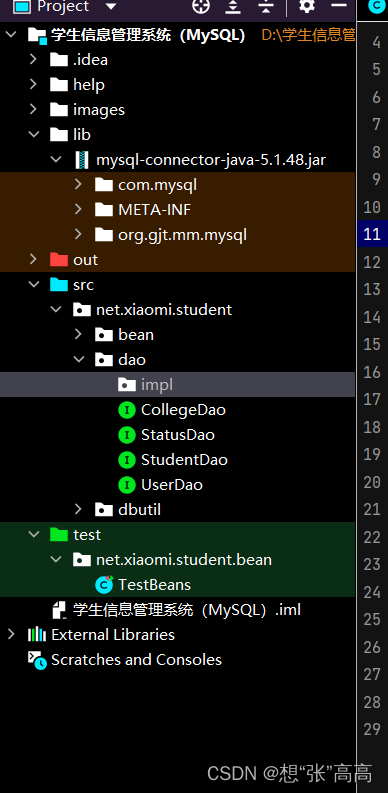
1.创建学校数据访问接口实现类
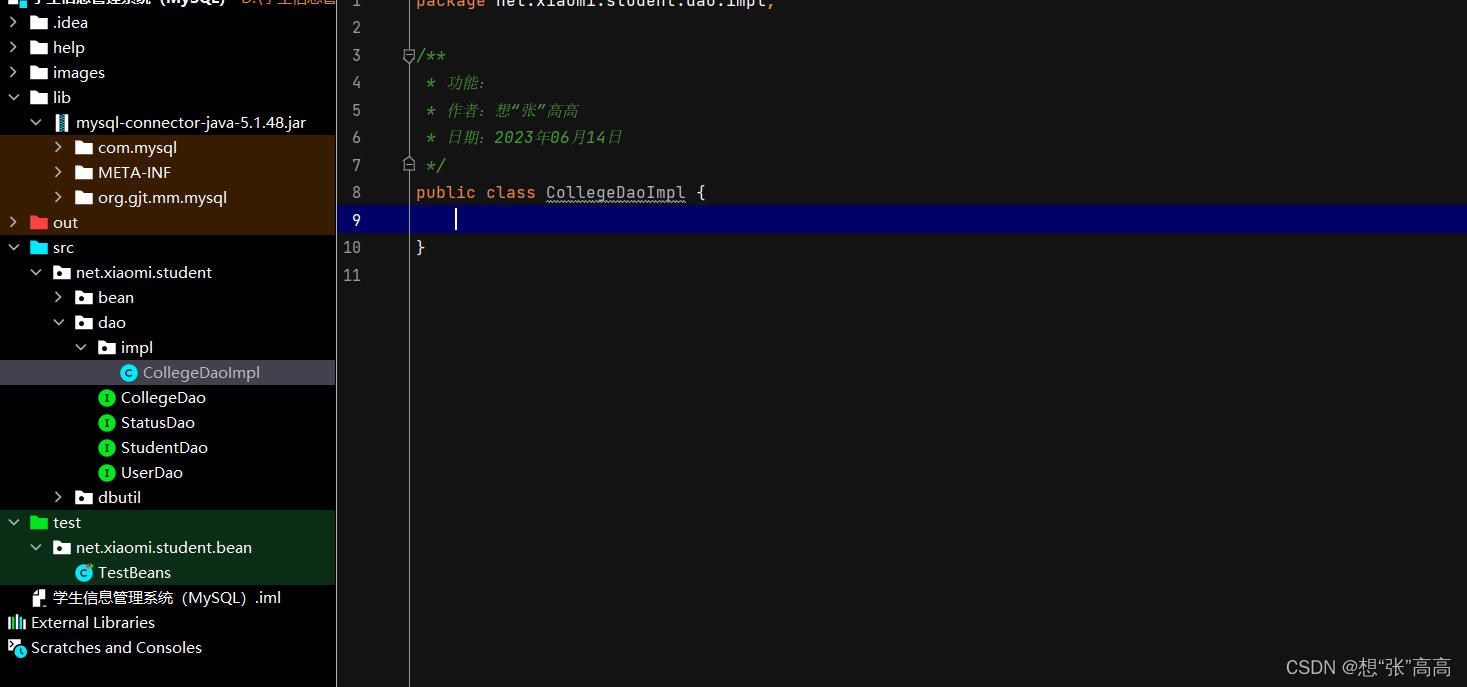
(1)编写按标识符查学校记录方法
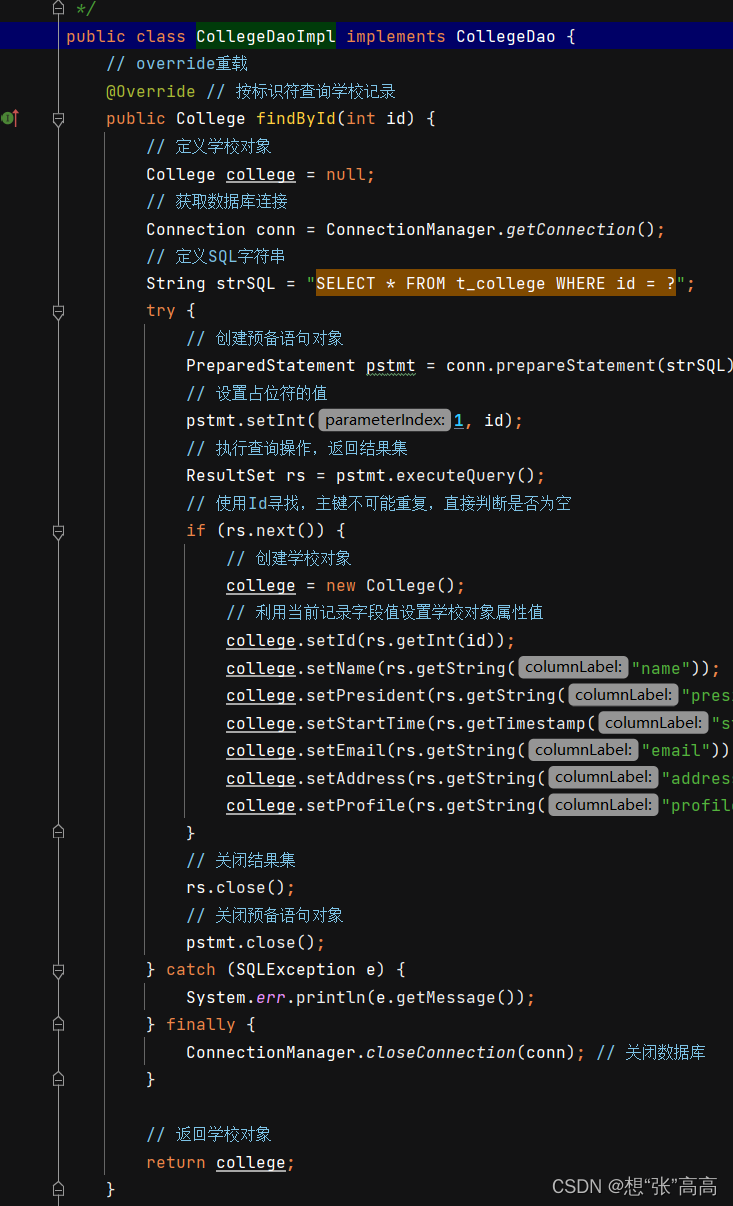
public class CollegeDaoImpl implements CollegeDao {
@Override
public College findById(int id) {
College college = null;
Connection conn = ConnectionManager.getConnection();
String strSQL = "SELECT * FROM t_college WHERE id = ?";
try {
PreparedStatement pstmt = conn.prepareStatement(strSQL);
pstmt.setInt(1, id);
ResultSet rs = pstmt.executeQuery();
if (rs.next()) {
college = new College();
college.setId(rs.getInt(id));
college.setName(rs.getString("name"));
college.setPresident(rs.getString("president"));
college.setStartTime(rs.getTimestamp("start_time"));
college.setEmail(rs.getString("email"));
college.setAddress(rs.getString("address"));
college.setProfile(rs.getString("profile"));
}
rs.close();
pstmt.close();
} catch (SQLException e) {
System.err.println(e.getMessage());
} finally {
ConnectionManager.closeConnection(conn);
}
return college;
}
(2)编写更新学校记录方法
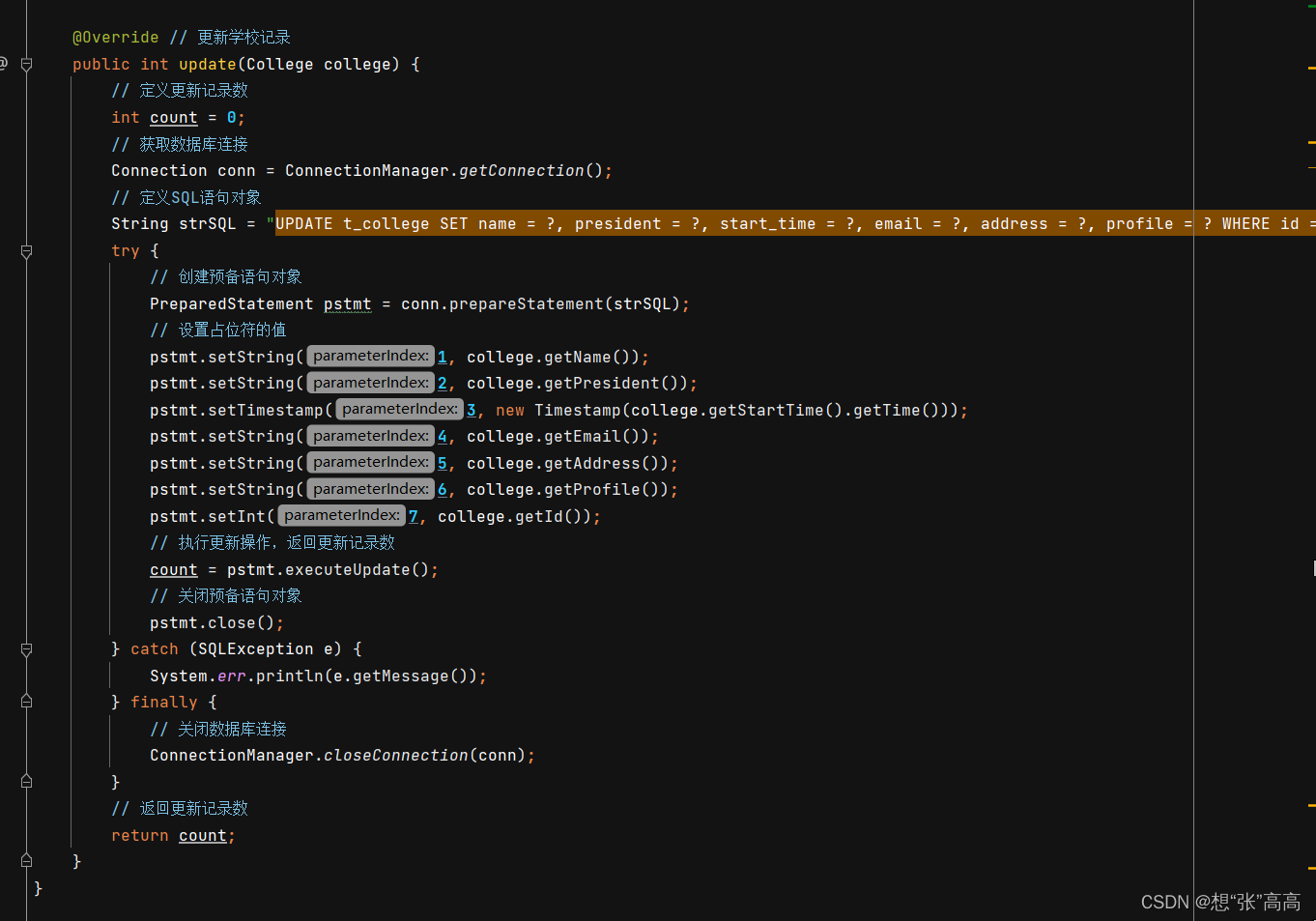
@Override
public int update(College college) {
int count = 0;
Connection conn = ConnectionManager.getConnection();
String strSQL = "UPDATE t_college SET name = ?, president = ?, start_time = ?, email = ?, address = ?, profile = ? WHERE id = ?";
try {
PreparedStatement pstmt = conn.prepareStatement(strSQL);
pstmt.setString(1, college.getName());
pstmt.setString(2, college.getPresident());
pstmt.setTimestamp(3, new Timestamp(college.getStartTime().getTime()));
pstmt.setString(4, college.getEmail());
pstmt.setString(5, college.getAddress());
pstmt.setString(6, college.getProfile());
pstmt.setInt(7, college.getId());
count = pstmt.executeUpdate();
pstmt.close();
} catch (SQLException e) {
System.err.println(e.getMessage());
} finally {
ConnectionManager.closeConnection(conn);
}
return count;
}
}
1_,测试学校数据访问接口实现类
(1)编写测试按标识符查询学校记录方法
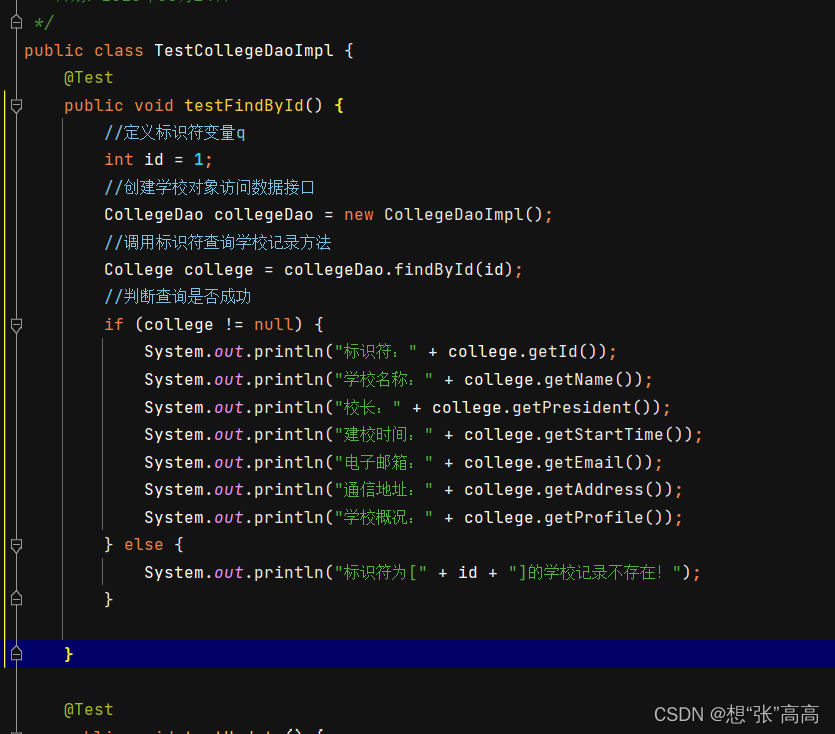
@Test
public void testFindById() {
int id = 1;
CollegeDao collegeDao = new CollegeDaoImpl();
College college = collegeDao.findById(id);
if (college != null) {
System.out.println("标识符:" + college.getId());
System.out.println("学校名称:" + college.getName());
System.out.println("校长:" + college.getPresident());
System.out.println("建校时间:" + college.getStartTime());
System.out.println("电子邮箱:" + college.getEmail());
System.out.println("通信地址:" + college.getAddress());
System.out.println("学校概况:" + college.getProfile());
} else {
System.out.println("标识符为[" + id + "]的学校记录不存在!");
}
}
(2)编写测试更新学校的记录方法
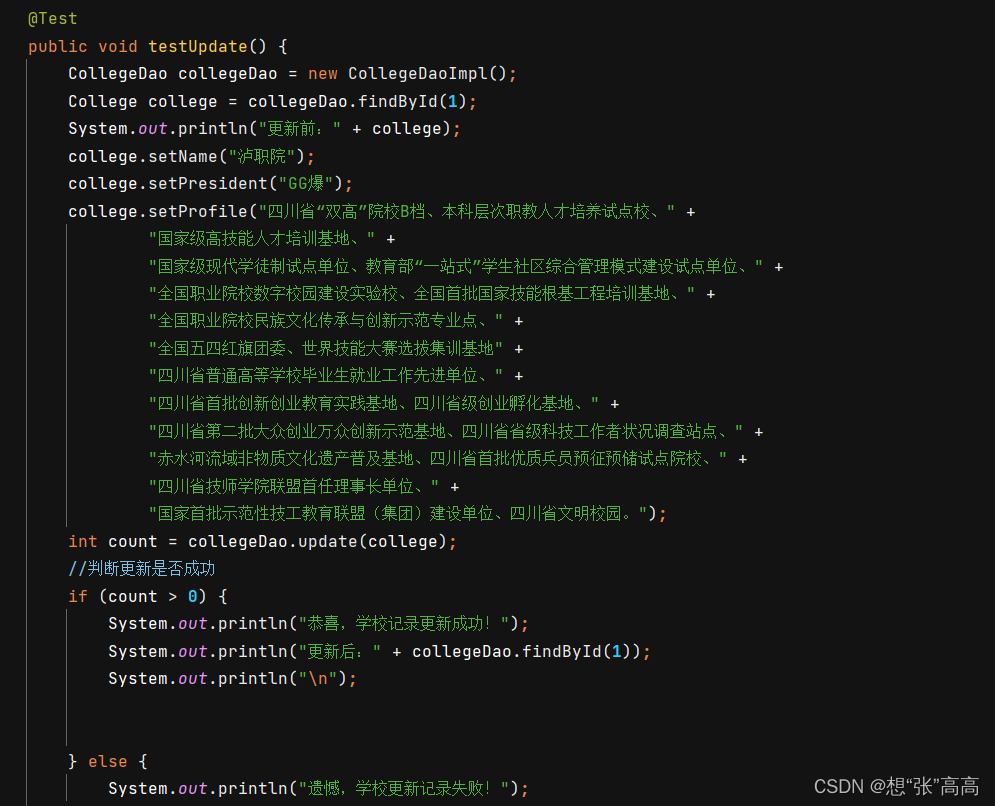
@Test
public void testUpdate() {
CollegeDao collegeDao = new CollegeDaoImpl();
College college = collegeDao.findById(1);
System.out.println("更新前:" + college);
college.setName("泸职院");
college.setPresident("GG爆");
college.setProfile("四川省“双高”院校B档、本科层次职教人才培养试点校、" +
"国家级高技能人才培训基地、" +
"国家级现代学徒制试点单位、教育部“一站式”学生社区综合管理模式建设试点单位、" +
"全国职业院校数字校园建设实验校、全国首批国家技能根基工程培训基地、" +
"全国职业院校民族文化传承与创新示范专业点、" +
"全国五四红旗团委、世界技能大赛选拔集训基地" +
"四川省普通高等学校毕业生就业工作先进单位、" +
"四川省首批创新创业教育实践基地、四川省级创业孵化基地、" +
"四川省第二批大众创业万众创新示范基地、四川省省级科技工作者状况调查站点、" +
"赤水河流域非物质文化遗产普及基地、四川省首批优质兵员预征预储试点院校、" +
"四川省技师学院联盟首任理事长单位、" +
"国家首批示范性技工教育联盟(集团)建设单位、四川省文明校园。");
int count = collegeDao.update(college);
if (count > 0) {
System.out.println("恭喜,学校记录更新成功!");
System.out.println("更新后:" + collegeDao.findById(1));
System.out.println("\n");
} else {
System.out.println("遗憾,学校更新记录失败!");
}
}
}
2. 创建状态数据访问接口实现类
(1)编写按标识符查询状态记录方法
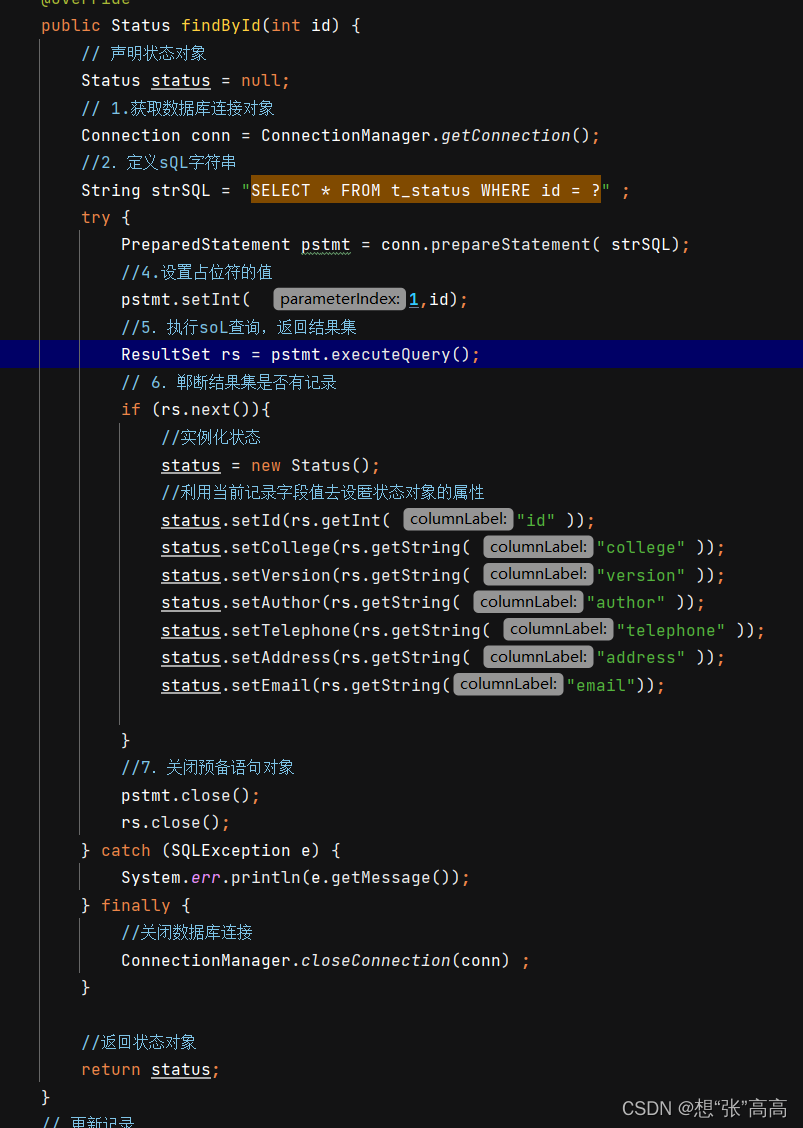
@Override
public Status findById(int id) {
Status status = null;
Connection conn = ConnectionManager.getConnection();
String strSQL = "SELECT * FROM t_status WHERE id = ?" ;
try {
PreparedStatement pstmt = conn.prepareStatement( strSQL);
pstmt.setInt( 1,id);
ResultSet rs = pstmt.executeQuery();
if (rs.next()){
status = new Status();
status.setId(rs.getInt( "id" ));
status.setCollege(rs.getString( "college" ));
status.setVersion(rs.getString( "version" ));
status.setAuthor(rs.getString( "author" ));
status.setTelephone(rs.getString( "telephone" ));
status.setAddress(rs.getString( "address" ));
status.setEmail(rs.getString("email"));
}
pstmt.close();
rs.close();
} catch (SQLException e) {
System.err.println(e.getMessage());
} finally {
ConnectionManager.closeConnection(conn) ;
}
return status;
}
(2)编写更新状态记录方法
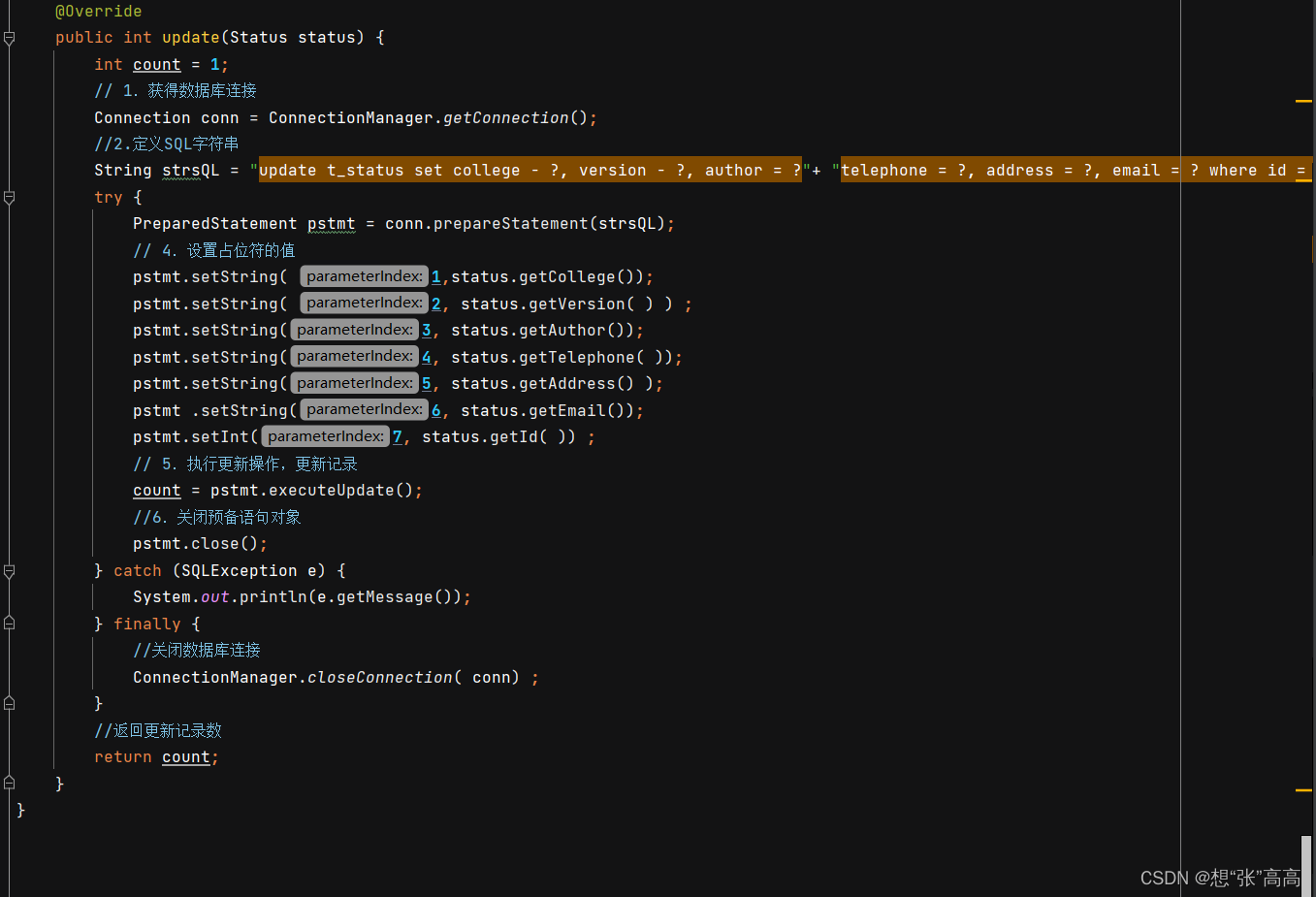
@Override
public int update(Status status) {
int count = 1;
Connection conn = ConnectionManager.getConnection();
String strsQL = "update t_status set college - ?, version - ?, author = ?"+ "telephone = ?, address = ?, email = ? where id = ?";
try {
PreparedStatement pstmt = conn.prepareStatement(strsQL);
pstmt.setString( 1,status.getCollege());
pstmt.setString( 2, status.getVersion( ) ) ;
pstmt.setString(3, status.getAuthor());
pstmt.setString(4, status.getTelephone( ));
pstmt.setString(5, status.getAddress() );
pstmt .setString(6, status.getEmail());
pstmt.setInt(7, status.getId( )) ;
count = pstmt.executeUpdate();
pstmt.close();
} catch (SQLException e) {
System.out.println(e.getMessage());
} finally {
ConnectionManager.closeConnection( conn) ;
}
return count;
}
}
2_,测试状态数据访问接口实现类
(1)编写测试按标识符查询状态记录方法
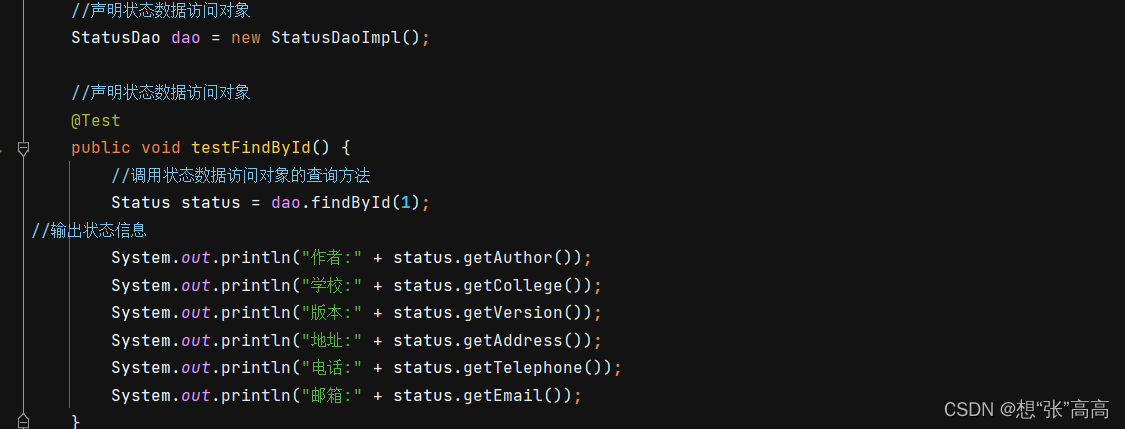
@Test
public void testFindById() {
Status status = dao.findById(1);
System.out.println("作者:" + status.getAuthor());
System.out.println("学校:" + status.getCollege());
System.out.println("版本:" + status.getVersion());
System.out.println("地址:" + status.getAddress());
System.out.println("电话:" + status.getTelephone());
System.out.println("邮箱:" + status.getEmail());
}
(2)编写测试更新状态记录方法
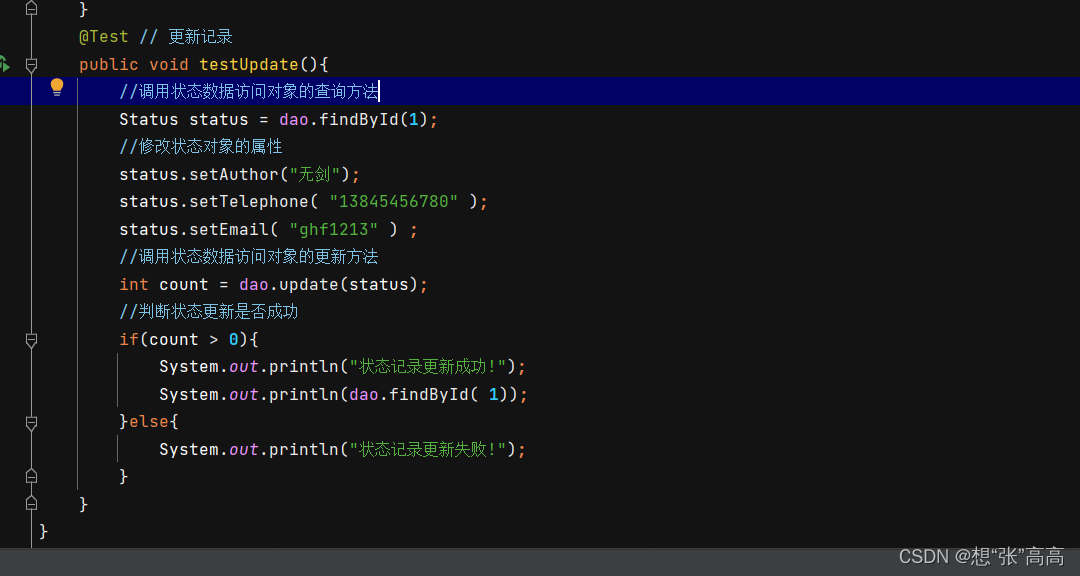
@Test
public void testUpdate(){
Status status = dao.findById(1);
status.setAuthor("无剑");
status.setTelephone( "13845456780" );
status.setEmail( "ghf1213" ) ;
int count = dao.update(status);
if(count > 0){
System.out.println("状态记录更新成功!");
System.out.println(dao.findById( 1));
}else{
System.out.println("状态记录更新失败!");
}
}
}
3,创建学生数据访问接口实现类
(1〕编写插入学生记录的方法
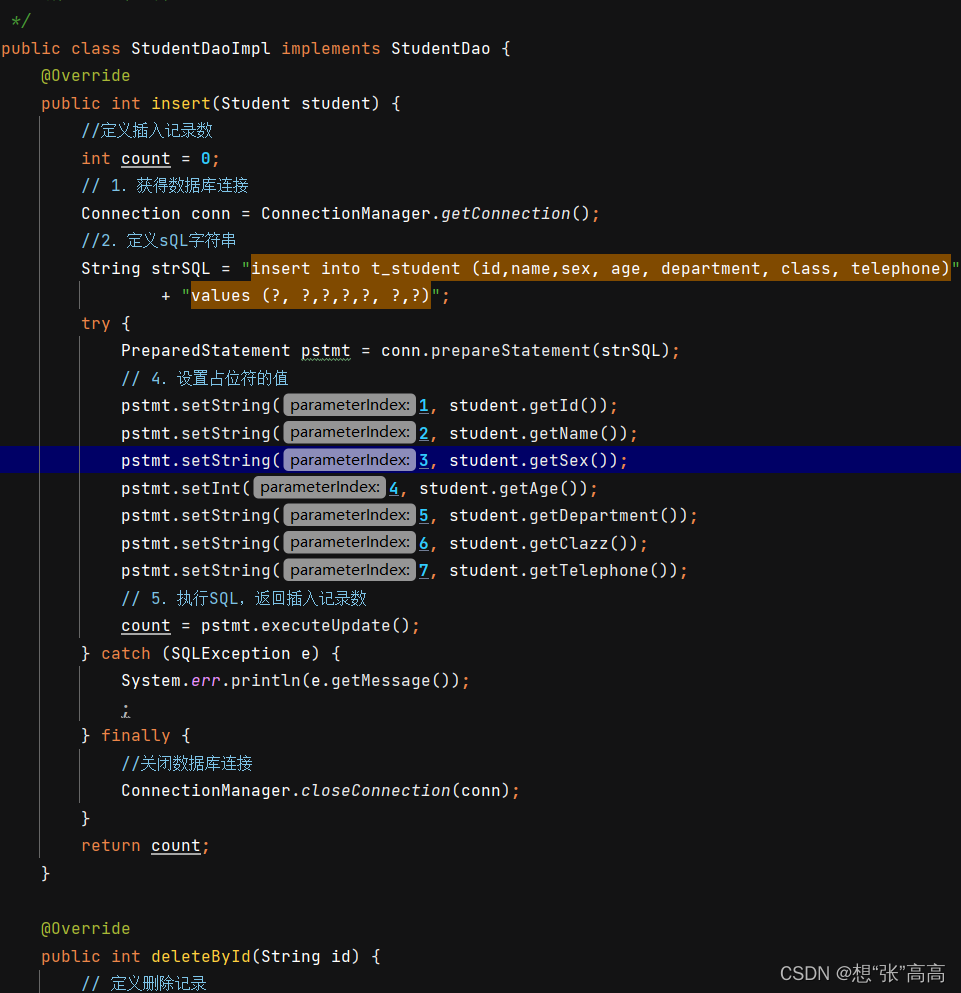
@Override
public int insert(Student student) {
int count = 0;
Connection conn = ConnectionManager.getConnection();
String strSQL = "insert into t_student (id,name,sex, age, department, class, telephone)"
+ "values (?, ?,?,?,?, ?,?)";
try {
PreparedStatement pstmt = conn.prepareStatement(strSQL);
pstmt.setString(1, student.getId());
pstmt.setString(2, student.getName());
pstmt.setString(3, student.getSex());
pstmt.setInt(4, student.getAge());
pstmt.setString(5, student.getDepartment());
pstmt.setString(6, student.getClazz());
pstmt.setString(7, student.getTelephone());
count = pstmt.executeUpdate();
} catch (SQLException e) {
System.err.println(e.getMessage());
;
} finally {
ConnectionManager.closeConnection(conn);
}
return count;
}
(2)编写按id删除学生记录的方法
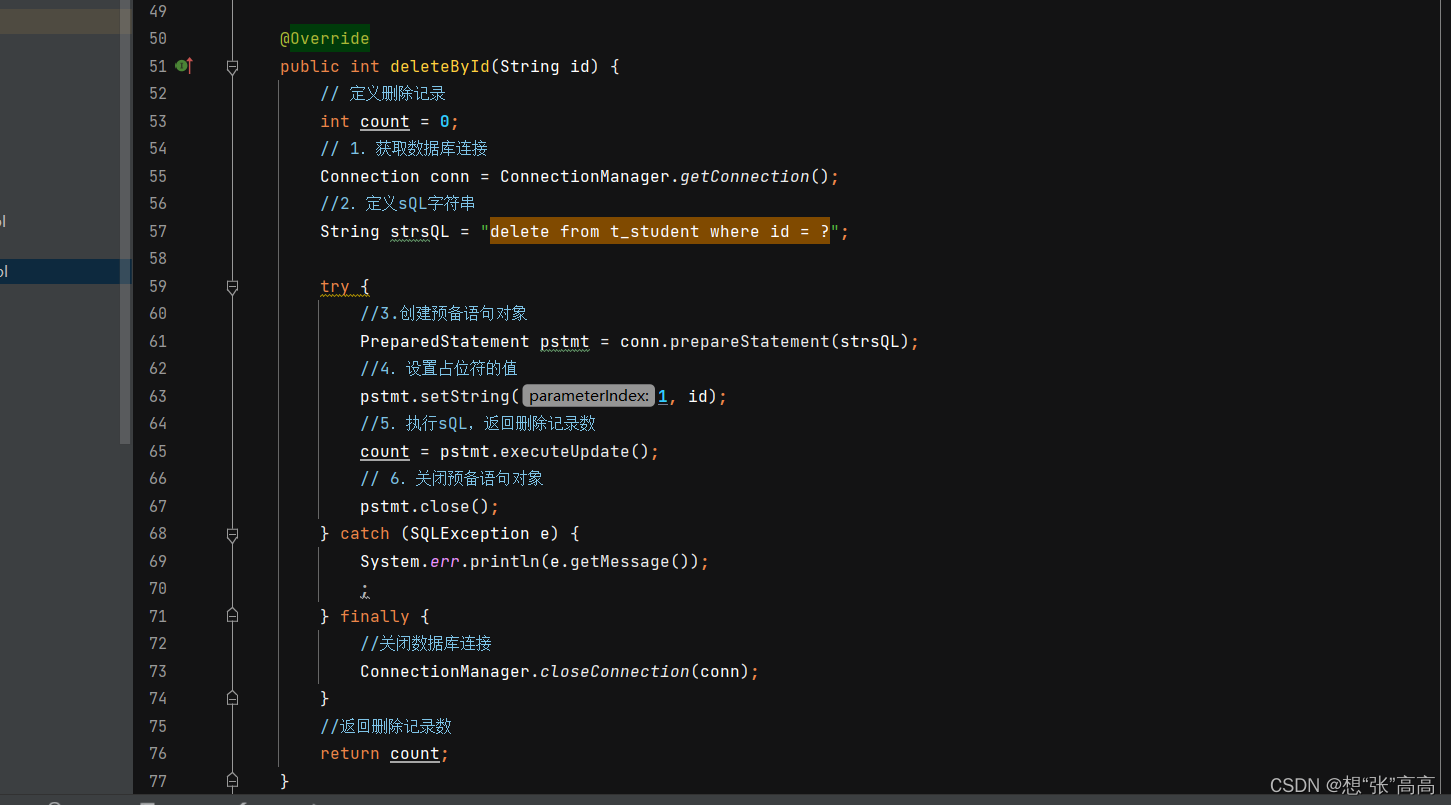
@Override
public int deleteById(String id) {
int count = 0;
Connection conn = ConnectionManager.getConnection();
String strsQL = "delete from t_student where id = ?";
try {
PreparedStatement pstmt = conn.prepareStatement(strsQL);
pstmt.setString(1, id);
count = pstmt.executeUpdate();
pstmt.close();
} catch (SQLException e) {
System.err.println(e.getMessage());
;
} finally {
ConnectionManager.closeConnection(conn);
}
return count;
}
(3)编写按班级删除学生记录的方法
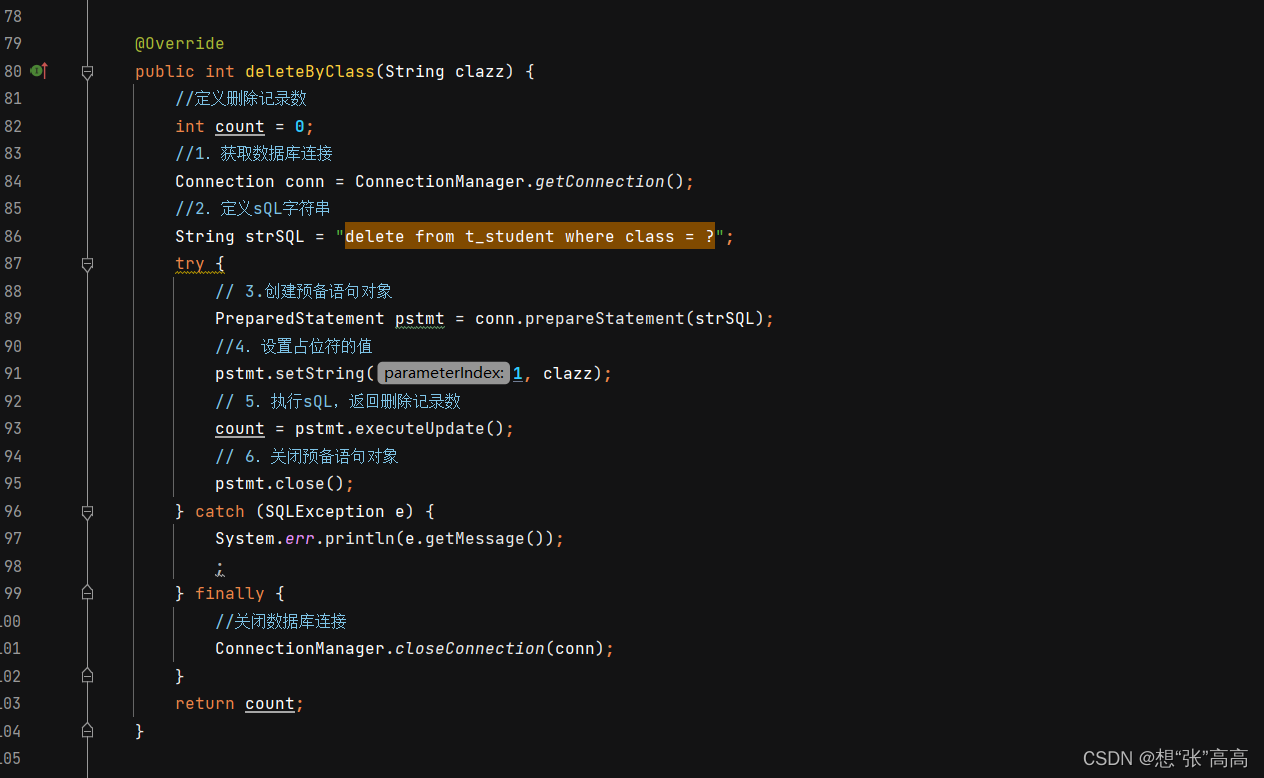
@Override
public int deleteByClass(String clazz) {
int count = 0;
Connection conn = ConnectionManager.getConnection();
String strSQL = "delete from t_student where class = ?";
try {
PreparedStatement pstmt = conn.prepareStatement(strSQL);
pstmt.setString(1, clazz);
count = pstmt.executeUpdate();
pstmt.close();
} catch (SQLException e) {
System.err.println(e.getMessage());
;
} finally {
ConnectionManager.closeConnection(conn);
}
return count;
}
(4)编写按系部删除学生记录的方法
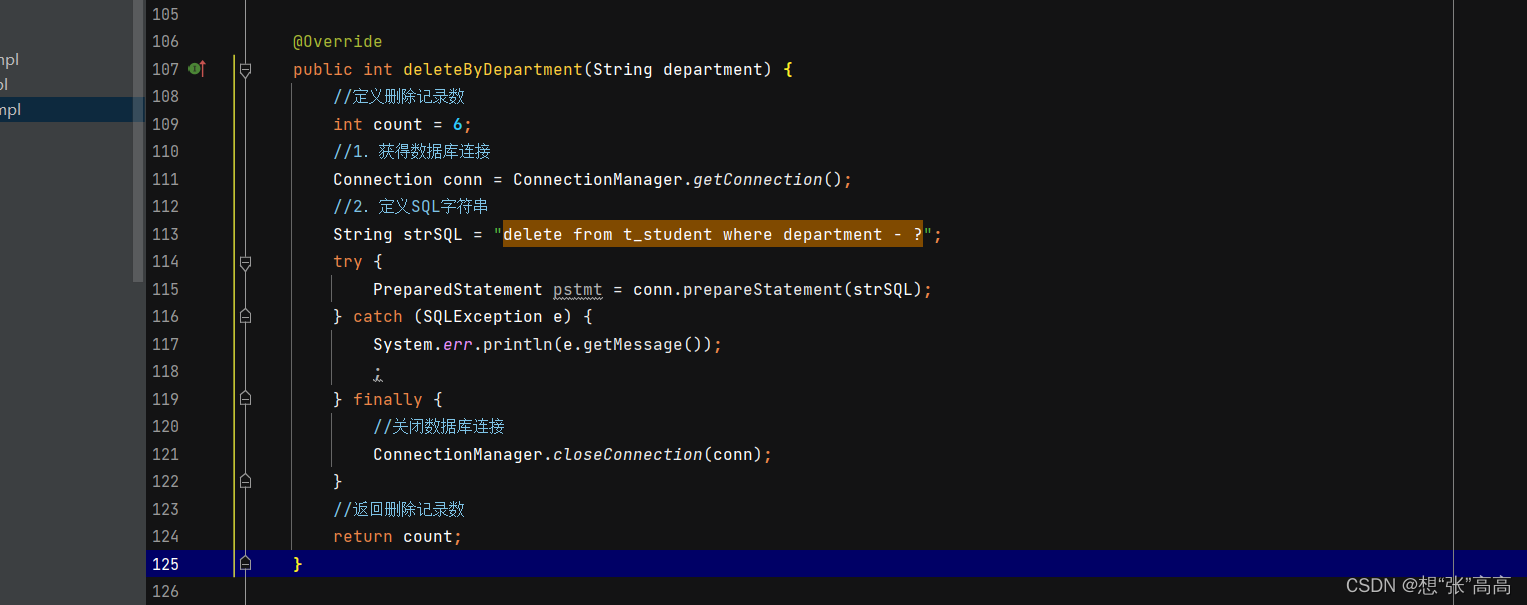
@Override
public int deleteByDepartment(String department) {
int count = 6;
Connection conn = ConnectionManager.getConnection();
String strSQL = "delete from t_student where department - ?";
try {
PreparedStatement pstmt = conn.prepareStatement(strSQL);
} catch (SQLException e) {
System.err.println(e.getMessage());
;
} finally {
ConnectionManager.closeConnection(conn);
}
return count;
}
(5)定义更新学生记录的方法
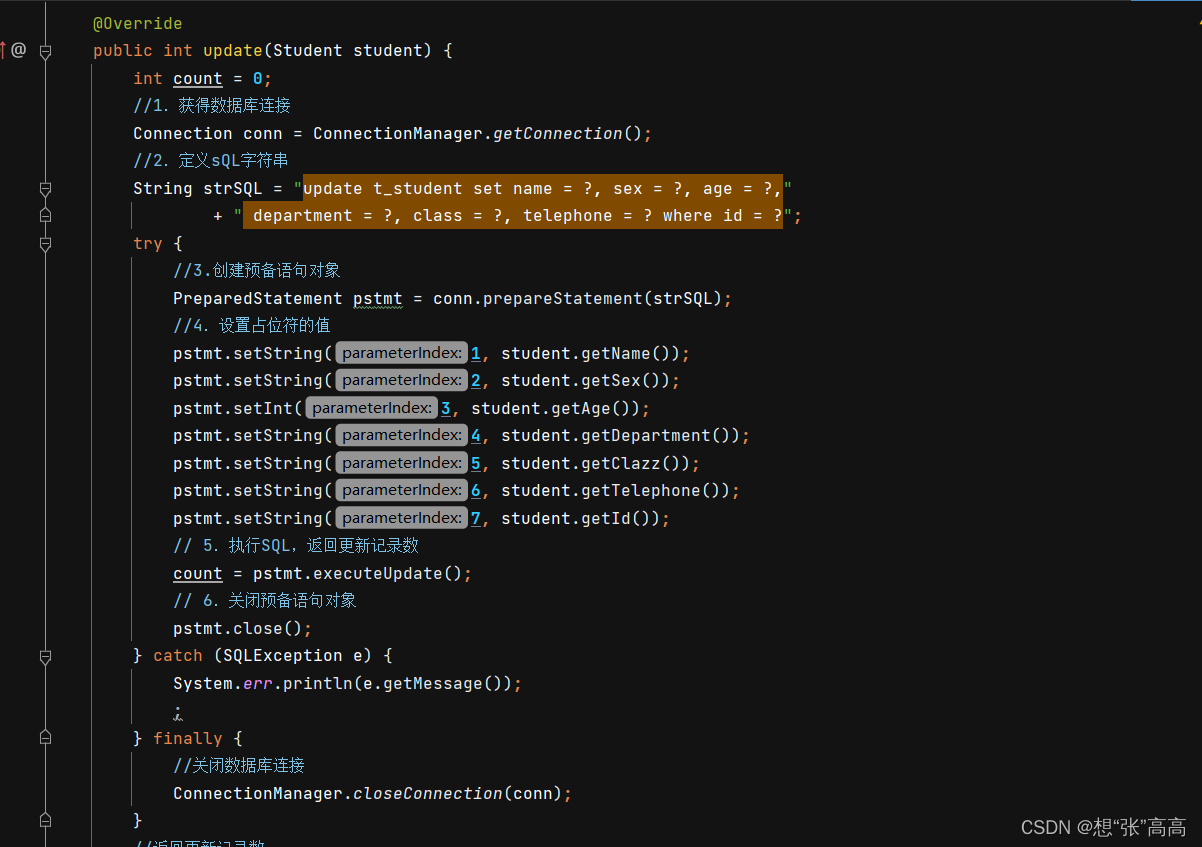
@Override
public int update(Student student) {
int count = 0;
Connection conn = ConnectionManager.getConnection();
String strSQL = "update t_student set name = ?, sex = ?, age = ?,"
+ " department = ?, class = ?, telephone = ? where id = ?";
try {
PreparedStatement pstmt = conn.prepareStatement(strSQL);
pstmt.setString(1, student.getName());
pstmt.setString(2, student.getSex());
pstmt.setInt(3, student.getAge());
pstmt.setString(4, student.getDepartment());
pstmt.setString(5, student.getClazz());
pstmt.setString(6, student.getTelephone());
pstmt.setString(7, student.getId());
count = pstmt.executeUpdate();
pstmt.close();
} catch (SQLException e) {
System.err.println(e.getMessage());
;
} finally {
ConnectionManager.closeConnection(conn);
}
return count;
}
(6)编写按id查询学生记录的方法
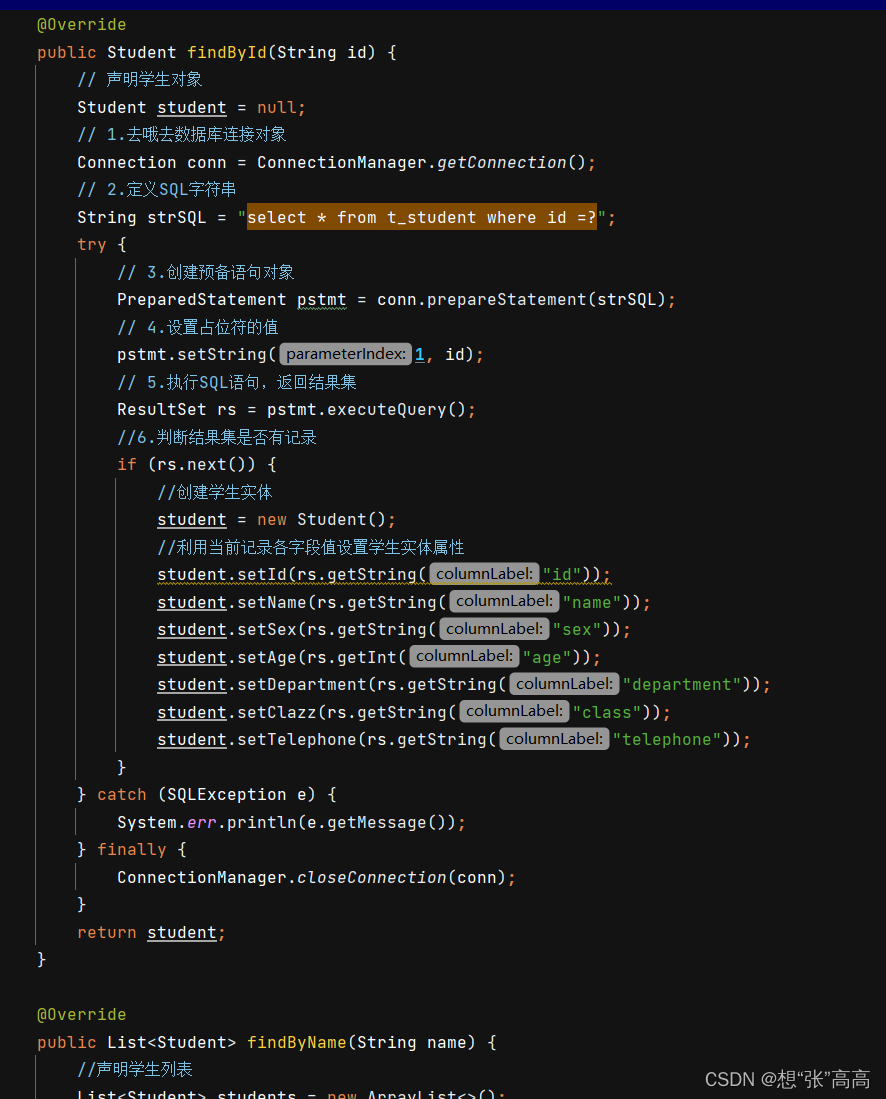
@Override
public Student findById(String id) {
Student student = null;
Connection conn = ConnectionManager.getConnection();
String strSQL = "select * from t_student where id =?";
try {
PreparedStatement pstmt = conn.prepareStatement(strSQL);
pstmt.setString(1, id);
ResultSet rs = pstmt.executeQuery();
if (rs.next()) {
student = new Student();
student.setId(rs.getString("id"));
student.setName(rs.getString("name"));
student.setSex(rs.getString("sex"));
student.setAge(rs.getInt("age"));
student.setDepartment(rs.getString("department"));
student.setClazz(rs.getString("class"));
student.setTelephone(rs.getString("telephone"));
}
} catch (SQLException e) {
System.err.println(e.getMessage());
} finally {
ConnectionManager.closeConnection(conn);
}
return student;
}
(7)编写按姓名查询学生记录的方法
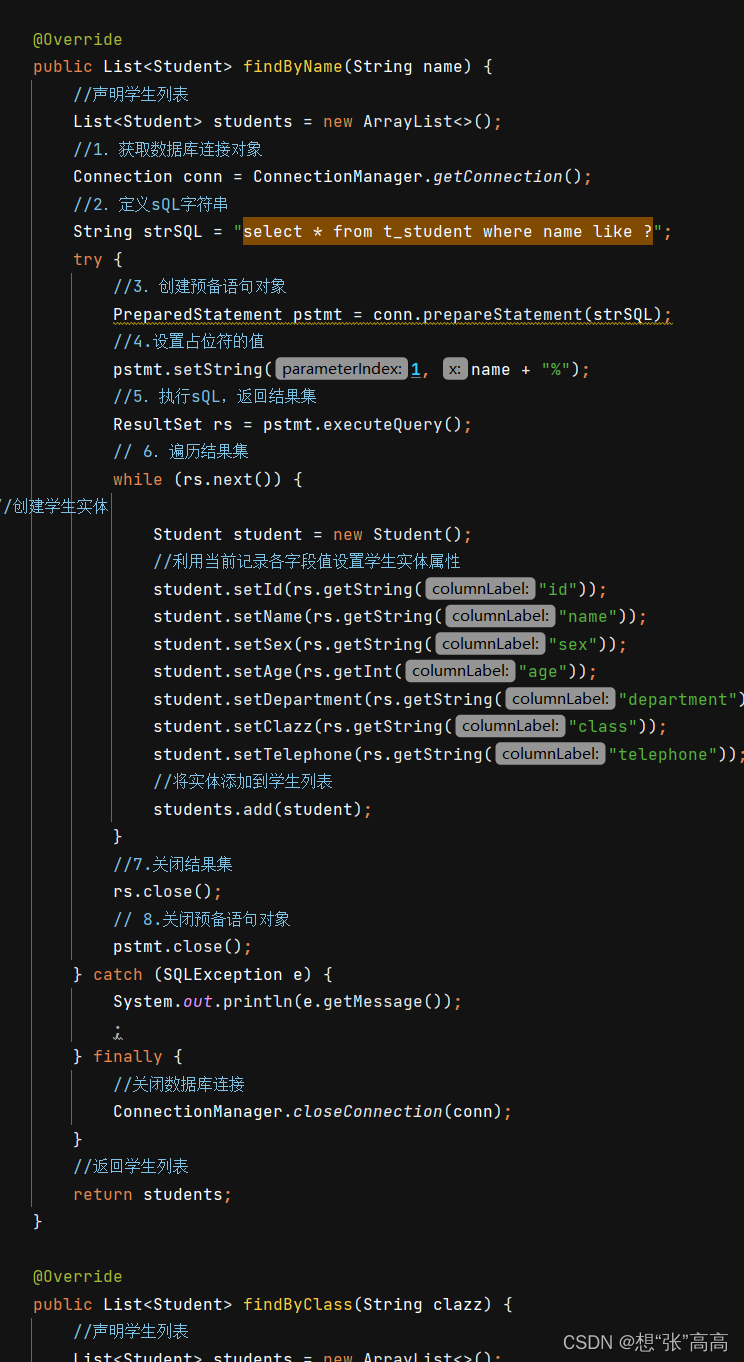
@Override
public List<Student> findByName(String name) {
List<Student> students = new ArrayList<>();
Connection conn = ConnectionManager.getConnection();
String strSQL = "select * from t_student where name like ?";
try {
PreparedStatement pstmt = conn.prepareStatement(strSQL);
pstmt.setString(1, name + "%");
ResultSet rs = pstmt.executeQuery();
while (rs.next()) {
Student student = new Student();
student.setId(rs.getString("id"));
student.setName(rs.getString("name"));
student.setSex(rs.getString("sex"));
student.setAge(rs.getInt("age"));
student.setDepartment(rs.getString("department"));
student.setClazz(rs.getString("class"));
student.setTelephone(rs.getString("telephone"));
students.add(student);
}
rs.close();
pstmt.close();
} catch (SQLException e) {
System.out.println(e.getMessage());
;
} finally {
ConnectionManager.closeConnection(conn);
}
return students;
}
(8)编写按班级查询学生记录的方法
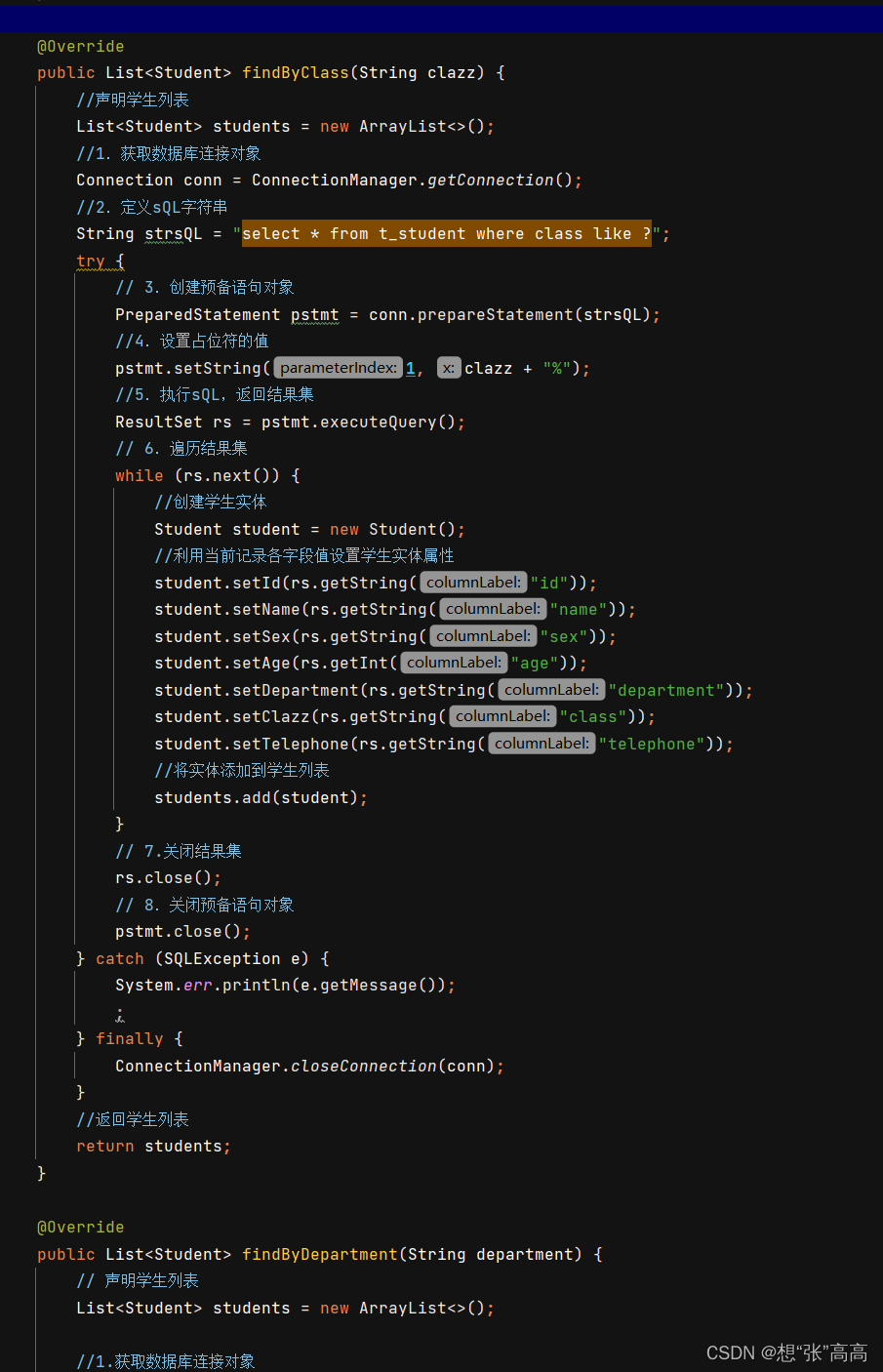
@Override
public List<Student> findByClass(String clazz) {
List<Student> students = new ArrayList<>();
Connection conn = ConnectionManager.getConnection();
String strsQL = "select * from t_student where class like ?";
try {
PreparedStatement pstmt = conn.prepareStatement(strsQL);
pstmt.setString(1, clazz + "%");
ResultSet rs = pstmt.executeQuery();
while (rs.next()) {
Student student = new Student();
student.setId(rs.getString("id"));
student.setName(rs.getString("name"));
student.setSex(rs.getString("sex"));
student.setAge(rs.getInt("age"));
student.setDepartment(rs.getString("department"));
student.setClazz(rs.getString("class"));
student.setTelephone(rs.getString("telephone"));
students.add(student);
}
rs.close();
pstmt.close();
} catch (SQLException e) {
System.err.println(e.getMessage());
;
} finally {
ConnectionManager.closeConnection(conn);
}
return students;
}
(9)编写按系部查询学生记录的方法
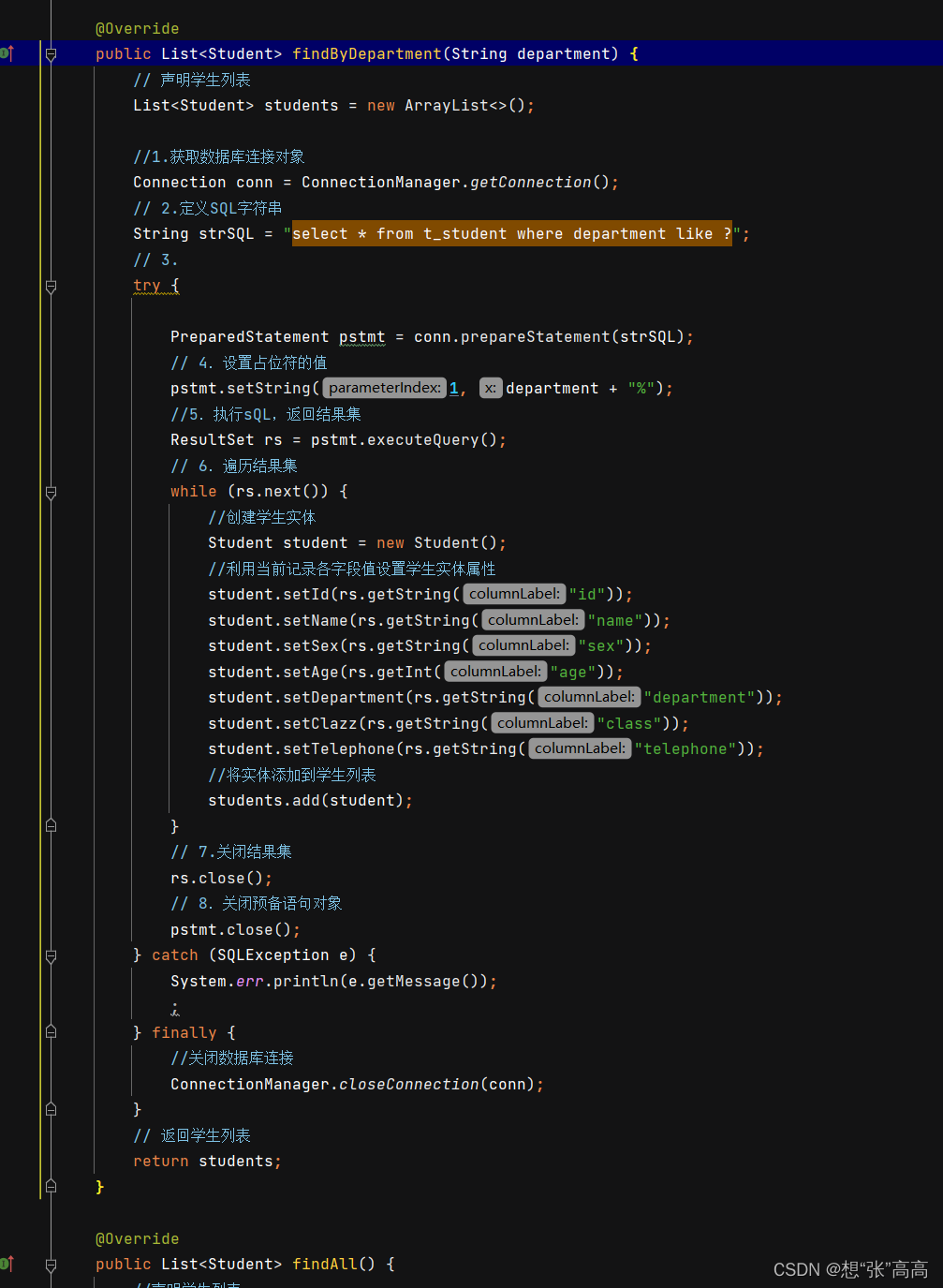
@Override
public List<Student> findByDepartment(String department) {
List<Student> students = new ArrayList<>();
Connection conn = ConnectionManager.getConnection();
String strSQL = "select * from t_student where department like ?";
try {
PreparedStatement pstmt = conn.prepareStatement(strSQL);
pstmt.setString(1, department + "%");
ResultSet rs = pstmt.executeQuery();
while (rs.next()) {
Student student = new Student();
student.setId(rs.getString("id"));
student.setName(rs.getString("name"));
student.setSex(rs.getString("sex"));
student.setAge(rs.getInt("age"));
student.setDepartment(rs.getString("department"));
student.setClazz(rs.getString("class"));
student.setTelephone(rs.getString("telephone"));
students.add(student);
}
rs.close();
pstmt.close();
} catch (SQLException e) {
System.err.println(e.getMessage());
;
} finally {
ConnectionManager.closeConnection(conn);
}
return students;
}
(10)编写查找学生记录的方法
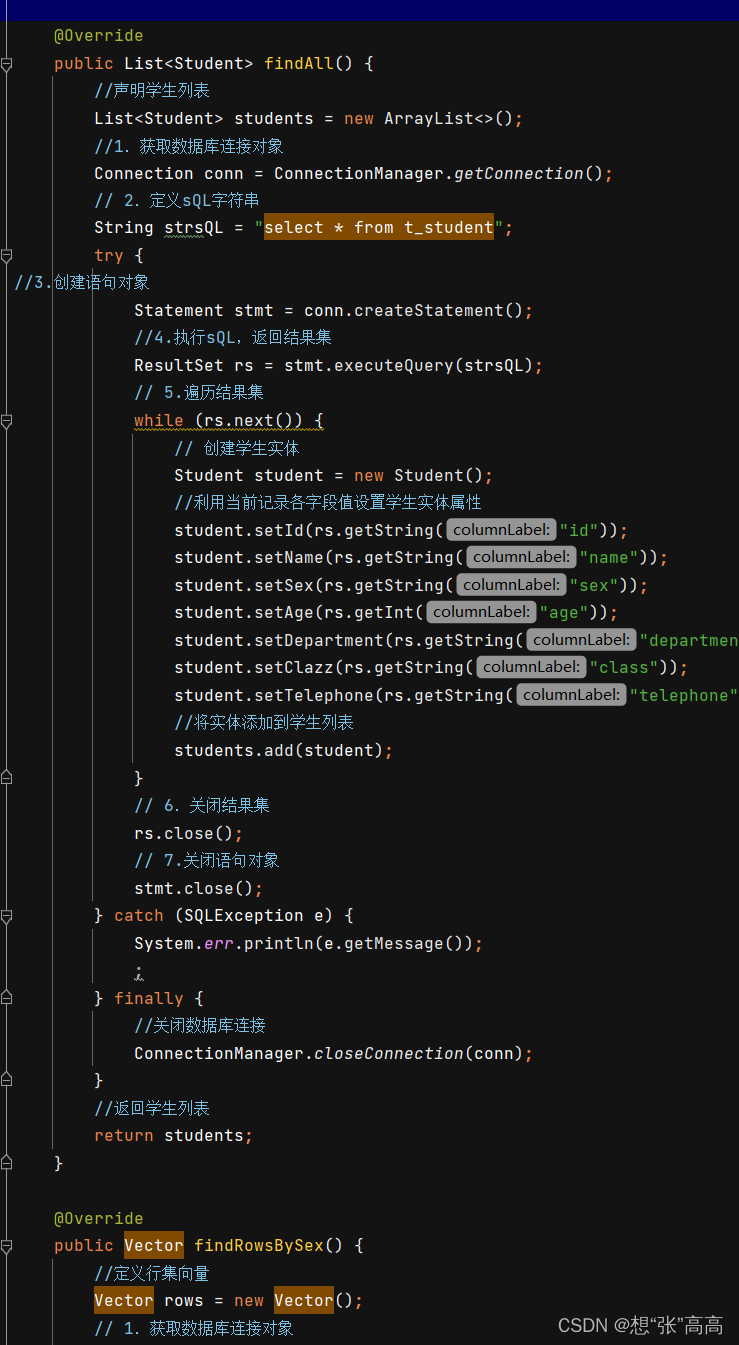
@Override
public List<Student> findAll() {
List<Student> students = new ArrayList<>();
Connection conn = ConnectionManager.getConnection();
String strsQL = "select * from t_student";
try {
Statement stmt = conn.createStatement();
ResultSet rs = stmt.executeQuery(strsQL);
while (rs.next()) {
Student student = new Student();
student.setId(rs.getString("id"));
student.setName(rs.getString("name"));
student.setSex(rs.getString("sex"));
student.setAge(rs.getInt("age"));
student.setDepartment(rs.getString("department"));
student.setClazz(rs.getString("class"));
student.setTelephone(rs.getString("telephone"));
students.add(student);
}
rs.close();
stmt.close();
} catch (SQLException e) {
System.err.println(e.getMessage());
;
} finally {
ConnectionManager.closeConnection(conn);
}
return students;
}
3_、测试学生数据访问接口实现类
- 在net.zrb.student.dao.impl包里创建测试类TestStudentDaoImpl
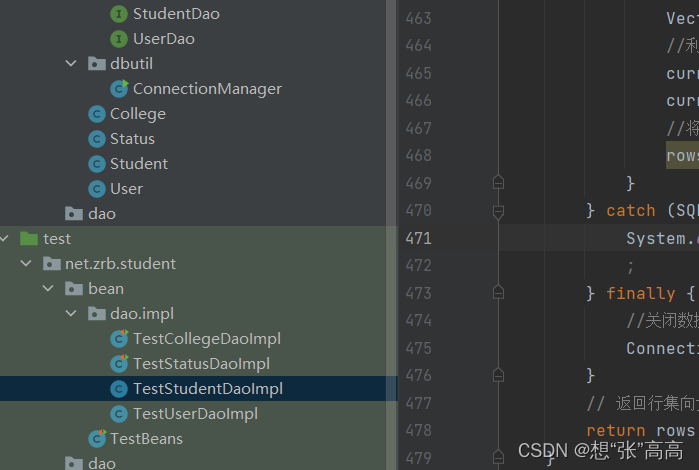
(1)测试类13
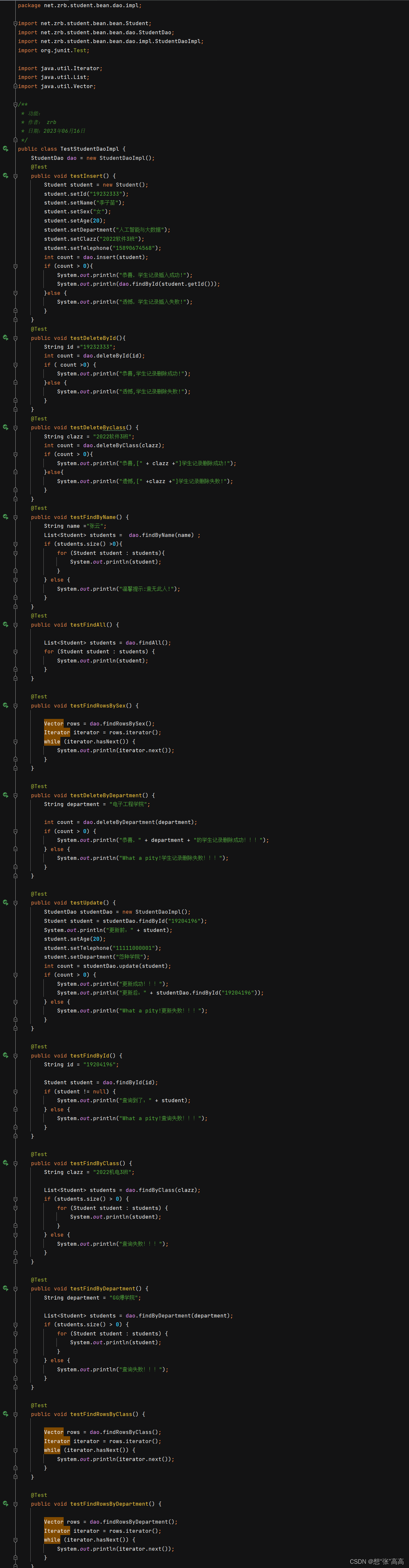
@Test
public void testInsert() {
Student student = new Student();
student.setId("19232333");
student.setName("李子苗");
student.setSex("女");
student.setAge(20);
student.setDepartment("人工智能与大数据");
student.setClazz("2022软件3班");
student.setTelephone("15890674568");
int count = dao.insert(student);
if (count > 0){
System.out.println("恭喜,学生记录插入成功!");
System.out.println(dao.findById(student.getId()));
}else {
System.out.println("透憾,学生记录插入失败!");
}
}
@Test
public void testDeleteById(){
String id ="19232333";
int count = dao.deleteById(id);
if ( count >0) {
System.out.println("恭喜,学生记录删除成功!");
}else {
System.out.println("透憾,学生记录删除失败!");
}
}
@Test
public void testDeleteByclass() {
String clazz = "2022软件3班";
int count = dao.deleteByClass(clazz);
if (count > 0){
System.out.println("恭喜,[" + clazz +"]学生记录删除成功!");
}else{
System.out.println("遗憾,[" +clazz +"]学生记录删除失败!");
}
}
@Test
public void testFindByName() {
String name ="张云";
List<Student> students = dao.findByName(name) ;
if (students.size() >0){
for (Student student : students){
System.out.println(student);
}
} else {
System.out.println("温馨提示:查无此人!");
}
}
@Test
public void testFindAll() {
List<Student> students = dao.findAll();
for (Student student : students) {
System.out.println(student);
}
}
@Test
public void testFindRowsBySex() {
Vector rows = dao.findRowsBySex();
Iterator iterator = rows.iterator();
while (iterator.hasNext()) {
System.out.println(iterator.next());
}
}
@Test
public void testDeleteByDepartment() {
String department = "电子工程学院";
int count = dao.deleteByDepartment(department);
if (count > 0) {
System.out.println("恭喜," + department + "的学生记录删除成功!!!");
} else {
System.out.println("What a pity!学生记录删除失败!!!");
}
}
@Test
public void testUpdate() {
StudentDao studentDao = new StudentDaoImpl();
Student student = studentDao.findById("19204196");
System.out.println("更新前:" + student);
student.setAge(20);
student.setTelephone("11111000001");
student.setDepartment("怨种学院");
int count = studentDao.update(student);
if (count > 0) {
System.out.println("更新成功!!!");
System.out.println("更新后:" + studentDao.findById("19204196"));
} else {
System.out.println("What a pity!更新失败!!!");
}
}
@Test
public void testFindById() {
String id = "19204196";
Student student = dao.findById(id);
if (student != null) {
System.out.println("查询到了:" + student);
} else {
System.out.println("What a pity!查询失败!!!");
}
}
@Test
public void testFindByClass() {
String clazz = "2022机电3班";
List<Student> students = dao.findByClass(clazz);
if (students.size() > 0) {
for (Student student : students) {
System.out.println(student);
}
} else {
System.out.println("查询失败!!!");
}
}
@Test
public void testFindByDepartment() {
String department = "GG爆学院";
List<Student> students = dao.findByDepartment(department);
if (students.size() > 0) {
for (Student student : students) {
System.out.println(student);
}
} else {
System.out.println("查询失败!!!");
}
}
@Test
public void testFindRowsByClass() {
Vector rows = dao.findRowsByClass();
Iterator iterator = rows.iterator();
while (iterator.hasNext()) {
System.out.println(iterator.next());
}
}
@Test
public void testFindRowsByDepartment() {
Vector rows = dao.findRowsByDepartment();
Iterator iterator = rows.iterator();
while (iterator.hasNext()) {
System.out.println(iterator.next());
}
}
}
4、创建用户数据访问接口实现类
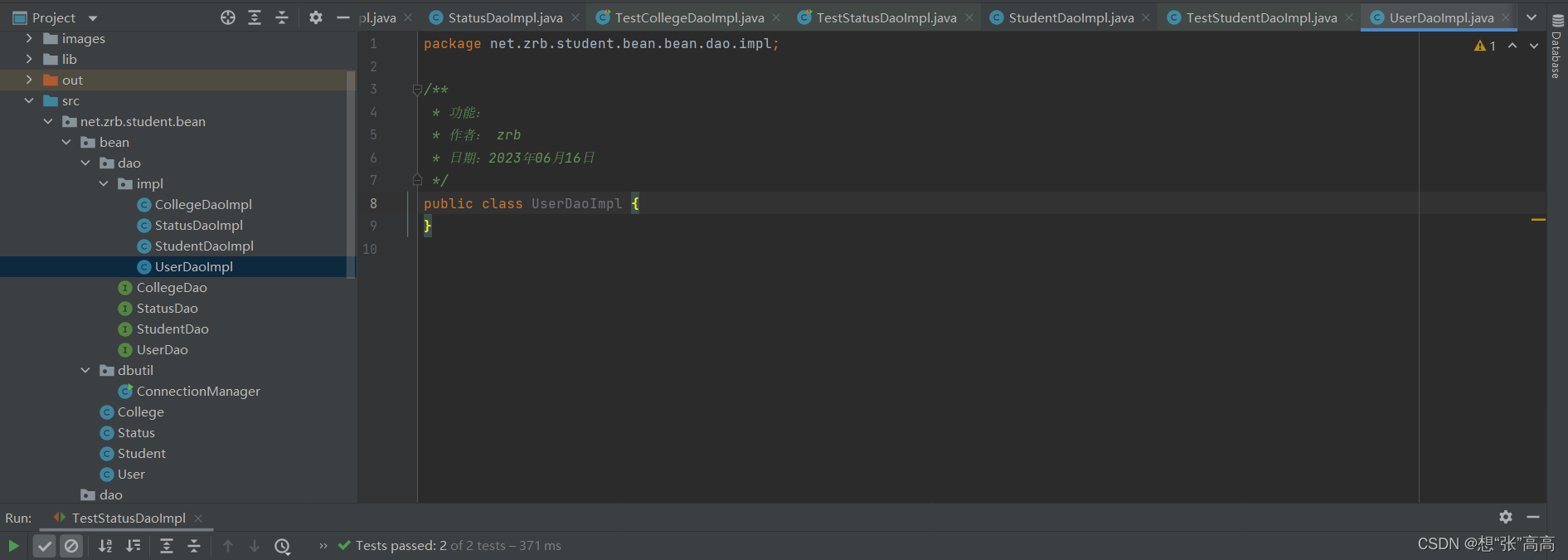
package net.zrb.student.bean.bean.dao.impl;
import net.zrb.student.bean.bean.User;
import net.zrb.student.bean.bean.dao.UserDao;
import net.zrb.student.bean.bean.dbutil.ConnectionManager;
import java.sql.*;
import java.util.ArrayList;
import java.util.List;
public class UserDaoImpl implements UserDao {
@Override
public int insert(User user) {
int count = 0;
Connection conn = ConnectionManager.getConnection();
String strSQL = "INSERT INTO t_user (username, password, telephone, register_time)"
+ " value (?, ?, ?, ?)";
if (!isUsernameExisted(user.getUsername())) {
try {
PreparedStatement pstmt = conn.prepareStatement(strSQL);
pstmt.setString(1, user.getUsername());
pstmt.setString(2, user.getPassword());
pstmt.setString(3, user.getTelephone());
pstmt.setTimestamp(4, new Timestamp(user.getRegisterTime().getTime()));
count = pstmt.executeUpdate();
pstmt.close();
} catch (SQLException e) {
System.err.println(e.getMessage());
} finally {
ConnectionManager.closeConnection(conn);
}
}
return count;
}
@Override
public int deleteById(int id) {
int count = 0;
Connection conn = ConnectionManager.getConnection();
String strSQL = "DELETE FROM t_user WHERE id = ?";
try {
PreparedStatement pstmt = conn.prepareStatement(strSQL);
pstmt.setInt(1, id);
count = pstmt.executeUpdate();
pstmt.close();
} catch (SQLException e) {
System.err.println(e.getMessage());
} finally {
ConnectionManager.closeConnection(conn);
}
return count;
}
@Override
public int update(User user) {
int count = 0;
Connection conn = ConnectionManager.getConnection();
String strSQL = "UPDATE t_user SET username = ?, password = ?, telephone = ?,"
+ "register_time = ? WHERE id = ?";
try {
PreparedStatement pstmt = conn.prepareStatement(strSQL);
pstmt.setString(1, user.getUsername());
pstmt.setString(2, user.getPassword());
pstmt.setString(3, user.getTelephone());
pstmt.setTimestamp(4, new Timestamp(user.getRegisterTime().getTime()));
count = pstmt.executeUpdate();
pstmt.close();
} catch (SQLException e) {
System.err.println(e.getMessage());
} finally {
ConnectionManager.closeConnection(conn);
}
return count;
}
@Override
public User findById(int id) {
User user = null;
Connection conn = ConnectionManager.getConnection();
String strSQL = "SELECT * FROM t_user WHERE id = ?";
try {
PreparedStatement pstmt = conn.prepareStatement(strSQL);
pstmt.setInt(1, id);
ResultSet rs = pstmt.executeQuery();
if (rs.next()) {
user = new User();
user.setId(rs.getInt("id"));
user.setUsername(rs.getString("username"));
user.setPassword(rs.getString("password"));
user.setTelephone(rs.getString("telephone"));
user.setRegisterTime(rs.getTimestamp("register_time"));
}
pstmt.close();
} catch (SQLException e) {
System.err.println(e.getMessage());
} finally {
ConnectionManager.closeConnection(conn);
}
return user;
}
@Override
public List<User> findAll() {
List<User> users = new ArrayList<User>();
Connection conn = ConnectionManager.getConnection();
String strSQL = "SELECT * FROM t_user";
try {
Statement stmt = conn.createStatement();
ResultSet rs = stmt.executeQuery(strSQL);
while (rs.next()) {
User user = new User();
user.setId(rs.getInt("id"));
user.setUsername(rs.getString("username"));
user.setPassword(rs.getString("password"));
user.setTelephone(rs.getString("telephone"));
user.setRegisterTime(rs.getTimestamp("register_time"));
users.add(user);
}
rs.close();
stmt.close();
} catch (SQLException e) {
System.err.println(e.getMessage());
} finally {
ConnectionManager.closeConnection(conn);
}
return users;
}
@Override
public User login(String username, String password) {
User user = null;
Connection conn = ConnectionManager.getConnection();
String strSQL = "SELECT * FROM t_user WHERE username = ? and password = ?";
try {
PreparedStatement pstmt = conn.prepareStatement(strSQL);
pstmt.setString(1, username);
pstmt.setString(2, password);
ResultSet rs = pstmt.executeQuery();
if (rs.next()) {
user = new User();
user.setId(rs.getInt("id"));
user.setUsername(rs.getString("username"));
user.setPassword(rs.getString("password"));
user.setTelephone(rs.getString("telephone"));
user.setRegisterTime(rs.getTimestamp("register_time"));
}
pstmt.close();
} catch (SQLException e) {
System.err.println(e.getMessage());
} finally {
ConnectionManager.closeConnection(conn);
}
return user;
}
@Override
public boolean isUsernameExisted(String username) {
boolean existed = false;
Connection conn = ConnectionManager.getConnection();
String strSQL = "SELECT * FROM t_user WHERE username = ?";
try {
PreparedStatement pstmt = conn.prepareStatement(strSQL);
pstmt.setString(1, username);
ResultSet rs = pstmt.executeQuery();
if (rs.next()) {
existed = true;
}
pstmt.close();
rs.close();
} catch (SQLException e) {
System.err.println(e.getMessage());
} finally {
ConnectionManager.closeConnection(conn);
}
return existed;
}
}
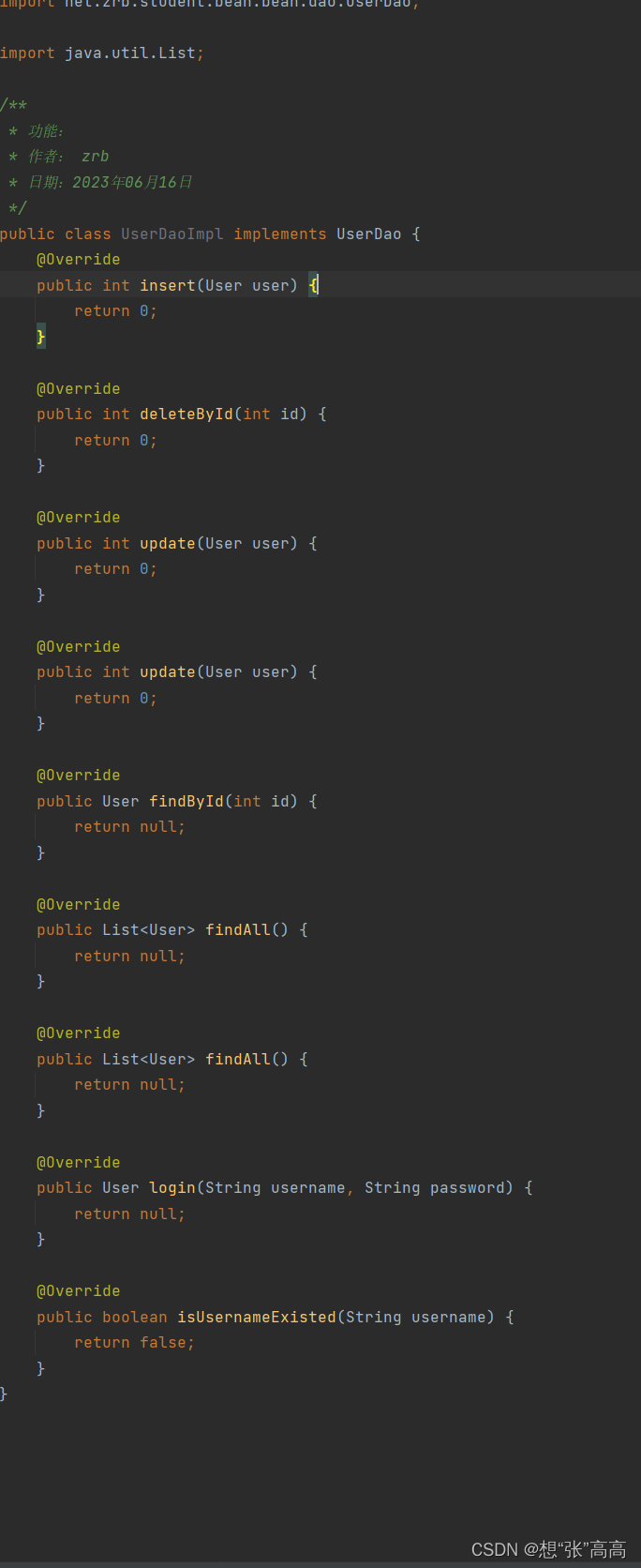
(1)编写插入用户名是否存在方法
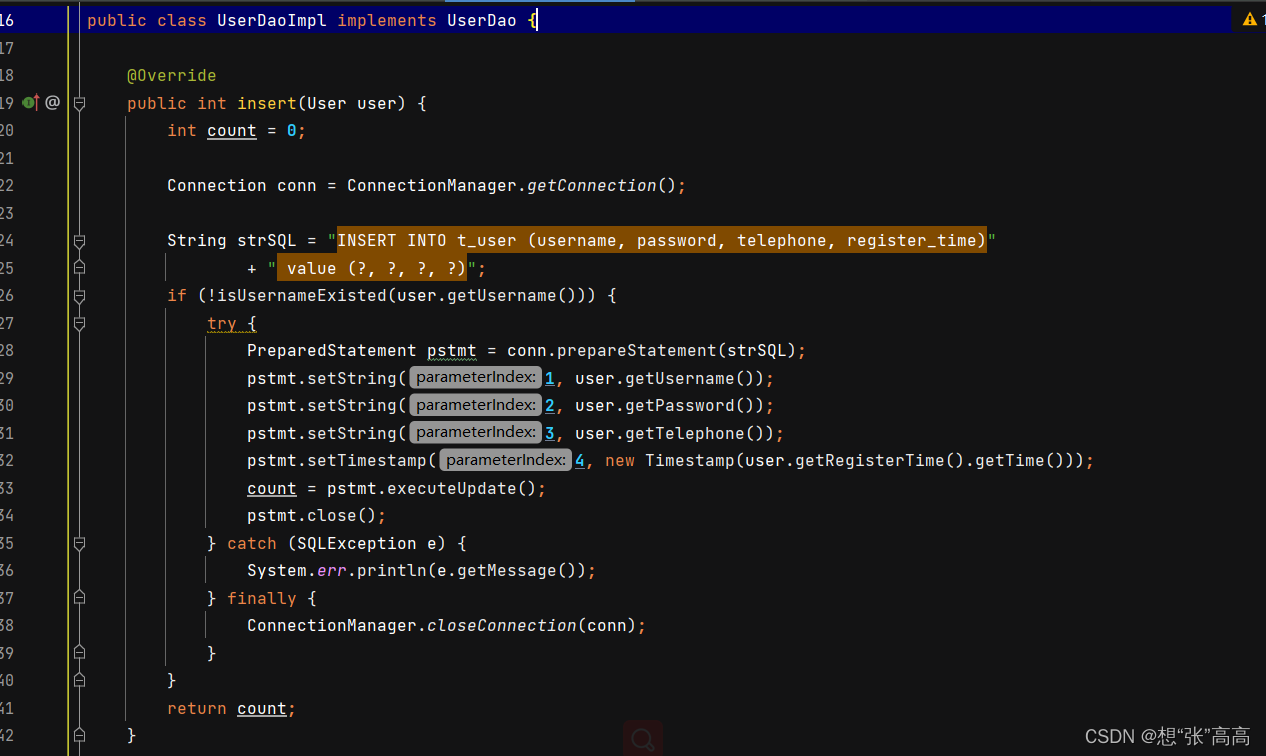
@Override
public int insert(User user) {
int count = 0;
Connection conn = ConnectionManager.getConnection();
String strSQL = "INSERT INTO t_user (username, password, telephone, register_time)"
+ " value (?, ?, ?, ?)";
if (!isUsernameExisted(user.getUsername())) {
try {
PreparedStatement pstmt = conn.prepareStatement(strSQL);
pstmt.setString(1, user.getUsername());
pstmt.setString(2, user.getPassword());
pstmt.setString(3, user.getTelephone());
pstmt.setTimestamp(4, new Timestamp(user.getRegisterTime().getTime()));
count = pstmt.executeUpdate();
pstmt.close();
} catch (SQLException e) {
System.err.println(e.getMessage());
} finally {
ConnectionManager.closeConnection(conn);
}
}
return count;
}
4_、测试用户数据访问接口实现类
(1)测试类
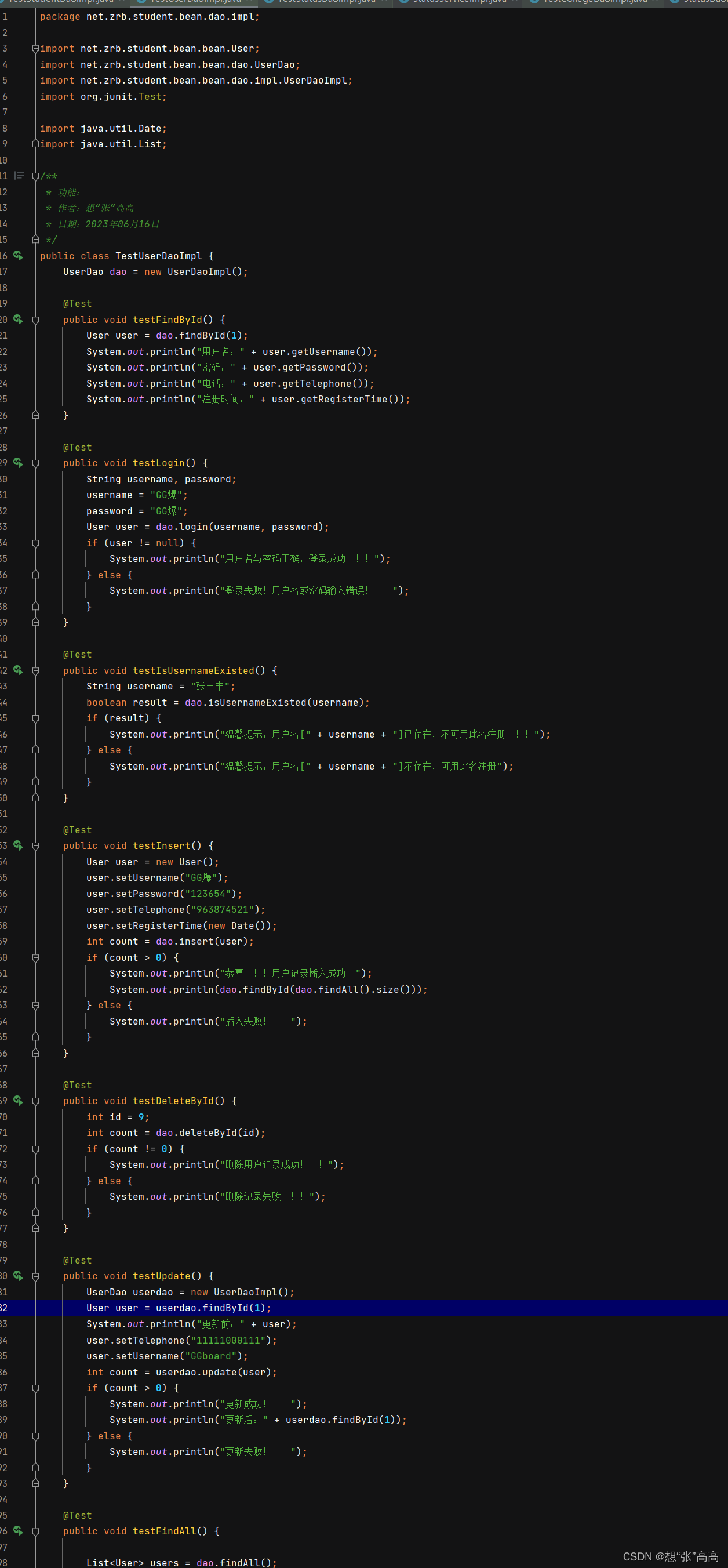
package net.zrb.student.bean.dao.impl;
import net.zrb.student.bean.bean.User;
import net.zrb.student.bean.bean.dao.UserDao;
import net.zrb.student.bean.bean.dao.impl.UserDaoImpl;
import org.junit.Test;
import java.util.Date;
import java.util.List;
public class TestUserDaoImpl {
UserDao dao = new UserDaoImpl();
@Test
public void testFindById() {
User user = dao.findById(1);
System.out.println("用户名:" + user.getUsername());
System.out.println("密码:" + user.getPassword());
System.out.println("电话:" + user.getTelephone());
System.out.println("注册时间:" + user.getRegisterTime());
}
@Test
public void testLogin() {
String username, password;
username = "GG爆";
password = "GG爆";
User user = dao.login(username, password);
if (user != null) {
System.out.println("用户名与密码正确,登录成功!!!");
} else {
System.out.println("登录失败!用户名或密码输入错误!!!");
}
}
@Test
public void testIsUsernameExisted() {
String username = "张三丰";
boolean result = dao.isUsernameExisted(username);
if (result) {
System.out.println("温馨提示:用户名[" + username + "]已存在,不可用此名注册!!!");
} else {
System.out.println("温馨提示:用户名[" + username + "]不存在,可用此名注册");
}
}
@Test
public void testInsert() {
User user = new User();
user.setUsername("GG爆");
user.setPassword("123654");
user.setTelephone("963874521");
user.setRegisterTime(new Date());
int count = dao.insert(user);
if (count > 0) {
System.out.println("恭喜!!!用户记录插入成功!");
System.out.println(dao.findById(dao.findAll().size()));
} else {
System.out.println("插入失败!!!");
}
}
@Test
public void testDeleteById() {
int id = 9;
int count = dao.deleteById(id);
if (count != 0) {
System.out.println("删除用户记录成功!!!");
} else {
System.out.println("删除记录失败!!!");
}
}
@Test
public void testUpdate() {
UserDao userdao = new UserDaoImpl();
User user = userdao.findById(1);
System.out.println("更新前:" + user);
user.setTelephone("11111000111");
user.setUsername("GGboard");
int count = userdao.update(user);
if (count > 0) {
System.out.println("更新成功!!!");
System.out.println("更新后:" + userdao.findById(1));
} else {
System.out.println("更新失败!!!");
}
}
@Test
public void testFindAll() {
List<User> users = dao.findAll();
for (User user : users) {
System.out.println(user);
}
}